Troubleshooting Spring Constructor Injection Issues
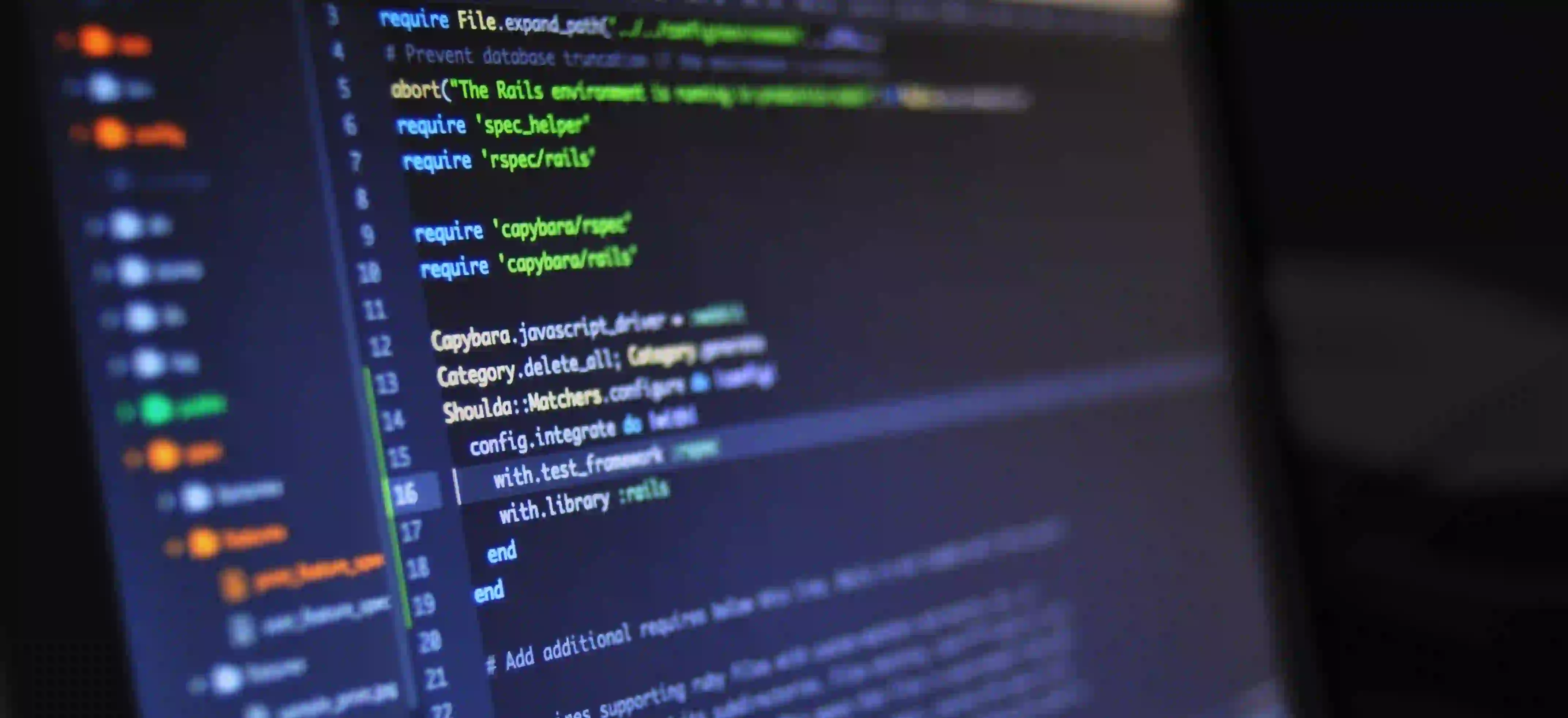
Troubleshooting Spring Constructor Injection Issues
Constructor injection is one of the three main ways to inject dependencies in Spring, with the other two being setter injection and field injection. While it promotes immutability and ensures that a bean is fully initialized before use, issues can arise during the configuration process that can lead to unexpected behavior. In this post, we’ll discuss common problems with constructor injection in Spring, their diagnosis, and solutions using relevant code snippets to illustrate each point.
What is Constructor Injection?
In Spring, constructor injection means providing the dependencies through a class constructor. This makes it easier to manage dependencies and keeps the codebase clean and maintainable.
@Component
public class BookService {
private final BookRepository bookRepository;
@Autowired
public BookService(BookRepository bookRepository) {
this.bookRepository = bookRepository;
}
}
In this example, BookService
relies on BookRepository
. The @Autowired
annotation tells Spring to inject an appropriate BookRepository
bean into the BookService
constructor.
Advantages of Constructor Injection
- Immutability: By using final fields, you ensure that once an object is created, it cannot change its dependencies.
- Easier Testing: Constructor injection makes unit testing easier as you can simply create an instance of a class along with its dependencies.
- Explicit Dependencies: It improves the readability of the code by clearly stating what dependencies are required by a class.
Common Issues with Constructor Injection
While constructor injection simplifies many aspects of dependency management in Spring, it can lead to several technical problems. Here are some of the most common issues:
1. No Qualifying Bean Found
Problem: You encounter an error stating that no qualifying bean of type X is available when the application context is starting.
Diagnosis: This error generally indicates that Spring could not find a bean definition that matches the type required by the constructor.
Solution: Double-check your Spring component scanning configurations. Ensure that the class you are trying to inject is annotated with @Component
, @Service
, @Repository
, or similar annotations. Additionally, for types that are not annotated, you might consider defining beans explicitly in your configuration class.
@Configuration
public class AppConfig {
@Bean
public BookRepository bookRepository() {
return new InMemoryBookRepository();
}
}
2. Circular Dependencies
Problem: If you have a situation where two or more beans depend on each other, Spring can throw a BeanCurrentlyInCreationException
.
Diagnosis: Circular dependency issues can lead to this error during the context initialization phase.
Solution: Break the circular dependency by refactoring your code. One common approach is to use setter injection for one of the components, thus eliminating the cycle.
@Component
public class UserService {
private final NotificationService notificationService;
@Autowired
public UserService(NotificationService notificationService) {
this.notificationService = notificationService;
}
}
@Component
public class NotificationService {
private UserService userService;
@Autowired
public void setUserService(UserService userService) {
this.userService = userService;
}
}
3. Constructor Argument Type Mismatches
Problem: If the argument type expected by the constructor does not match any available bean, you will receive a UnsatisfiedDependencyException
.
Diagnosis: This typically occurs because either the type of dependency is wrong or there is a misconfiguration in your Spring context.
Solution: Double-check the type names and ensure that the correct class is being injected. You can also use the @Qualifier
annotation when multiple beans of the same type exist.
@Autowired
public UserService(@Qualifier("hibernateBookRepository") BookRepository bookRepository) {
this.bookRepository = bookRepository;
}
4. Missing @Autowired Annotation
Problem: Forgetting to use the @Autowired
annotation can lead to constructor injection not occurring.
Diagnosis: If the dependency is not auto-wired, you will likely encounter a NullPointerException
when trying to use the class.
Solution: Verify that you have applied the @Autowired
annotation on your constructor.
5. Improper Configuration File
Problem: If you are using XML-based configuration and the file is not properly set up, the Spring context may fail to initialize.
Diagnosis: Check the XML configuration file for errors or missing bean definitions.
Solution: Ensure that the XML file correctly defines beans and that it is loaded in your application context.
<bean id="bookRepository" class="com.example.repository.BookRepository"/>
<bean id="bookService" class="com.example.service.BookService">
<constructor-arg ref="bookRepository"/>
</bean>
Best Practices for Constructor Injection
- Use Final Fields: Declare your dependencies as
final
to enforce immutability. - Keep Constructor Simple: A constructor should not have more than 3-4 parameters to maintain comprehensibility.
- Consider Optional Dependencies: For optional dependencies, you may use either the
@Autowired(required = false)
attribute or theOptional<>
class in Java.
public BookService(@Autowired(required = false) Optional<BookRepository> bookRepository) {
this.bookRepository = bookRepository.orElseThrow(() -> new IllegalArgumentException("BookRepository is required"));
}
- Document Dependencies: It's a good practice to comment on what each dependency does, particularly for larger, more complex classes.
The Bottom Line
Understanding constructor injection is vital for any Spring developer, as it directly influences how dependencies are managed. Remember that while Spring provides excellent tools to manage beans, it's crucial to be proactive in diagnosing and resolving issues that may arise. By following the best practices shared in this article, you can minimize potential pitfalls and maintain clean, efficient code.
For more detailed insights into dependency injection, check the official Spring documentation on Spring Dependency Injection. This will further solidify your understanding of dependency management in your applications.
Feel free to share your experiences or questions regarding constructor injection troubleshooting in the comments below!