Mastering Android Launch Activities: Common Pitfalls to Avoid
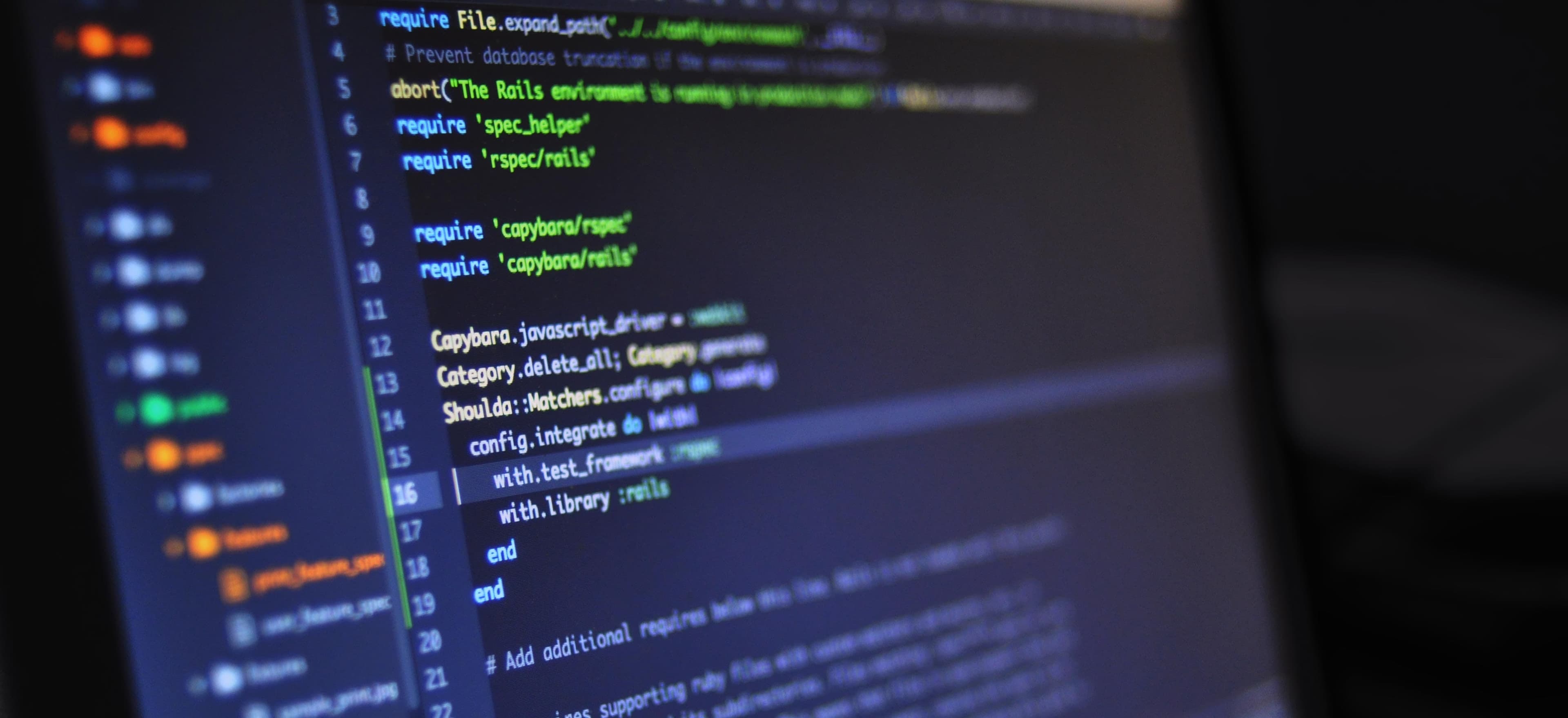
- Published on
Mastering Android Launch Activities: Common Pitfalls to Avoid
As Android developers, one of the fundamental building blocks we must understand is the activity lifecycle, particularly how launch activities function within it. Activities are the entry points for users to interact with your app, so mastering their launch is crucial. In this blog post, we will explore common pitfalls associated with launch activities and provide practical insights into avoiding them. Whether you're a beginner or an experienced developer, this guide will help you enhance your understanding and create smoother applications.
Understanding Activities in Android
In Android, an activity represents a single screen with a user interface. It’s the interface through which users interact with your app. When users launch your app, they are usually greeted by a launch activity—often referred to as the main activity. Understanding the activity lifecycle is key to creating responsive and efficient apps.
The main lifecycle methods include:
- onCreate(): Initializes the activity.
- onStart(): Makes the activity visible.
- onResume(): Brings the activity to the foreground.
- onPause(): The activity loses focus.
- onStop(): The activity is no longer visible.
- onDestroy(): Cleaning up resources before the activity is destroyed.
Common Pitfalls with Launch Activities
1. Incorrect Intent Handling
When launching activities, it's important to handle intents correctly. A common issue arises when developers unintentionally create new instances of an activity instead of using an existing one.
For example, consider this code snippet:
Intent intent = new Intent(this, HomeActivity.class);
startActivity(intent);
If HomeActivity
is already running and you want to navigate back to it, this code will create a new instance instead of using the already existing one.
The Solution
You can manage activity instances effectively by utilizing flags in your intent. Use FLAG_ACTIVITY_SINGLE_TOP
to ensure the existing instance of HomeActivity
is brought to the foreground instead of creating a new one.
Intent intent = new Intent(this, HomeActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP);
startActivity(intent);
2. Misconfiguring the AndroidManifest.xml
The AndroidManifest.xml
file defines how activities behave. A common pitfall is misconfiguration of task affinity and launch modes, leading to unexpected behavior.
For instance, if you want HomeActivity
to be a single instance across your application, you can set its launch mode:
<activity
android:name=".HomeActivity"
android:launchMode="singleTask">
</activity>
Why This Matters
Using the correct launch mode can ensure that users don't accidentally create multiple instances of the same activity, providing a smoother experience.
3. Not Handling Configuration Changes
Android devices come in various shapes and sizes, and one common pitfall is not appropriately handling configuration changes, such as screen rotation or changes in language.
When a configuration change occurs, the default behavior is to destroy and recreate the activity. If you haven't saved instance state, you'll lose critical data.
Optimizing for Configuration Changes
To retain data, you can override onSaveInstanceState()
to save important information:
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("myKey", myValue); // Store the value
}
And restore it in onCreate()
or onRestoreInstanceState()
:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (savedInstanceState != null) {
myValue = savedInstanceState.getString("myKey");
}
}
4. Ignoring Back Stack Management
The back stack plays a significant role in how users navigate an app. Ignoring back stack management can lead to a cluttered navigation experience.
When you launch a new activity, it gets pushed onto the stack. However, you may need to pop an activity off the stack in certain scenarios, such as when a user completes a task and you want to return them to the previous screen.
Implementing Efficient Navigation
To handle this scenario appropriately, consider calling finish()
on the current activity before starting a new one:
Intent intent = new Intent(CurrentActivity.this, NextActivity.class);
startActivity(intent);
finish(); // This ensures we return to the previous activity
5. Not Using Fragments When Appropriate
Fragments offer a more flexible way to manage the UI and lifecycle than activities alone. Using fragments can reduce the complexity of your navigation and help manage screen orientation changes.
If your launch activity has a significant amount of UI that can be modularized, consider using fragments to encapsulate those functionalities.
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.replace(R.id.fragment_container, new MyFragment());
fragmentTransaction.commit();
6. Overcomplicating the Launch Sequence
Sometimes, developers overcomplicate the launch sequence by chaining multiple activities together. This can confuse users and make it difficult to navigate back.
A Simpler Approach
Instead, consider using a single launch activity that can dynamically load fragments based on user actions. This organized approach will lead to better maintainability and a smoother user experience.
7. Neglecting User Experience During Launch
Finally, it’s essential to consider the user experience during the launch of your app. Long loading times or unresponsive interactions can frustrate users and may lead them to abandon your app.
Optimizing for Launch Performance
To improve launch times, consider:
- Optimizing your layouts by using
ConstraintLayout
. - Avoiding heavy computations in
onCreate()
. - Deferring non-critical initializations to later in the app lifecycle.
For more tips on improving performance in Android, refer to Google's Developer Guides.
The Bottom Line
Mastering Android launch activities requires a good grasp of intents, activity lifecycle management, and user experience considerations. By avoiding common pitfalls such as incorrect intent handling, misconfigured manifest files, and setup complexities, you can create a more seamless app experience. Always remember to consider how your activity interacts with the back stack and use fragments when appropriate for modularization.
With these practices in mind, you'll be well on your way to becoming a proficient Android developer. If you have further questions or want to share your experiences, feel free to leave a comment below!
Further Reading
For those eager to deepen their knowledge, consider exploring the following resources:
- Android Official Documentation - Activities and Intents
- Google Codelabs for Android Development
This information not only prepares you to handle launch activities effectively but also enhances your overall development skills. Happy coding!
Checkout our other articles