Boost Your Android App: 5 Performance Pitfalls to Avoid
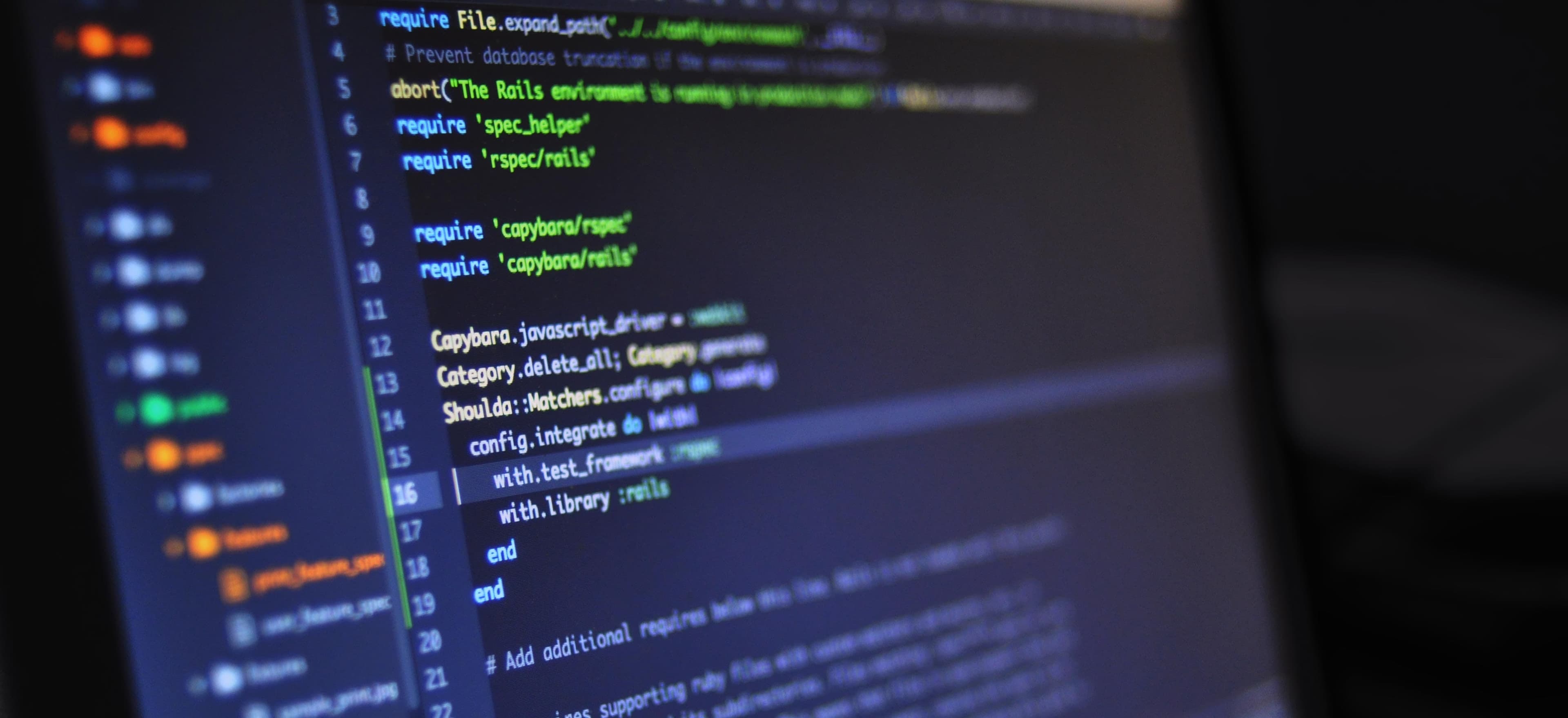
- Published on
Boost Your Android App: 5 Performance Pitfalls to Avoid
Building Android applications is an exciting and rewarding challenge for any developer. However, your app's success depends not just on its features but also on performance. A slow or unresponsive app could drive users away, no matter how great its functionality is. To help you ensure your Android app runs smoothly, we're diving into five common performance pitfalls to avoid. Each pitfall will include tips and best practices to enhance your app’s performance.
1. Memory Leaks
The Issue
Memory leaks occur when an app holds references to objects it no longer needs. This can quickly lead to increased memory usage, causing slowdowns and even crashes.
The Solution
Utilizing tools such as Android Profiler can help you detect memory leaks. Additionally, using the following code snippet can effectively mitigate memory leaks:
public class MainActivity extends AppCompatActivity {
private SomeObject someObject; // Potential for memory leak
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
someObject = new SomeObject(this);
// ...
}
@Override
protected void onDestroy() {
super.onDestroy();
someObject.cleanup(); // Release resources here
someObject = null; // Clear reference
}
}
Why?
By cleaning up references in the onDestroy
method, you significantly reduce the risk of memory leaks. This practice ensures that your app frees up resources when they are no longer needed, ultimately leading to improved performance.
2. Inefficient Layouts
The Issue
Using deep and complex layouts can lead to excessive processing requirements, causing UI thread blocking. This results in slow rendering and an unresponsive interface.
The Solution
Using the ConstraintLayout can help you flatten your view hierarchy. Here’s an example of replacing a nested layout setup with a more efficient one:
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/title"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Hello World"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
app:layout_constraintTop_toBottomOf="@id/title"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Why?
ConstraintLayout allows you to create complex layouts without the need for nested ViewGroups, which can slow down the rendering process. By flattening hierarchies, your app can render faster and consume less memory.
3. Excessive Overuse of Background Operations
The Issue
While background tasks are necessary for performing tasks without blocking the UI, improper use can lead to over-consumption of CPU and battery.
The Solution
Utilize WorkManager for tasks that require guaranteed completion. Here’s how you can set it up:
public class MyWorker extends Worker {
public MyWorker(@NonNull Context context, @NonNull WorkerParameters workerParams) {
super(context, workerParams);
}
@NonNull
@Override
public Result doWork() {
// Do the background task here
// ...
return Result.success();
}
}
// Enqueue the task
WorkManager.getInstance(context)
.enqueue(new OneTimeWorkRequest.Builder(MyWorker.class).build());
Why?
Using WorkManager allows Android to schedule your work optimally, thereby preventing it from running too frequently or holding too many resources simultaneously. This enhances your app's responsiveness while maintaining battery performance.
4. Doing Too Much on the UI Thread
The Issue
The UI thread is responsible for user interactions. Performing heavy tasks here can lead to a complete freeze of your app, negatively affecting user experience.
The Solution
Offload intensive operations onto background threads using AsyncTask (or alternatives like LiveData with coroutines). Here’s how:
new AsyncTask<Void, Void, String>() {
@Override
protected String doInBackground(Void... voids) {
// Simulate heavy task, e.g., fetching data from a server
return fetchData();
}
@Override
protected void onPostExecute(String result) {
// Update UI with result
textView.setText(result);
}
}.execute();
Why?
By utilizing AsyncTask, you ensure long-running operations do not block the UI thread. This separation of operations allows your app to remain responsive, improving user experience significantly.
5. Forgetting About Network Calls
The Issue
Relying on synchronous network calls can freeze your app, hindering user experience. Users expect smooth functioning, even when your app communicates with external servers.
The Solution
Always perform network operations asynchronously. Here's an example using Retrofit, a popular library for network calls:
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
MyApiService apiService = retrofit.create(MyApiService.class);
Call<Item> call = apiService.getItem();
call.enqueue(new Callback<Item>() {
@Override
public void onResponse(Call<Item> call, Response<Item> response) {
if (response.isSuccessful()) {
// Update UI with fetched data
}
}
@Override
public void onFailure(Call<Item> call, Throwable t) {
// Handle error
}
});
Why?
Using asynchronous callbacks allows your app to handle network responses cleanly, preventing the UI from freezing while waiting for data retrieval. This enhances user experience by ensuring quick access to app functionalities.
In Conclusion, Here is What Matters
Performance optimization is a critical aspect of Android development. Avoiding these five common pitfalls can help you ensure your application performs effectively, is responsive, and delivers a pleasant user experience.
For further insights, consider checking out Android Performance Patterns to improve performance further and stay updated on best practices.
By being proactive in addressing common issues, you stand to build an app that not only meets user expectations but exceeds them, leading to a higher retention rate and overall success.
Happy coding!