Dynamically Changing Kafka Listeners in Spring Boot Applications
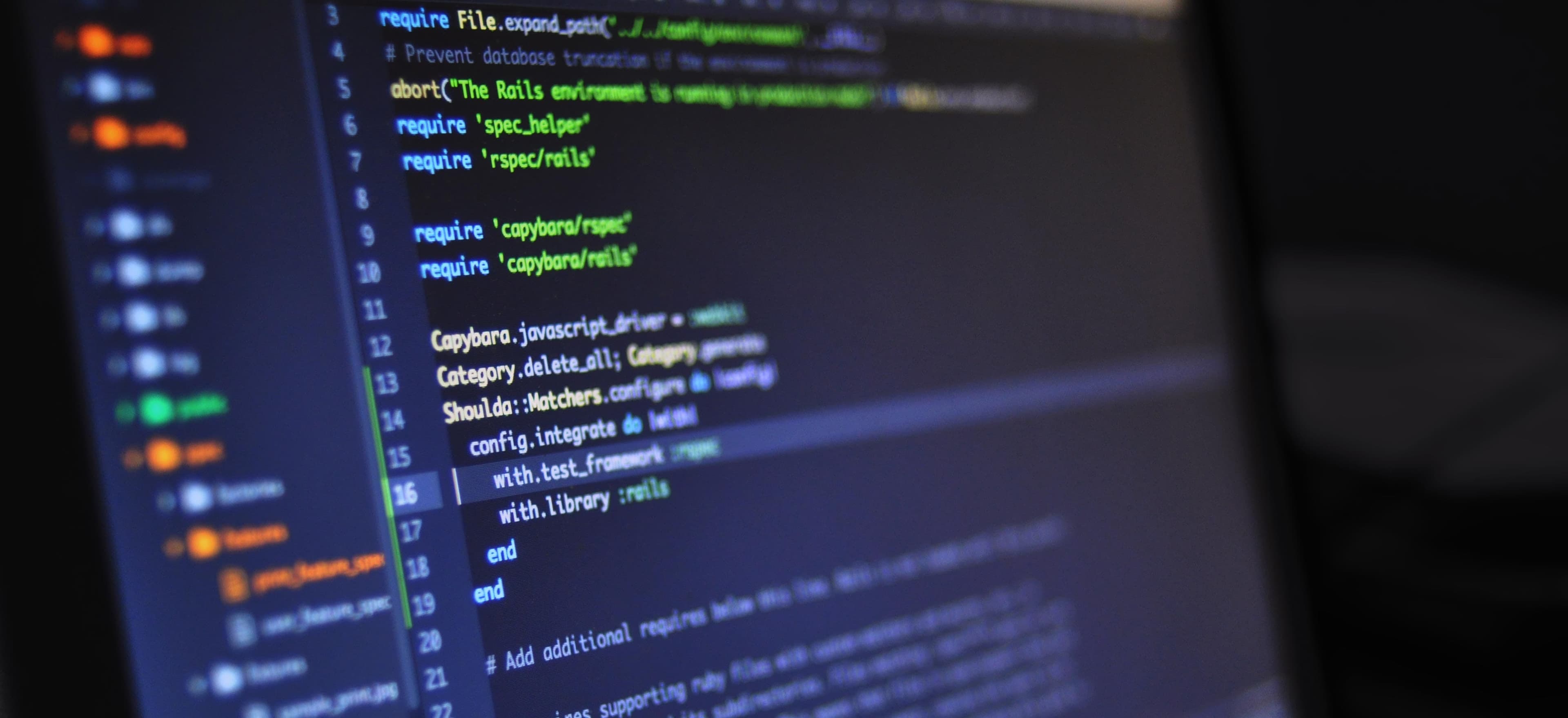
- Published on
Dynamically Changing Kafka Listeners in Spring Boot Applications
Apache Kafka has become a prevalent choice for data streaming and messaging due to its distributed, fault-tolerant architecture. Spring Boot simplifies integrating Kafka into applications, allowing developers to focus on business logic rather than boilerplate code. This blog post will explore how to dynamically change Kafka listeners at runtime in a Spring Boot application.
Why Dynamic Kafka Listeners
In a typical application, Kafka listeners are configured to handle specific topics and messages defined at startup. However, there are valid scenarios where you might need to change these configurations dynamically:
- Multi-Tenant Applications: Different clients may require different configurations based on their needs.
- Real-Time Changes: New topics may be added or modified in response to changing business requirements.
- Resource Allocation: For applications needing to scale, dynamically adding or removing listeners can optimize resource usage.
Prerequisites
Before proceeding, ensure you have:
- A basic understanding of Kafka and how it works.
- A Spring Boot application set up with Kafka dependencies. If you haven't done so, you can get started with the Spring Kafka documentation.
Setting Up a Basic Kafka Listener
First, let's set up a simple Kafka listener. Create a new Spring Boot application with a Kafka dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.kafka</groupId>
<artifactId>spring-kafka</artifactId>
</dependency>
Next, create a Kafka configuration class:
import org.apache.kafka.clients.consumer.ConsumerConfig;
import org.apache.kafka.common.serialization.StringDeserializer;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.kafka.annotation.EnableKafka;
import org.springframework.kafka.config.ConcurrentKafkaListenerContainerFactory;
import org.springframework.kafka.core.ConsumerFactory;
import org.springframework.kafka.core.DefaultKafkaConsumerFactory;
import java.util.HashMap;
import java.util.Map;
@Configuration
@EnableKafka
public class KafkaConfig {
@Value("${kafka.bootstrap-servers}")
private String bootstrapServers;
@Bean
public ConsumerFactory<String, String> consumerFactory() {
Map<String, Object> props = new HashMap<>();
props.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, bootstrapServers);
props.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class);
props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class);
return new DefaultKafkaConsumerFactory<>(props);
}
@Bean
public ConcurrentKafkaListenerContainerFactory<String, String> kafkaListenerContainerFactory() {
ConcurrentKafkaListenerContainerFactory<String, String> factory = new ConcurrentKafkaListenerContainerFactory<>();
factory.setConsumerFactory(consumerFactory());
return factory;
}
}
This configuration sets up the basics for consuming messages from Kafka.
A Simple Kafka Listener
Here's a basic listener that waits for messages on a specific topic called "my-topic":
import org.springframework.kafka.annotation.KafkaListener;
import org.springframework.stereotype.Service;
@Service
public class MessageListener {
@KafkaListener(topics = "my-topic", groupId = "group_id")
public void listen(String message) {
System.out.println("Received Message: " + message);
}
}
Dynamically Changing Listeners
To dynamically change Kafka listeners, you can employ Spring's KafkaListenerEndpointRegistry
. This registry allows you to manage listener containers at runtime easily:
Step 1: Autowire KafkaListenerEndpointRegistry
First, inject the KafkaListenerEndpointRegistry
in your service or controller.
import org.springframework.kafka.listener.KafkaListenerEndpointRegistry;
import org.springframework.stereotype.Service;
import javax.annotation.PostConstruct;
@Service
public class ListenerService {
private final KafkaListenerEndpointRegistry endpointRegistry;
public ListenerService(KafkaListenerEndpointRegistry endpointRegistry) {
this.endpointRegistry = endpointRegistry;
}
@PostConstruct
public void init() {
// You can start/stop existing listeners or adjust settings here
}
// Other methods to dynamically manage listeners
}
Step 2: Managing Listeners
You can create methods within your service to start, stop, or modify listeners dynamically. Below are examples of how to stop and start listeners:
public void stopListener(String listenerId) {
endpointRegistry.getListenerContainer(listenerId).stop();
System.out.println("Stopped listener: " + listenerId);
}
public void startListener(String listenerId) {
endpointRegistry.getListenerContainer(listenerId).start();
System.out.println("Started listener: " + listenerId);
}
Dynamic Topic Subscription
To change the topics a listener subscribes to at runtime, you will need to create new listener containers or update existing ones. Here is an example that shows how to add (subscribe) a new topic to an existing listener programmatically:
public void changeTopic(String listenerId, String newTopic) {
var container = endpointRegistry.getListenerContainer(listenerId);
if (container != null) {
// Stop the existing listener
container.stop();
// Modify the Container properties to change the topic
container.getContainerProperties().setTopic(newTopic);
// Start the listener back up
container.start();
System.out.println("Listener " + listenerId + " changed to topic: " + newTopic);
}
}
Make sure to adjust the container properties properly according to the changes in your application logic.
Best Practices
-
Error Handling: Implement proper error handling when changing listener configurations. This ensures that your application remains robust even when changes go awry.
-
Testing: Test dynamically changing listeners in a staging environment before deploying to production. This helps catch issues that may arise during runtime.
-
Monitoring & Metrics: Integrate monitoring tools to observe the performance and behavior of dynamically managed listeners. Spring Actuator can give insights into listener health.
Final Thoughts
Dynamically changing Kafka listeners in a Spring Boot application offers extensive flexibility. This allows you to adapt to various runtime requirements, whether adjusting for multi-tenant scenarios, responding to business changes, or optimizing resource allocation.
With the demonstrated methods in this post, you can easily create an adaptive Kafka listener setup. You can find further reading materials on implementing Kafka listeners and other Spring topics at the official Spring Kafka documentation or explore various Kafka configurations through the Apache Kafka documentation.
In summary, leveraging Spring's capabilities for managing Kafka listeners allows you to build highly flexible and robust applications capable of accommodating dynamic requirements without significant overhead.
Feel free to reach out if you have any questions or need further clarifications! Happy coding!