Navigating the Pitfalls of Default Methods in Interfaces
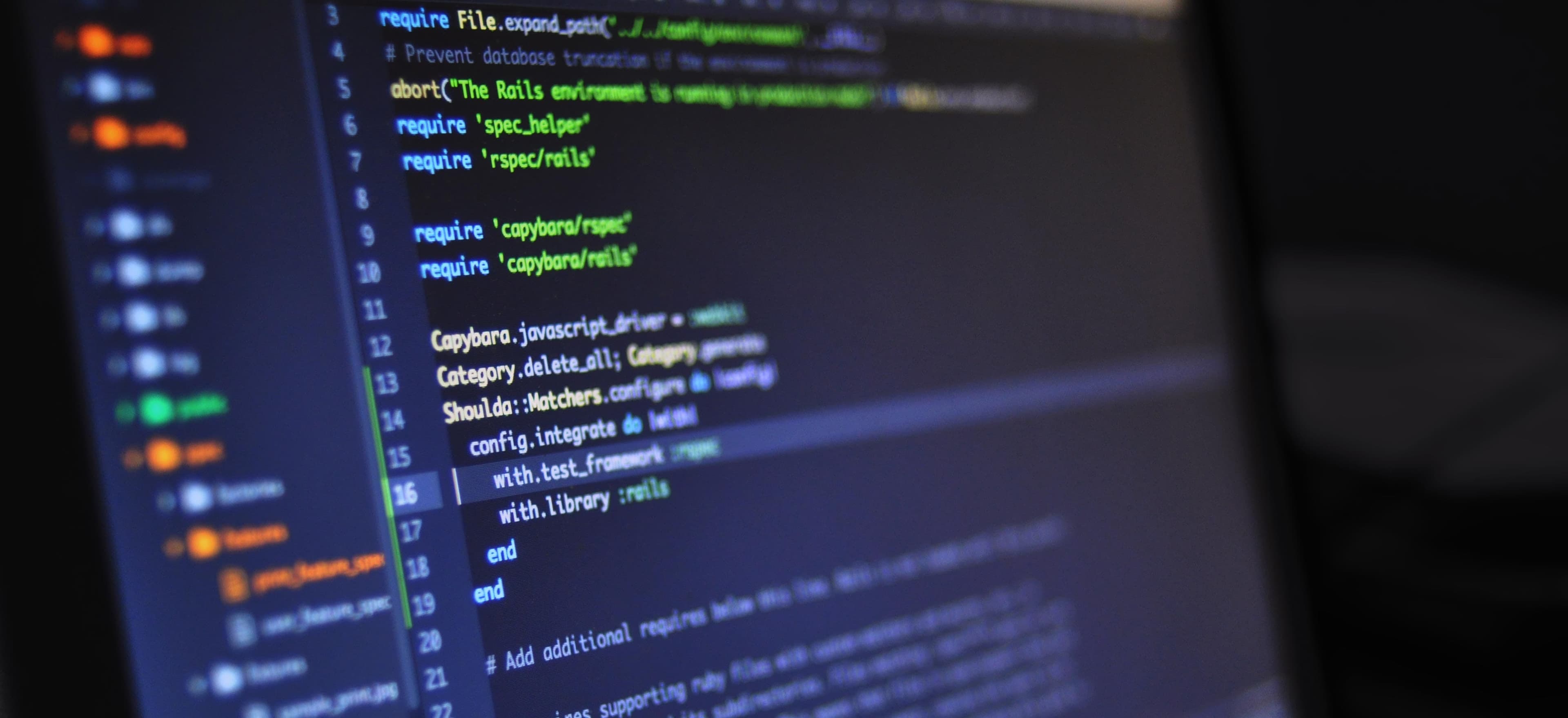
- Published on
Navigating the Pitfalls of Default Methods in Interfaces in Java
In the evolving landscape of Java programming, interfaces have undergone significant changes, especially with the introduction of default methods in Java 8. This feature brought a new level of flexibility to interfaces, allowing developers to add methods with default implementations. However, with great power comes great responsibility. Default methods can lead to several pitfalls if not handled appropriately. In this blog post, we will dissect default methods in interfaces, explore some common pitfalls, and provide strategies to navigate them.
What are Default Methods?
Before diving into the complications, it is crucial to understand what default methods are. A default method is a method defined in an interface that has a body. This means that classes implementing the interface do not need to provide an implementation for the method unless they want to override it.
Example of Default Method
Here’s a straightforward example:
public interface Greeting {
// Default method
default void greet() {
System.out.println("Hello from the Greeting interface!");
}
}
public class Greeter implements Greeting {
// No need to implement greet() unless overriding
}
public class Main {
public static void main(String[] args) {
Greeter greeter = new Greeter();
greeter.greet(); // Outputs: Hello from the Greeting interface!
}
}
In this example, Greeter
class automatically inherits the greet
method without needing to implement it.
Advantages of Default Methods
Default methods provide benefits such as:
- Backward Compatibility: Default methods allow developers to add new methods to interfaces without breaking existing implementations.
- Code Reusability: They promote code reuse, reducing boilerplate in implementing classes.
- Extended Functionality: Default methods facilitate the addition of new behavior to interfaces, enhancing their expressiveness.
Pitfalls of Default Methods
While default methods offer significant advantages, they come with their own set of complications. Below are the major pitfalls associated with implementing default methods in interfaces:
1. Ambiguous Method Resolution
One of the most common pitfalls is ambiguous method resolution. If a class implements multiple interfaces that contain a default method with the same name, the compiler will raise an ambiguity error.
Conflict Example
Consider the following scenario:
public interface A {
default void commonMethod() {
System.out.println("From interface A");
}
}
public interface B {
default void commonMethod() {
System.out.println("From interface B");
}
}
public class MyClass implements A, B {
@Override
public void commonMethod() {
// Resolve ambiguity by overriding
A.super.commonMethod(); // Calling A's method
B.super.commonMethod(); // Calling B's method
}
}
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.commonMethod();
}
}
Why Ambiguity Occurs
In this code, MyClass
implements both interfaces A
and B
, which have the identical method commonMethod()
. Since both interfaces provide a default implementation, the compiler does not inherently know which method to call, leading to ambiguity.
2. Increased Complexity
Adding default methods to interfaces can lead to increased complexity. When an interface has multiple default methods that can be overridden in a class with its own methods, it can make the design less clear.
3. Violation of Interface Segregation Principle
The introduction of default methods can lead to bulky interfaces, violating the Interface Segregation Principle (ISP). ISP advocates for smaller, more focused interfaces. With default methods, developers may be tempted to add utility methods, making interfaces too large.
4. Confusion Over Implementation
Using default methods can sometimes confuse developers, especially those new to Java. They might not immediately grasp when to implement a method versus when to use a default method. This confusion can lead to inconsistencies in code quality and maintainability.
Navigating the Pitfalls
Now that we've discussed some potential pitfalls, how can you navigate these waters safely? Here are some strategies:
1. Use Default Methods Sparingly
Limit the use of default methods to situations where they add clear value. Avoid overloading interfaces with numerous default methods that may confuse users and lead to maintenance challenges.
2. Documentation is Key
Document default methods thoroughly. Explain what they do and how they should be used. Providing clear guidelines will help other developers understand and work with these methods effectively.
3. Consider Composition Over Inheritance
Instead of relying heavily on interfaces with default methods, consider using composition as an alternative. By favoring composition, you can manage behavior more explicitly without the ambiguities default methods may introduce.
4. Be Explicit in Method Resolution
When facing ambiguities, always make explicit choices in your implementations, as shown in the ambiguous method resolution example. Specify which default method to use by leveraging the InterfaceName.super.methodName()
syntax.
5. Design Thoughtfully
Apply the Interface Segregation Principle rigorously. Design small, focused interfaces that do not try to do too much. If an interface is doing a lot, it likely needs to be split up.
Lessons Learned
Default methods in Java interfaces can be powerful tools, enhancing flexibility and maintaining backward compatibility. However, they also introduce complexities and potential pitfalls that developers must navigate carefully. By adhering to careful design practices, using documentation effectively, and favoring simplicity, you can harness the advantages of default methods while mitigating their risks.
For further reading on Java interfaces and design principles, consider looking at the following resources:
Whether you are a seasoned Java developer or just starting out, understanding how to use default methods wisely can lead to cleaner, more maintainable code. Happy coding!
Checkout our other articles