Choosing the Right Number of Threads for Your Project
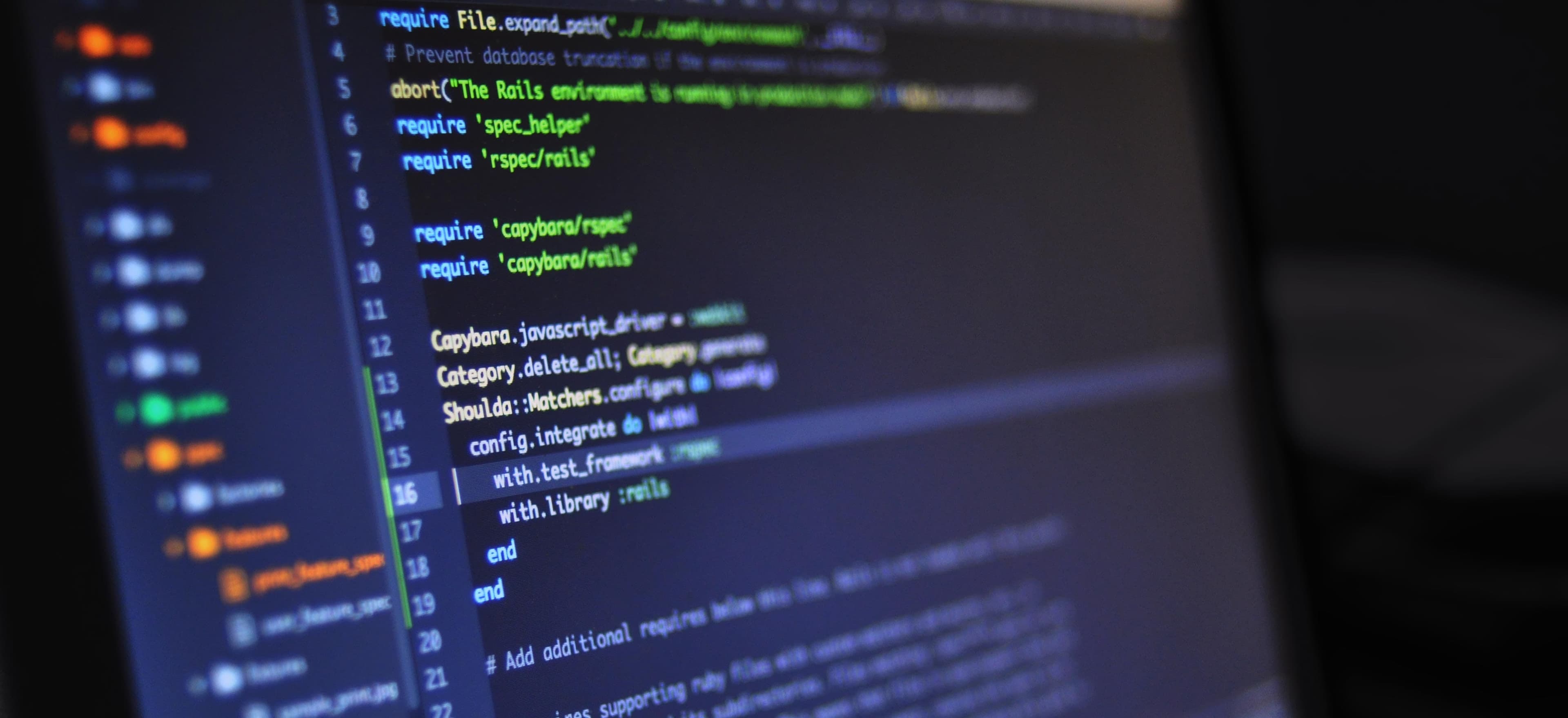
- Published on
Choosing the Right Number of Threads for Your Java Project
When it comes to building Java applications, one of the critical considerations developers face is the choice of how many threads to utilize. Multithreading is a powerful feature of Java, enabling concurrent execution, which can improve performance dramatically. However, the optimal number of threads for your project can vary significantly based on several factors, including the type of application, the workload, and the hardware capabilities.
In this blog post, we'll explore the intricacies of multithreading in Java and provide guidance on selecting the right number of threads for your project. We will cover the following topics:
- Understanding Multithreading
- When to Use Threads
- Factors Influencing Thread Count
- How to Calculate the Right Number of Threads
- Performance Monitoring and Metrics
- Conclusion
Understanding Multithreading
Multithreading is the ability to execute multiple threads simultaneously, making it possible for a Java application to perform several operations at the same time. Java provides built-in support for multithreading, with the Thread
class and the Runnable
interface being two primary ways to create threads.
Here’s a simple example of creating a thread:
public class MyFirstThread implements Runnable {
@Override
public void run() {
System.out.println("Hello from the thread!");
}
public static void main(String[] args) {
Thread myThread = new Thread(new MyFirstThread());
myThread.start(); // Start the thread
}
}
Why Use this code?
This snippet demonstrates how to implement the Runnable
interface to create a new thread. The run
method contains the code that will execute concurrently. Using Runnable
over extending Thread
allows for better flexibility and reuse of code.
When to Use Threads
Deciding whether or not to use threads can depend on the specific requirements of your application:
-
I/O-Bound Operations: If your application performs tasks like reading and writing files, network calls, or interfacing with databases, using threads can help improve responsiveness and utilization.
-
CPU-Bound Operations: For tasks that require intensive computational power, multithreading can speed up execution by dividing workloads among multiple CPU cores.
-
Responsive UIs: In desktop or mobile applications, using threads can prevent a UI from freezing by moving long-running tasks to the background.
Factors Influencing Thread Count
Choosing the right number of threads involves considering various aspects of your application's environment:
-
Hardware Specifications: The number of CPU cores on the server will significantly influence the thread count. As a general rule, the number of threads should not exceed the number of available cores.
-
Nature of the Task: Tasks that spend a lot of time waiting (I/O-bound) can benefit from more threads than CPU-bound tasks.
-
Concurrency Level: If you are managing multiple tasks that can run in parallel, such as handling multiple requests in a web server, more threads may be necessary.
-
Java Virtual Machine (JVM) Settings: Different JVM configurations can also play a role, including heap size and garbage collection settings.
-
Thread Management Overhead: Each thread incurs overhead for the JVM, so having too many threads can lead to performance degradation due to context switching.
How to Calculate the Right Number of Threads
The Basic Rule
A common guideline is to use the following formula to determine the optimal number of threads:
Threads = Number of Cores x (1 + Wait Time / Compute Time)
- Number of Cores: This is the total number of logical cores available to your Java application.
- Wait Time: Time spent waiting for resources (like I/O)
- Compute Time: Time spent on computations
Example Scenario
Consider that you have a server with 4 cores, and the ratio of wait time to compute time is 3:1. Using the formula:
Threads = 4 x (1 + 3/1) => 4 x 4 = 16
In this case, you might want to start with 16 threads.
Code Snippet: Implementing a Simple Thread Pool
It's often better to use a thread pool to manage threads efficiently. Java provides the ExecutorService
framework to simplify thread management:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Create a thread pool with a fixed number of threads
ExecutorService executorService = Executors.newFixedThreadPool(4); // Choose an appropriate number based on your calculations
for (int i = 0; i < 10; i++) {
executorService.execute(new Task(i));
}
executorService.shutdown(); // Don't forget to shut down the executor
}
}
class Task implements Runnable {
private final int taskId;
public Task(int taskId) {
this.taskId = taskId;
}
@Override
public void run() {
System.out.println("Task " + taskId + " is running.");
}
}
Why Use This Code?
This code illustrates creating a thread pool using Executors.newFixedThreadPool(int nThreads)
. It allows you to manage a set number of threads efficiently. You can submit multiple tasks to the executor, and it will manage how they run, thus optimizing performance.
Performance Monitoring and Metrics
Once you implement multithreading, it is essential to monitor your application’s performance. Profiling tools such as JVisualVM, YourKit, or integrated monitoring tools in cloud platforms can help you track metrics like:
- Thread count
- Memory usage
- CPU usage
- Response times
Continuously monitoring these metrics will allow you to adapt the number of threads based on actual application behavior.
My Closing Thoughts on the Matter
Choosing the right number of threads for your Java application is crucial for maximizing performance and efficiency. It involves a careful analysis of your application's needs, the workload, and the available resources. By understanding the fundamental concepts of multithreading in Java and leveraging tools like thread pools, you can improve the responsiveness and capability of your applications.
For more in-depth reading, check out the following resources:
- Java Concurrency in Practice - A comprehensive guide to mastering concurrency in Java.
- Java Platform, Standard Edition - Official documentation for Java SE, providing detailed insights into threading.
Remember, effective multithreading requires both a strategic approach and continuous performance assessment to achieve optimal results. Happy coding!
Checkout our other articles