Mastering the Factory Pattern: Common Pitfalls to Avoid
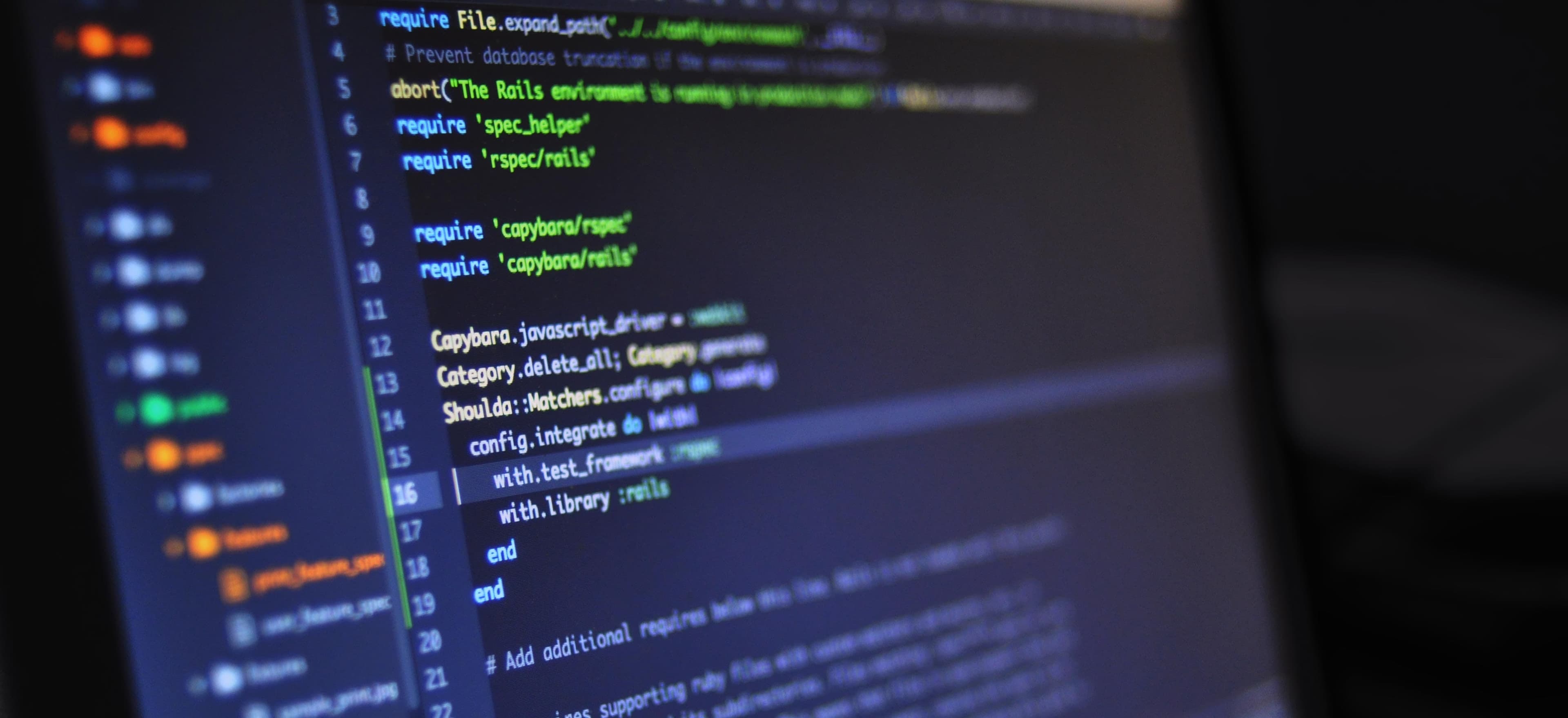
- Published on
Mastering the Factory Pattern: Common Pitfalls to Avoid
In the realm of software development, design patterns serve as elegant solutions to common problems developers face. One such pattern is the Factory Pattern. This article will delve into the intricacies of the Factory Pattern, common pitfalls developers encounter, and best practices to avoid them. By the end of this post, you’ll be equipped with the knowledge to implement this pattern effectively.
What is the Factory Pattern?
The Factory Pattern is a creational design pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. The main aim of the Factory Pattern is to decouple the creation of objects from their usage, promoting loose coupling and enhancing code maintainability.
Types of Factory Patterns
- Simple Factory: A static method to create objects.
- Factory Method: An interface for creating objects, but the instantiation is left to subclasses.
- Abstract Factory: An interface for creating families of related or dependent objects without specifying their concrete classes.
Benefits of the Factory Pattern
- Decoupling: Separates the instantiation process from the usage of the object.
- Flexibility: Enables the easy addition of new product types without altering existing code.
- Simplified Code: Reduces code complexity by centralizing instantiation logic.
Common Pitfalls in Implementing the Factory Pattern
While the Factory Pattern provides numerous advantages, developers can easily fall into several traps. Below are some common pitfalls and how to avoid them.
1. Overcomplicating the Factory
Pitfall
Developers often over-engineer the factory by adding unnecessary complexity. For instance, using multiple levels of abstract classes and interfaces can confuse the clients who will instantiate the objects.
Solution
Stick to simplicity. For example, here’s a basic implementation of a Factory Method in Java.
interface Shape {
void draw();
}
class Circle implements Shape {
public void draw() {
System.out.println("Drawing a Circle");
}
}
class Square implements Shape {
public void draw() {
System.out.println("Drawing a Square");
}
}
abstract class ShapeFactory {
abstract Shape createShape();
}
class CircleFactory extends ShapeFactory {
Shape createShape() {
return new Circle();
}
}
class SquareFactory extends ShapeFactory {
Shape createShape() {
return new Square();
}
}
In this example, even though we have a simple factory setup, you can still easily extend it with more shape classes or factories without additional complexity.
2. Not Following the Open/Closed Principle
Pitfall
When adding new products, developers sometimes modify existing factory code rather than extending it. This violates the Open/Closed Principle, which states that software entities should be open for extension but closed for modification.
Solution
Utilize polymorphism effectively. Here’s how you can do that:
class Triangle implements Shape {
public void draw() {
System.out.println("Drawing a Triangle");
}
}
class TriangleFactory extends ShapeFactory {
@Override
Shape createShape() {
return new Triangle();
}
}
By simply extending the factory and adding a new concrete product, you preserve existing code integrity and prevent it from breaking.
3. Hardcoding Types in Factory
Pitfall
Another common mistake is hardcoding product types inside the factory class. This approach makes the addition of new types cumbersome and tends to clutter the factory.
Solution
Utilize a configuration or mapping approach for type creation. Example:
class ShapeFactory {
public Shape createShape(String shapeType) {
if ("CIRCLE".equalsIgnoreCase(shapeType)) {
return new Circle();
} else if ("SQUARE".equalsIgnoreCase(shapeType)) {
return new Square();
} else {
throw new IllegalArgumentException("Unknown shape type");
}
}
}
In this scenario, if you wish to add a new shape, you only have to modify the factory method to add a new condition without disrupting other code.
4. Ignoring Thread Safety
Pitfall
In multi-threaded applications, factories can become thread-unsafe if they're not properly synchronized. This oversight can lead to inconsistent object states and unexpected behaviors.
Solution
Ensure thread safety in your factories. For example, if your factory maintains shared state, make use of synchronized blocks:
class ThreadSafeShapeFactory {
public synchronized Shape createShape(String shapeType) {
// Same implementation as above
}
}
5. Lack of Unit Tests
Pitfall
Omitting unit tests for the factory can lead to issues going undetected into production. Factories play a crucial role in object creation, and failing to test them may introduce subtle bugs.
Solution
Write thorough unit tests for your factory methods. Here's a sample JUnit test for the ShapeFactory
:
import static org.junit.Assert.*;
import org.junit.Test;
public class ShapeFactoryTest {
@Test
public void testCreateCircle() {
ShapeFactory factory = new ShapeFactory();
Shape shape = factory.createShape("CIRCLE");
assertTrue(shape instanceof Circle);
}
@Test(expected = IllegalArgumentException.class)
public void testCreateUnknownShape() {
ShapeFactory factory = new ShapeFactory();
factory.createShape("UNKNOWN");
}
}
Key Takeaways
Mastering the Factory Pattern is essential for every Java developer who seeks to build clean, maintainable code. The discussed pitfalls highlight the importance of simplicity, adherence to design principles, type management, thread safety, and testing.
By avoiding common mistakes and following best practices, you can leverage the Factory Pattern to create robust applications. For further reading, check out the official Java Design Patterns documentation and explore design patterns in-depth.
In summary, be mindful of these pitfalls, ensure your implementations are clean and organized, and you will reap the benefits of the Factory Pattern in your software development journey. Happy coding!
Checkout our other articles