Common Mistakes to Avoid When Writing Test Cases
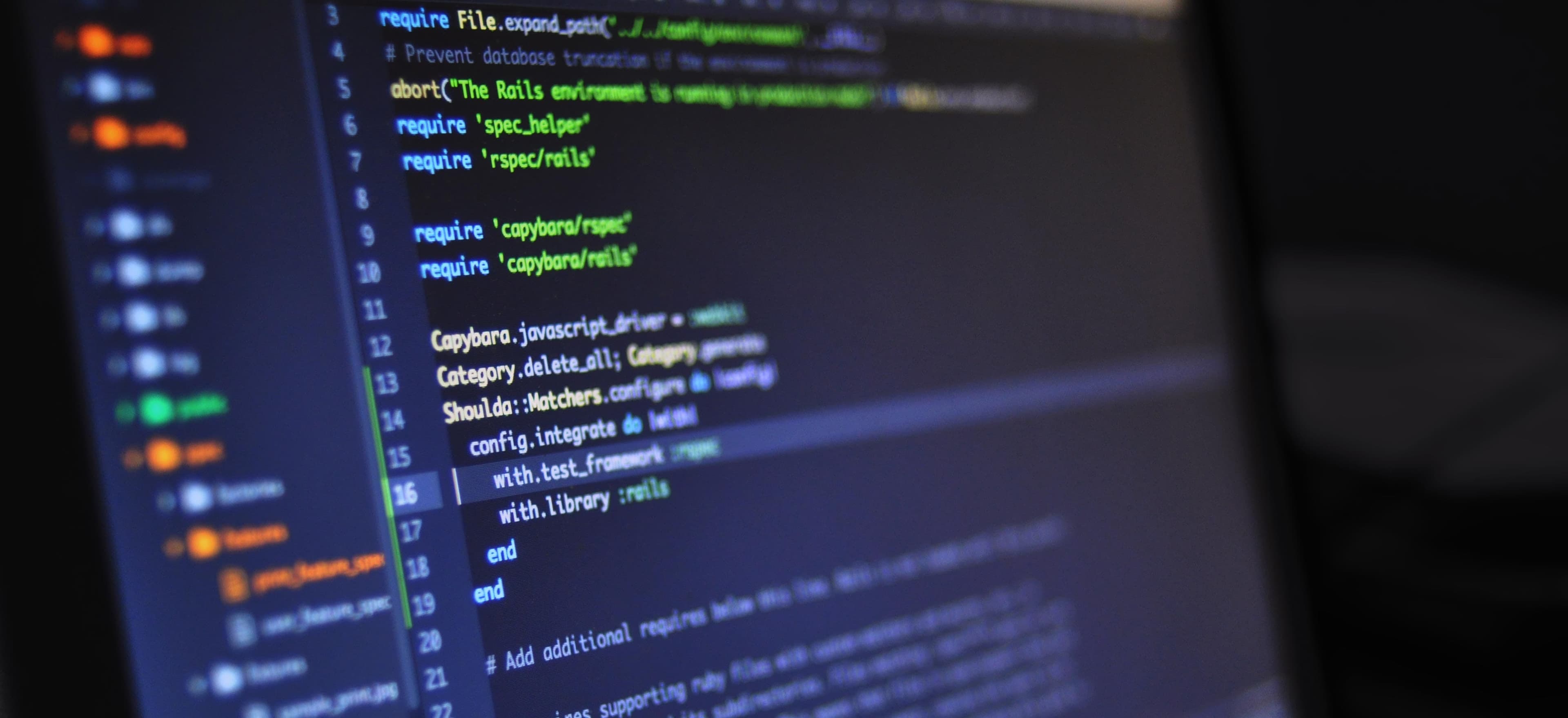
- Published on
Common Mistakes to Avoid When Writing Test Cases
Writing effective test cases is crucial for successful software development. Test cases not only validate functionality but also ensure that the software behaves as expected under various conditions. However, many developers and testers make common mistakes that undermine the effectiveness of their test cases. In this blog post, we'll discuss these common pitfalls and offer guidance on how to write better test cases for your applications.
1. Lack of Clarity
The Mistake
One of the most prevalent mistakes when writing test cases is a lack of clarity in the language used. Test cases should be easily understandable not only by the developer or the tester but also by stakeholders, such as product owners or project managers.
Why It Matters
Clarity ensures that anyone reading the test case can comprehend its purpose and logic without confusion, which promotes better communication within the team.
How to Improve
Use Simple Language: When documenting your test cases, opt for simple and plain language. Avoid jargon unless absolutely necessary, and explain any technical terms.
Example:
Test Case Title: Verify User Login
Preconditions: User must have a registered account.
Steps:
1. Navigate to the login page.
2. Enter the username and password.
3. Click the 'Login' button.
Expected Result: The user should be redirected to the dashboard.
Commentary: This example contains clear and straightforward language that delineates the objective, steps, and expected outcome.
2. Incomplete Test Coverage
The Mistake
Another common pitfall is incomplete test coverage, where test cases miss critical paths or scenarios.
Why It Matters
Incomplete test coverage can lead to undiscovered bugs that may surface in production, increasing costs and damaging user trust.
How to Improve
Identify Edge Cases: Make a comprehensive list of possible inputs and conditions, including both positive and negative scenarios.
Example: Consider a login function. Test cases should include:
- Valid and invalid credentials
- Empty fields
- SQL injection attempts
- Passwords with special characters
Test Coverage Matrix: Create a matrix or a requirement traceability matrix to ensure all features are tested.
3. Writing Too Many Redundant Tests
The Mistake
Redundant test cases can clutter your test suite, making it hard to navigate and maintain.
Why It Matters
Redundant tests waste resources and increase the time it takes to run your test suite, which slows down the development process.
How to Improve
Consolidate Similar Tests: Review your test cases periodically to identify duplicates. Use parameterized tests where applicable.
Example: Instead of writing separate tests for each password complexity requirement, you can write one parameterized test that checks all combinations of valid and invalid passwords.
@ParameterizedTest
@ValueSource(strings = { "Password123!", "P@ssw0rd" })
void testValidPasswords(String password) {
assertTrue(isPasswordValid(password));
}
@ParameterizedTest
@ValueSource(strings = { "", "abc", "short" })
void testInvalidPasswords(String password) {
assertFalse(isPasswordValid(password));
}
Commentary: In this example, we reduce redundancy by consolidating valid and invalid password tests into parameterized tests.
4. Ignoring Maintenance
The Mistake
Neglecting maintenance of test cases can lead to a bloated test suite filled with outdated or irrelevant tests.
Why It Matters
As applications evolve, so too should the test cases. If they are not updated, tests will fail without clear reasons, frustrating developers.
How to Improve
Regularly Review and Refactor: Incorporate test case reviews in your development cycle. Refactor tests that no longer serve a purpose.
Example: If a user interface changes, you should update your UI-related test cases accordingly. If features are deprecated, remove those test cases.
5. Poorly Defined Preconditions and Postconditions
The Mistake
Another frequent mistake is failing to properly define preconditions and postconditions for test cases.
Why It Matters
Clearly defined conditions provide context and help testers understand the necessary setup for the test, ensuring reliable test execution.
How to Improve
Detail All Preconditions: Explicitly outline what must happen before the test can run and what should be true after the test is executed.
Example:
Test Case Title: Update User Profile
Preconditions: User is logged in and on the profile page.
Postconditions: User profile is updated with new information.
Commentary: Defining preconditions and postconditions helps clarify the requirements of the test's environment.
6. Overly Complex Test Cases
The Mistake
Overly complex test cases can hinder the testing process and make debugging difficult.
Why It Matters
Complex tests can create confusion and are more prone to failures due to their interconnected paths.
How to Improve
Break Down Into Smaller Tests: When possible, divide complex tests into smaller, more manageable components.
Example: Instead of testing several functionalities of a user profile in one test, separate them into "Update Address," "Change Password," and "Upload Profile Picture."
7. Lack of Prioritization
The Mistake
Failing to prioritize test cases can lead to inefficiencies, especially when testing time is limited.
Why It Matters
Prioritizing tests based on risk and functionality allows you to address the most critical areas first.
How to Improve
Risk-based Testing: Use a risk-based approach to determine which tests should be executed first, focusing on business-critical functionalities.
Example:
- High Priority: User Authentication, Payment Processing
- Medium Priority: User Profile Update
- Low Priority: Cosmetic Changes
A Final Look
Writing effective test cases is both an art and a science. By avoiding the common mistakes highlighted in this blog post, you can enhance the reliability of your testing process and ensure that your software meets business needs.
To summarize:
- Ensure clarity and simplicity in language.
- Maintain complete test coverage including edge cases.
- Avoid redundancy by consolidating similar tests.
- Regularly review and refactor your test cases to maintain relevance.
- Clearly define preconditions and postconditions.
- Simplify overly complex tests by breaking them down.
- Prioritize tests based on risk and functionality.
Taking the time to implement these best practices will not only improve the quality of your test cases but will also contribute to the overall health of your software development lifecycle.
For more insights on writing effective test cases and improving your testing strategies, you may want to browse resources such as Guru99 or Software Testing Help. Happy testing!
By following these guidelines, you can avoid common pitfalls and enhance the delivery of quality software.