Debugging ReactJS in Java: Bridging Frameworks for Success
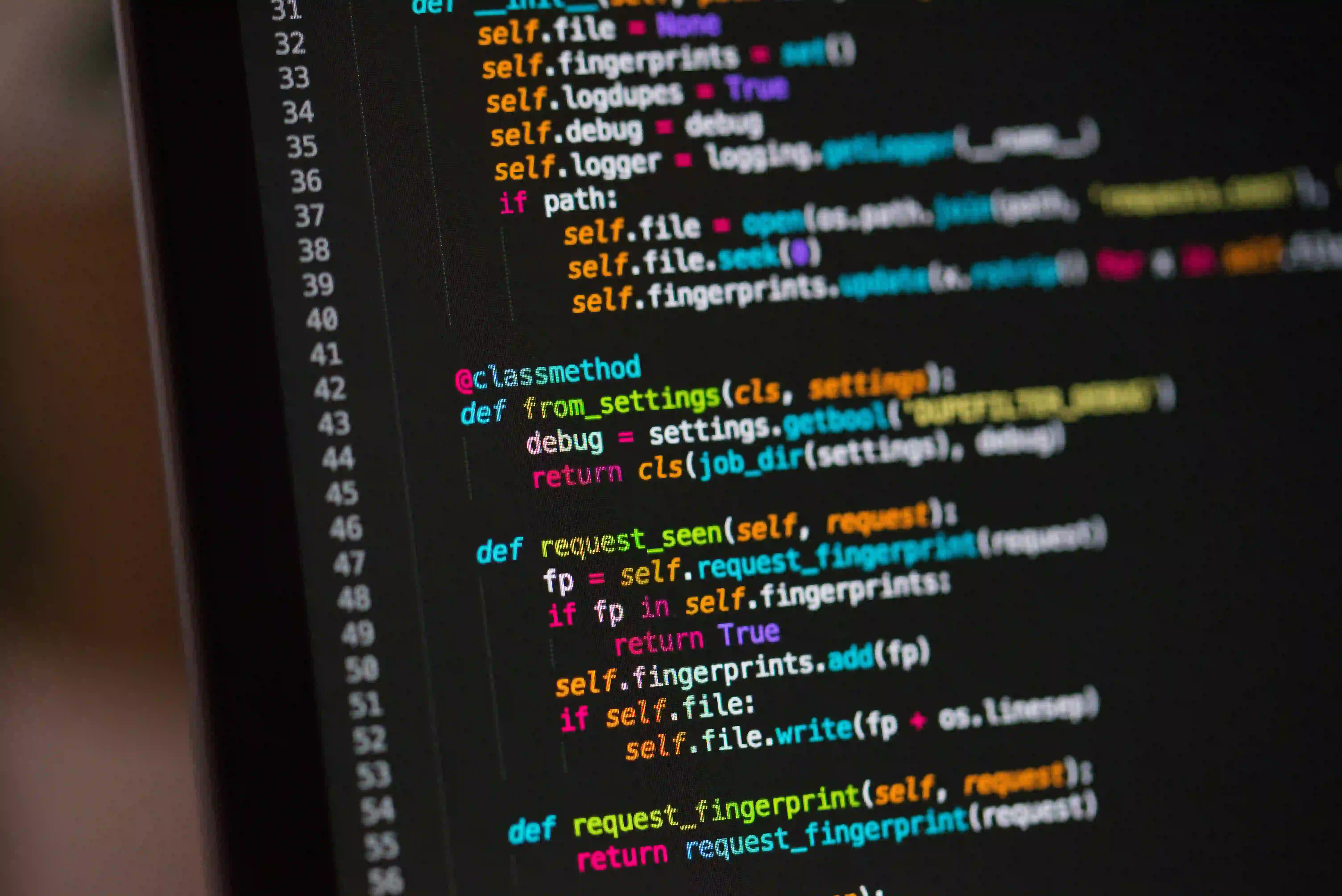
Debugging ReactJS in Java: Bridging Frameworks for Success
When it comes to web development, the intersection of front-end libraries like ReactJS and back-end languages like Java often prevails. Being proficient in both is crucial for building modern web applications. In this blog post, we will explore effective debugging techniques for ReactJS code while leveraging Java-based frameworks. We will cover code snippets, discuss common pitfalls, and guide you through the complex process of debugging to make your development experience as smooth as possible.
Understanding the ReactJS Landscape
ReactJS, a JavaScript library for building user interfaces, is known for its declarative style and component-based architecture. One of the common challenges developers face is debugging their ReactJS applications. As you develop rich UIs, minor errors can lead to significant issues, ultimately hampering user experience.
To gain a deeper understanding of how to tackle these challenges, be sure to check out Why Your ReactJS Code Isn't Working: Common Mistakes. This resource outlines some of the most frequent mistakes developers make, including issues with state management and prop handling.
Debugging Techniques for ReactJS
1. Console Logging
Console logging is one of the simplest yet most effective debugging techniques. By printing the values of variables and states to the console, you can follow the flow of your application and diagnose problems with ease.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
console.log("Before increment:", count); // Log before state update
setCount(count + 1);
console.log("After increment:", count); // Log after state update
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
Why this works: Logging before and after significant state changes helps you visualize data flow and track how state updates impact the rest of your components. This is useful because React's state updates may not be immediately reflected after a setCount
call due to asynchronous behavior.
2. React Developer Tools
React Developer Tools is a browser extension that offers additional debugging capabilities, allowing you to inspect your React component hierarchy.
- Install the extension for Chrome or Firefox.
- Open your application and click on the React tab.
- Inspect the props and state of your components.
This is a game changer for understanding how your components interact and for diagnosing misbehaviors caused by incorrect prop values or state states.
3. Error Boundaries
Error boundaries are a React feature that provides a way to catch JavaScript errors anywhere in the child component tree, log those errors, and display a fallback UI.
import React from 'react';
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
console.log("Logging error:", error);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
Why use error boundaries: They ensure that a JavaScript error in one part of the UI does not affect the entire application. Using them helps you maintain UI integrity while allowing you to catch specific errors.
Bridging ReactJS with Java
As your front-end code grows, it's essential to establish clear communication with your back-end services, often built using frameworks like Spring or Jakarta EE. These frameworks facilitate data handling and state management, which are crucial components for any robust application.
RESTful APIs in Java
Building a RESTful API using Spring Boot can be a great way to serve data to your ReactJS application. Asking for data via HTTP requests can sometimes lead to network errors, or you might be consuming the wrong endpoint.
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public ResponseEntity<List<User>> getAllUsers() {
List<User> users = userService.getAllUsers();
return new ResponseEntity<>(users, HttpStatus.OK);
}
}
Why it matters: Providing a clear API endpoint helps your React application know where to get data, thus streamlining development. Debugging here involves ensuring that your API responds correctly and that the front end handles the data as expected.
Handling Fetch Requests in React
Integrating with your Java back-end is seamless with modern JavaScript features like fetch
. Here’s a snippet to show how you can get data from your Java-based service:
import React, { useEffect, useState } from 'react';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('/api/users')
.then(response => response.json())
.then(data => {
setUsers(data);
console.log(data); // Log fetched data for debugging
})
.catch(error => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h2>User List</h2>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
}
export default UserList;
Why utilize fetch: The fetch
API is a built-in JavaScript function that allows communication with your Java back-end. Logging errors can provide insight into issues like network failures or unexpected responses.
Common Errors in ReactJS
Even with the right tools, errors will occur. Here are some common errors and how to debug them:
- Incorrect Prop Types: Utilizing prop types in your components can help catch bugs early.
- State Not Updating: Recognizing when state updates are asynchronous is critical. Use logging to trace state flow.
- Uncaught Errors: Use try-catch blocks when necessary and consider implementing a global error handler.
My Closing Thoughts on the Matter
Debugging your ReactJS applications while integrating them with a Java back-end can certainly be challenging. However, mastering a few key techniques can make all the difference. From basic console logging to more sophisticated tools like error boundaries and React Developer Tools, you will find that debugging is an integral part of the development process.
For advanced error-checking and common pitfalls, look back at the insightful article, Why Your ReactJS Code Isn't Working: Common Mistakes. By embracing debugging best practices and bridging the gap between front-end and back-end technologies, you can build better, more robust, and user-friendly applications.
Happy coding!