Stop Formatting Dates: Embrace SQL Date Literals!
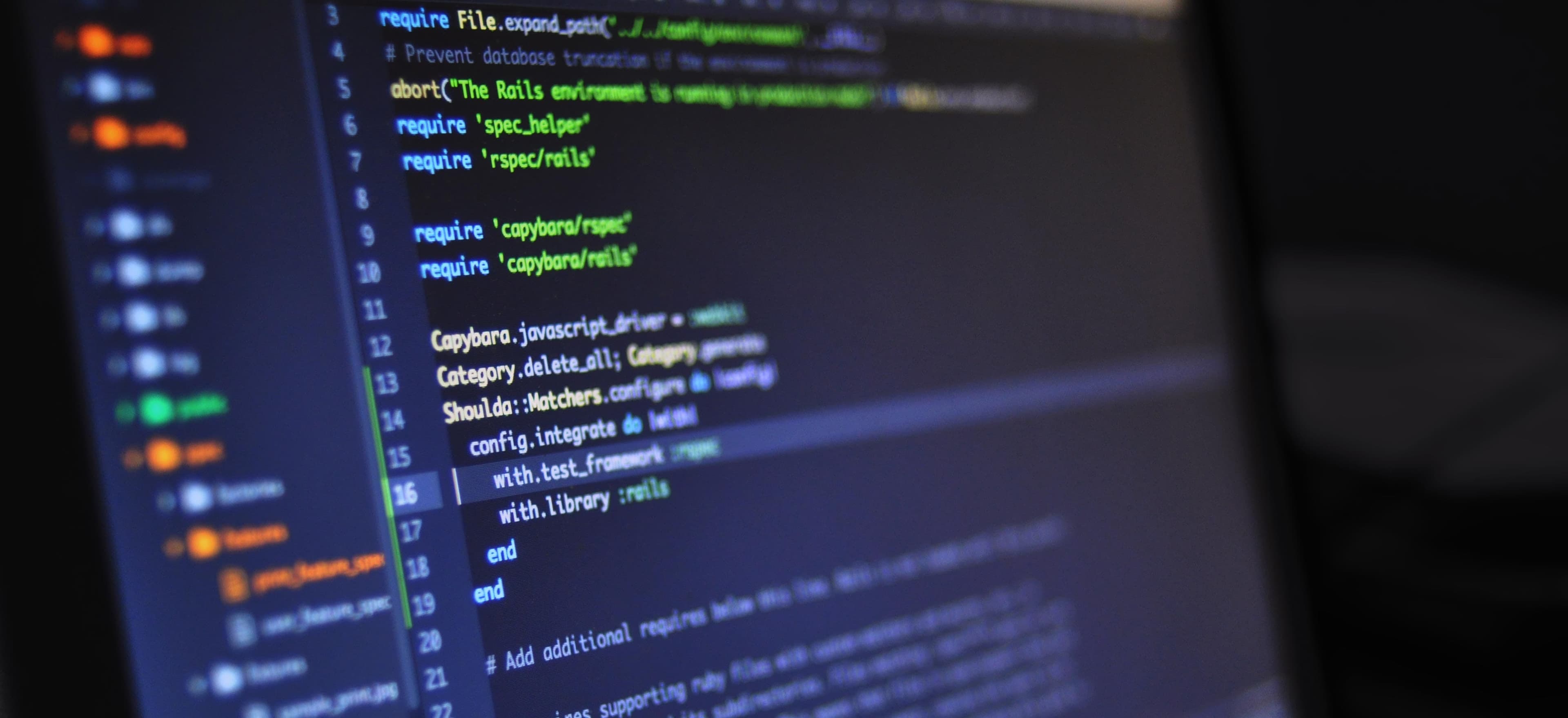
- Published on
Stop Formatting Dates: Embrace SQL Date Literals!
When working with databases, especially SQL-based systems, handling date formats can often be a cumbersome task. The complexity arises primarily due to the different standards, formats, and regional settings involved. Date formats can impact not just queries but also application logic and data integrity. Today, we will delve into the world of SQL date literals, exploring how they can simplify our work and why you should consider embracing them instead of formatting dates.
Understanding SQL Date Literals
SQL date literals are a way to represent dates directly within SQL queries. They ensure consistency and reduce human error when inserting or querying data. By using date literals, you avoid the cumbersome task of converting and formatting dates in your application code.
What Are SQL Date Literals?
A SQL date literal allows you to specify a date value in a straightforward and standardized manner. For example, in SQL standards, the date literal format is typically represented as:
DATE 'YYYY-MM-DD'
This makes your queries easier to read and helps prevent common mistakes associated with date formatting.
Advantages of Using SQL Date Literals
- Clarity and Readability: Using literals improves code readability. It's immediately clear that you're working with a date.
- Consistency: This approach creates a uniform structure, regardless of the regional settings of the environment.
- Reduced Errors: Say goodbye to formatting issues that arise from date string mismatches.
- Performance: SQL databases can often optimize date literals better than text strings or formatted dates.
Example
Consider a table named orders
that includes an order_date
column. Using a date literal simplifies the querying process like so:
SELECT *
FROM orders
WHERE order_date = DATE '2023-10-01';
In contrast, if you were to use a formatted text string, it might look like this:
SELECT *
FROM orders
WHERE order_date = '2023-10-01';
While both may work in many cases, the first option is generally more robust and less prone to error, especially with different date formats across various databases or locales.
Key Points to Note When Using SQL Date Literals
1. Compatibility with SQL Variants
While the date literal syntax is widely supported, be aware of differences across SQL flavors. For instance, Oracle, PostgreSQL, and SQL Server each have slight variations. Always refer to the official documentation for any specifics.
For example, in PostgreSQL, you might also see:
SELECT *
FROM orders
WHERE order_date = '2023-10-01'::DATE;
2. Time Considerations
When dealing with timestamps, it’s essential to be cautious about time zones. If your date includes a time component, be sure to include it in your date literal:
SELECT *
FROM orders
WHERE order_timestamp = TIMESTAMP '2023-10-01 10:00:00';
3. Formatting Functions vs. Date Literals
While it might be tempting to format dates using TO_DATE()
or similar functions, it is often redundant when dealing with SQL date literals.
For example:
SELECT *
FROM orders
WHERE order_date = TO_DATE('2023-10-01', 'YYYY-MM-DD');
This approach is unnecessary when you can leverage literals to convey the same information more straightforwardly.
Incorporating SQL Date Literals in Application Code
When writing applications that interact with a SQL database, you can directly incorporate date literals in your SQL statements. Here is an example using Java with JDBC:
Java Example
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class OrderQuery {
private static final String URL = "jdbc:your_database_url";
private static final String USER = "username";
private static final String PASSWORD = "password";
public void fetchOrders() {
String query = "SELECT * FROM orders WHERE order_date = DATE '2023-10-01'";
try (Connection con = DriverManager.getConnection(URL, USER, PASSWORD);
PreparedStatement pst = con.prepareStatement(query);
ResultSet rs = pst.executeQuery()) {
while (rs.next()) {
System.out.println("Order ID: " + rs.getInt("id") +
", Date: " + rs.getDate("order_date"));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
new OrderQuery().fetchOrders();
}
}
In this code snippet, we directly build our SQL query with a date literal. No additional formatting or transformation is needed, reducing the potential for errors and simplifying code maintenance.
Best Practices
- Consistently Use Date Literals: Make it a habit to use date literals rather than strings or functions for date values.
- Documentation: Document the SQL dialect your application uses, including any deviations from standard SQL.
- Unit Tests: Write unit tests to ensure correct handling of dates, especially if your application deals with various locales or formats.
- Educate Team Members: Make sure your development team understands the benefits of using SQL date literals and how to implement them correctly.
In Conclusion, Here is What Matters: Embrace SQL Date Literals
To streamline working with dates in SQL, embracing date literals is a choice that will serve you well. By opting for clarity, consistency, and reduced error rates, you create a more maintainable and efficient codebase.
For anyone working with databases, understanding the nuances of date handling is essential. SQL date literals simplify this process, enhancing the overall quality of your applications.
Ready to take your SQL skills to the next level? Dive deeper into this topic by reading about SQL Data Types and improve your understanding of how data is handled in databases!
Get started today, and stop formatting dates—embrace SQL date literals!