Mastering Date-Time in Spring Boot with Thymeleaf
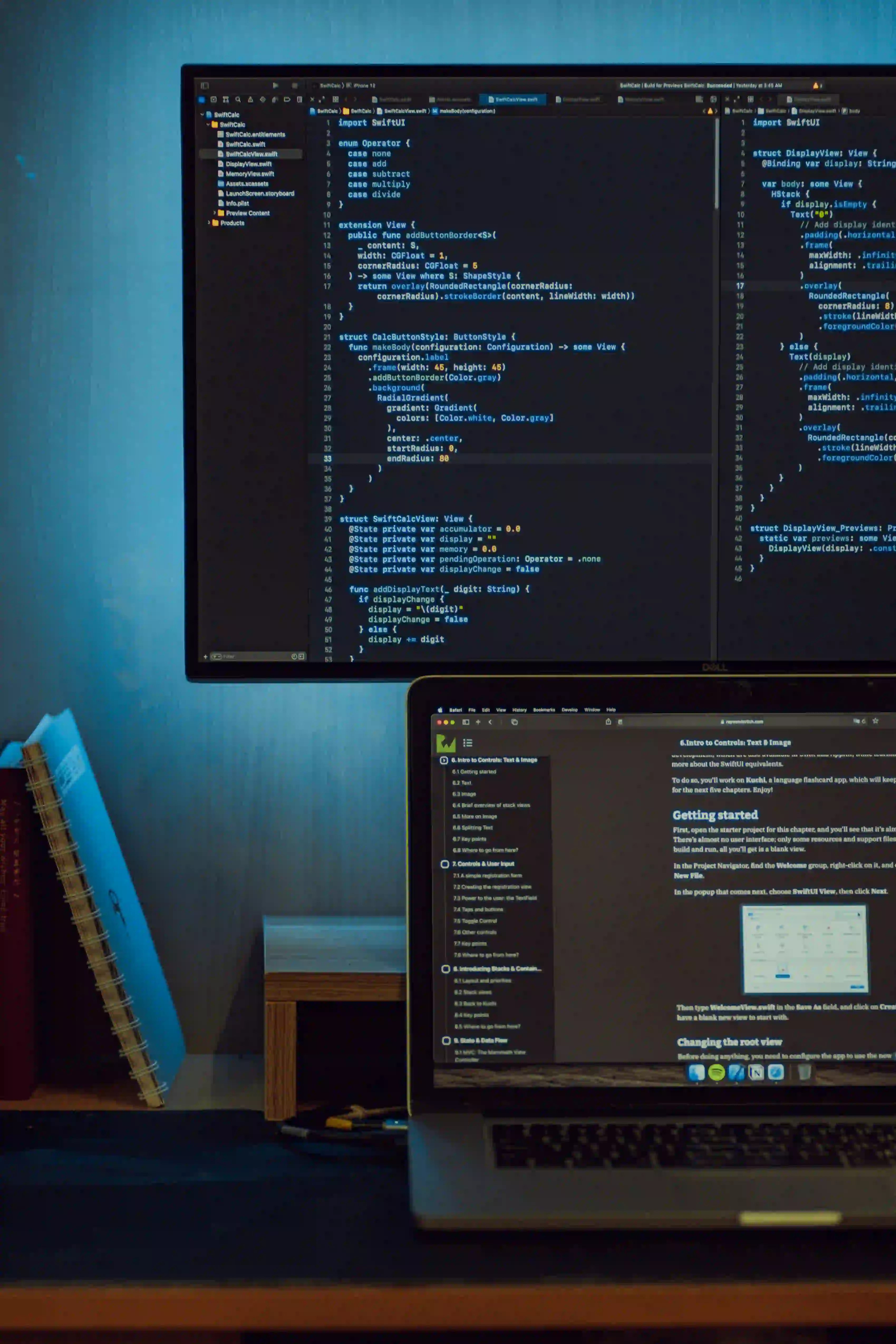
Mastering Date-Time in Spring Boot with Thymeleaf
Working with date and time can often be challenging. This is especially true when developing web applications where both the backend and frontend need to handle date-time representations consistently. In modern web development, frameworks like Spring Boot and Thymeleaf offer powerful tools to manage date-time with simplicity and efficiency. In this blog post, we will explore how to master date and time in your Spring Boot applications using Thymeleaf templates.
Table of Contents
- Introduction to Date-Time in Java
- Setting Up Your Spring Boot Application
- Handling Date-Time in Spring Boot
- Date and Time Converters
- Using Java 8 Time (java.time)
- Integrating Thymeleaf
- Displaying Date-Time in Thymeleaf
- Form Handling with Date-Time
- Conclusion
- References
1. Introduction to Date-Time in Java
Java has evolved to handle date and time more efficiently. Before Java 8, developers relied heavily on the java.util.Date
and java.util.Calendar
classes, which had limitations. Java 8 introduced the java.time
package, which brought a more intuitive API for date and time manipulation.
You can work with local dates, local times, and perform various operations easily using the classes from this package. The key classes include:
- LocalDate: Represents a date without time-zone.
- LocalTime: Represents a time without date.
- LocalDateTime: Represents both date and time without time-zone.
- ZonedDateTime: Represents date-time with time-zone.
Benefits of Using java.time
- Immutable objects.
- Better type-safety.
- Clearer API for operations.
2. Setting Up Your Spring Boot Application
Let's start with setting up a basic Spring Boot application. You can use Spring Initializr to set up your project.
-
Select your project metadata:
- Project: Maven Project
- Language: Java
- Spring Boot: 3.x.x (latest stable version)
- Dependencies: Spring Web, Spring Boot DevTools, Thymeleaf, Spring Data JPA, H2 Database (or your preferred database).
-
Generate the project and unpack it in your development environment.
-
Your project structure should look like this:
πsnippet.txtsrc βββ main βββ java β βββ com β βββ example β βββ demo β βββ DemoApplication.java β βββ controller β βββ model β βββ repository βββ resources βββ application.properties βββ templates
3. Handling Date-Time in Spring Boot
Date and Time Converters
Spring Boot relies on @InitBinder
to allow custom binding of form fields. Hereβs how you can set it up to properly format date inputs.
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.WebDataBinder;
import org.springframework.web.bind.annotation.InitBinder;
import org.springframework.web.bind.annotation.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import java.time.LocalDate;
@Controller
public class DateTimeController {
@InitBinder
public void initBinder(WebDataBinder binder) {
// Custom date format
binder.registerCustomEditor(LocalDate.class,
new CustomDateEditor(new SimpleDateFormat("yyyy-MM-dd"), true));
}
@GetMapping("/date-form")
public String showForm(Model model) {
model.addAttribute("localDate", LocalDate.now());
return "date-form";
}
@PostMapping("/date-submit")
public String submitForm(@DateTimeFormat(iso = DateTimeFormat.ISO.DATE) LocalDate localDate) {
// Add your logic here
return "date-result";
}
}
Why do we use @InitBinder
?
The @InitBinder
annotation helps customize specific binding from the view to the model. By registering a custom date editor, we can ensure that incoming strings are automatically converted to LocalDate
objects.
Using Java 8 Time (java.time)
To use date-time types with JPA entities, modify your entities as follows:
import javax.persistence.Entity;
import javax.persistence.Id;
import java.time.LocalDate;
@Entity
public class Event {
@Id
private Long id;
private String name;
private LocalDate eventDate;
// getters and setters
}
The use of LocalDate
directly in the entity simplifies your database interactions.
4. Integrating Thymeleaf
Thymeleaf is a modern server-side Java template engine that can handle text, XML, JavaScript, CSS, and even plain HTML. It's particularly useful for rendering dates seamlessly.
Displaying Date-Time in Thymeleaf
You can render date-time objects in your Thymeleaf templates using the following syntax:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Date-Time Example</title>
</head>
<body>
<h1>Event Date</h1>
<p th:text="${event.eventDate}">Date goes here</p>
</body>
</html>
Why is this useful?
Using th:text
, you can dynamically display the event date directly from your model object. Thymeleaf takes care of converting LocalDate
to a readable format.
Form Handling with Date-Time
To effectively handle form submissions with date-time values, create a Thymeleaf form:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Date Form</title>
</head>
<body>
<form action="#" th:action="@{/date-submit}" th:object="${localDate}" method="post">
<label>Date (YYYY-MM-DD):</label>
<input type="date" th:field="*{eventDate}" />
<button type="submit">Submit</button>
</form>
</body>
</html>
In this example, the th:field
expands to the correct attribute path, making it easy to bind the form input with the model directly.
5. Conclusion
Mastering date-time in Spring Boot with Thymeleaf empowers developers to create robust, user-friendly applications. By leveraging the features of Java's date and time API, custom data binding, and Thymeleaf template capabilities, you can ensure a seamless user experience.
As you work with date-time in your applications, always consider:
- User Locale: To make your application more user-friendly, consider leveraging locale-based formatting.
- Time Zones: If your application deals with users across different time zones, consider using
ZonedDateTime
to avoid discrepancies.
6. References
By mastering these concepts, you'll be well on your way to developing powerful, date-sensitive web applications that meet your users' needs. Happy coding!