Avoiding Common Pitfalls in Java Code Generation
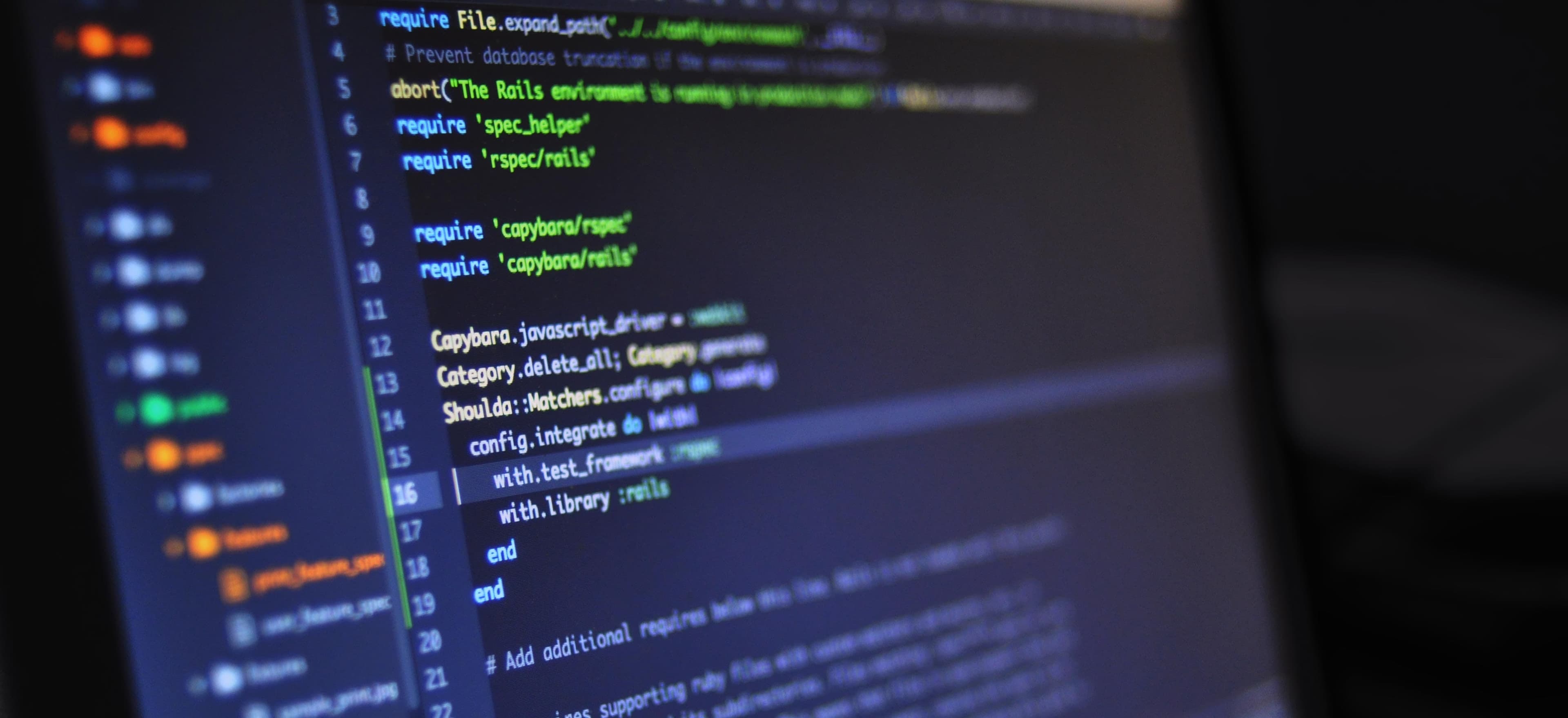
- Published on
Avoiding Common Pitfalls in Java Code Generation
Code generation is a vital aspect of modern software development, especially in Java. It allows developers to automate the creation of repetitive code patterns, leading to increased productivity and fewer errors. However, the process isn't without its pitfalls. In this blog post, we will explore common mistakes in Java code generation and provide solutions to avoid them.
What is Code Generation in Java?
Code generation in Java refers to the automated creation of Java source files based on predefined templates and parameters. This can involve anything from generating boilerplate code, like getters and setters, to creating complex data models or even entire applications based on user inputs or database schemas.
While code generation can significantly reduce the workload on developers, it can lead to issues if not handled correctly. Let’s take a look at some common pitfalls and how to avoid them.
Common Pitfalls in Java Code Generation
1. Over-Generating Code
It's easy to fall into the trap of generating too much code. You might start with a clear intent but end up with a bulky class filled with unnecessary methods or attributes.
Solution: Always apply the Single Responsibility Principle. Each class should have one reason to change. Keep your generated code as lightweight as possible.
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Only add getters and setters as needed
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
In this example, the User
class is simple and focuses solely on user data. By minimizing unnecessary code, you're making it easier to maintain.
2. Lack of Customization Options
Mass-produced code generators often fail to meet specific project needs, resulting in frustration for developers who have to make numerous modifications.
Solution: Provide customizable templates.
By using template engines like FreeMarker or Mustache, you can create dynamic templates that can be filled with specific context data.
<#-- Example FreeMarker Template -->
public class ${className} {
<#list fields as field>
private ${field.type} ${field.name};
</#list>
<#list fields as field>
public ${field.type} get${field.name?cap_first}() {
return ${field.name};
}
public void set${field.name?cap_first}(${field.type} ${field.name}) {
this.${field.name} = ${field.name};
}
</#list>
}
This template allows developers to define their own classes with just a few variables, reducing effort and maintaining specific needs.
3. Overlooking Exception Handling
Manual coding often includes responsibility for exception handling, but generated code may skip this vital aspect. Ignoring exceptions can lead to poor user experiences.
Solution: Always implement standard exception handling in your templates.
As a simple illustration, consider adding a try-catch block to your data fetching code:
public User getUserById(int userId) {
try {
// Assume findUserById method retrieves user from database
return findUserById(userId);
} catch (SQLException e) {
// Log the error and rethrow a custom exception
System.err.println("Failed to fetch user: " + e.getMessage());
throw new CustomDatabaseException("User retrieval failed", e);
}
}
4. Ignoring Code Quality
Automatically generated code can occasionally lack the polish expected from hand-written code. This includes poor formatting, inconsistent naming conventions, and the absence of JavaDoc comments.
Solution: Utilize tools such as Checkstyle or PMD to enforce coding standards on your generated codebase.
Ensure your templates include appropriately formatted sections and documentation comments:
/**
* Represents a user in the application.
*/
public class User {
// User attributes and methods...
}
5. Not Including Unit Tests
Failing to create unit tests alongside generated code can lead to serious issues down the line. Even the best generation tools can't predict all edge cases.
Solution: Integrate test generation with the code generation process.
For example, consider generating basic unit tests alongside your class:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class UserTest {
@Test
public void testUserCreation() {
User user = new User("John", "john@example.com");
assertEquals("John", user.getName());
assertEquals("john@example.com", user.getEmail());
}
}
6. Forgetting to Keep Updated with Best Practices
Java evolves continually, and best practices can change. Over time, code generators that rely on outdated methods can create suboptimal or deprecated code.
Solution: Regularly review and update your code generation tools to accommodate the latest Java standards and libraries. This is especially important for tech stacks that depend on frameworks like Spring Boot or Hibernate.
To Wrap Things Up
Code generation can greatly enhance the efficiency and productivity of Java developers. However, as we've seen, it's essential to remain vigilant against common pitfalls. By following these best practices—such as customizing templates, implementing robust exception handling, and enforcing code quality—you can ensure that your generated code is not only functional but also maintainable.
For more insights on efficient Java coding practices, refer to Java Code Geeks. Additionally, consider exploring Oracle's Java Documentation for up-to-date information on Java standards.
By staying informed and applying these principles diligently, you can unlock the full potential of Java code generation while avoiding its common traps. Happy coding!
Checkout our other articles