Mastering Relative Scaling in Blue-Green Deployments
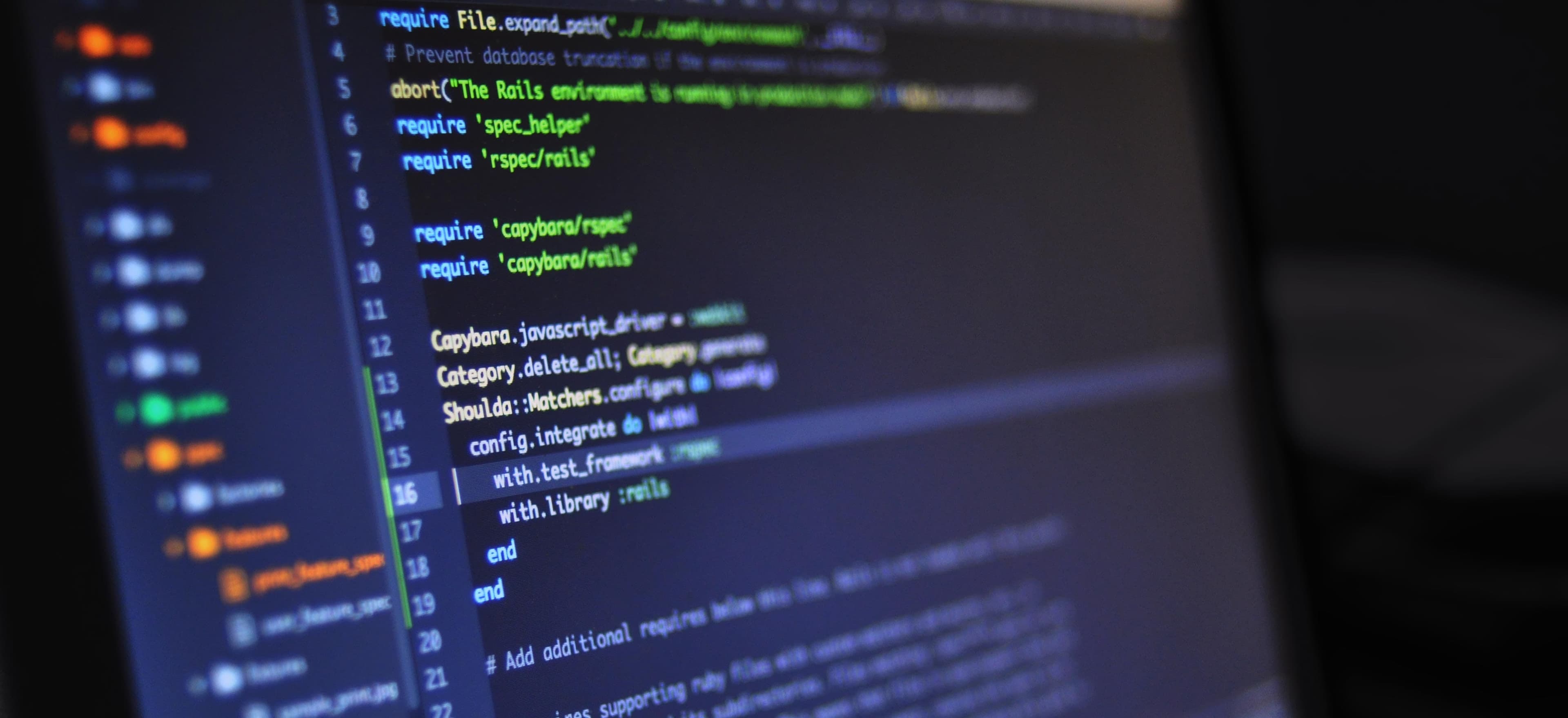
- Published on
Mastering Relative Scaling in Blue-Green Deployments
Blue-green deployments are a popular strategy for releasing new software versions. This deployment strategy minimizes downtime and reduces risks via parallel environments. However, one often overlooked aspect is relative scaling – adjusting resource allocations based on different environmental demands. In this blog post, we will explore how to master relative scaling in blue-green deployments, enhancing your application performance while ensuring seamless releases.
Understanding Blue-Green Deployments
Before delving into relative scaling, let's clarify what blue-green deployments entail. Simply put, this method involves two identical environments:
- Blue: The current production environment.
- Green: The new version that is being prepared for release.
In a typical scenario, the traffic is routed to the blue environment while the green one is being updated and tested. Once the new version is validated, traffic is switched to the green, making it live. This strategy offers several benefits:
- Reduced downtime.
- Easier rollback to the previous version in case of issues.
- Better testing in a production-like environment.
Why Relative Scaling Matters
Tests in a blue-green setup often yield varied results across environments. The green environment may perform differently due to new features, changed workloads, or variations in user behavior. Relative scaling ensures that both the blue and green environments are optimally configured to handle traffic and resource needs adequately.
Implementing relative scaling can:
- Enhance performance.
- Reduce costs by allocating only the needed resources.
- Avoid overwhelming the green environment during traffic switch.
Principles of Relative Scaling
Relative scaling in blue-green deployments revolves around three main principles:
-
Monitoring Performance: Continuous monitoring of both environments is necessary. Utilize tools like New Relic or Prometheus to gather metrics such as CPU utilization, memory usage, and response time.
-
Dynamic Resource Allocation: Depending on the performance metrics collected, you can adjust resource allocations dynamically. Use orchestration tools like Kubernetes to automate scaling.
-
User Behavior Modeling: Understanding how users interact with your application helps predict traffic patterns. Analyze data trends to scale resources proactively.
Implementing Relative Scaling
Step 1: Set Up Monitoring
To effectively scale resources, you first need to monitor your applications in real-time. You can achieve this with a combination of logging and monitoring.
Here is a simple Java code snippet for setting up a monitoring service using Micrometer, a library that integrates well with Prometheus:
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Tag;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MonitoringService {
private final MeterRegistry meterRegistry;
@Autowired
public MonitoringService(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
public void recordRequest(String status) {
meterRegistry.counter("http_requests_total", Tag.of("status", status)).increment();
}
}
Why this code? This setup records the total number of HTTP requests based on their status. Understanding the request flow helps identify bottlenecks and performance issues promptly.
Step 2: Implement Dynamic Resource Allocation
Dynamic scaling requires automated configurations based on the current needs. When using a Kubernetes cluster, you can leverage Horizontal Pod Autoscaling (HPA) to automatically adjust the number of pod replicas.
An HPA configuration could resemble the following YAML file:
apiVersion: autoscaling/v1
kind: HorizontalPodAutoscaler
metadata:
name: my-app-autoscaler
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-app
minReplicas: 1
maxReplicas: 5
targetCPUUtilizationPercentage: 70
Why this configuration? Here, the HPA automatically adjusts the number of pod replicas based on the CPU usage. If the average CPU utilization exceeds 70%, Kubernetes scales up the replicas. On the other hand, it scales down when the load decreases, optimizing resource usage.
Learn more about Kubernetes HPA here.
Step 3: Analyze User Behavior
Incorporating user behavior analytics can contribute significantly to your scaling strategy. Here's an example of how you might collect and analyze user data using Spring Data JPA:
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserActivityRepository extends JpaRepository<UserActivity, Long> {
List<UserActivity> findByActivityDateBetween(LocalDate start, LocalDate end);
}
Why this repository? This allows you to query user activity data efficiently. By analyzing patterns, you can estimate traffic spikes, adjusting your deployments dynamically before problems arise.
Final Thoughts on Relative Scaling in Blue-Green Deployments
Mastering relative scaling in blue-green deployments is integral to maintaining high availability and performance. While blue-green deployments simplify the release process, a strategic approach to resource allocation ensures that both environments can effectively handle user demands.
To summarize, here are key points to remember:
- Utilize monitoring to understand application performance.
- Implement dynamic scaling to optimize resource usage.
- Analyze user behavior to anticipate traffic patterns.
By following these principles and utilizing the snippets highlighted, your transition into blue-green deployment strategies paired with relative scaling can lead not just to robust releases, but to a more fortified infrastructure capable of handling ever-changing user demands.
For further reading on blue-green deployments and resource scaling, check out Martin Fowler's article.
By mastering relative scaling strategies, you not only improve your application's performance but also guarantee a smoother experience for your users. Happy coding!