Common Issues with Android Broadcast Receivers Explained
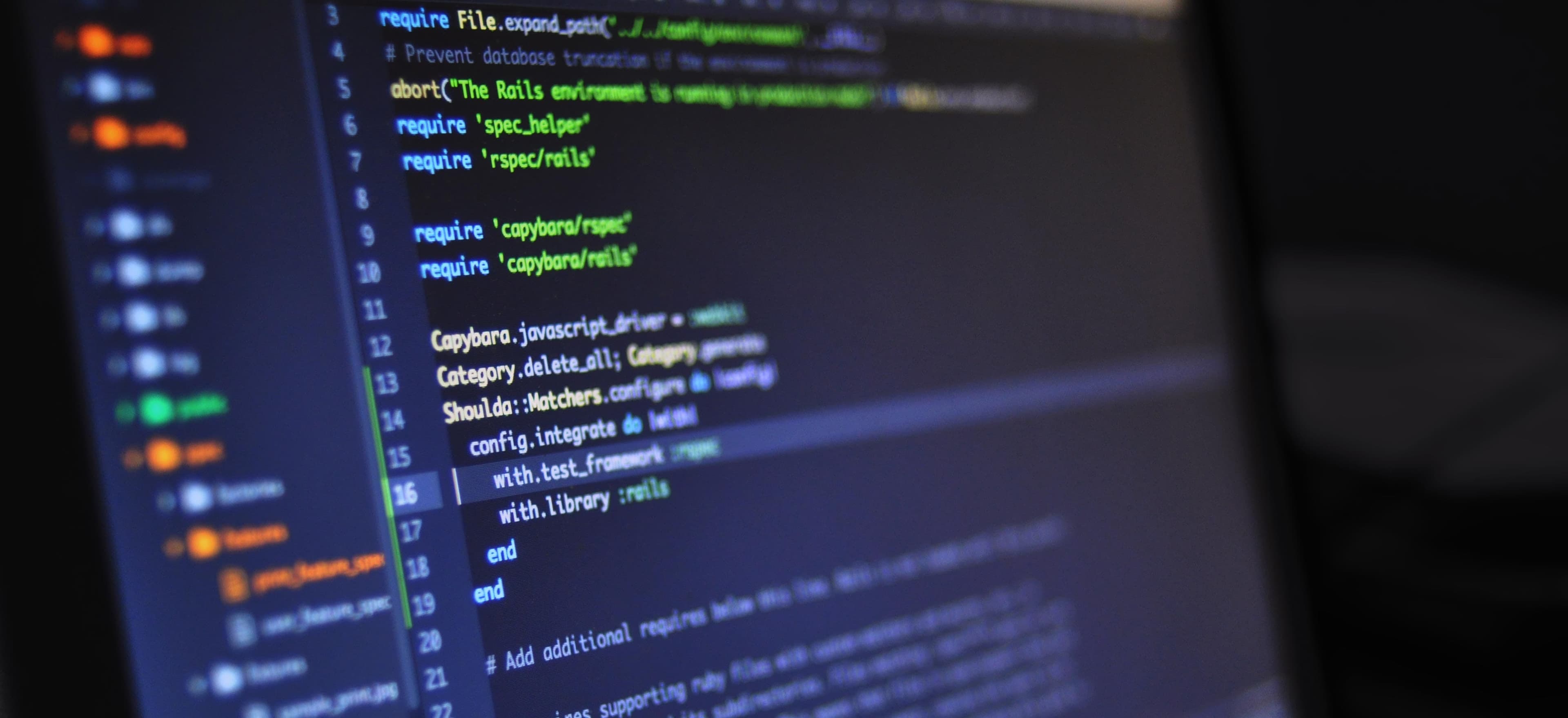
- Published on
Common Issues with Android Broadcast Receivers Explained
Android's Broadcast Receiver component plays a vital role in the event-driven architecture of the Android operating system. They are used for responding to various system-wide broadcast announcements, such as when the device is charging, when an incoming call is received, or when the network status changes. However, working with Broadcast Receivers can sometimes lead to common pitfalls that developers must navigate carefully. In this blog post, we will discuss these common issues, potential solutions, and provide illustrative examples to ensure your implementations are robust and error-free.
What Are Broadcast Receivers?
Before diving into the issues, let's briefly understand what a Broadcast Receiver is. A Broadcast Receiver is an Android component that allows applications to listen for and respond to broadcast messages from other applications or the system itself. They play a crucial role in enabling inter-application communication.
Basic Structure of a Broadcast Receiver
Here is a simple implementation of a Broadcast Receiver:
public class MyBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
// Code to execute when the broadcast is received
Log.d("MyBroadcastReceiver", "Broadcast received!");
}
}
In this code snippet, we define a MyBroadcastReceiver
class that extends BroadcastReceiver
. The onReceive
method is where you specify the actions to take when the broadcast is received.
Registering a Broadcast Receiver
You can register a Broadcast Receiver either in the AndroidManifest.xml file or dynamically in your application code.
Static Registration (AndroidManifest.xml)
<receiver android:name=".MyBroadcastReceiver">
<intent-filter>
<action android:name="com.example.MY_ACTION"/>
</intent-filter>
</receiver>
Static registration makes your receiver always active, even when your app is not running.
Dynamic Registration (in Code)
IntentFilter intentFilter = new IntentFilter("com.example.MY_ACTION");
MyBroadcastReceiver myReceiver = new MyBroadcastReceiver();
registerReceiver(myReceiver, intentFilter);
Dynamic registration is helpful for limiting the scope of the receiver's activity to specific lifecycle events.
Common Issues with Broadcast Receivers
- Receiver Not Triggered
One of the most common issues developers face is the receiver not being triggered when expected. This can occur due to incorrect action names in the Intent filter.
Solution
Ensure that the action string you're using in the intent filter exactly matches the action being broadcast. Strings in programming are case-sensitive.
Intent intent = new Intent("com.example.MY_ACTION");
sendBroadcast(intent); // Ensure this matches the action in your filter
- Memory Leaks
Dynamic registration can lead to memory leaks if not handled properly. When you register your receiver in an Activity but forget to unregister it, you're still holding a reference to that Activity, which prevents it from being garbage collected.
Solution
Always unregister the Broadcast Receiver in the onStop or onDestroy methods of your Activity or Fragment.
@Override
protected void onStop() {
super.onStop();
unregisterReceiver(myReceiver);
}
- Broadcasts Not Received After App Reboot
If you're sending broadcasts but not receiving them after the device reboots, this issue could be due to the receiver not being registered correctly for BOOT_COMPLETED.
Solution
To receive broadcasts after the device has booted, make sure to register the BOOT_COMPLETED
action in the manifest and add the appropriate permission.
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED"/>
<receiver android:name=".MyBootReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED"/>
</intent-filter>
</receiver>
Additionally, ensure that your receiver is capable of handling this event efficiently.
- Ordering Issues with Broadcasts
When multiple receivers are registered for the same action, they can interfere with one another. Broadcasts in Android are delivered in the order they are registered, which may not be what you expect.
Solution
Use setPriority()
on the IntentFilter
to manage order.
IntentFilter filter = new IntentFilter("com.example.MY_ACTION");
filter.setPriority(10); // Higher numbers have higher priority
registerReceiver(myReceiver, filter);
- Receiver Called Even When App is Closed
Static receivers defined in the manifest can still respond to broadcasts when the app is not running. This can drain resources or lead to unexpected behavior.
Solution
If you want to control this behavior, you should consider using dynamic registration, as mentioned earlier. This way, you have more control over when your receiver is active.
Best Practices
Understanding the common issues is a good first step, but implementing best practices is essential for creating efficient applications. Here are some best practices to consider:
- Use LocalBroadcastManager: If the communication is only needed within your app, consider using
LocalBroadcastManager
to prevent other apps from receiving your broadcast and improve performance.
LocalBroadcastManager.getInstance(context).sendBroadcast(new Intent("com.example.MY_ACTION"));
-
Minimize Work in onReceive: Keep the
onReceive
method lightweight. Perform heavy operations in a separate thread or a service to avoid blocking the main thread. -
Use Sticky Broadcasts Relevantly: Sticky broadcasts can be useful but be careful. They can hold onto memory longer than necessary and are deprecated in most scenarios.
-
Testing: Regularly test your receivers during development to ensure that all scenarios are handled accurately, including state changes, system shutdown, and app updates.
The Bottom Line
Broadcast Receivers are crucial to building responsive, interactive Android applications. Understanding common issues and their solutions empowers developers to create more resilient and user-friendly apps. By adhering to best practices, you can minimize the pitfalls usually associated with Broadcast Receivers.
For more detailed guidelines, you may refer to the official Android documentation on Broadcast Receivers.
By being aware of these common problems and employing the suggestions provided, you can enhance the reliability and performance of your Android applications. Happy coding!
Checkout our other articles