Mastering MyBatis: Common Mapping Pitfalls to Avoid
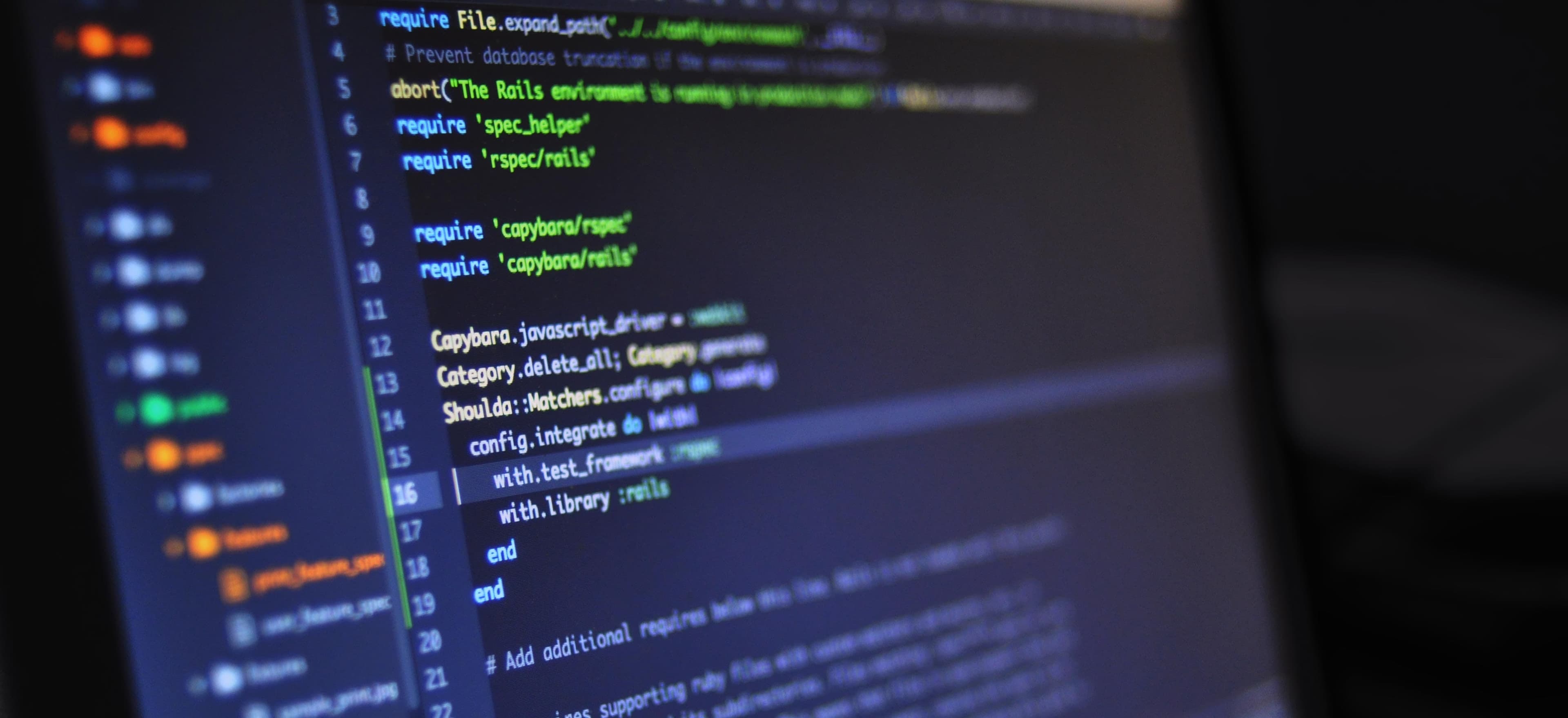
- Published on
Mastering MyBatis: Common Mapping Pitfalls to Avoid
MyBatis is a popular persistence framework in Java that facilitates database operations. It offers flexibility and control by allowing developers to write SQL queries directly. However, like all powerful tools, it can lead to pitfalls if not used correctly. In this blog post, we will discuss the common mapping pitfalls you may encounter when using MyBatis, and how to avoid them, ensuring efficient data transactions in your applications.
What is MyBatis?
MyBatis is an open-source persistence framework primarily used for data mapping in Java applications. Unlike other ORM frameworks like Hibernate, it focuses on mapping SQL statements to objects in an environment where you control SQL queries directly. This gives you a high degree of flexibility for optimizing queries according to your specific needs.
Common Mapping Pitfalls
1. Lack of Proper Result Mapping
Pitfall: Failing to define appropriate mapping for the results returned from SQL queries can lead to null
values or runtime exceptions.
Solution: Always specify the result type in your MyBatis configuration. This ensures MyBatis can map the SQL query results to the appropriate Java objects.
<select id="selectUser" resultType="User">
SELECT id, username, password FROM users WHERE id = #{id}
</select>
In this example, specifying resultType="User"
tells MyBatis how to map the SQL results into a User
object. Without this, MyBatis would not know how to populate the fields of the User
class.
2. Striking a Balance with SQL Queries
Pitfall: Overcomplicating SQL queries can lead to harder-to-maintain code and poor performance. Complex queries can also result in issues with object mapping.
Solution: Strive for clarity and simplicity in your SQL statements. Consider breaking down complex operations into smaller, manageable queries.
<select id="getUsersByStatus" resultType="User">
SELECT * FROM users WHERE status = #{status}
</select>
Here, we keep the SQL simple and focused. This avoids the complexity of joins and nested queries, leading to easier debugging and maintenance.
3. Inconsistent Naming Conventions
Pitfall: Utilizing inconsistent naming conventions between your database schema and Java objects can lead to confusion and mapping failures.
Solution: Stick to a unified naming convention across your database and Java classes. This makes it easier to understand and maintain your application.
For example, if your database columns are named user_id
, ensure your Java class has a corresponding field like userId
.
4. Ignoring Lazy Loading
Pitfall: Not utilizing lazy loading can result in fetching unnecessary data. This can severely impact performance, especially with large datasets.
Solution: Leverage the lazy loading feature of MyBatis by deferring the loading of certain associations until they are actually needed.
<resultMap id="userResultMap" type="User">
<id property="id" column="id"/>
<result property="orders" column="id" select="getOrdersByUserId" fetchType="lazy"/>
</resultMap>
In this result map, we specify that the user's orders should only be loaded when explicitly accessed, thus optimizing performance.
5. Not Handling Null Values Correctly
Pitfall: Assuming that all fields will always have values can lead to NullPointerExceptions
.
Solution: Implement proper checks in your SQL queries or Java objects to handle nullable fields.
<select id="selectUser" resultType="User">
SELECT id, username, password FROM users WHERE id = #{id}
</select>
In your Java code, ensure to handle potential null values carefully.
User user = sqlSession.selectOne("selectUser", userId);
if (user != null) {
// Proceed with user information
} else {
// Handle the case for a non-existing user
}
6. Overusing XML Configuration
Pitfall: Relying solely on XML configuration for MyBatis can lead to complex configurations and harder-to-read code.
Solution: Utilize annotations for simpler queries or when working with straightforward functionality. This can reduce the boilerplate code.
@Mapper
public interface UserMapper {
@Select("SELECT * FROM users WHERE id = #{id}")
User selectUserById(int id);
}
Using annotations like @Select
streamlines the code and makes it more readable while still providing the necessary SQL functionality.
7. Ignoring the Benefits of Caching
Pitfall: Neglecting the caching mechanisms built into MyBatis can lead to performance bottlenecks, particularly in high-load applications.
Solution: Use MyBatis’s built-in caching capabilities effectively. Set up level 1 and level 2 caches to optimize repeated queries.
<configuration>
<settings>
<setting name="cacheEnabled" value="true"/>
</settings>
</configuration>
This configuration enables caching at the MyBatis level, helping you minimize database hits for frequently accessed data.
The Closing Argument
Mastering MyBatis and avoiding common mapping pitfalls requires an understanding of its features and best practices. By ensuring proper result mapping, maintaining consistency in naming, optimizing SQL queries, handling lazy loading, managing null values, utilizing annotations over XML where appropriate, and leveraging caching, you can create a more efficient and maintainable data access layer.
For additional resources, consider checking out:
- MyBatis Documentation
- Understanding Caching in MyBatis
By implementing these strategies, you’ll not only improve the performance of your applications but also enhance your development experience in Java. Happy coding!
Checkout our other articles