Common Spring Batch Groovy Mistakes and How to Fix Them
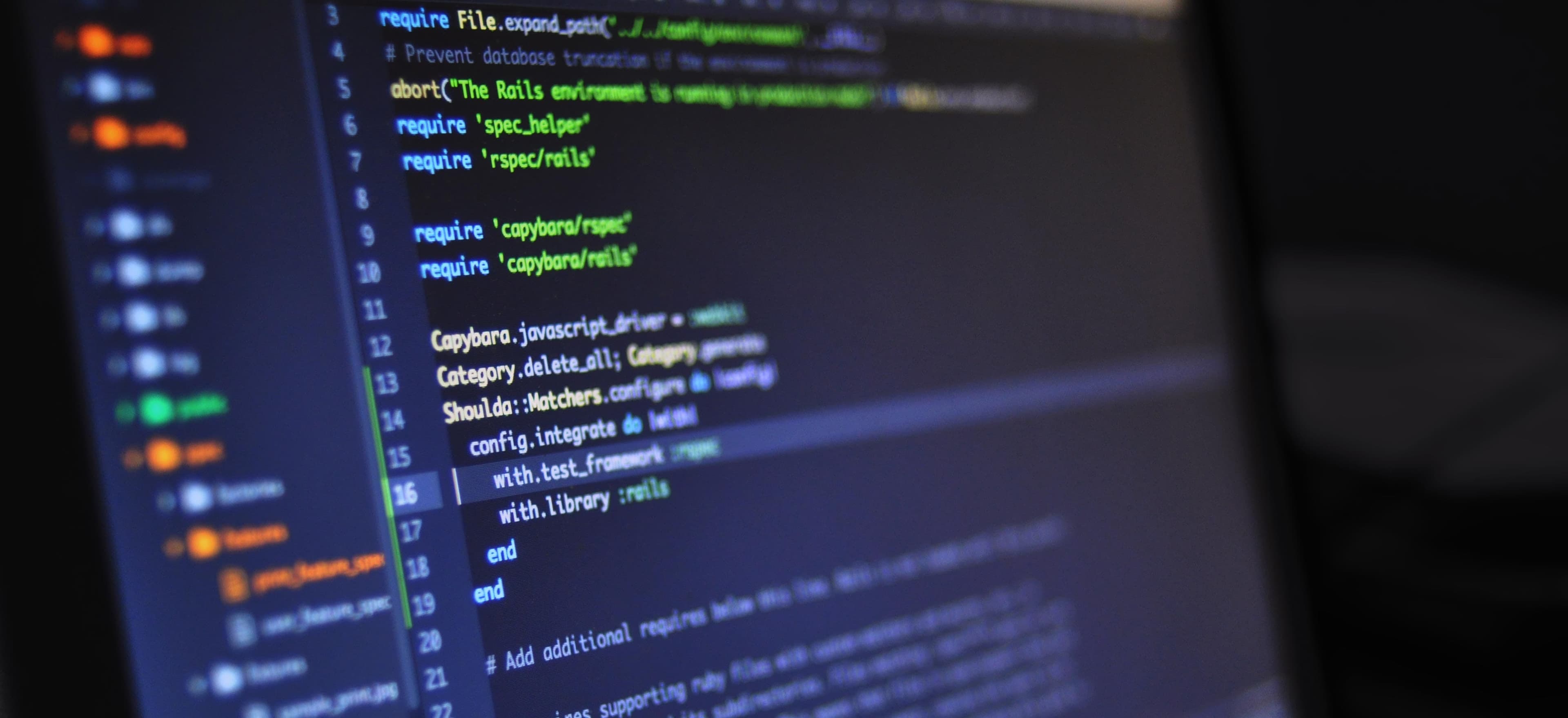
- Published on
Common Spring Batch Groovy Mistakes and How to Fix Them
Spring Batch is a powerful framework that simplifies the development of batch processing applications. Its well-defined features such as job processing, logging, and transaction management, make it a preferred choice for batch operations in Java. However, when Groovy is integrated into Spring Batch, certain mistakes can commonly occur. In this article, we will explore the common pitfalls developers encounter when using Groovy with Spring Batch and provide actionable fixes for these issues.
Why Use Groovy with Spring Batch?
Before we dive into the mistakes, let’s briefly touch on why Groovy is often used in conjunction with Spring Batch. Groovy offers:
- Concise Syntax: Groovy reduces boilerplate code, making the implementation shorter and easier to read.
- Dynamic Features: Groovy supports dynamic method calls and closures, allowing for more flexible code.
- Seamless Integration: Groovy integrates seamlessly with Java, which means you can leverage existing Java libraries and frameworks, including Spring.
Mistake 1: Misconfigured Job Parameters
One of the most common mistakes is mishandling job parameters. Spring Batch heavily relies on job parameters to control job behavior and execution flow.
Problem:
If you fail to pass job parameters correctly, Spring Batch might end up executing an empty job or misconfigured steps.
Fix:
Ensure you correctly configure job parameters. Here’s a simple example of setting job parameters in Groovy:
import org.springframework.batch.core.JobParameters
import org.springframework.batch.core.JobParametersBuilder
def jobParameters = new JobParametersBuilder()
.addString('input.file.name', 'input.txt')
.addLong('time', System.currentTimeMillis())
.toJobParameters()
In this example, we include a timestamp to ensure job instances are always unique when required. This approach also prevents the accidental reuse of the same job parameters.
Mistake 2: Incorrect Step Configuration
Spring Batch steps must be properly configured as they perform the bulk of the processing work. Frequently, developers overlook the setup.
Problem:
Steps may fail if configurations like readers, writers, or processors are not properly set up or wired.
Fix:
Here's how to effectively configure a step in Groovy:
import org.springframework.batch.core.Step
import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing
import org.springframework.batch.core.configuration.annotation.StepBuilderFactory
import org.springframework.batch.item.ItemProcessor
import org.springframework.batch.item.ItemReader
import org.springframework.batch.item.ItemWriter
@EnableBatchProcessing
class BatchConfig {
Step step(StepBuilderFactory stepBuilderFactory) {
return stepBuilderFactory.get('myStep')
.<InputType, OutputType>chunk(10)
.reader(reader())
.processor(processor())
.writer(writer())
.build()
}
ItemReader<InputType> reader() {
// Implementation of reader
}
ItemProcessor<InputType, OutputType> processor() {
// Implementation of processor
}
ItemWriter<OutputType> writer() {
// Implementation of writer
}
}
In the above code, ensure that the chunk()
size aligns with your use case, as improper chunk sizes can lead to memory issues or inefficient processing.
Mistake 3: Forgetting to Handle Exceptions Properly
Groovy’s dynamic nature can sometimes lead to oversight in error handling, especially in batch jobs where you might blindly assume processes will succeed.
Problem:
Unhandled exceptions can cause batch jobs to fail abruptly without any logged context.
Fix:
Always implement proper exception handling in your job execution. For instance:
import org.springframework.batch.core.StepExecution
import org.springframework.batch.core.listener.StepExecutionListener
class MyStepListener implements StepExecutionListener {
@Override
void beforeStep(StepExecution stepExecution) {
// Initialization logic
}
@Override
ExitStatus afterStep(StepExecution stepExecution) {
if (stepExecution.getFailureExceptions().size() > 0) {
println "Errors occurred: ${stepExecution.getFailureExceptions()}"
}
return stepExecution.getExitStatus()
}
}
By implementing StepExecutionListener
, you can get detailed insight into the execution context and handle exceptions effectively.
Mistake 4: Ignoring Transaction Management
Batch processing often involves handling large volumes of data, which necessitates careful transaction management.
Problem:
Overlooking transaction boundaries can lead to data inconsistency in case of failures.
Fix:
You can manage transactions using Spring’s built-in support. Here’s how you can ensure transactional integrity:
import org.springframework.transaction.annotation.Transactional
@Transactional
def myBatchProcess() {
// Processing logic
}
Wrapping your batch process with @Transactional
annotation ensures that the entire process is atomic. If one part fails, it will roll back all changes, maintaining data integrity.
Mistake 5: Underestimating the Importance of Logging
Logging is vital for diagnosing issues in batch jobs, especially in production.
Problem:
Insufficient logging can create challenges in debugging and monitoring batch jobs.
Fix:
Incorporate robust logging within your Groovy code using Spring's @Slf4j
to log important events:
import groovy.util.logging.Slf4j
@Slf4j
class MyBatchJob {
def execute() {
log.info("Job execution started")
// Job logic
log.info("Job execution finished successfully")
}
}
By using @Slf4j
, you can log the flow of your batch job efficiently, making it easier to trace back any issues that might arise.
Resources for Additional Learning
While the above points cover common mistakes, further resources can help deepen your understanding:
Closing Remarks
In this post, we explored common pitfalls when integrating Groovy with Spring Batch and discussed how to fix them. As you implement batch processing, remember the importance of job parameters, step configuration, exception handling, transaction management, and robust logging. By avoiding these pitfalls and applying best practices, you enhance the maintainability and reliability of your batch applications.
Remember, every mistake is an opportunity to learn. Embrace Groovy with confidence, and let Spring Batch handle the heavy lifting. Happy coding!
Checkout our other articles