The Hidden Struggles of Adopting Java 8 in Legacy Systems
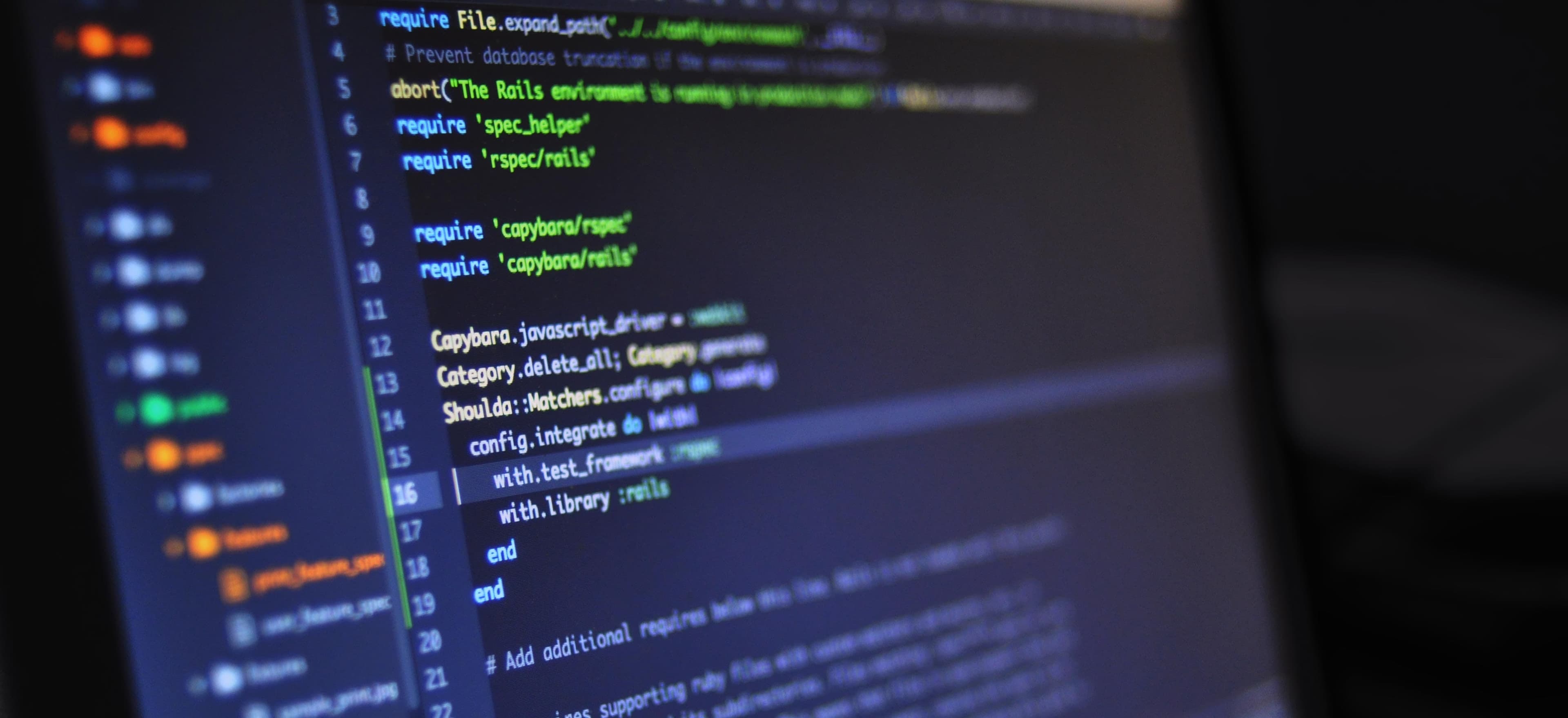
- Published on
The Hidden Struggles of Adopting Java 8 in Legacy Systems
Java has undergone significant evolution over the years, with each version bringing enhancements and features that improve the developer experience and application performance. One of the major updates, Java 8, introduced several powerful features, such as lambda expressions, streams, and the new Date and Time API. However, integrating Java 8 into legacy systems can present numerous challenges that might not be immediately apparent.
In this blog post, we will explore the hidden struggles of adopting Java 8 in legacy systems while providing insights and solutions to overcome these challenges.
Understanding Legacy Systems
Before delving into the challenges, it's crucial to understand what legacy systems are. These are typically older software systems that may rely on outdated technology, programming languages, and frameworks. Many businesses use these systems due to their reliability, historical significance, and the high cost of replacing or migrating them.
As organizations aim to modernize their technology stack, transitioning to Java 8 becomes attractive due to new features that can enhance application productivity and maintainability. However, this move is not without its pitfalls.
1. Compatibility Issues
One of the most significant challenges when adopting Java 8 is compatibility with the existing environment. Legacy applications might have been developed using older versions of Java, libraries, and frameworks that are incompatible with Java 8.
Example
Consider this example, where a legacy application uses an old JDBC driver. Upgrading to Java 8 could break this:
Connection conn = DriverManager.getConnection("jdbc:olddriver://localhost");
Why this Matters
If the JDBC driver is not updated to support Java 8, you may run into issues with connection establishment. The solution here is to identify and update all dependencies and libraries to their latest versions compatible with Java 8.
2. Code Refactoring
Legacy codebases are often filled with procedural programming constructs rather than modern object-oriented or functional paradigms that Java 8 promotes. Refactoring this code can be a daunting task.
Example
Here's an example showing the traditional loop versus a Java 8 stream:
Traditional Loop
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = new ArrayList<>();
for (String name : names) {
if (name.startsWith("A")) {
filteredNames.add(name);
}
}
Using Streams
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Why this Matters
While stream operations make code simpler and more readable, refactoring a large legacy codebase can introduce bugs and bugs during testing can be time-consuming. In some cases, business-critical components may be closely tied to specific implementations, making the process riskier.
3. Lack of Knowledge and Expertise
Many organizations have technical staff familiar with earlier Java versions but may lack proficiency in newer features introduced in Java 8.
The Solution
Investing in training or hiring experienced Java 8 developers is crucial. Online resources, like the official Java documentation or courses on platforms like Coursera and Udemy, can be invaluable sources of knowledge.
4. Performance Concerns
While Java 8 comes with performance improvements, some features like Streams can introduce overhead if implemented carelessly. Specifically, using parallel streams can lead to performance degradation in certain scenarios.
Example of Parallel Stream Misuse
List<String> largeList = getLargeList();
List<String> processedList = largeList.parallelStream()
.filter(this::someExpensiveOperation)
.collect(Collectors.toList());
Why this Matters
Using parallel streams indiscriminately can lead to performance issues when the underlying tasks are small or the data set is not sufficiently large. Conduct performance testing to ensure that the benefits of parallel processing are truly being realized.
5. Deploying the New Environment
Transitioning to Java 8 may necessitate upgrading the application servers where legacy systems run. This involves significant system changes, affecting dependencies, system properties, and configurations.
The Solution
Plan your deployment strategy carefully. Use testing environments that mirror production as closely as possible. Tools like Docker can assist in creating consistent environments that help ease the deployment process.
6. Regression Testing
Having a suite of tests for the legacy application before migration is essential, enabling the team to catch regressions introduced by the transition to Java 8. However, many legacy systems lack a proper testing framework.
Implementing Test Automation
Start implementing test automation using JUnit or TestNG. Write unit tests for critical functions and integration tests to cover interactions between different components.
import org.junit.Assert;
import org.junit.Test;
public class ExampleTest {
@Test
public void testFiltering() {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> result = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Assert.assertEquals(Collections.singletonList("Alice"), result);
}
}
Why this Matters
Automated tests ensure that legacy functionality remains intact throughout the migration. Also, consider using Mockito for mocking dependencies in your tests.
7. Change Management
Finally, change management is a critical aspect of any significant software upgrade. Employees may resist changes due to fear of disruption or lack of understanding of new features.
The Solution
Communicate transparently about the benefits of Java 8, offer training, and engage team members in the transition process. By demonstrating the benefits, you can alleviate concerns and foster a culture of innovation.
Bringing It All Together
Adopting Java 8 in legacy systems is a worthwhile endeavor, but it presents unique challenges. From compatibility issues and code refactoring to the need for extensive testing and change management, the path to modernization requires careful planning. However, by embracing the strategies outlined in this post, organizations can minimize risks and reap the benefits of modern Java features.
Navigating through this transition may seem daunting initially, but with a well-thought-out approach, the advantages of Java 8 can significantly enhance the performance and maintainability of your legacy systems.
For further reading, consider checking out the Java 8 Features page for in-depth technical details. Happy coding!