Common Database Mistakes That Can Ruin Your Project
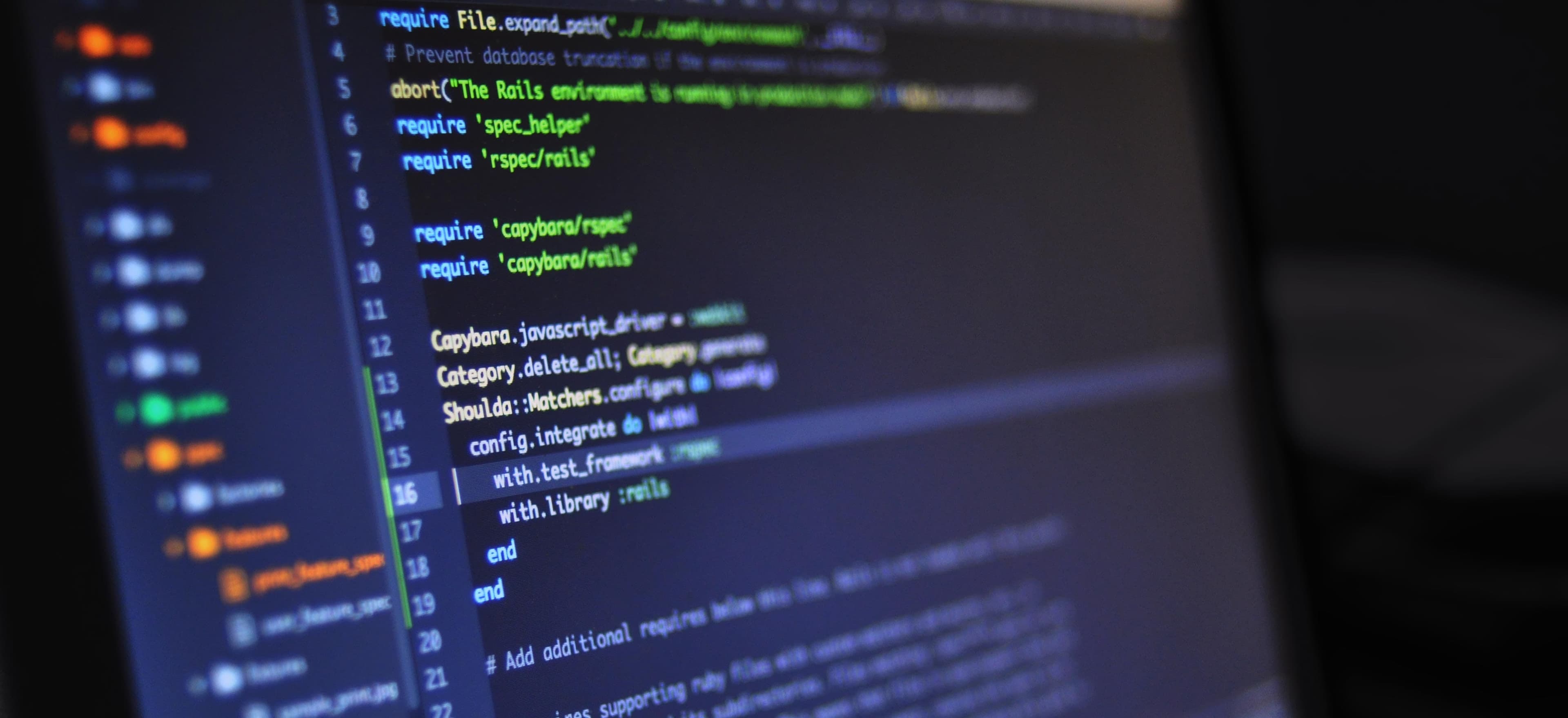
- Published on
Common Database Mistakes That Can Ruin Your Project
In the realm of software development, databases serve as the backbone for data storage and retrieval. However, even the most seasoned developers can fall prey to common pitfalls. This blog post will explore critical database-related mistakes, why they happen, and how they can be avoided to ensure project success.
1. Poor Database Design
Why It Matters
The architecture of your database dictates how efficiently it operates. A poorly designed database can lead to performance issues, data redundancy, and complications during expansion.
Common Mistakes
- Ignoring Normalization: Not normalizing your database can lead to data redundancy and integrity issues.
- Over-Normalization: On the flip side, over-normalizing can make your database complicated and slow.
Solutions
Use standard normalization rules, such as dividing the database into multiple tables while ensuring that every piece of information is represented logically. Here’s a simple example:
-- Normalized Example
CREATE TABLE students (
student_id INT PRIMARY KEY,
student_name VARCHAR(50) NOT NULL
);
CREATE TABLE classes (
class_id INT PRIMARY KEY,
class_name VARCHAR(50) NOT NULL
);
CREATE TABLE enrollments (
student_id INT,
class_id INT,
FOREIGN KEY (student_id) REFERENCES students(student_id),
FOREIGN KEY (class_id) REFERENCES classes(class_id)
);
Why this is effective: By normalizing the data, we can avoid duplication and maintain cardinality.
2. Failing To Use Transactions Properly
Why It Matters
Transactions ensure data integrity. Without them, your database could end up in an inconsistent state.
Common Mistakes
- Not Using Transactions: Relying on single statements instead of wrapping operations in transactions.
Solutions
Wrap database operations that must succeed or fail together in a transaction block:
try (Connection conn = DriverManager.getConnection(DB_URL, USER, PASS)) {
conn.setAutoCommit(false);
// Perform multiple operations
// Pseudo-code
performUpdate(conn);
performInsert(conn);
conn.commit();
} catch (SQLException e) {
conn.rollback();
e.printStackTrace();
}
Why this is effective: Using transactions ensures that either all changes happen or none at all, thus maintaining database integrity.
3. Not Indexing Properly
Why It Matters
Indexes improve the speed of data retrieval operations, which is crucial for performance, especially in large databases.
Common Mistakes
- Over-indexing: Having too many indexes can slow down data insertion, update, and deletion.
- Under-indexing: Failing to create indexes on frequently queried fields.
Solutions
Use indexes judiciously. Here’s an example SQL statement to create an index:
CREATE INDEX idx_student_name ON students(student_name);
Why this is effective: This makes select queries that filter by student_name
much faster, enhancing query performance.
4. Neglecting Security Best Practices
Why It Matters
Database breaches can have catastrophic consequences, exposing sensitive data and potentially putting you out of business.
Common Mistakes
- Hardcoding Credentials: Storing usernames and passwords within your code.
- Empty Default Permissions: Enabling excessive permissions for database users.
Solutions
- Store credentials securely using environment variables or vault services.
- Implement minimum required permissions for users.
String url = System.getenv("DB_URL");
String user = System.getenv("DB_USER");
String password = System.getenv("DB_PASSWORD");
Connection connection = DriverManager.getConnection(url, user, password);
Why this is effective: The code snippet reduces risk exposure by keeping sensitive information out of the source code.
5. Failing to Back Up Data
Why It Matters
Loss of data can happen due to unexpected circumstances like hardware failures or accidental deletions. Without a backup, you may lose significant work.
Common Mistakes
- Irregular Backups: Not having a consistent backup schedule.
- Ignoring Restoration Processes: Never testing backup restoration procedures.
Solutions
Implement regular automated backups with a recovery plan. Here’s a simple example of a cron job for Linux systems:
0 2 * * * mysqldump -u USERNAME -pPASSWORD DATABASE_NAME > /path/to/backup.sql
Why this is effective: Scheduled backups ensure that even in case of failure, you can restore your data without significant downtime or data loss.
6. Not Monitoring Database Performance
Why It Matters
Regularly monitoring your database can help catch performance issues before they escalate into serious problems.
Common Mistakes
- Neglecting Logs: Ignoring query logs and performance statistics.
Solutions
Use tools like New Relic or Datadog to monitor performance metrics such as query execution times, request load, and uptime. Additionally, logging queries can help identify long-running processes.
-- Enable logging in MySQL
SET GLOBAL general_log = 'ON';
Why this is effective: Logging helps you identify slow queries that may need indexing or optimization.
7. Not Handling Scalability
Why It Matters
As your project grows, your database must be able to handle increased load without causing downtime or slow responses.
Common Mistakes
- Centralized Database Architecture: Relying on a single database instance can be limiting.
Solutions
Consider database sharding or replication strategies. This distributes the load, thereby improving reliability and performance. Here’s a simple example of read replication in a MySQL setup:
-- On Master
GRANT REPLICATION SLAVE ON *.* TO 'replica'@'%' IDENTIFIED BY 'password';
-- On Replica
CHANGE MASTER TO
MASTER_HOST='master_host',
MASTER_USER='replica',
MASTER_PASSWORD='password',
MASTER_LOG_FILE='recorded_log_file_name',
MASTER_LOG_POS=recorded_log_position;
START SLAVE;
Why this is effective: Replication allows read operations to be distributed across multiple nodes.
Lessons Learned
Avoiding common database mistakes can significantly enhance your project's functionality and performance. Adopting best practices in database design, security, and performance monitoring helps mitigate risks. Whether you are creating a small application or a large enterprise system, the principles discussed in this article can lead to smoother operations and a more robust architecture.
Further Reading:
- Database Normalization Basics
- Understanding Transactions
- MySQL Monitor
By implementing these strategies, you increase the chances of your project being a success and reduce potential roadblocks in your development process. Happy coding!