The Silent Struggle: Bridging Builders with Manipulators
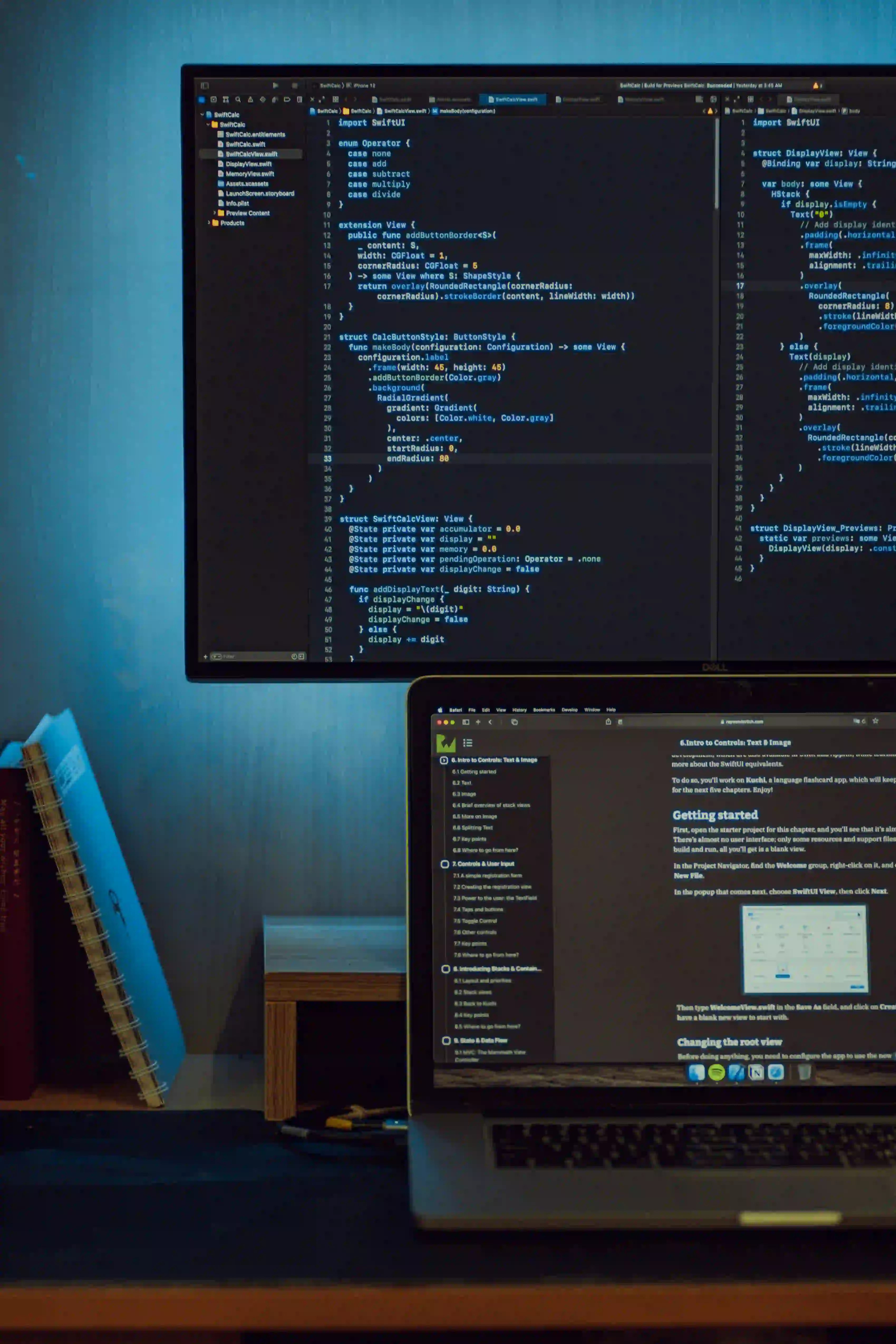
The Silent Struggle: Bridging Builders with Manipulators
In the world of software development, two distinct types of individuals often emerge: the Builders and the Manipulators. Builders are the architects and coders who create intricate systems, while Manipulators are the ones who navigate and interact with these systems, often using existing tools and frameworks to get results. Understanding the dynamics between these groups can lead to efficient collaboration and innovation.
Understanding Builders and Manipulators
Who are Builders?
Builders are the individuals who construct the foundational blocks of software applications. They write code, engineer features, and design databases. Their prowess lies in creating robust systems that perform efficiently. Builders are often detail-oriented, focusing on scalability, maintainability, and performance.
Characteristics of Builders:
- They thrive on challenges and seek to develop solutions.
- They possess deep technical knowledge and problem-solving skills.
- They often favor clean, efficient code over quick hacks.
Who are Manipulators?
Manipulators, on the other hand, are the users of the software builders create. They leverage these systems to achieve goals and drive outcomes within businesses. Their role is less about creating code and more about optimizing the use of existing technologies to solve problems effectively.
Characteristics of Manipulators:
- They are adept at assessing systems and finding pathways to success.
- They focus on usability and outcome rather than underlying technical specifics.
- They often utilize existing tools and frameworks to enhance their productivity.
The Silent Struggle
The gap between Builders and Manipulators often leads to miscommunication. Builders might feel their work is being undervalued. Conversely, Manipulators may feel constrained by the limitations of the systems built by Builders. Bridging this divide requires understanding, collaboration, and effective communication.
Building Bridges Through Communication
To foster better collaboration, both Builders and Manipulators must engage in open lines of communication. This involves not only sharing knowledge but also understanding each other's pain points. Here’s how to do it effectively:
1. Regular Meetings
Regular stand-up meetings or check-ins can help clarify expectations and address issues as they arise. These meetings should encourage open discussions about challenges encountered by both groups.
2. Documentation
Both Builders and Manipulators benefit from thorough documentation. Builders can create detailed technical guides, while Manipulators should provide feedback on usability. Documentation ensures that everyone is on the same page.
3. Unified Goals
Understanding and aligning on common goals is paramount. This could mean focusing on user satisfaction, system performance, or business outcomes. Identifying shared objectives fosters collaboration.
The Technical Aspect: Code Snippet Breakdown
For Illustrative purposes, let's examine a simple Java implementation that highlights the collaboration between Builders and Manipulators.
public class Calculator {
// Method to add two numbers
public int add(int a, int b) {
return a + b;
}
// Method to subtract two numbers
public int subtract(int a, int b) {
return a - b;
}
// Main method to demonstrate usage
public static void main(String[] args) {
Calculator calculator = new Calculator();
// Manipulators can use this method directly for simple calculations
System.out.println("Addition: " + calculator.add(10, 5));
System.out.println("Subtraction: " + calculator.subtract(10, 5));
}
}
Why This Code Matters
-
Simplicity: The
Calculator
class provides a simple interface for performing basic arithmetic operations. Manipulators can easily use these methods without needing to understand the underlying complexities. -
Modularity: Builders typically build components in a modular fashion, allowing easier updates and maintenance. If a new operation needs to be added, it can be done without impacting existing methods.
-
Usability: By making this functionality available in a straightforward manner, Builders empower Manipulators to execute their tasks efficiently without getting bogged down in technical details.
Overcoming Challenges
Despite the potential for collaboration, several challenges must be navigated successfully. Some common obstacles include:
-
Technical Jargon: Builders may use language that is too technical for Manipulators. Simplifying terms and using relatable metaphors can bridge understanding.
-
Differing Priorities: Builders may prioritize code efficiency, while Manipulators focus on user experience. Joint meetings can ensure that both perspectives are considered.
Tools for Collaboration
Several tools can aid in fostering communication and collaboration between Builders and Manipulators:
1. Agile Boards
Adopting Agile methodologies can help prioritize tasks and visualize project progress. Tools like Jira or Trello enable Builders and Manipulators to see where collaboration is necessary.
2. Code Repositories
Using platforms like GitHub fosters a shared space for Builders to showcase their work. Manipulators can also contribute through issue reporting or feature requests.
3. Feedback Loops
Encouraging feedback from Manipulators can provide Builders with insights on usability issues. Implementing user experience tests can be invaluable.
For further reading on the importance of UX design in software development, check out this comprehensive UI/UX Guide which covers many best practices.
In Conclusion, Here is What Matters
Bridging the divide between Builders and Manipulators can empower organizations to build better products and achieve business outcomes more efficiently. By fostering communication, embracing collaboration, and utilizing the right tools, both groups can work harmoniously toward common goals.
In the end, it’s about recognizing the value each role brings to the table. With a silenced struggle transformed into a collaborative effort, the resulting synergy can lead to remarkable advancements in the world of software development.
Let’s continue to break down those walls and build a more integrated future for developers and users alike!