Why Can't Non-Static Methods Access Static Variables in Java?
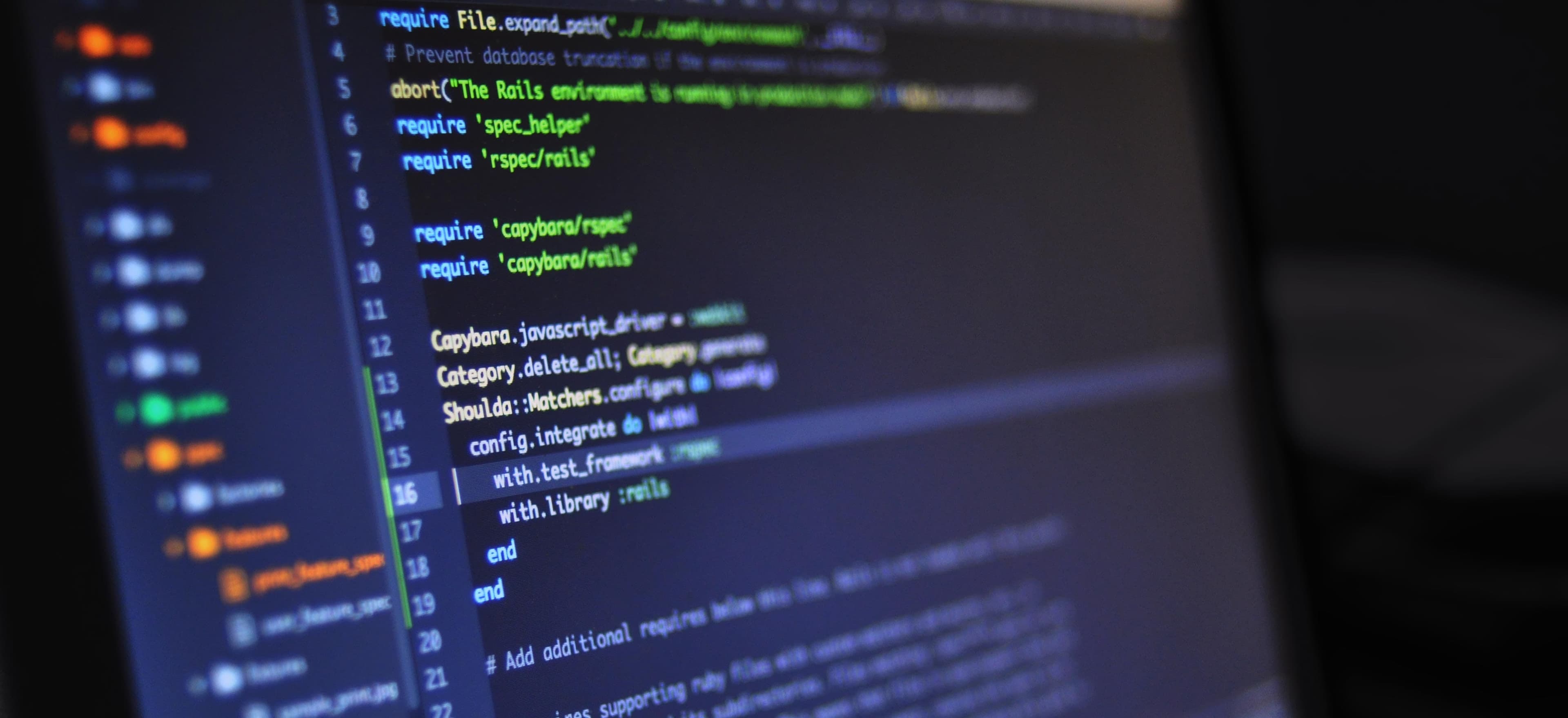
- Published on
Why Can't Non-Static Methods Access Static Variables in Java?
When diving into the intricacies of Java programming, one cannot overlook the nuances between static and non-static entities. Although this distinction might appear trivial at first glance, it is fundamental to understanding how Java handles memory allocation, object behaviors, and application design. One of the common queries among Java newcomers is: Why can't non-static methods access static variables in Java? This blog post aims to dissect this query, shedding light on static and non-static concepts while providing relevant examples.
Understanding the Basics
To clarify the relationship between static and non-static contexts, we first need to define what these terms involve.
Static Variables
A static variable is associated with the class itself rather than with any specific instance. This means that all instances of the class share this variable. Static variables are created when the class is loaded and destroyed when the class is unloaded:
public class MyClass {
public static int staticVariable = 0; // Static variable
}
Non-Static Methods
A non-static method, also known as an instance method, operates on the instance of the class and has access to instance variables:
public class MyClass {
public int instanceVariable;
public void nonStaticMethod() {
System.out.println("Instance Variable: " + instanceVariable);
}
}
Interaction between Static and Non-Static
Let's consider what happens when we try to access a static variable within a non-static method in Java.
Example Code
public class Demo {
public static int staticVar = 10; // Static variable
public void nonStaticMethod() {
// This will work
System.out.println("Static Variable: " + staticVar);
}
public static void main(String[] args) {
Demo demo = new Demo();
demo.nonStaticMethod();
}
}
Explanation
In the above example, we see a non-static method (nonStaticMethod
) accessing a static variable (staticVar
). This works perfectly fine and prints the expected output, which shows that non-static methods can access static variables.
Now, let’s flip the scenario to see when the confusion arises.
Misconception Clarified
The misconception often stems from the phrasing, "non-static methods cannot access static variables.” In reality, it's not that non-static methods cannot access static variables, but rather that they do not need to reference them in a way that suggests an instance context.
When a non-static method accesses a static variable, it is done directly through the variable name, as shown above. However, if a static method were to try to reference a non-static variable, that would lead to issues.
Exploring Scope and Memory
Understanding how Java manages memory sheds additional light on this topic.
-
Static Context: The static context is shared across all instances of the class. There is only one copy of static variables per class, irrespective of how many objects are created.
-
Instance Context: The instance context is unique to each object. Each object has its own copy of instance variables.
This means a static method cannot reference instance members or methods because they belong to specific instances, not to the class as a whole.
Example Code: Static vs. Non-Static Access
public class Example {
public int instanceVar = 5; // Instance variable
public static int staticVar = 10; // Static variable
public void instanceMethod() {
System.out.println("Accessing Instance Variable: " + instanceVar);
System.out.println("Accessing Static Variable: " + staticVar);
}
public static void staticMethod() {
// System.out.println("Accessing Instance Variable: " + instanceVar); // This line will cause an error
System.out.println("Accessing Static Variable: " + staticVar);
}
}
Output
If you invoke the methods like this:
public class Main {
public static void main(String[] args) {
Example obj = new Example();
// Call instance method
obj.instanceMethod(); // This works
// Call static method
Example.staticMethod(); // This works
}
}
In this case, the instance method smoothly accesses both the instance and static variables, while the static method can only access the static variable. Attempting to access instanceVar
within staticMethod
will result in a compilation error.
Highlights of the Concept
- Static methods can only directly access static variables.
- Instance methods can access both instance and static variables.
- Static variables exist at the class level, while instance variables pertain to object instances.
Closing Remarks
In summary, the core of the question lies in understanding the nature of static and non-static contexts:
- Non-static methods can access static variables without complication.
- The reverse is where confusion arises; static methods cannot access instance variables since they are tied to specific object instances, which don't exist in the static context.
Understanding these concepts is crucial to designing effective applications in Java. By navigating these principles, developers can leverage the robust capabilities of Java to create efficient, organized, and maintainable code.
Further Reading
These resources provide additional reading and examples to deepen your understanding of Java programming concepts. Happy coding!
Checkout our other articles