The Hidden Dangers of Using Global Variables in Programming
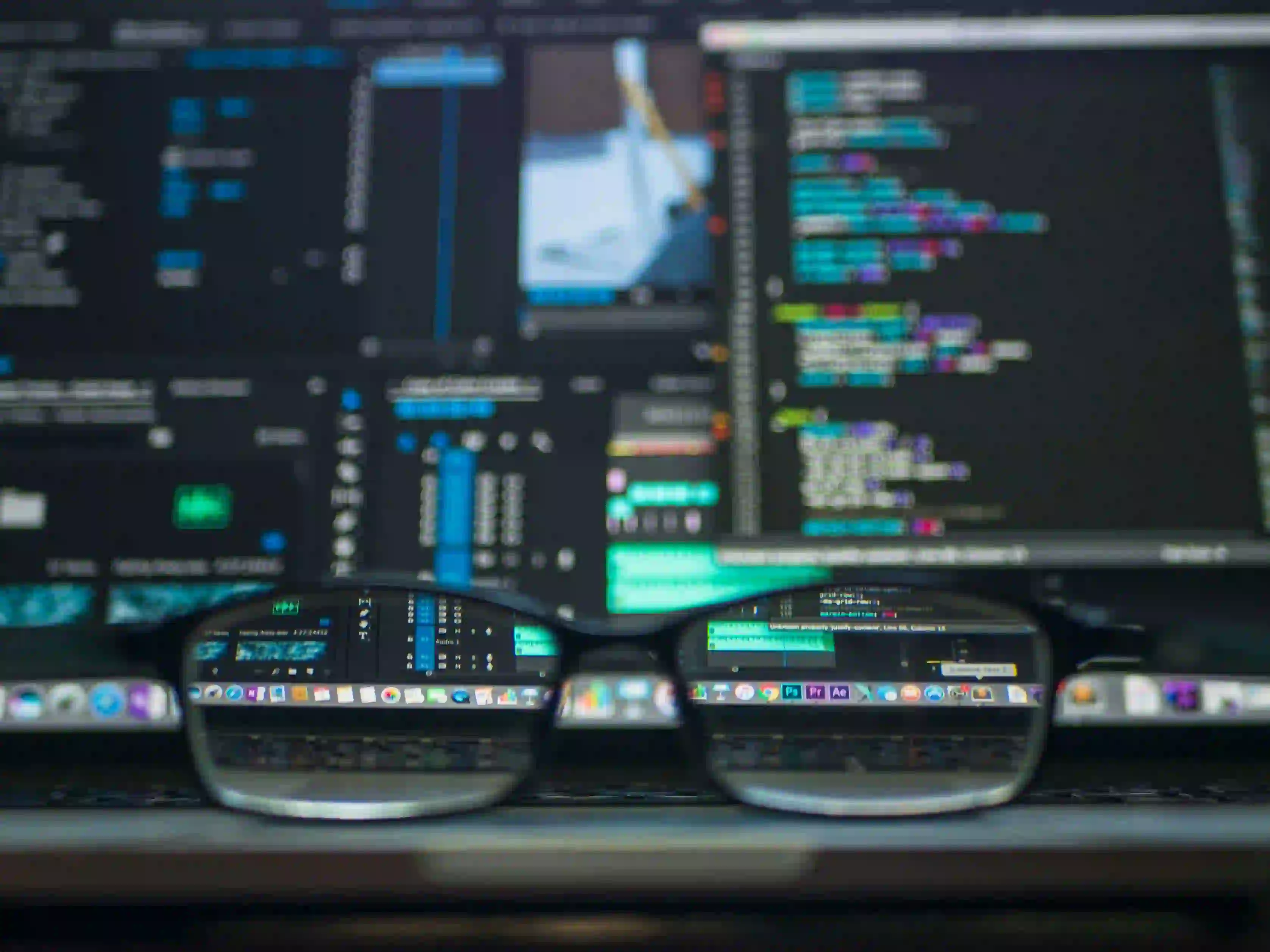
The Hidden Dangers of Using Global Variables in Programming
In the world of programming, variables typically serve as the backbone of functionality—holding data that code can manipulate. However, the way we define and use variables can significantly affect the integrity and maintainability of our code. One particularly controversial practice is the use of global variables. While they offer certain conveniences, they also carry hidden dangers that can lead to elusive bugs and unreliable code.
In this article, we'll delve into the pitfalls of using global variables in programming, especially in languages like Java. We will also explore alternatives that can make your code more robust, maintainable, and reusable.
What Are Global Variables?
Global variables are defined outside of any function or block, making them accessible from anywhere in the code. Here's a simple example to illustrate:
public class GlobalVariableExample {
static int globalNumber = 10; // Global variable
public static void main(String[] args) {
System.out.println("Initial global number: " + globalNumber);
modifyGlobalNumber();
System.out.println("Modified global number: " + globalNumber);
}
static void modifyGlobalNumber() {
globalNumber += 5;
}
}
In this example, globalNumber
is accessible both in the main
method and the modifyGlobalNumber
method. This convenience, however, comes at a cost.
The Dangers of Global Variables
1. Uncontrolled State Changes
One of the primary issues with global variables is that they can lead to uncontrolled state changes. In the example above, any method can alter the value of globalNumber
, potentially leading to unexpected behavior in the program. For instance, if multiple threads were to access and modify this variable concurrently, it could lead to race conditions, resulting in unpredictable outcomes.
public class ThreadExample {
static int globalCounter = 0;
public static void main(String[] args) {
Thread t1 = new Thread(() -> incrementCounter());
Thread t2 = new Thread(() -> incrementCounter());
t1.start();
t2.start();
}
static void incrementCounter() {
for (int i = 0; i < 1000; i++) {
globalCounter++;
}
System.out.println("Final global counter: " + globalCounter);
}
}
2. Difficulty in Debugging
When you use global variables, tracking down where and how a variable's value was changed becomes a cumbersome task. Without a controlled access point, a global variable can be altered in numerous locations throughout the code, complicating debugging and maintenance efforts.
3. Namespace Pollution
Global variables reside in a shared namespace, which increases the risk of naming collisions. If two parts of the program inadvertently declare global variables with the same name, it can lead to confusion and errors.
4. Coupling of Code
Global variables can tightly couple different parts of your codebase. If one section of the code changes how it uses a global variable, another unrelated section may inadvertently fail or behave unpredictably, making code reuse problematic.
Best Practices for Avoiding Global Variables
1. Encapsulation
Use encapsulation to restrict access to data. By keeping variables local to the classes and methods that need them, you enhance modularity.
public class EncapsulatedClass {
private int counter = 0; // Private variable
public void incrementCounter() {
counter++;
}
public int getCounter() {
return counter;
}
}
2. Dependency Injection
In larger systems, consider using dependency injection. This design pattern allows you to provide the dependencies that a class needs, promoting loose coupling and making the code easier to test.
3. Use Singleton for Shared Resources
If you need to share a variable across classes, consider the Singleton design pattern. It provides a controlled access point to global-like variables and ensures that only one instance exists.
public class Singleton {
private static Singleton instance;
private int value;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
The Last Word
While global variables may offer ease and convenience, the hidden dangers they introduce can lead to significant challenges in debugging, testing, and maintenance. Proper encapsulation, dependency injection, and intentional design through Singleton patterns can help mitigate these risks and create a more robust application architecture.
For a deeper understanding of design patterns, consider referencing The Gang of Four Design Patterns and the principles of SOLID programming.
Ultimately, as with any development practice, understanding the trade-offs is crucial. By being aware of the implications of global variables, programmers can write cleaner, more maintainable code that stands the test of time. Embrace best practices, and your code will thank you for it in the long run.