5 Common API Security Mistakes You Must Avoid
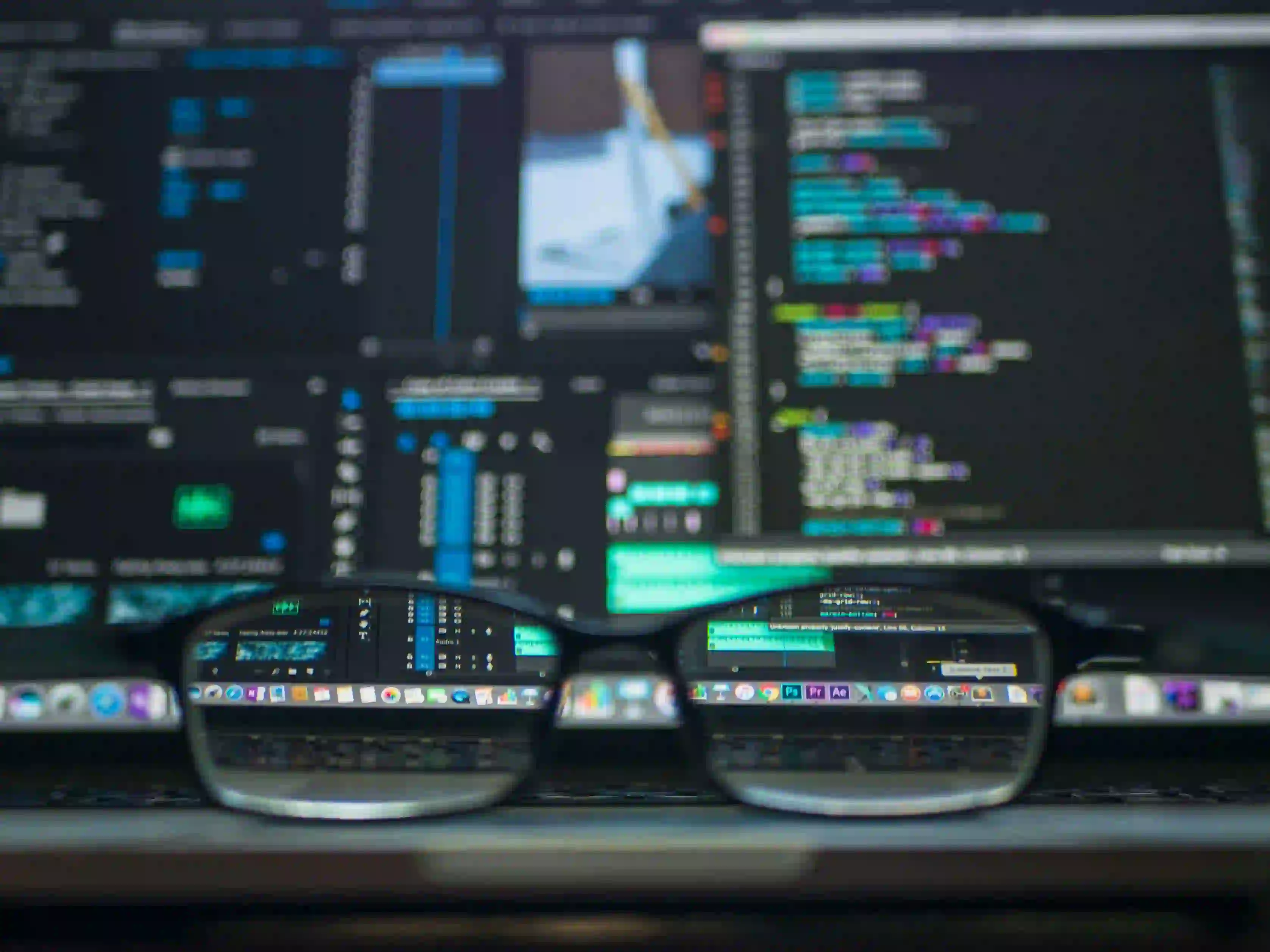
5 Common API Security Mistakes You Must Avoid
In today’s digital landscape, APIs (Application Programming Interfaces) are the backbone of modern applications. They facilitate communication between different systems, allowing developers to create robust functionalities with ease. However, with great power comes great responsibility, particularly in the realm of security. This blog post unveils the five most common API security mistakes that developers make, and offers guidance on how to avoid them.
1. Insufficient Authentication
The Mistake
Many developers underestimate the importance of authentication when designing an API. Insufficient authentication leaves the door wide open for malicious actors.
Why It Matters
Without strong authentication mechanisms, unauthorized users can gain access to sensitive data, leading to potential data breaches. It’s imperative to ensure that every API endpoint is protected.
Best Practices
- Use OAuth 2.0: Implement OAuth 2.0 for token-based authentication. This allows a secure way to authorize third-party applications without sharing user credentials.
- Secure Token Storage: Store authentication tokens securely; avoid exposing them in client-side code.
// Example of securing an API with OAuth 2.0
@RestController
@RequestMapping("/api")
public class SecureApiController {
@GetMapping("/user")
@PreAuthorize("hasRole('USER')")
public ResponseEntity<User> getUser(Authentication authentication) {
User user = userService.loadUserByUsername(authentication.getName());
return ResponseEntity.ok(user);
}
}
Commentary
The PreAuthorize annotation checks if the authenticated user has the required role before granting access. This keeps unauthorized requests at bay.
2. Lack of Input Validation
The Mistake
Another significant oversight is failing to validate inputs. This oversight can lead to various attacks, including SQL Injection, Command Injection, and Cross-Site Scripting (XSS).
Why It Matters
Input validation acts as the first line of defense, preventing malicious data from being processed by your system.
Best Practices
- Validate All Inputs: Ensure that all input data meets predefined criteria.
- Sanitize User Inputs: Clean and sanitize inputs to eliminate harmful scripts.
// Example of input validation in Java
@PostMapping("/submit")
public ResponseEntity<String> submitData(@Valid @RequestBody UserData userData) {
// Business logic here
return ResponseEntity.ok("Data submitted successfully");
}
Commentary
By using the @Valid
annotation, we ensure only valid data structures are processed. This minimizes the risk of intrusion through malicious input.
3. Not Implementing Rate Limiting
The Mistake
Many APIs lack rate limiting, allowing unlimited requests from a single source. This failure can lead to abuse or denial-of-service (DoS) attacks, overwhelming the system.
Why It Matters
Rate limiting safeguards your API against excessive usage or malicious attacks by controlling traffic flow.
Best Practices
- Implement Rate Limiting: Use tools like API Gateway or libraries dedicated to rate limiting.
- Use Response Headers: Communicate usage limits with clear headers in the API response.
// Example of rate limiting using Spring
@Bean
public FilterRegistrationBean<RateLimitingFilter> rateLimitingFilter() {
FilterRegistrationBean<RateLimitingFilter> registrationBean = new FilterRegistrationBean<>();
registrationBean.setFilter(new RateLimitingFilter());
registrationBean.addUrlPatterns("/api/*");
return registrationBean;
}
Commentary
Utilizing a filter for rate limiting helps restrict the number of requests from clients, preserving your API's performance and resilience.
4. Insecure Data Transmission
The Mistake
APIs that do not secure data in transit expose themselves to interception. Failing to use HTTPS leaves data vulnerable to eavesdropping attacks.
Why It Matters
Sensitive data, such as passwords or personal information, can easily be exploited if transmitted over unsecured connections.
Best Practices
- Use HTTPS: Always enforce HTTPS to encrypt data during transmission, ensuring a secure channel.
- Implement HSTS: Enable HTTP Strict Transport Security to force clients to connect using HTTPS only.
// Example of configuring HTTPS in Spring Boot
@Configuration
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requiresChannel()
.anyRequest()
.requiresSecure();
}
}
Commentary
Requiring secure channels helps in protecting data in transit, securing it against potential man-in-the-middle attacks.
5. Ignoring API Versioning
The Mistake
Neglecting to implement versioning in your API can lead to breaking changes affecting consumers of the API.
Why It Matters
Proper versioning allows for incremental updates without disrupting your users. This ensures backward compatibility and aids in smoother transitions.
Best Practices
- URI Versioning: Include version info in the API endpoint (e.g., /v1/resource).
- Deprecation Strategy: Clearly communicate deprecations to API consumers, providing adequate transition time.
// Example of versioned API endpoint in Spring
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
// Implement endpoint logic here
}
Commentary
By incorporating versioning into your API's URI, you enable clients to choose which version to interact with, promoting flexibility and reducing disruption.
Final Thoughts
The importance of API security cannot be overstated. By addressing these five common security mistakes—insufficient authentication, lack of input validation, absent rate limiting, insecure data transmission, and ignoring versioning—you can significantly enhance your API's security framework.
Implementing robust security measures not only protects your data and users but also builds trust in your brand. Always stay updated with the best practices and continue seeking knowledge in API security realms. For further reading, explore resources like OWASP API Security Top 10 and API Security Best Practices.
By being proactive in addressing these issues, you can contribute to a safer digital environment while optimizing the user experience with your APIs.