Why Java Developers Struggle with Trusted Timestamping
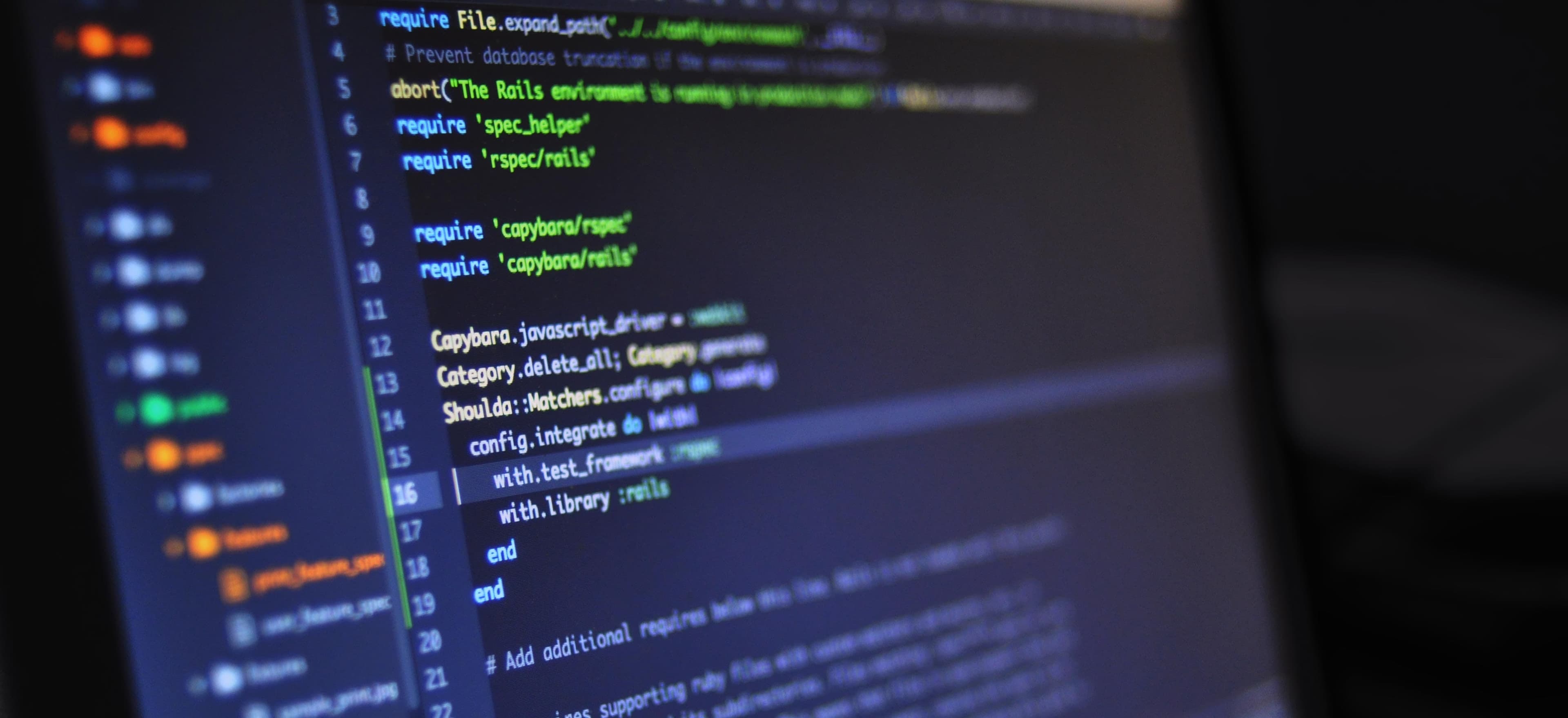
- Published on
Why Java Developers Struggle with Trusted Timestamping
As technology evolves, the need for secure and reliable systems has never been greater. One area where many developers seek to enhance their applications is trusted timestamping. Trusted timestamping assures that a specific piece of data was created or modified at a specific point in time. This post explores why Java developers face challenges when implementing trusted timestamping, delves into the technical complexities, and offers solutions to streamline the process.
The Importance of Trusted Timestamping
Before attempting to address these challenges, let us first understand why trusted timestamping is crucial. In situations where data integrity and security are paramount—such as in legal documents, financial transactions, or sensitive health records—timestamping serves as not just a personal assurance but also a legal safeguard. It provides proof that data existed at a given moment, which can be pivotal in disputes or investigations.
Key Benefits of Trusted Timestamping
- Data Integrity: Ensures that files have not been altered since the timestamp was applied.
- Legal Security: Serves as proof of existence in case of disputes.
- Reputation Management: Builds trust with users through secure practices.
Challenges in Implementing Trusted Timestamping
Java is one of the most widely used programming languages, known for its versatility and platform independence. However, several challenges faced by Java developers in trusted timestamping can complicate its implementation:
1. Complexity of Cryptographic Standards
Trusted timestamping relies heavily on cryptographic principles. Java provides robust libraries like Bouncy Castle for cryptographic operations, but they can be complex to use.
Example Code Snippet
Here’s a basic example of generating a hash using Bouncy Castle:
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import java.security.MessageDigest;
import java.security.Security;
public class HashExample {
public static byte[] getSHA256Hash(String data) throws Exception {
Security.addProvider(new BouncyCastleProvider());
MessageDigest digest = MessageDigest.getInstance("SHA-256");
return digest.digest(data.getBytes("UTF-8"));
}
public static void main(String[] args) throws Exception {
String data = "Hello, Trusted Timestamping!";
byte[] hash = getSHA256Hash(data);
System.out.println(bytesToHex(hash));
}
public static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
Why This Matters: This snippet hashes data with SHA-256, a cryptographic function essential for ensuring data integrity. However, the complexity of integrating such cryptographic functions often stymies developers who lack experience in security practices.
2. Lack of Built-in Support
Unlike other programming languages that may provide built-in timestamping functionalities, Java developers frequently depend on external libraries or APIs, which can lead to inconsistencies.
Example Code Snippet
Using an external API, here's how a developer might request a trusted timestamp from a Timestamp Authority (TSA):
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class TrustedTimestamp {
public static void main(String[] args) {
try (CloseableHttpClient client = HttpClients.createDefault()) {
HttpPost post = new HttpPost("https://tsa.example.com/timestamp");
// Add required headers and payload
// Execute the request and handle the response
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters: While using an external TSA can simplify the implementation, it requires careful error-handling and validation to prevent connectivity issues or unauthorized access, which are always concerns in a network-based solution.
3. Knowledge Gap in Security Practices
Many Java developers may not have expertise in security practices, making it difficult to implement timestamping that meets industry standards.
Example Code Snippet
Improper encryption can lead to vulnerabilities. An example of poor key management is shown below.
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class KeyManagement {
public static void main(String[] args) throws Exception {
// Poor Key Management (Demonstration)
SecretKey key = KeyGenerator.getInstance("AES").generateKey();
// Key stored insecurely
System.out.println(key);
}
}
Why This Matters: The above code demonstrates a naive approach to key management where keys are not securely stored or handled. Strong security practices should be an integral part of any timestamping solution.
4. Integration with Existing Systems
Incorporating timestamping into legacy systems can pose significant challenges due to differing architectures or outdated frameworks.
Integrating with existing systems often requires:
- Refactoring or rewriting parts of the code to accommodate newer libraries.
- Ensuring backward compatibility to maintain functionality.
- Testing thoroughly to prevent introducing bugs.
Best Practices for Implementing Trusted Timestamping in Java
Here are some best practices and techniques Java developers can use to overcome these challenges:
Utilize Established Libraries
Invest in well-documented libraries such as Bouncy Castle and Java Cryptography Architecture (JCA). They simplify cryptographic operations and provide much-needed utility functions.
Secure Key Management
Use secure storage solutions, such as Java’s KeyStore or third-party libraries, to handle cryptographic keys. This prevents unauthorized access and reduces the risk of key compromise.
import java.security.KeyStore;
public class SecureKeyStore {
public static void main(String[] args) throws Exception {
KeyStore keyStore = KeyStore.getInstance("JKS");
keyStore.load(null, null); // Load your keystore
// Handle keys securely
}
}
Use Trusted Timestamp Authorities (TSA)
Select a reliable Timestamp Authority that adheres to international standards. Using standard formats such as CAdES (CMS Advanced Electronic Signature) ensures compatibility and reliability.
Testing and Validation
Conduct thorough unit testing and integration testing to catch possible issues before deploying any solution.
- Automated Tests: Consider adding automated tests using JUnit or TestNG to validate functionality.
- Security Audits: Regular security reviews of your codebase will help identify vulnerabilities early.
To Wrap Things Up
Trusted timestamping is vital for ensuring data integrity and security, yet Java developers often face various challenges in its implementation. By understanding these hurdles—from cryptographic complexities and integration issues to knowledge gaps in security practices—developers can better prepare themselves.
Adopting best practices, such as utilizing established libraries and focused testing methodologies, can mitigate these issues. Consequently, Java applications can operate with the confidence that their data is protected and that they meet the necessary legal requirements.
Additional Resources
- Bouncy Castle
- Java Cryptography Architecture
- CAdES Specification
Navigating the world of trusted timestamping can be challenging, but with a solid understanding and the right tools, Java developers can successfully implement it in their applications, ultimately enhancing their product's reliability and trustworthiness.
Checkout our other articles