Fixing SWT Listener Notification Issues with SWTEventHelper
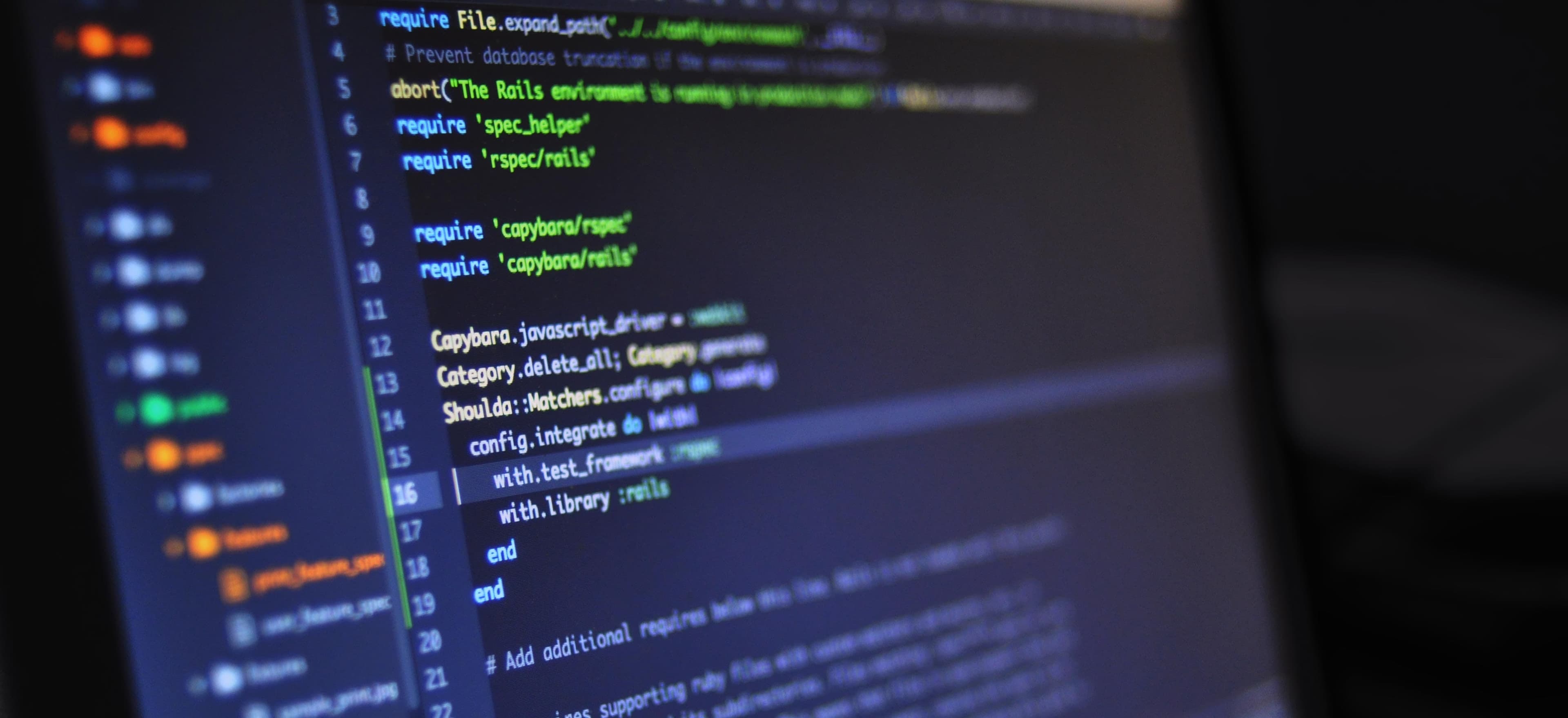
- Published on
Fixing SWT Listener Notification Issues with SWTEventHelper
Setting the Scene
In the realm of GUI programming, especially when using the Standard Widget Toolkit (SWT) in Java, properly managing event listeners is crucial for responsive applications. However, developers often face various notification issues, leading to glitches in user experience. This blog post delves into the intricacies of using SWTEventHelper
to effectively manage these issues, ensuring that notifications are sent reliably and efficiently.
Understanding SWT and Event Listeners
SWT is a popular library built for Java applications to utilize native GUI components on different platforms. An application developed using SWT can work seamlessly on Windows, macOS, and Linux, harnessing the underlying OS's widgets.
An essential feature of SWT applications is event handling through listeners. Listeners are objects that wait for certain events to occur, such as mouse clicks or key presses. When such an event occurs, the listener responds appropriately.
Common Notification Issues
-
Missed Events: One of the frequent problems developers encounter is missed events due to not properly attaching listeners.
-
Duplicate Notifications: Sometimes, a single event can trigger multiple notifications, which can lead to inconsistent behavior.
-
Memory Leaks: Incorrectly managed listeners can lead to memory leaks, as event listeners may keep references to the objects they shouldn't.
To address these issues, developers have found that using a utility class like SWTEventHelper
can mitigate many common pitfalls.
What is SWTEventHelper?
SWTEventHelper
is a utility designed to simplify managing event listeners and notifications. Its main purpose is to streamline the registration and notification processes to ensure that events are handled efficiently without redundancy.
Key Features of SWTEventHelper
- Centralized Event Management: This allows developers to manage event listeners in a single location.
- Debouncing Events: It prevents the same event from triggering multiple notifications.
- Automatic Listener Deregistration: Helps in managing memory by removing listeners when they are no longer needed.
Setting Up SWTEventHelper
Adding Dependencies
First, ensure you have SWT in your project's build path. If you're using Maven, you can add it via your pom.xml
:
<dependency>
<groupId>org.eclipse.swt</groupId>
<artifactId>org.eclipse.swt.win32.win64</artifactId>
<version>3.113.0</version>
</dependency>
Implementing SWTEventHelper
Here is a basic implementation for SWTEventHelper
. Assuming you have this class set up as follows:
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import java.util.HashMap;
import java.util.Map;
public class SWTEventHelper {
private final Map<String, Listener> listeners = new HashMap<>();
public void registerListener(String eventType, Listener listener) {
listeners.put(eventType, listener);
}
public void notifyListeners(String eventType, Event event) {
Listener listener = listeners.get(eventType);
if (listener != null) {
listener.handleEvent(event);
}
}
public void deregisterListener(String eventType) {
listeners.remove(eventType);
}
}
Explanation of the Code
-
Map<String, Listener>
: This map holds the event types as keys and their corresponding listeners as values. This centralized location for listeners aids in managing event handlers effectively. -
registerListener
Method: Allows you to add a listener for a particular event type. This prevents accidental re-registration of the same listener. -
notifyListeners
Method: This method is responsible for notifying listeners when an event occurs. It checks if a listener exists for a specific event and invokes it. -
deregisterListener
Method: This is essential for resource management, allowing you to remove listeners that are no longer needed, thus preventing memory leaks.
Utilizing SWTEventHelper in Your Application
Example Scenario
Imagine you are building an SWT application that requires a button to perform an action. You want to ensure that clicking this button triggers the action only once within a short time interval (debouncing).
Here’s how you can implement it using SWTEventHelper
:
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class MyApp {
private final SWTEventHelper eventHelper = new SWTEventHelper();
public void createAndRunUI() {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Event Example");
shell.setSize(300, 200);
Button button = new Button(shell, SWT.PUSH);
button.setText("Click Me");
button.setBounds(100, 50, 100, 30);
eventHelper.registerListener("buttonClick", e -> {
// Debouncing mechanism: Check if the last click was within 1 second
if(System.currentTimeMillis() - e.time < 1000) return;
System.out.println("Button clicked!");
// Update last click time
e.time = System.currentTimeMillis();
});
button.addListener(SWT.Selection, event -> {
eventHelper.notifyListeners("buttonClick", event);
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
public static void main(String[] args) {
MyApp app = new MyApp();
app.createAndRunUI();
}
}
Code Breakdown
-
Event Registration: We register a listener for the button click event with the key "buttonClick".
-
Debouncing Logic: Before executing the button click logic, we added a check for the last click time. This prevents rapid successive clicks from triggering the action repeatedly.
-
Event Notification: The event listener included a straightforward implementation that prints a message when clicked, encapsulated within the button's
addListener
.
The Last Word
Managing event listeners in an SWT application may seem daunting, but with the assistance of SWTEventHelper
, developers can create a more robust, manageable, and efficient GUI application. By centralizing listener management, implementing debouncing, and ensuring proper deregistration of listeners, developers can enhance their applications' performance and reliability.
For more detailed information on SWT and event handling, consider checking the official SWT documentation. It will provide further insights into creating rich client applications.
Further Learning
If you wish to expound your knowledge in GUI programming with Java, consider exploring additional resources like:
- Java GUI Programming with SWT - A comprehensive guide on building applications with SWT.
- Debouncing Events: Best Practices - Insights into optimizing event handling.
Now, go ahead and start building your next great SWT application with confidence!
This concludes the post on fixing SWT Listener Notification Issues with SWTEventHelper
. Implementing such helpers has proven to streamline the process of handling complex event-driven designs, improving user experiences in your applications.
Checkout our other articles