Common Pitfalls When Using XStream for XML in Java
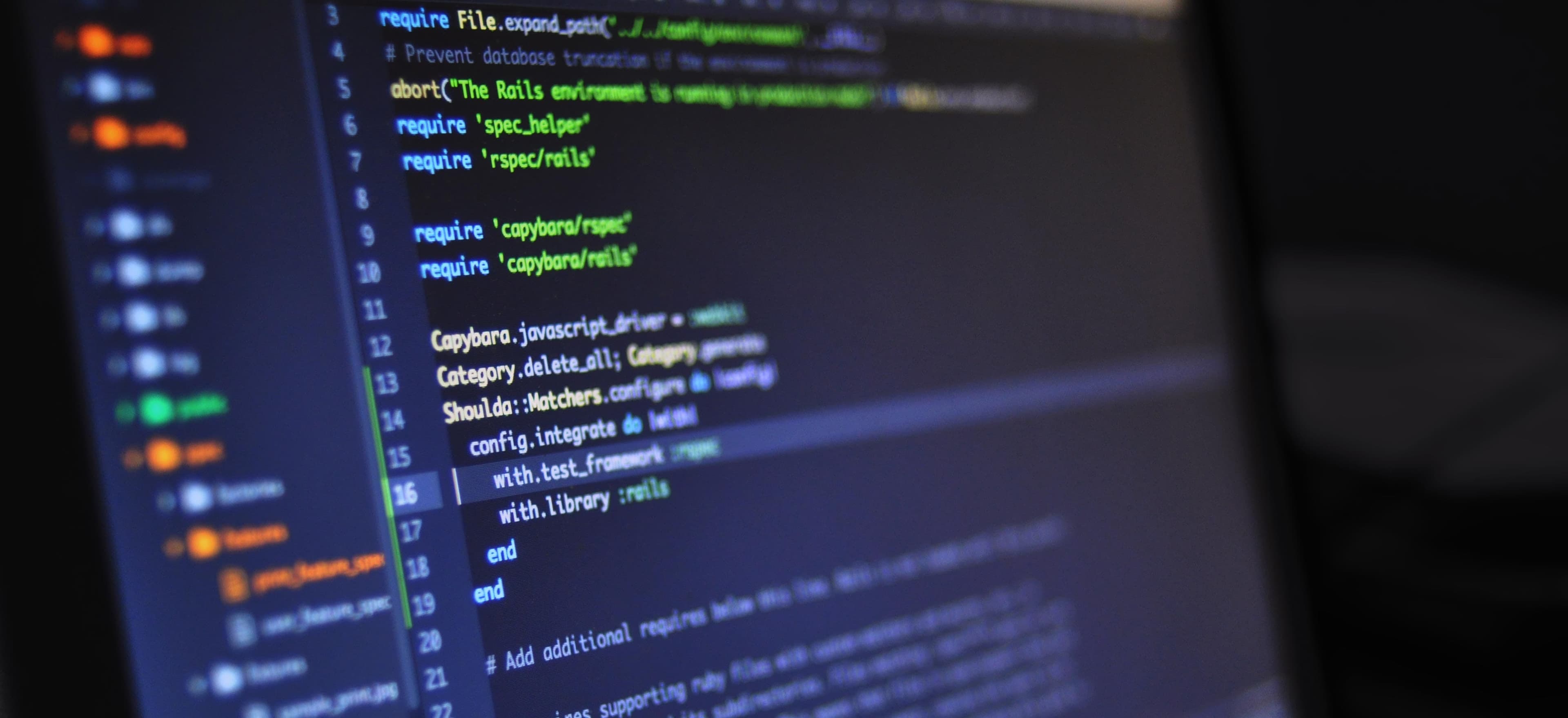
- Published on
Common Pitfalls When Using XStream for XML in Java
XStream is a powerful library in Java that facilitates the serialization of Java objects into XML and vice versa. It is valued for its simplicity and effectiveness, making it a popular choice for Java developers who require easy-to-use XML mapping solutions. However, like any framework, it has its quirks and potential pitfalls. In this blog post, we will explore the common pitfalls when using XStream for handling XML in Java, along with tips and best practices to avoid these issues.
Understanding XStream
Before we delve into potential issues, it's important to understand what XStream offers. XStream allows developers to create XML representations from Java objects without extensive configurations. Its core features include:
- Bidirectional conversion: Easily convert Java objects to XML and back.
- Annotations support: Using Java annotations for flexible XML configurations.
- Custom converters: Ability to create custom serialization and deserialization processes.
Setting Up XStream
To start using XStream, you'll need to add it to your project. If you are using Maven, include the following in your pom.xml
:
<dependency>
<groupId>com.thoughtworks.xstream</groupId>
<artifactId>xstream</artifactId>
<version>1.4.18</version> <!-- Check for the latest version -->
</dependency>
This dependency pulls in the latest version of XStream for your project.
Common Pitfalls When Using XStream
1. Not Configuring XStream Security
One of the most common pitfalls when using XStream is neglecting its security configurations. XStream has a security feature that prevents the deserialization of arbitrary classes. If you do not configure it, you may face ConversionException
.
Why is this important?
Not configuring security can allow unintended, potentially harmful classes to be instantiated during deserialization, leading to security vulnerabilities.
Example:
import com.thoughtworks.xstream.XStream;
public class XmlSecurityExample {
public static void main(String[] args) {
XStream xstream = new XStream();
// Initializing security
xstream.addPermission(AnyTypePermission.ANY); // This can be dangerous
// Alternatively, specify allowed classes
xstream.allowTypes(new Class[]{MyClass.class});
}
}
2. Ignoring Default Constructors
XStream requires default constructors for classes involved in XML serialization/deserialization. Failing to include a no-argument constructor can lead to errors during deserialization.
Why is this important?
Without a default constructor, XStream cannot instantiate the objects it tries to convert back from XML.
Example:
public class MyClass {
private String name;
// No-arg Constructor
public MyClass() {
}
public MyClass(String name) {
this.name = name;
}
}
3. Misusing Annotations
While XStream supports annotations for configuration, improper use can lead to confusion and unexpected results. Developers often mix XML configuration with annotations, which can result in conflicting serialization rules.
Why is this important?
Conflicting annotations can cause inconsistencies in the XML output, making the serialization process unpredictable.
Example of Proper Annotations Usage:
import com.thoughtworks.xstream.annotations.XStreamAlias;
@XStreamAlias("person")
public class Person {
@XStreamAlias("fullName")
private String name;
// Default constructor
public Person() {
}
public Person(String name) {
this.name = name;
}
}
4. Not Using Custom Converters
Often, developers overlook the benefits of custom converters, especially when working with complex objects or collections. Not utilizing them can lead to inefficient serialization, and loss of data integrity.
Why is this important?
Custom converters give you precise control over how your objects are serialized, ensuring that you can manage complex data types and relationships effectively.
Example of a Custom Converter:
import com.thoughtworks.xstream.converters.Converter;
import com.thoughtworks.xstream.converters.MarshallingContext;
import com.thoughtworks.xstream.converters.UnmarshallingContext;
import com.thoughtworks.xstream.io.HierarchicalStreamReader;
import com.thoughtworks.xstream.io.HierarchicalStreamWriter;
public class PersonConverter implements Converter {
@Override
public boolean canConvert(Class clazz) {
return clazz.equals(Person.class);
}
@Override
public void marshal(Object source, HierarchicalStreamWriter writer, MarshallingContext context) {
Person person = (Person) source;
writer.addAttribute("name", person.getName());
}
@Override
public Object unmarshal(HierarchicalStreamReader reader, UnmarshallingContext context) {
return new Person(reader.getAttribute("name"));
}
}
5. Ignoring the Importance of XML Structure
When serializing objects, you may inadvertently create complex or flat structures that don't accurately represent the original Java class design. How you design your class hierarchy affects the resulting XML.
Why is this important?
A poor XML structure can make it challenging to maintain, read, and process the generated XML documents.
Example of XML Structure Consideration:
public class Company {
private String name;
private List<Employee> employees;
public Company() {
this.employees = new ArrayList<>();
}
// Getters, Setters omitted for brevity
}
6. Assuming XML Is Always Well-formed
When using XStream, developers sometimes assume that the XML they are deserializing is well-formed. However, this assumption can result in significant errors when the XML structure does not align with the expected format.
Why is this important?
Malformed XML can lead to XStreamException
, disrupting the deserialization process and requiring additional debugging efforts.
Example Check:
String xml = "<company><name>MyCompany</name></company>";
try {
Company company = (Company) xstream.fromXML(xml);
} catch (XStreamException e) {
System.out.println("Caught exception: " + e.getMessage());
}
Best Practices to Avoid Pitfalls
-
Always Configure Security: Ensure you set up the necessary security permissions when initializing XStream.
-
Include Default Constructors: Check that all classes involved in serialization/deserialization have no-arg constructors.
-
Carefully Use Annotations: Prefer either XML configuration or annotations, but avoid mixing them to minimize conflicts.
-
Utilize Custom Converters When Necessary: For complex types and specific serialization needs, implement custom converters.
-
Maintain a Clear XML Structure: Be mindful of how classes are structured naturally and how that will reflect in the generated XML.
-
Validate XML Input: Always validate the XML before deserialization to ensure you're working with well-formed documents.
The Closing Argument
While XStream is an excellent tool for managing XML and Java objects, it is crucial to remain aware of potential pitfalls. By understanding these common issues and following best practices, you can leverage XStream's capabilities effectively. Whether you are dealing with simple objects or complex hierarchies, maintaining clarity and care when using XStream can lead to smoother development experiences and robust applications.
For further reading on XStream and enhancing XML handling in Java, check out the official XStream documentation and explore Java Object Serialization. Happy coding!