Java 8 Streams: Common Pitfalls Affecting Performance
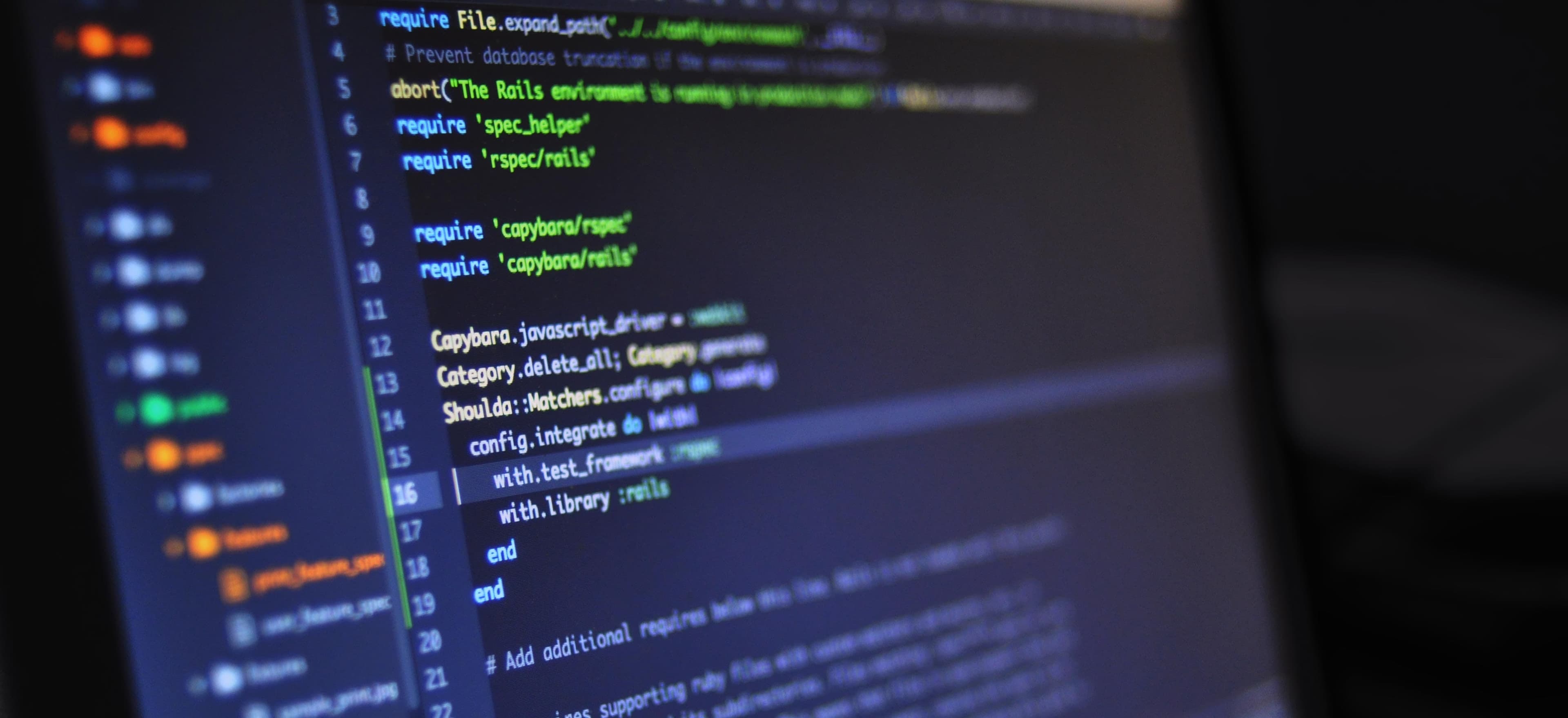
- Published on
Java 8 Streams: Common Pitfalls Affecting Performance
With the introduction of Java 8, streams have become a crucial feature for handling collections in a more functional style. While they offer certain conveniences and efficiency improvements, they also come with some common pitfalls that can negatively affect performance.
In this article, we will explore these pitfalls, give code examples, and explain why they matter. By the end, you'll not only understand how to avoid these issues but also be better equipped to write efficient Java stream code.
What are Java Streams?
Java streams are sequences of elements that support various methods which can be pipelined to produce a desired result. They are designed to work in a functional style, allowing for operations such as filtering, mapping, and collecting. This brings about clearer and more concise code.
The stream API can significantly enhance performance, especially when dealing with large data sets. However, misuse or misunderstandings can lead to unexpected performance issues.
Key Pitfalls Affecting Java Stream Performance
Let’s dive into some of the most common pitfalls with Java Streams and how to avoid them.
1. Creating Streams in a Non-Optimal Way
Creating a stream should be a straightforward task, yet developers often overlook the importance of how they initialize streams.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Optimal way to create a stream
Stream<String> nameStream = names.stream();
Why this matters: Avoid creating a stream from a collection every time you need to process it. Instead, create it once and reuse it. If you repeatedly create streams from a collection like shown below, it could lead to performance issues.
List<String> allNames = new ArrayList<>(...);
for (int i = 0; i < 1000; i++) {
allNames.stream().filter(name -> name.startsWith("A")).forEach(System.out::println);
}
Recommendation: Always prefer to create your streams once and process them multiple times when necessary.
2. Not Using Parallel Streams Correctly
Java 8 allows the use of parallel streams for better utilization of multi-core processors. However, blindly using parallel streams can lead to performance degradation.
List<Integer> numberList = Arrays.asList(1, 2, 3, ..., 1_000_000);
// Incorrect use of parallel streams
numberList.parallelStream().map(n -> n * 2).forEach(System.out::println);
Why this matters: The overhead associated with splitting tasks can outweigh the benefits if the data set is small or the operations are light in computation.
Recommendation: Measure performance. Use a parallel stream when the dataset is large and operations are computationally expensive.
3. Using Stateful Operations in Streams
Stateful operations, such as sorted() or distinct(), can cause performance issues, especially in parallel streams. This is because they require storing the state of the elements, which can be costly.
List<String> list = Arrays.asList("B", "C", "A", "D", "C");
// Inefficient usage causing potential performance setback
list.stream()
.distinct() // Stateful operation
.sorted() // Another stateful operation
.forEach(System.out::println);
Why this matters: Stateful operations particularly slow down processing because they require maintaining a state across all elements.
Recommendation: Try to minimize the number of stateful operations in your pipelines. If you must use them, position them wisely; consider performing filtering operations first.
4. Collecting Too Early
One common mistake is collecting intermediate results too early. This can lead to unnecessary overhead, especially if the results are not needed.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Collecting too early before filtering
List<String> filtered = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Why this matters: By collecting too early, you prevent further optimizations that the stream can perform.
Recommendation: Only collect when absolutely necessary, and design your stream operations to defer collection until the very end.
5. Overusing Intermediate Operations
While streams encourage a functional style, overusing intermediate operations can lead to performance bottlenecks.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Overusing intermediate operations
numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.distinct()
.forEach(System.out::println);
Why this matters: Every intermediate operation creates a new stream pipeline. Too many operations can lead to unnecessary computation.
Recommendation: Favor combining operations where possible and minimize extraneous intermediate steps.
Summary of Performance Tips
- Create streams once and reuse them when possible.
- Use parallel streams judiciously, only when the dataset is appropriately large.
- Avoid stateful operations where possible, and minimize their usage.
- Defer collection until it’s absolutely necessary; optimizing on the fly can reduce overhead.
- Minimize intermediate operations and prefer combined operations.
Wrapping Up
Understanding how to effectively use streams in Java 8 is crucial for writing efficient and maintainable code. By being aware of these common pitfalls and their implications on performance, developers can take advantage of the power of streams without incurring unnecessary overhead.
For further reading, consider exploring these links:
- Java Stream API Documentation
- Utmost Guide to Java 8 Streams
- Understanding Java Parallel Streams
By keeping these tips in mind, you can elevate your Java programming skills and ensure that your applications run smoothly and efficiently. Happy coding!