Mastering Recursive Functions in Functional Programming
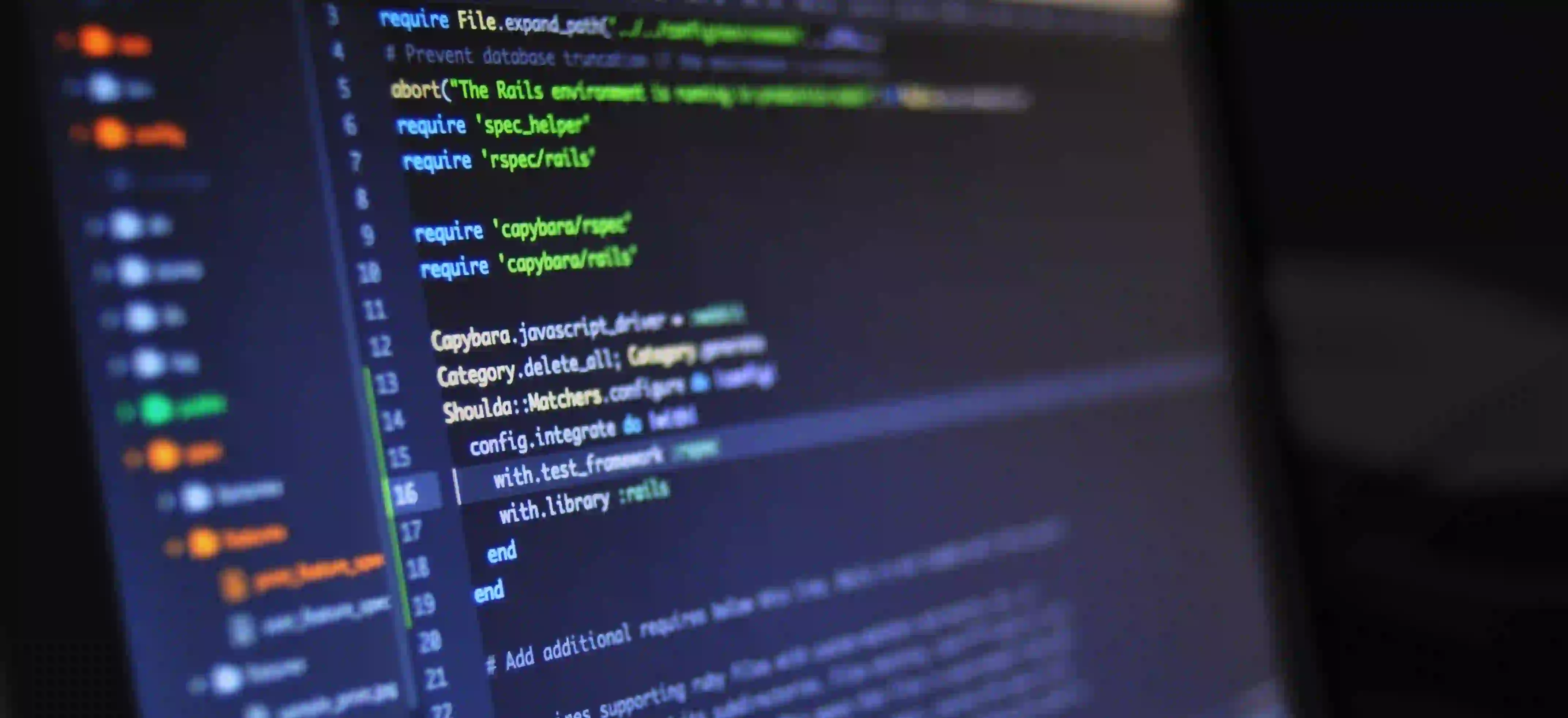
Mastering Recursive Functions in Functional Programming
Recursive functions are a hallmark of functional programming, and they can lead to elegant, concise solutions to problems. In this blog post, we will explore what recursion is, when and how to use it effectively, and examine examples in Java. Additionally, we will look at the pros and cons of recursive approaches compared to iterative solutions. Whether you're a beginner or an experienced developer, mastering recursion is a valuable skill that will enhance your programming toolkit.
What is Recursion?
Recursion refers to the process of a function calling itself to solve smaller instances of the same problem until a base condition is met. This divide-and-conquer approach can simplify difficult problems, making them easier to solve.
For instance, consider the classic problem of calculating the factorial of a number:
public static int factorial(int n) {
if (n == 0) {
return 1; // Base case: the factorial of 0 is 1
} else {
return n * factorial(n - 1); // Recursive case
}
}
Explanation of the Code
- Base Case: The function first checks if n is zero, which is our base case. Returning 1 in this case stops further recursion.
- Recursive Call: If n is greater than zero, the function calls itself with
n - 1
. This continues until the base case is reached.
The recursive approach breaks the problem down into smaller pieces, allowing for a straightforward solution.
When to Use Recursion?
Recursion is particularly useful in scenarios such as:
-
Tree Traversal: Recursion is ideal for traversing data structures like trees or graphs. For example, navigating a binary tree can be naturally expressed with recursive functions.
-
Divide and Conquer Algorithms: Algorithms like QuickSort or MergeSort rely heavily on recursion. The problems are split into smaller, more manageable parts and then solved independently.
-
Dynamic Programming: Many dynamic programming problems (like Fibonacci series calculations) can benefit from recursive functions, though memoization may be needed to avoid redundant calculations.
Pros and Cons of Recursive Functions
Pros:
- Simplicity: Recursive functions can make the code easier to read and understand. Problems often have a natural recursive structure.
- Reduction in Complexity: Recursive solutions can reduce the complexity of the code significantly, making it cleaner and easier to maintain.
Cons:
- Performance: Recursive calls add overhead due to function management and stack frame creation. This can lead to slower performance than iterative solutions, especially for large inputs.
- Stack Overflow: Deep recursion can lead to stack overflow errors because each recursive call consumes stack space.
Examples of Recursive Functions in Java
1. Fibonacci Series
The Fibonacci series is defined as follows:
F(0) = 0, F(1) = 1, F(n) = F(n-1) + F(n-2) for n > 1.
We can implement this using recursion:
public static int fibonacci(int n) {
if (n <= 1) {
return n; // Base cases
} else {
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
}
Commentary on Fibonacci Implementation
The Fibonacci function demonstrates the use of recursion beautifully. Each call to fibonacci
generates two more calls until the base cases are reached. However, this naive approach has exponential time complexity due to repeated calculations. This can be optimized using memoization.
2. Factorial Using Tail Recursion
Tail recursion is a special case of recursion where the recursive call is the last operation in the function. Java does not optimize tail recursion, but the concept is essential in functional programming:
public static int tailFactorial(int n, int accumulator) {
if (n == 0) {
return accumulator; // Base case
} else {
return tailFactorial(n - 1, n * accumulator); // Tail recursive case
}
}
public static int calculateFactorial(int n) {
return tailFactorial(n, 1); // Initial accumulator is 1
}
Explanation of Tail Recursive Factorial
-
Accumulator Parameter: The function takes an additional parameter,
accumulator
, which holds the intermediate result. This allows the function to complete its calculation without waiting for further calls to return. -
Efficiency: Although Java does not inherently optimize tail recursion, this method effectively simulates the iterative process by passing the accumulated result along.
Key Takeaways
-
Mastering Recursion: Understanding recursion can drastically improve your problem-solving skills. It allows for clean, expressive code that is often easier to reason about.
-
Balance Between Iterative and Recursive: While recursive solutions can be elegant, they are not always the most efficient. Assess your problem and consider both options.
-
Explore Advanced Concepts: Once comfortable with basic recursion, delve into more advanced concepts such as memoization and functional programming paradigms in Java (Oracle's Guide on Java Functional Programming).
The Closing Argument
In conclusion, mastering recursive functions in functional programming is not just about knowing how to implement them but understanding when to use them effectively. By evaluating the complexities of different approaches and leveraging the strengths of recursive solutions, you can write cleaner, more maintainable code.
Be sure to experiment with recursion in your projects, and over time, you will find it becoming a natural part of your programming repertoire. Happy coding!
For more insights on recursion and other programming concepts, check out this article on The Importance of Recursion in Computer Science that delves deeper into the nuances of recursive techniques.