Overcoming Challenges in Bitwise String Property Conversion
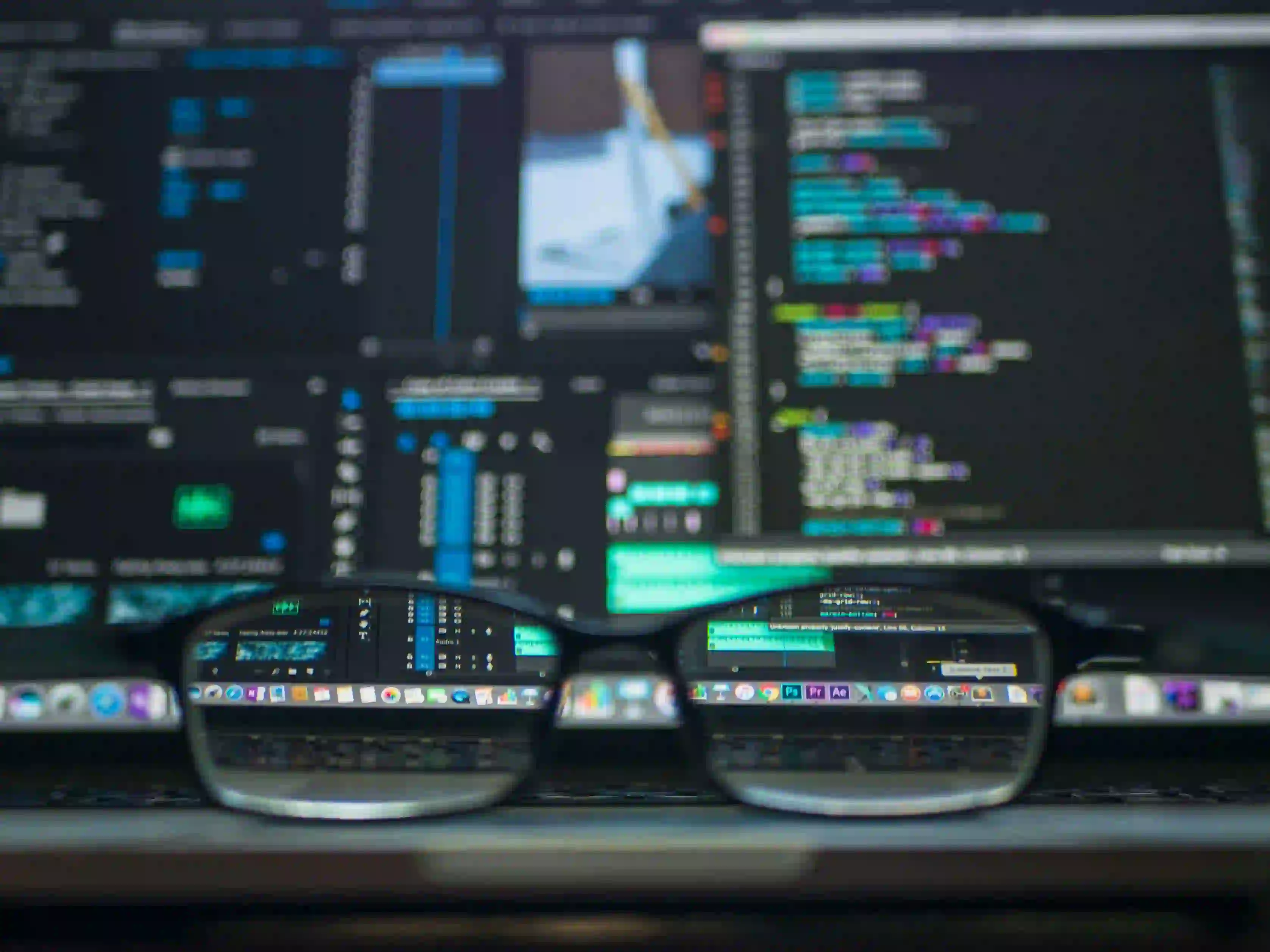
Overcoming Challenges in Bitwise String Property Conversion
Bitwise operations are a vital aspect of computer science and programming. In Java, developers often face challenges when converting string properties into their bitwise equivalents. This blog post will delve into these challenges and offer solutions, including code snippets to illustrate key concepts while ensuring the content remains engaging and informative.
Understanding Bitwise Operations
Before we dive into the challenges, let's clarify what bitwise operations are. Bitwise operations allow manipulation of individual bits of data types using operators such as AND, OR, NOT, XOR, and bit shifts. These operations can significantly enhance performance and memory optimization, particularly when working with flags, masks, and binary representations.
Bitwise Operators in Java
Java supports several bitwise operators:
- AND (
&
) - OR (
|
) - XOR (
^
) - NOT (
~
) - Left Shift (
<<
) - Right Shift (
>>
and>>>
)
Understanding these will provide a solid foundation for addressing string property conversions.
Challenges in String Property Conversion
When converting strings to their bitwise equivalents, developers encounter various challenges:
- Data Types Handling: The string may represent various data types (integers, booleans, etc.), making direct conversion non-trivial.
- String Complexity: Strings can incorporate complex formats, including leading zeros, whitespace, or special characters.
- Error Management: Conversion failures can lead to runtime exceptions or incorrect values if not appropriately managed.
The Solution: Step-by-Step Conversion Process
To overcome these challenges, we can follow a structured approach to convert string properties bitwise. Here’s an outline of the process:
- Identify Data Type: Determine the expected data type based on the string's content.
- Sanitize Input: Clean the string for processing by removing unwanted characters.
- Perform Conversion: Employ bitwise operations to map the string to its numeric value.
- Error Handling: Implement try-catch blocks to manage unforeseen exceptions gracefully.
Example Code Snippet: String to Integer Conversion
Let’s start with a simple example where we will convert a string that represents a binary number into an integer.
public class BitwiseStringConversion {
public static void main(String[] args) {
String binaryString = "101010"; // A binary number as a string
try {
// Clean string - remove any whitespace
binaryString = binaryString.trim();
// Perform Conversion
int decimalValue = Integer.parseInt(binaryString, 2); // base 2 for binary
System.out.println("Binary: " + binaryString + " -> Decimal: " + decimalValue);
} catch (NumberFormatException e) {
System.err.println("Invalid binary string: " + e.getMessage());
}
}
}
Commentary on the Code Snippet
In this example:
- We start with a binary string
101010
. - We trim white spaces to prevent format issues.
- The
Integer.parseInt()
method converts the binary string to its decimal equivalent, specifying base 2. - Wrapping the conversion in a try-catch cloud ensures that we gracefully handle invalid formats.
Handling Boolean Strings
Now, let's tackle converting string representations of booleans into bitwise operations. Consider a situation where we receive "true" or "false" as a string.
public class BooleanBitwiseConversion {
public static void main(String[] args) {
String booleanString = "true"; // It could also be "false"
boolean bitwiseValue = Boolean.parseBoolean(booleanString);
// Demonstrating usage in bitwise operation
int number = 5; // 0101 in binary
// OR operation
int result = number | (bitwiseValue ? 1 : 0); // Convert boolean to 0 or 1
System.out.println("Original Number: " + number);
System.out.println("Boolean Value: " + bitwiseValue);
System.out.println("Result after OR operation: " + result);
}
}
Key Takeaways
- The
Boolean.parseBoolean(string)
method converts string representations to boolean values seamlessly. - In this code, we demonstrate how to treat a boolean as a bit during a bitwise OR operation.
Converting Complex Strings
Sometimes string properties can be more complex. For example, suppose we have a hex string, and we need to convert it to a binary representation.
public class HexToBinaryConversion {
public static void main(String[] args) {
String hexString = "1A3"; // Example Hexadecimal String
try {
// Perform conversion to decimal first
int decimalValue = Integer.parseInt(hexString, 16);
String binaryValue = Integer.toBinaryString(decimalValue); // Convert decimal to binary
System.out.println("Hex: " + hexString + " -> Decimal: " + decimalValue + " -> Binary: " + binaryValue);
} catch (NumberFormatException e) {
System.err.println("Invalid hex string: " + e.getMessage());
}
}
}
Analysis of the Example
In this case:
- We process a hexadecimal string input.
- The provided hex string is first converted into decimal using
Integer.parseInt()
with a base of 16. - Finally, we use
Integer.toBinaryString()
to get the binary representation.
Error Handling and Validation
One of the significant challenges during string property conversion is managing invalid inputs. In all previous examples, we have wrapped conversion processes within try-catch blocks. This approach is essential to handle exceptions effectively.
However, additional validation can improve user experience:
- Input Validation: Before conversion, check if the string matches expected formats (e.g., regex for binary or hex).
- Logging Errors: Implement logging for tracking errors and boosting maintainability.
- User Feedback: Inform the user of input issues, guiding them on correct formats.
Key Takeaways
The art of converting string properties to their bitwise counterparts in Java may be fraught with challenges. Nevertheless, by employing structured methodologies and robust error handling, developers can navigate these challenges effectively.
As you've learned from this blog post, understanding the nuances of string manipulation and bitwise operations is key. By following the steps outlined here, you can confidently tackle similar conversion tasks in your projects.
For more information on bitwise operations and Java programming, consider visiting Oracle's Java Documentation, or explore additional resources on Java Bitwise Operators. Keep experimenting and happy coding!