Mastering Java: Compile and Run Without an IDE in Minutes
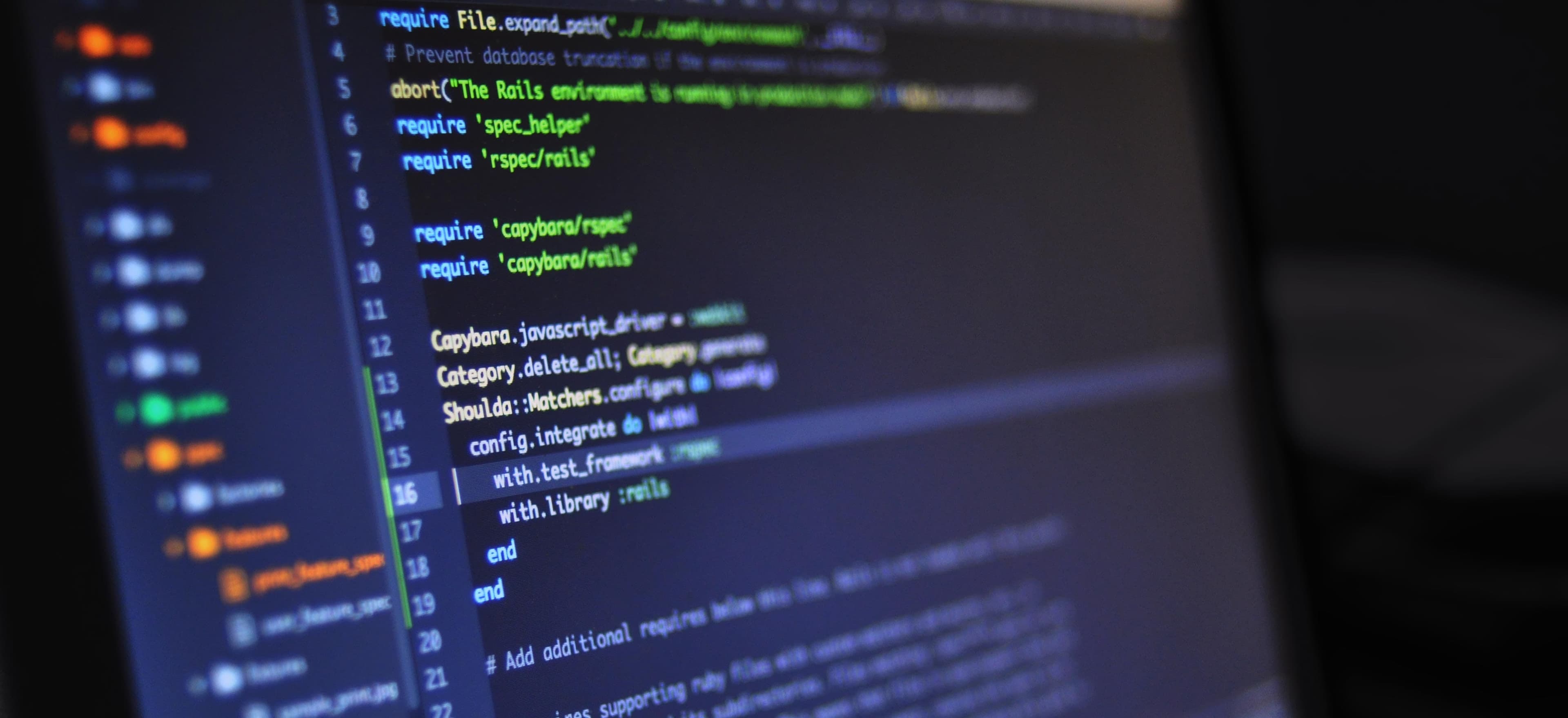
- Published on
Mastering Java: Compile and Run Without an IDE in Minutes
Java is one of the most popular programming languages in the world, widely recognized for its portability and scalability. While most developers use Integrated Development Environments (IDEs) like Eclipse, IntelliJ IDEA, or NetBeans to write and manage their Java projects, it's also beneficial to understand how to compile and run Java applications directly from the command line. This blog post will guide you through the steps to compile and run Java code without using any IDE.
Why Compile and Run Java without an IDE?
-
Understanding Core Concepts: Using the command line allows you to grasp how the Java Development Kit (JDK) works, which ultimately enhances your understanding of the Java programming cycle: writing code, compiling it into bytecode, and executing that bytecode on the Java Virtual Machine (JVM).
-
Lightweight Development: For simple programs and quick experiments, using the command prompt or terminal reduces overhead and streamlines the process. It’s a great way to test snippets of code without the additional features of an IDE.
-
Remote Development: When working on remote servers or cloud environments, the command line is often the only option. Understanding how to compile and run Java in this way prepares you for a more versatile development experience.
Prerequisites
Before we dive into the code, make sure that you have the following:
- Java Development Kit (JDK): Download the latest version from the Oracle website or OpenJDK.
- Environment Variables: Ensure that the JDK's
bin
directory is added to your system's path variable. This allows you to runjavac
andjava
commands from any command line interface.
To check if JDK is correctly installed, open your terminal or command prompt and run:
java -version
You should see the version information for Java if it is set up correctly.
Writing Your First Java Program
Let's start with a simple Java program: a "Hello, World!" application. This classic example will introduce you to the basics of Java syntax and structure.
HelloWorld.java
Create a new text file named HelloWorld.java
and add the following code:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Explanation of the Code
public class HelloWorld
declares a public class namedHelloWorld
. In Java, every application must have at least one class.public static void main(String[] args)
defines themain
method. This is the entry point where the Java Virtual Machine starts executing the program.System.out.println("Hello, World!");
outputs the string "Hello, World!" to the console.
Saving Your Code
Make sure to save the file with the .java
extension and that it matches the class name. Java is case-sensitive and class names must match the filename.
Compiling Java Code from the Command Line
Once you've saved your HelloWorld.java
file, open your terminal or command prompt and navigate to the directory where your file is located.
You can compile your Java program using the javac
command:
javac HelloWorld.java
Explanation of the Compile Command
javac
is the Java compiler that transforms your Java source code (.java
file) into bytecode (.class
file).- If successful, the command will not produce any output, but you will see a new file named
HelloWorld.class
in the same directory.
Running the Compiled Java Program
To execute your compiled Java program, you can use the java
command:
java HelloWorld
Explanation of the Run Command
java
is the Java runtime that interprets bytecode and executes it on the JVM.- You don’t include the
.class
extension when running the command.
If everything is set up correctly, this will output:
Hello, World!
Troubleshooting Common Issues
- Class Not Found/Error Messages: Ensure that your file names and class names match precisely, as Java is case-sensitive.
- JAVA_HOME Not Set: If you encounter errors indicating that Java is not recognized, verify that the JDK path is added to your environment variables correctly.
- File Not Found: Make sure you are in the correct directory when running
javac
orjava
. Use thecd
command to change directories.
Compiling and Running Multiple Files
In real-world applications, you often work with multiple .java
files. To compile them together, you can simply list all the source files in the javac
command:
javac File1.java File2.java
Alternatively, if you are inside a package or need to handle all Java files in the directory:
javac *.java
After compiling, run the main class as shown previously.
Bringing It All Together
Mastering the command-line basics of Java will empower you as a developer. Not only does it enhance your understanding of how Java compiles and runs, but it also equips you to work efficiently in various environments.
Next Steps
- Experiment with more complex Java programs to deepen your understanding.
- Explore additional features of the Java language, such as object-oriented programming principles, which can significantly expand your programming capabilities.
- Check out online resources and documentation for Java tutorials and best practices.
Getting comfortable with compiling and running Java code from the command line will make you a more versatile and capable Java developer in the long run. Happy coding!
Checkout our other articles