Overcoming Common Pitfalls in Custom Spring Security
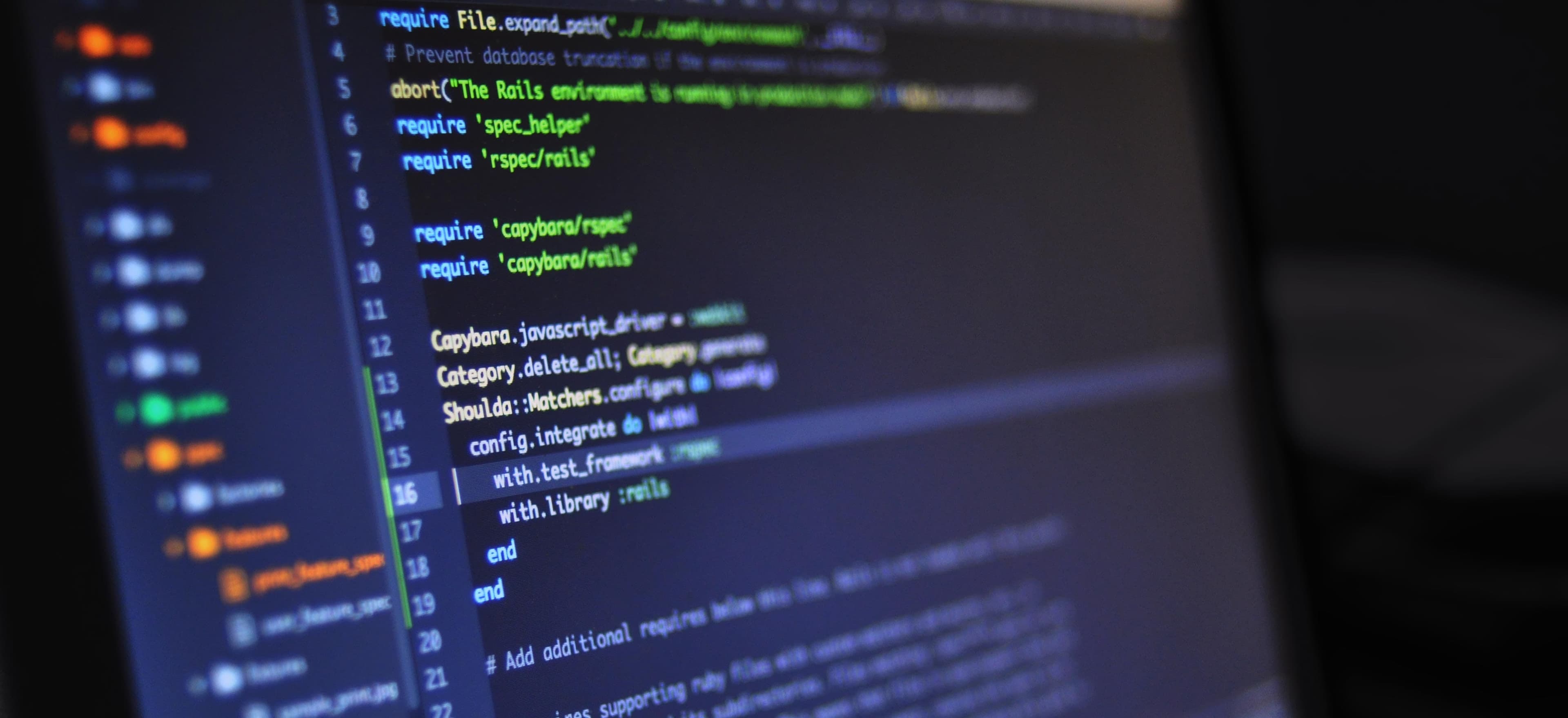
- Published on
Overcoming Common Pitfalls in Custom Spring Security
Spring Security is a powerful and customizable authentication and access-control framework for Java applications. By leveraging its capabilities, developers can manage security for their applications effectively. However, it is not uncommon to encounter pitfalls when customizing it to meet specific application requirements. This blog post will guide you through some common pitfalls and how to overcome them, ensuring robust security for your Java applications.
What is Spring Security?
Before diving into the pitfalls, let’s briefly discuss what Spring Security does. Essentially, it provides comprehensive security services for Spring applications. It manages authentication, authorization, and even safeguards against common vulnerabilities like CSRF (Cross-Site Request Forgery) and XSS (Cross-Site Scripting).
Common Pitfalls in Custom Spring Security
1. Ignoring Default Security Settings
Why This is a Pitfall
Spring Security comes with a host of default settings that are designed to protect your application. Ignoring these can leave your application vulnerable to threats.
How to Overcome It
Review the default settings provided by Spring Security and understand their implications. You can always extend or override default configurations in your WebSecurityConfigurerAdapter
.
Here’s an example of a typical Spring Security configuration:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll() // Allow unrestricted access to public resources
.anyRequest().authenticated() // All other requests need authentication
.and()
.formLogin() // Enables form-based login
.permitAll()
.and()
.logout() // Enables logout
.permitAll();
}
}
In this configuration, the use of .permitAll()
for public endpoints allows unauthenticated users access, while anyRequest().authenticated()
ensures that all other endpoints require authentication.
2. Over-complicating Security Configuration
Why This is a Pitfall
While customizing security is essential, becoming overly complicated can make your application hard to maintain and debug.
How to Overcome It
Aim for simplicity. Start with standard configurations and only add complexities as needed. A clear, well-commented configuration is easier to understand.
For example, consider a simple URL-based authorization method:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // Only admins can access
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN") // Users and admins can access
.antMatchers("/", "/home").permitAll() // Allow access to home route for everyone
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.logoutSuccessUrl("/home")
.permitAll();
}
This configuration clearly defines access roles while maintaining readability.
3. Failing to Secure REST APIs
Why This is a Pitfall
In a microservices architecture or applications heavily reliant on APIs, it’s vital to secure REST endpoints. Failing to do so can expose your service to attacks.
How to Overcome It
Implement token-based authentication, commonly using JWT (JSON Web Tokens). Here is an example of how to secure a REST endpoint with JWT:
@RestController
@RequestMapping("/api/v1")
public class ApiController {
@GetMapping("/secure-data")
@PreAuthorize("hasRole('USER')") // Ensures that only users with a role can access this endpoint
public ResponseEntity<String> getSecureData() {
return ResponseEntity.ok("This is secured data available to only authorized users.");
}
}
Using @PreAuthorize
allows fine-grained control over access at the method level.
4. Inadequate Error Handling
Why This is a Pitfall
Verbose error messages can lead to security vulnerabilities, as they may reveal sensitive information about the application’s structure.
How to Overcome It
Customize your error responses. Ensure that your application does not display stack traces or detailed error messages to end users. Here's how to handle access denied exceptions:
import org.springframework.security.access.AccessDeniedException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(AccessDeniedException.class)
@ResponseStatus(HttpStatus.FORBIDDEN)
public ResponseEntity<String> handleAccessDenied() {
return ResponseEntity.status(HttpStatus.FORBIDDEN)
.body("You do not have permission to access this resource.");
}
}
By providing a global exception handler, you ensure users receive friendly error messages without exposing sensitive system information.
5. Not Conducting Adequate Testing
Why This is a Pitfall
Security issues can remain hidden without thorough testing, leading to serious vulnerabilities.
How to Overcome It
Conduct regular tests, including penetration testing and code reviews. Employ tools like OWASP ZAP to check for vulnerabilities and perform automated security testing.
Additional Resources
For more in-depth information on securing your applications, consider checking the Spring Security Reference Documentation and OWASP Top Ten Project for a list of common security vulnerabilities and their mitigations.
Final Thoughts
Customizing Spring Security can indeed be a challenging endeavor, yet it is equally essential for safeguarding your application. By being aware of common pitfalls and taking a proactive approach when addressing them, you can develop a more secure Java application. Remember to continuously refine your security practices and stay updated with the latest security trends.
Now armed with this knowledge, go ahead and ensure your applications are equipped with the best security practices that Spring Security offers. Happy coding!