Mastering Java Reflection: Retrieve Class Method Details Easily
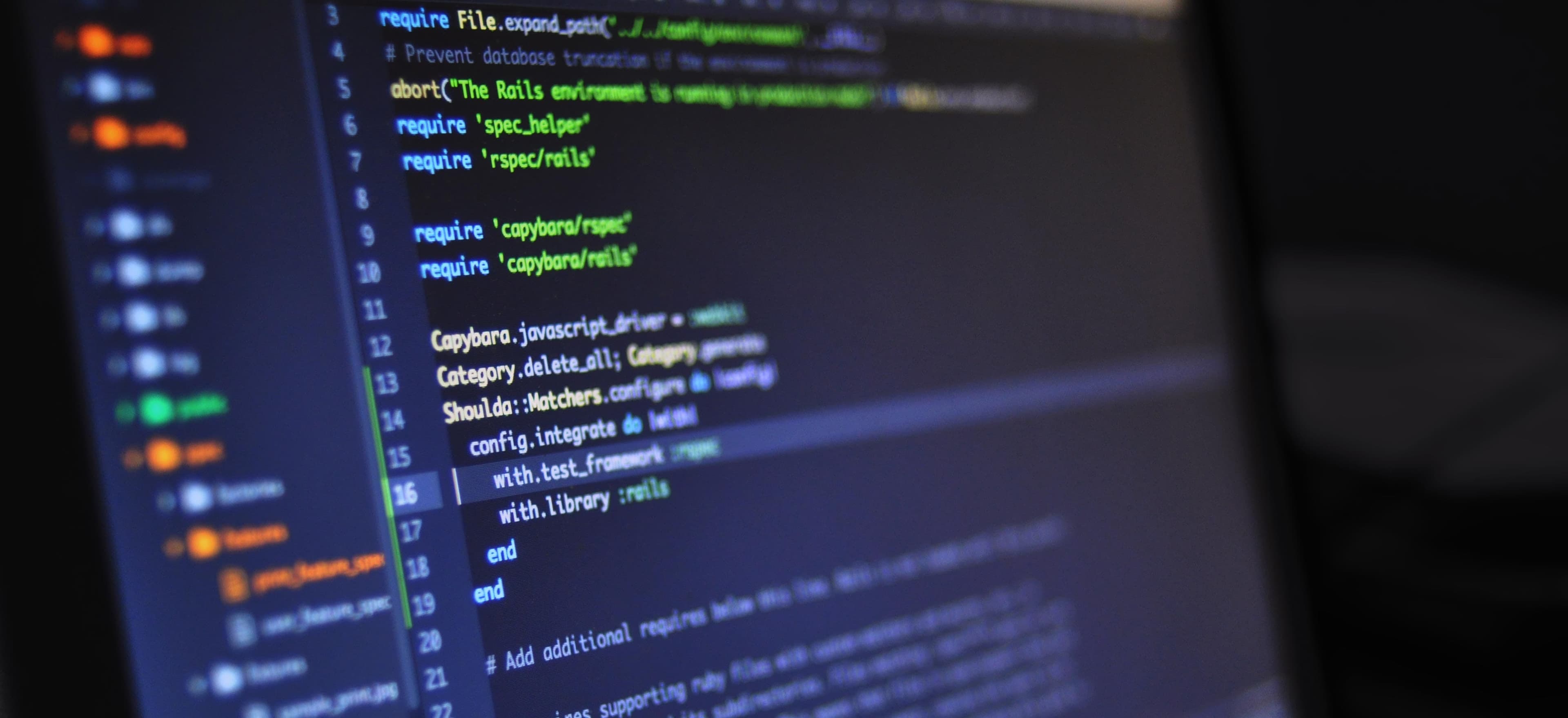
- Published on
Mastering Java Reflection: Retrieve Class Method Details Easily
Java is a powerful and versatile programming language, loved by millions for its simplicity, portability, and rich ecosystem. One of its advanced features is reflection, which allows a program to inspect and manipulate its own structure at runtime. In this blog post, we will delve into the intricacies of Java reflection, particularly focusing on how to retrieve method details from a class.
Understanding Java Reflection
Reflection is a concept that enables you to analyze and interact with classes, interfaces, fields, and methods at runtime. This capability opens up numerous possibilities, such as:
- Dynamic method invocation
- Class loading at runtime
- Inspecting class annotations
- Building flexible frameworks and libraries.
However, it’s important to use reflection judiciously, as it can lead to performance overhead and compromise type safety. The ability to manipulate types dynamically can introduce bugs that are hard to track down, making debugging more complicated.
Why Use Reflection?
- Dynamic Behavior: Reflection allows you to implement dynamic behavior in your applications, for instance, factories that can create objects at runtime without knowing their types at compile time.
- Framework Development: Many frameworks like Spring and Hibernate make extensive use of reflection to manage and manipulate Java objects.
- Tooling and APIs: Reflection is essential for many tools and libraries that depend on modifying or analyzing types, like serialization libraries and testing frameworks.
Retrieving Class Method Details
To retrieve method details from a class using reflection, we make use of the java.lang.reflect.Method
class. This class provides methods to inspect the details of methods declared in a class—such as the method name, return type, parameter types, and annotations.
Example: Retrieving Method Details
Let's start by creating a simple Java class and then use reflection to retrieve its method details.
Step 1: Create a Sample Class
public class SampleClass {
public void methodOne() {
System.out.println("Method One Executed");
}
private int methodTwo(int number) {
return number * 2;
}
protected String methodThree(String str) {
return "Hello, " + str;
}
}
In this SampleClass
, there are three methods: methodOne
, methodTwo
, and methodThree
. They illustrate different access modifiers: public, private, and protected.
Step 2: Use Reflection to Retrieve Method Details
Here's how you can use Java reflection to retrieve and print the details of the methods in the SampleClass
.
import java.lang.reflect.Method;
public class ReflectionDemo {
public static void main(String[] args) {
Class<SampleClass> sampleClass = SampleClass.class;
// Retrieve all methods
Method[] methods = sampleClass.getDeclaredMethods();
for (Method method : methods) {
// Print method name
System.out.println("Method Name: " + method.getName());
// Print return type
System.out.println("Return Type: " + method.getReturnType());
// Print parameter types
Class<?>[] parameterTypes = method.getParameterTypes();
System.out.print("Parameter Types: ");
for (Class<?> paramType : parameterTypes) {
System.out.print(paramType.getSimpleName() + " ");
}
System.out.println("\n");
}
}
}
Explanation of the Code
-
Get Class Reference: We create a variable called
sampleClass
formatted to referenceSampleClass
. -
Retrieve Methods: Using
getDeclaredMethods()
, we obtain an array ofMethod
objects representing the methods declared in the class. -
Iterate Over Methods: A
for
loop iterates through each method, allowing us to retrieve details. -
Details Printed:
- Method Name:
method.getName()
retrieves the method's name. - Return Type:
method.getReturnType()
returns the class object of the return type. - Parameter Types:
method.getParameterTypes()
retrieves an array of classes that represent the parameter types.
- Method Name:
Output
When you run the code, the output will display the details of all methods in SampleClass
, as follows:
Method Name: methodOne
Return Type: void
Parameter Types:
Method Name: methodTwo
Return Type: int
Parameter Types: int
Method Name: methodThree
Return Type: String
Parameter Types: String
Access Modifiers and Reflection
Reflection allows you to access private methods but requires use of setAccessible(true)
for accessibility. It is crucial to understand the implications of accessing non-public members. Here's how you could invoke the private method from earlier:
public class ReflectionDemoUtil {
public static void main(String[] args) throws Exception {
SampleClass sampleObj = new SampleClass();
Method methodTwo = SampleClass.class.getDeclaredMethod("methodTwo", int.class);
methodTwo.setAccessible(true); // Make it accessible
int result = (int) methodTwo.invoke(sampleObj, 5); // Invoke with argument
System.out.println("Result of private method: " + result);
}
}
Important Note
When manipulating methods using reflection, you compromise encapsulation and increase the risk of security vulnerabilities. Always validate the use case before accessing private methods or fields.
Further Learning Resources
To deepen your understanding of Java reflection, consider visiting the following resources:
Wrapping Up
Java reflection is a powerful feature that enables you to retrieve method details and perform dynamic operations. While it offers flexible and dynamic programming capabilities, it should be used judiciously, respecting principles such as code maintainability and type safety.
By mastering Java reflection, you will be better equipped to build sophisticated frameworks and dynamic applications. Happy coding!