Struggling with Spring Boot Database Initialization? Here's Help!
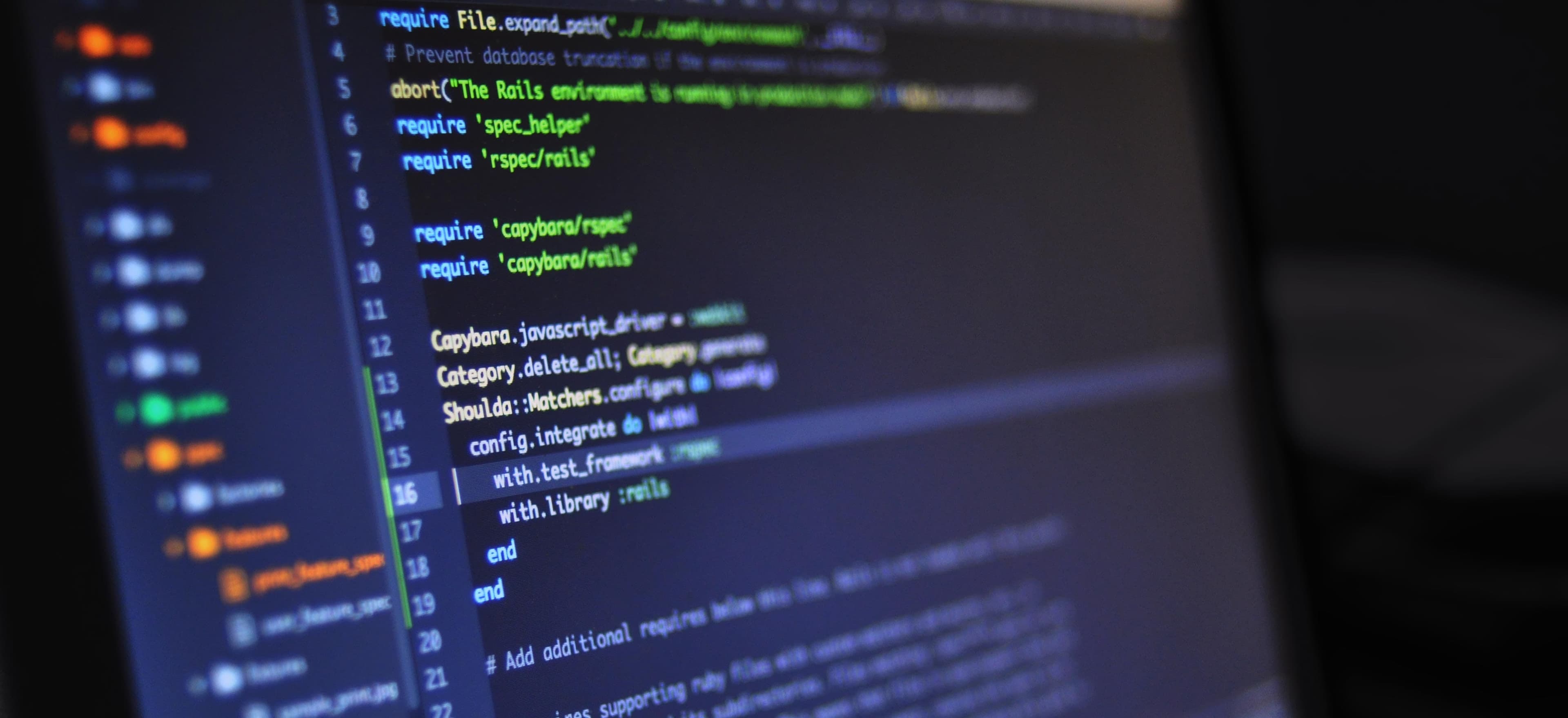
- Published on
Struggling with Spring Boot Database Initialization? Here's Help!
Spring Boot has revolutionized the landscape of Java development, especially when it comes to creating stand-alone, production-grade applications. However, one common point of confusion many developers face is the database initialization process. In this blog post, we'll dive into the essentials of this topic, exploring how to set up your database effectively within a Spring Boot application. Whether you're a seasoned developer or just starting out, this guide will equip you with the knowledge to seamlessly initialize your database.
Understanding Database Initialization
Database initialization refers to the process of setting up your database schema and populating it with initial data when your Spring Boot application starts. This is crucial for testing, seeding data for development, and ensuring that your application runs smoothly in production.
Spring Boot simplifies this process through various properties and configurations. The framework can automatically create tables and load initial data based on certain conditions. Let's explore how it works.
Spring Boot Data JPA Setup
First, ensure that you have the necessary dependencies in your Maven pom.xml
. Spring Data JPA will ease the communication between the application and database, and Hibernate will implement the actual ORM to facilitate object-relational interactions.
pom.xml Example
<dependencies>
<!-- Spring Boot Starter Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Driver for your specific database, e.g., H2 Database -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Explanation
-
Spring Boot Starter Data JPA: This starter package includes Spring Data JPA and the necessary dependencies for your data access layer.
-
H2 Database: Here, we are using H2 as an example. It's an in-memory database useful for development and testing. In a production environment, you'd replace this with your actual database driver.
Configuring Application Properties
Next, you'll need to configure your application properties for the database connection in the application.properties
file located in the src/main/resources
directory.
Example application.properties
# Spring datasource configuration
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
# JPA configuration
spring.jpa.hibernate.ddl-auto=create-drop
spring.jpa.show-sql=true
Explanation
-
Datasource Configuration: This creates an in-memory database called
testdb
. Customize the URL, username, and password to match your specific database requirements. -
Hibernate DDL Auto: The
create-drop
command instructs Hibernate to create the schema at startup and drop it when the application closes. This is useful for testing but should be set toupdate
orvalidate
in production. -
Show SQL: Enables logging of the SQL commands Hibernate executes—helpful for debugging.
Using SQL Scripts for Initialization
Spring Boot can also execute SQL scripts to initialize your database with structured data. By default, it will run schema.sql
to create tables and data.sql
to load initial data.
Creating SQL Scripts
- schema.sql: Create this file to define your database schema.
CREATE TABLE user (
id BIGINT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL
);
- data.sql: Populate your database with this file.
INSERT INTO user (name, email) VALUES ('John Doe', 'john@example.com');
INSERT INTO user (name, email) VALUES ('Jane Smith', 'jane@example.com');
Explanation
- The
schema.sql
file sets up the structure of the database, whiledata.sql
injects the initial data.
Important Note
Make sure both files are located in src/main/resources
, and Spring Boot will automatically load them during the initialization phase. You can read more about database populator scripts in the Spring Boot documentation.
Using JPA Entity Classes
To connect your database with Java classes, you should define Entities. These classes represent your database tables and manage data persistence.
User Entity Example
import javax.persistence.*;
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String name;
@Column(unique = true, nullable = false)
private String email;
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Explanation
- Annotations:
@Entity
marks the class as a JPA entity.@Table
specifies the table name.@Id
and@GeneratedValue
define the primary key and its generation strategy.
Utilizing entities allows JPA to abstract most of the boilerplate code involved in managing database connections.
Repository Layer
To interact with the database, you'll need to create a repository. Spring Data JPA simplifies this via interfaces.
UserRepository Example
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
// No additional code needed, Spring Data JPA provides implementations
}
Explanation
- By extending
JpaRepository
, yourUserRepository
inherits various methods for CRUD operations without needing to implement them manually.
Running the Application
With everything set up, you can now run your Spring Boot application. Upon startup, Spring Boot will initialize the database automatically based on your properties and SQL scripts.
To best verify that everything is working correctly, it can be helpful to create a simple REST controller.
UserController Example
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/users")
public class UserController {
private final UserRepository userRepository;
public UserController(UserRepository userRepository) {
this.userRepository = userRepository;
}
@GetMapping
public List<User> getUsers() {
return userRepository.findAll();
}
}
Explanation
- This controller exposes a REST endpoint that retrieves user data from the database. When you hit the
/users
URL, you should see the initialized data.
Troubleshooting Common Issues
-
Database Connection Issues: Ensure your database URL, username, and password are correct.
-
Schema Creation: If tables aren’t created, verify your Hibernate DDL settings and ensure your SQL files are correctly placed.
-
Dependency Conflicts: Ensure that all dependencies are compatible with each other; check the versions specified in your
pom.xml
.
Final Thoughts
Initializing your database in a Spring Boot application doesn't have to be a daunting task. With a clear understanding of the configurations, SQL scripts, and entity management, you can set up your application efficiently.
Feel free to experiment with different configurations and data setups based on your needs. By following this guide, you should now be well-equipped to tackle any challenges related to Spring Boot database initialization.
For further reading, refer to the official documentation on Spring Data JPA and explore more advanced topics in database management.
Happy coding!