Common Git Flow Mistakes and How to Avoid Them
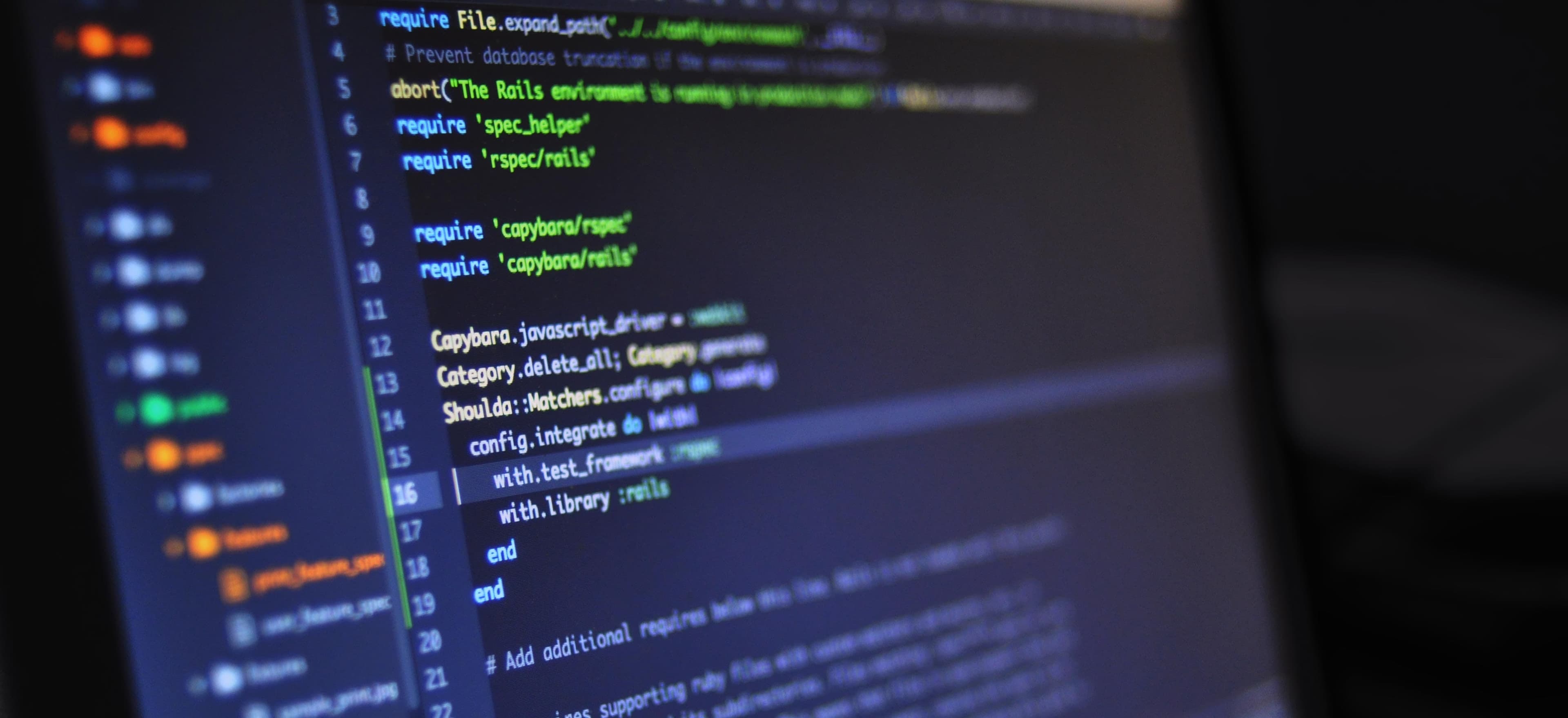
- Published on
Common Git Flow Mistakes and How to Avoid Them
Git is a powerful version control system that streamlines collaboration and helps manage changes in code effectively. However, as with any tool, users can encounter pitfalls. This blog post aims to highlight common Git Flow mistakes and offer practical tips on how to avoid them.
Understanding Git Flow
Before diving into mistakes, it’s important to have an understanding of Git Flow. Git Flow is a branching model for Git, designed by Vincent Driessen. It distinguishes between different branches:
- Master: The main production branch.
- Develop: The integration branch for features.
- Feature branches: Used to develop new features.
- Release branches: For preparing for production releases.
- Hotfix branches: To quickly address production issues.
Understanding these branches and their purposes is key to using Git effectively. Now, let’s take a look at common mistakes developers make when using Git Flow.
Common Mistakes
1. Ignoring Branch Naming Conventions
Naming conventions are crucial for maintaining clarity in a project. Failure to adhere to these can lead to confusion about which branch serves which purpose.
Example Mistake:
Using non-descriptive names like myfeature
instead of feature/user-authentication
.
How to Avoid:
Establish and document a clear naming convention for your branches. For instance, use prefixes to categorize branches:
feature/
for new featuresbugfix/
for bug fixeshotfix/
for urgent bug addresses
2. Not Keeping Your Branch Updated
One of the principal mistakes developers make is neglecting to regularly synchronize their feature branches with the develop
branch. This can lead to larger merge conflicts later.
Example Mistake:
Developing on an outdated branch which conflicts with the latest changes.
How to Avoid:
Regularly fetch and rebase from the develop
branch. Use the following commands:
git fetch origin
git checkout feature/my-feature
git rebase origin/develop
This keeps your branch updated and minimizes merge conflicts.
3. Committing Large Changes
Another common mistake is committing a large number of changes at once. This makes it challenging to review, leads to messy commit history, and can introduce numerous bugs.
Example Mistake:
A single commit with a whole new module and a set of bug fixes bundled together.
How to Avoid:
Break down changes into smaller, logical commits. Each commit should represent a single change or related set of changes. Use the following structure:
git commit -m "Add user authentication module"
git commit -m "Fix bug in user login"
This enhances the clarity of your project’s history.
4. Forgetting to Pull Requests
In many environments, team collaboration relies on pull requests (PRs) to review code before it is merged into develop
. Skipping this step can undermine code quality.
Example Mistake:
Merging a feature branch directly into develop
without a PR.
How to Avoid:
Encourage a pull request culture in your team. Always create a PR and get feedback before merging. This ensures code is reviewed and discussed.
5. Not Writing Descriptive Commit Messages
Poor commit messages can lead to confusion and difficulty when tracking changes over time. Commit messages serve as documentation for the history of a project.
Example Mistake:
Using vague messages like fixed stuff
.
How to Avoid:
Follow a consistent format for commit messages. A widely accepted format is:
<type>(<scope>): <subject>
<body>
Example:
feature(auth): add password reset functionality
- Implement reset password logic.
- Create email service for sending reset links.
6. Overusing Force Push
Forcing a push (git push --force
) can overwrite changes in the remote repository, causing potential loss of work for others.
Example Mistake:
Using --force
after rebasing without clearing communication with the team.
How to Avoid:
Only use force push in isolated scenarios when you are sure others are not relying on that branch. When collaboration is involved, prefer using git push --force-with-lease
, which protects against overwriting others' work.
7. Failing to Branch for Hotfixes
Sometimes issues arise that need immediate attention. Failing to create a hotfix branch can lead to unintended changes being pushed to production.
Example Mistake:
Pushing a fix directly to develop
and later realizing it needs to be in production.
How to Avoid:
Always create hotfix branches from master
for urgent fixes. The command would look like this:
git checkout -b hotfix/fix-issue master
This helps isolate the changes and goes through proper processes for a production fix.
8. Not Testing Changes Before Merging
Before merging changes into develop
or master
, it's crucial to test them. Skipping this step can introduce bugs into the main branch.
Example Mistake:
Merging changes without running tests that could have caught issues in functionality.
How to Avoid:
Implement a robust testing process with Continuous Integration (CI) tools like Jenkins or Travis CI. Ensure tests are run before a pull request is merged.
9. Not Using Git Tags
Tags are useful for marking specific points in your repository’s history as important, for instance, releases. Neglecting to tag releases results in lost historical reference points.
Example Mistake:
Deploying version 1.0.0
without tagging it.
How to Avoid:
Use tags for every release with clear versioning. The tags can be created using:
git tag -a v1.0.0 -m "Release version 1.0.0"
git push origin v1.0.0
10. Poor Documentation
In a team environment, failing to document your Git processes can lead to inconsistency and confusion among team members.
Example Mistake:
Assuming every team member knows the workflow without any formal process.
How to Avoid:
Maintain clear, accessible documentation of your Git Flow, including branching strategy, commit message guidelines, and the pull request process. Tools like Markdown or wikis can efficiently house this information.
To Wrap Things Up
Mastering Git Flow takes practice, but being aware of common mistakes is the first step in improving your workflow. By adopting better branch naming conventions, keeping branches updated, committing logical changes, utilizing pull requests, and ensuring thorough documentation, you can enhance your use of Git Flow.
To dive deeper into Git best practices, visit the Atlassian Git Tutorials and consider contributing to open-source projects to gain real-world experience.
In conclusion, mastering Git Flow is not just about technical proficiency but also about fostering a collaborative team environment that values quality, efficiency, and organization. Happy coding!