Overcoming Slow PageRank Calculations in Hadoop
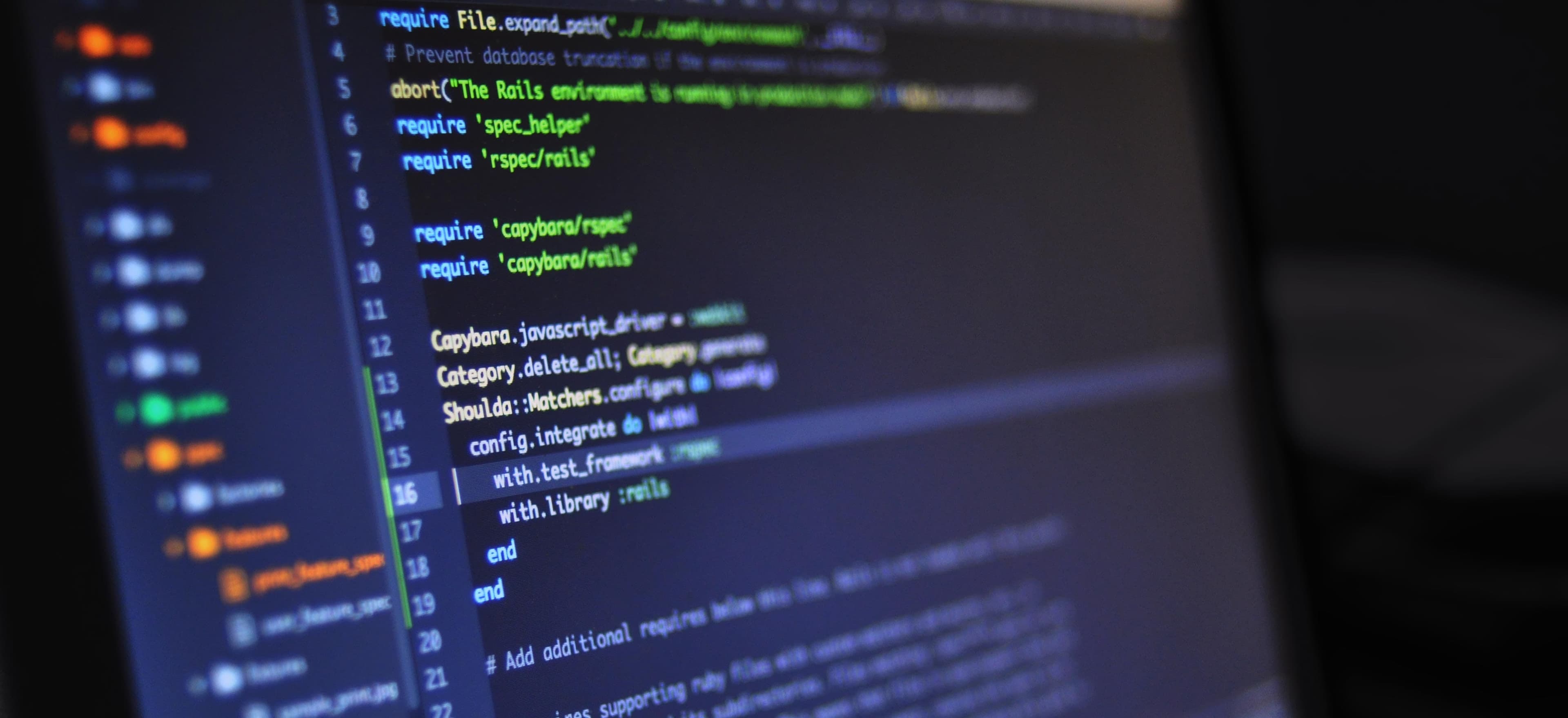
- Published on
Overcoming Slow PageRank Calculations in Hadoop
The PageRank algorithm, developed by Google founders Larry Page and Sergey Brin, is an essential tool for ranking web pages in search results. It uses a system of link analysis to assign a numerical weight to each web page, indicating its relative importance. Implementing this algorithm efficiently in a distributed environment like Hadoop can present challenges, particularly in terms of performance. This post will delve into the intricacies of optimizing PageRank calculations in Hadoop, helping you navigate and overcome the slow processing issues associated with large datasets.
Understanding PageRank and Hadoop
Before diving into optimizations, it is vital to understand how PageRank works and how it integrates with Hadoop.
How PageRank Works
PageRank operates on the principle that important pages are likely to link to other important pages. The basic formula can be summarized as follows:
PR(A) = (1 - d) + d * (PR(T1)/C(T1) + PR(T2)/C(T2) + ... + PR(Tn)/C(Tn))
Where:
- PR(A) is the PageRank of page A.
- d is the damping factor (usually set around 0.85).
- T1, T2, ..., Tn are pages that link to page A.
- C(Ti) is the number of outbound links on page Ti.
Hadoop's Role in PageRank Calculations
Hadoop is a powerful framework designed for distributed storage and processing of large data sets. It uses the MapReduce paradigm to break tasks into subtasks that can be executed in parallel across multiple nodes. This feature makes it ideal for running complex algorithms like PageRank, especially when dealing with large-scale web graphs.
However, calculating PageRank can become slow when processing vast amounts of data. This post aims to tackle these performance issues and improve the overall efficiency of your PageRank computations in Hadoop.
Challenges in PageRank Calculations with Hadoop
- Data Size: PageRank requires processing large datasets, particularly when dealing with extensive links from numerous web pages.
- Communication Overhead: Each iteration of PageRank involves significant data transfer among nodes, leading to increased latency.
- Convergence Requirements: Reaching convergence in PageRank iterations often requires a substantial number of passes over the data.
- Inefficient Algorithm Implementation: A naive implementation can lead to bottlenecks in data processing.
Optimizing PageRank Calculations in Hadoop
To overcome the challenges associated with slow PageRank calculations in Hadoop, consider the following strategies:
1. Efficient Data Representation
Using Compressed Data Formats
Use compressed data formats such as Avro, Parquet, or ORC. These formats can significantly reduce data size, leading to faster processing times.
// Example of setting up Parquet in a Hadoop job
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "PageRank");
job.setOutputFormatClass(ParquetOutputFormat.class);
ParquetOutputFormat.setOutputPath(job, new Path(outputDir));
Why: Compressed data formats reduce I/O operations and storage requirements, enabling faster reading and writing processes during MapReduce tasks.
2. Optimize the MapReduce Code
Bursting the Iteration Bottleneck
You can adjust the number of iterations based on data convergence. Setting an adaptive threshold for stopping criteria can reduce unnecessary iterations.
if (Math.abs(prValue - oldPrValue) < convergenceThreshold) {
context.getCounter("Custom", "Converged").increment(1);
return;
}
Why: By checking for convergence before proceeding to the next iteration, you minimize the overall number of iterations, which can lead to significant time savings.
3. Use Efficient Algorithms
Implementing the GraphChi or Pregel Approach
Consider using Pregel-like abstractions or specialized libraries designed for graph algorithms, such as GraphX (for Spark) or GraphChi (for disk-based processing). These frameworks can perform better than traditional MapReduce models in certain contexts.
// Pseudo-code representation of a Pregel-like iteration
pregel.run(graph, iterations, new MessageCombiner());
Why: Algorithms optimized for graph processing can exploit data locality and minimize communication overhead, resulting in faster computations.
4. Reduce Data Transfer
Local Aggregation
Aggregate results locally in the Mapper before sending data to the Reducers. This reduces the volume of data transmitted between nodes.
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// Local aggregation of PageRank contributions
String[] parts = value.toString().split("\t");
double prContribution = calculateContribution(parts);
localSum += prContribution;
}
Why: Minimizing the data sent over the network reduces latency and can significantly improve job execution time.
5. Utilize Caching and Memory Management
Make Use of Distributed Cache
Keep frequently accessed data in memory using the Hadoop distributed cache. This minimizes the need to repeatedly read from disk.
DistributedCache.addCacheFile(new URI("cacheFile.txt#cache"), conf);
Why: Accessing data from memory is an order of magnitude faster than reading from disk, directly speeding up computations.
6. Tuning Hadoop Settings
Adjusting the Configuration Parameters
Optimize Hadoop configuration settings to ensure that memory and computational resources are appropriately allocated.
<property>
<name>mapreduce.map.memory.mb</name>
<value>2048</value>
</property>
<property>
<name>mapreduce.reduce.memory.mb</name>
<value>2048</value>
</property>
Why: Proper tuning of resource allocations based on the specific job requirements can prevent resource contention and speed up processing.
7. Evaluate Alternate Tools
Consider Apache Spark
If your use case allows, consider switching to Apache Spark for running PageRank. Spark can leverage in-memory computing and optimized transformations that can drastically reduce computation time for iterative algorithms like PageRank.
val graph = GraphLoader.edgeListFile(sc, "edges.txt")
val ranks = graph.pageRank(0.0001).vertices
Why: Spark’s resilient distributed datasets (RDDs) help minimize data movement and offer high performance for iterative computations.
The Last Word
Improving the performance of PageRank calculations in Hadoop is not just about code optimization; it's also about data management, resource allocation, and utilizing the right tools. By addressing the challenges mentioned and implementing the proposed solutions, you can effectively overcome the slow performance issues associated with PageRank calculations on large datasets.
Efficiently calculating PageRank enables better search results and richer web experiences. For more comprehensive resources on PageRank and web algorithms, consider checking out the following:
- Understanding PageRank
- Hadoop Tutorial
- Apache Spark Official Documentation
With these strategies, you can ensure that your PageRank implementation in Hadoop is optimized for speed, providing timely results and better performance overall. Happy coding!
Checkout our other articles