Common Selenium Mistakes: How to Avoid Them in Testing
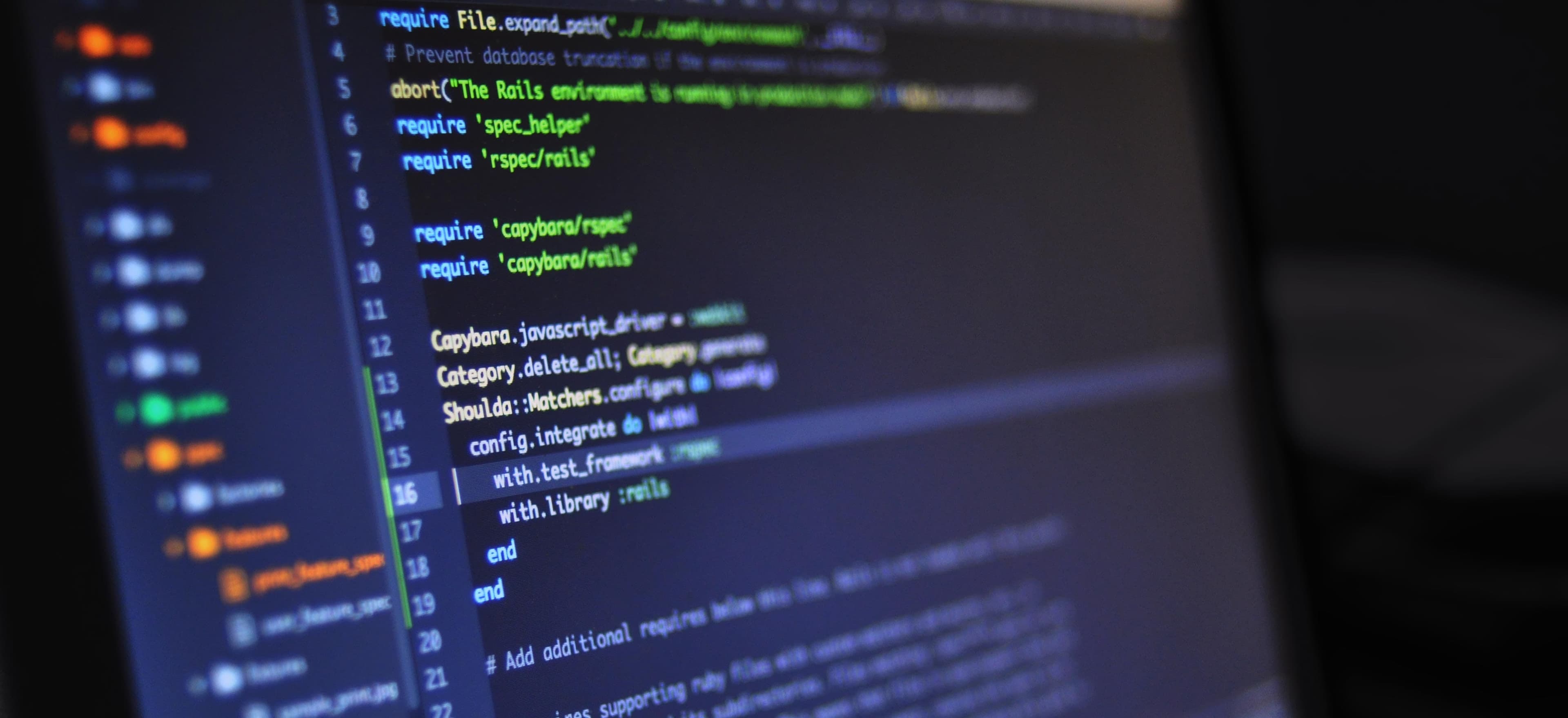
- Published on
Common Selenium Mistakes: How to Avoid Them in Testing
Selenium is an exceptionally powerful tool for automating web applications for testing purposes. As with any technology, developers and testers can stumble into common pitfalls that hinder the effectiveness of their automated tests. In this post, we’ll explore common Selenium mistakes, offering practical solutions to help you avoid them.
Mistake 1: Ignoring Wait Strategies
One of the most frequent mistakes when working with Selenium is neglecting to implement proper wait strategies. When interacting with web elements, developers often face issues with timing. Actions in web applications—such as loading new pages or waiting for elements to appear—can result in NoSuchElementException
or ElementNotInteractableException
.
Solution: Utilize Explicit and Implicit Waits
Using wait strategies correctly can save you from many headaches. Below is an example demonstrating both explicit and implicit waits.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class WaitExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10)); // Implicit wait
driver.get("https://example.com");
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(20)); // Explicit wait
// Example of using explicit wait
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("myElement")));
element.click(); // Ensure the element is present before clicking
}
}
By using this dual approach of implicit and explicit waits, you're ensuring your code is resilient against fluctuating page load times. Always strive to optimize waits according to the needs of your web application, as too much waiting can lead to longer test execution times.
For further insights into using Selenium waits effectively, check out this guide.
Mistake 2: Over-Reliance on Xpath
Xpath is a powerful tool for locating elements, but over-relying on it can introduce performance issues and fragility in your tests. For instance, complex Xpath expressions can slow down execution and lead to brittle code that breaks frequently when the UI changes.
Solution: Prefer CSS Selectors When Possible
CSS selectors are usually faster and more efficient than Xpath. They can also be easier to read and maintain. Here’s how you can implement CSS selectors in your Selenium tests:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CssSelectorExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Use a CSS selector instead of XPath
WebElement element = driver.findElement(By.cssSelector("button#submit"));
element.click();
}
}
In this code snippet, we’ve replaced an Xpath locator with a CSS selector, leading to faster execution times and clearer code. Prioritize using CSS selectors, reserving Xpath for more complex scenarios when absolutely necessary.
Mistake 3: Not Cleaning Up After Tests
Neglecting to close or clean up browser instances after test execution is another commonly overlooked issue. Failing to do so can lead to memory leaks and consume excessive system resources.
Solution: Always Use driver.quit()
To ensure that resources are released properly, use driver.quit()
at the end of your tests. Here’s an illustration:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class CleanupExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
try {
driver.get("https://example.com");
// Perform test actions here
} finally {
driver.quit(); // Ensure browser is closed properly
}
}
}
By implementing driver.quit()
within a finally
block, you can ensure that the browser is closed even if an exception occurs. This tiny addition can lead to improved performance and less clutter.
Mistake 4: Lack of Exception Handling
Failing to implement error-handling mechanisms can result in extensive test failures, making debugging difficult. It can also prevent your automated tests from continuing when facing transient issues like temporary network interruptions.
Solution: Use Try-Catch Blocks
Here is an example of how to use try-catch blocks for effective error handling.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class ExceptionHandlingExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
try {
driver.get("https://example.com");
try {
WebElement element = driver.findElement(By.id("nonExistentElement"));
element.click();
} catch (NoSuchElementException e) {
System.out.println("Element not found, continuing with the next test.");
}
} finally {
driver.quit();
}
}
}
In this example, we’re wrapping our element interaction in an inner try-catch block to handle specific exceptions. This practice improves resilience, allowing your automated tests to continue running even when minor issues occur.
Mistake 5: Poor Test Organization
Disorganized code can quickly become unwieldy. As your Selenium test suite grows, messy code can make it almost impossible to maintain and debug.
Solution: Implement Page Object Model (POM)
Utilizing the Page Object Model (POM) pattern can significantly bolster the organization of your tests. POM promotes the separation of page-specific logic from your test scripts, making your code cleaner, more readable, and easier to maintain.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
class LoginPage {
WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
WebElement usernameField = driver.findElement(By.id("username"));
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.sendKeys(password);
}
public void clickSubmit() {
WebElement submitButton = driver.findElement(By.id("submit"));
submitButton.click();
}
}
This example shows how to create a page object for a login page. Each page class should contain methods that encapsulate interactions with that page’s elements. This separation allows you to update the UI in one place without affecting multiple test files.
Closing Remarks
Selenium is an invaluable tool in a tester’s arsenal, but it comes with its own set of common pitfalls. By avoiding mistakes such as ignoring wait strategies, over-reliance on Xpath, failing to clean up resources, neglecting exception handling, and poor test organization, you can elevate your test automation to the next level.
Always strive for clarity, maintainability, and robustness in your test code. For in-depth learning on test automation strategies, consider this comprehensive Selenium testing guide.
With these insights, you should be well-equipped to sidestep common Selenium mistakes, making your testing efforts more effective and efficient. Happy testing!