Struggling with JUnit 5 Support in Ant? Here’s the Fix!
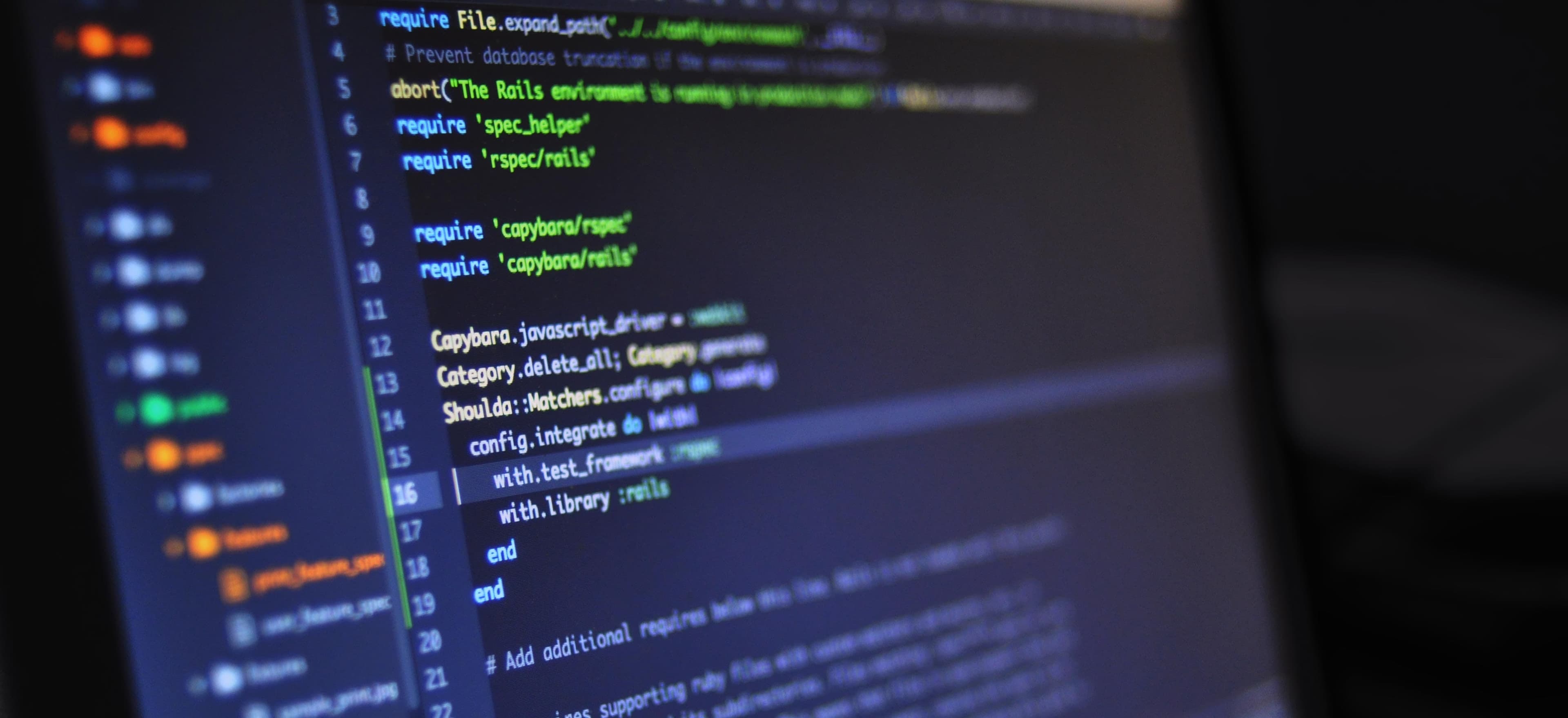
- Published on
Struggling with JUnit 5 Support in Ant? Here’s the Fix!
JUnit is a widely used testing framework for Java that allows developers to write and run repeatable tests. With the release of JUnit 5, the framework has undergone significant changes and improvements. However, integrating JUnit 5 into Apache Ant can be a headache for some developers. If you're facing issues with JUnit 5 support in Ant, you've come to the right place. In this blog post, we'll discuss the necessary steps to integrate JUnit 5 with Ant seamlessly.
Table of Contents
- Understanding JUnit 5
- Setting Up Your Project
- Configuring Ant for JUnit 5
- Writing Your First JUnit 5 Test
- Running Tests with Ant
- Conclusion
Understanding JUnit 5
JUnit 5, also known as Jupiter, is a complete rewrite of the popular Java testing framework. It introduces several improvements over its predecessor, including:
- Modular Architecture: JUnit 5 is composed of three sub-projects: JUnit Platform, JUnit Jupiter, and JUnit Vintage. This allows better customization and flexibility.
- New Annotations: Annotations like
@BeforeEach
,@AfterEach
, and@DisplayName
offer more control and readability in your tests. - Lambda Support: JUnit 5 uses functional interfaces, which means you can write more expressive and concise code.
For deeper insights, you might want to check out the official JUnit 5 documentation.
Setting Up Your Project
Before getting into the Ant configuration, it's essential to set up your Java project correctly.
-
Create a Directory Structure: Make a directory for your project. Inside, create
src/test/java
for your test files andlib
for the JUnit libraries.my-project/ ├── lib/ └── src/ └── test/ └── java/
-
Download JUnit 5 Libraries: You will need the following JAR files:
junit-jupiter-api-{version}.jar
junit-jupiter-engine-{version}.jar
junit-platform-launcher-{version}.jar
Download these files from the JUnit releases page.
-
Place the JAR Files: Place the downloaded JAR files in the
lib
directory.
Configuring Ant for JUnit 5
To run your JUnit 5 tests using Ant, you'll need to configure the build script (usually build.xml
). The following is a basic example of how to set up your build.xml
file to support JUnit 5.
<project name="MyJUnit5Project" default="test">
<property name="lib.dir" location="lib"/>
<property name="test.dir" location="src/test/java"/>
<property name="bin.dir" location="bin"/>
<path id="classpath">
<fileset dir="${lib.dir}">
<include name="*.jar"/>
</fileset>
<pathelement path="${bin.dir}"/>
</path>
<target name="compile">
<mkdir dir="${bin.dir}"/>
<javac srcdir="${test.dir}" destdir="${bin.dir}" classpathref="classpath"/>
</target>
<target name="test" depends="compile">
<java classname="org.junit.platform.console.ConsoleLauncher" fork="true">
<classpath refid="classpath"/>
<arg value="--scan-classpath"/>
</java>
</target>
</project>
Explanation of the Ant Build Script
- Property Definitions: We define properties for library, test, and binary directories for easy reference.
- Classpath: The
path
element constructs the classpath from all JAR files in thelib
directory. - Compile Target: This target compiles Java test files from the
src/test/java
directory into thebin
directory. - Test Target: This target executes the tests using the JUnit Platform Console Launcher, which is required to run JUnit 5 tests.
With this setup, you are ready to start writing your tests.
Writing Your First JUnit 5 Test
Now that Ant is configured, let's write a simple test to validate our setup. Create a new file called CalculatorTest.java
in the src/test/java
directory with the following content:
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
class CalculatorTest {
@Test
void addition() {
Assertions.assertEquals(5, 2 + 3, "2 + 3 should equal 5");
}
@Test
void subtraction() {
Assertions.assertEquals(1, 3 - 2, "3 - 2 should equal 1");
}
}
Explanation of the Test Code
- Annotations: We're using the
@Test
annotation to indicate that each method is a test case. - Assertions: Using
Assertions.assertEquals()
, we verify the expected outcomes. If the assertion fails, a message is provided for clarity.
Running Tests with Ant
To execute your tests, open a terminal, navigate to your project root directory, and run:
ant test
You should see output indicating the results of your tests. If everything is set up correctly, the console will display the results of the test cases.
Closing Remarks
Integrating JUnit 5 with Apache Ant might seem challenging at first, but following the steps outlined in this guide will help ease that burden. With a proper understanding of the JUnit 5 framework and an Ant build setup tailored for testing, you can effectively run and manage your Java tests.
For more help on Ant or JUnit 5, consider checking out these additional resources:
Feel free to leave comments or ask questions below if you need further assistance. Happy testing!