Streamlining XML to JSON Conversion in Spring Batch with MongoDB
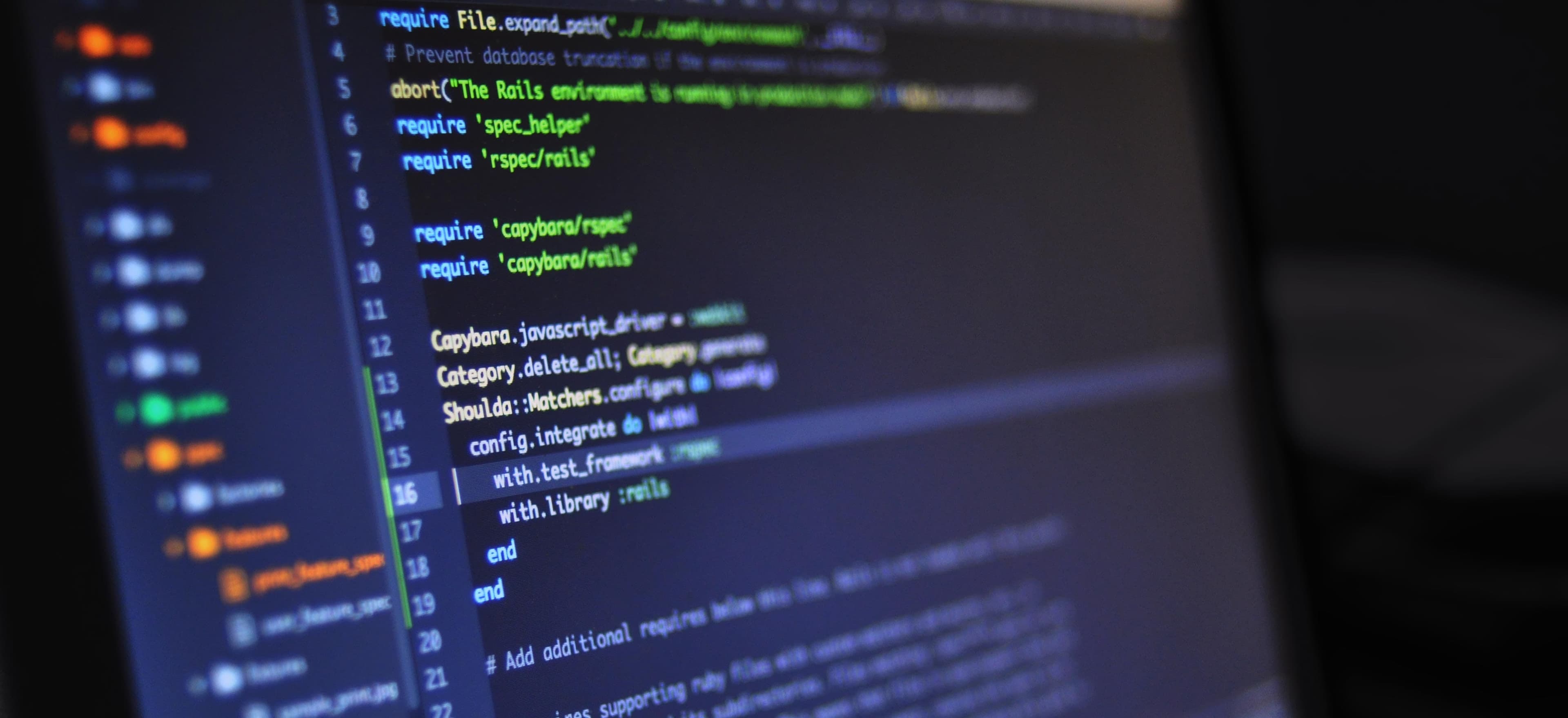
- Published on
Streamlining XML to JSON Conversion in Spring Batch with MongoDB
In today's data-driven world, the seamless integration of various data formats is crucial. With the increasing popularity of JSON over XML, converting XML data to JSON format has become an essential task for many applications. This blog post will explore an efficient technique to achieve XML to JSON conversion using Spring Batch while leveraging MongoDB as the data storage solution.
Overview of Spring Batch
Spring Batch is a powerful framework designed for processing large volumes of data both reliably and efficiently. It provides robust features such as chunk processing, transaction management, and job scheduling. This makes it an ideal choice for tasks that require batch processing, including data transformation between different formats.
Why Use MongoDB?
MongoDB is a NoSQL database that offers flexibility and scalability. It stores data in a document format, which aligns well with JSON structure. This makes it an excellent fit for a JSON-focused application.
Project Setup
To get started, ensure you have the following prerequisites installed on your machine:
- JDK 8 or higher
- Maven or Gradle
- Spring Boot
- MongoDB
Start by creating a Spring Boot project. You can use Spring Initializr to generate the project structure. Make sure to include the following dependencies:
- Spring Batch
- Spring Web
- Spring Data MongoDB
- Jackson XML (for XML parsing)
Here's an example of the Maven dependencies you might include in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-batch</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
Configuration
After setting up the project, we need to configure Spring Batch and MongoDB. Create a new configuration class:
import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.mongodb.config.AbstractMongoClientConfiguration;
@Configuration
@EnableBatchProcessing
public class BatchConfiguration extends AbstractMongoClientConfiguration {
@Override
protected String getDatabaseName() {
return "yourDatabase"; // Specify your MongoDB database name here
}
}
Why BatchConfiguration?
This class sets up the Spring Batch framework and the connection to MongoDB. By extending AbstractMongoClientConfiguration
, you can easily configure MongoDB settings.
XML to JSON Conversion Process
1. Job Configuration
Next, you need to create a Spring Batch job configuration that defines the steps for processing XML files and writing the results to MongoDB.
import org.springframework.batch.core.Step;
import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing;
import org.springframework.batch.core.configuration.annotation.StepBuilderFactory;
import org.springframework.batch.core.launch.support.RunIdIncrementer;
import org.springframework.batch.core.Job;
import org.springframework.batch.core.configuration.annotation.JobBuilderFactory;
import org.springframework.batch.item.ItemProcessor;
import org.springframework.batch.item.ItemReader;
import org.springframework.batch.item.ItemWriter;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class JobConfiguration {
private final JobBuilderFactory jobBuilderFactory;
private final StepBuilderFactory stepBuilderFactory;
public JobConfiguration(JobBuilderFactory jobBuilderFactory, StepBuilderFactory stepBuilderFactory) {
this.jobBuilderFactory = jobBuilderFactory;
this.stepBuilderFactory = stepBuilderFactory;
}
@Bean
public Job xmlToJsonJob() {
return jobBuilderFactory.get("xmlToJsonJob")
.incrementer(new RunIdIncrementer())
.flow(xmlToJsonStep())
.end()
.build();
}
@Bean
public Step xmlToJsonStep() {
return stepBuilderFactory.get("xmlToJsonStep")
.<YourDomainObject, YourDomainObject>chunk(10)
.reader(xmlItemReader())
.processor(xmlToJsonProcessor())
.writer(mongoItemWriter())
.build();
}
// Define your readers, processors, and writers here.
}
Why Job Configuration?
The JobConfiguration
class outlines the steps of your Spring Batch job, using a fluent builder API to create a complex workflow. You can easily adjust parameters, like chunk processing sizes for improved performance.
2. Item Reader
Now, let's implement the ItemReader
, which will read XML files and convert each one into a Java object.
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import org.springframework.batch.item.ItemReader;
import org.springframework.batch.item.file.mapping.DefaultLineMapper;
import org.springframework.batch.item.file.transform.LineTokenizer;
import org.springframework.batch.item.file.transform.DelimitedLineTokenizer;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import java.io.File;
import java.io.IOException;
public class XmlItemReader implements ItemReader<YourDomainObject> {
private final Resource resource = new ClassPathResource("input.xml");
private XmlMapper xmlMapper = new XmlMapper();
private Iterator<YourDomainObject> iterator;
@Override
public YourDomainObject read() throws Exception {
if (iterator == null) {
List<YourDomainObject> items = xmlMapper.readValue(resource.getFile(), new TypeReference<List<YourDomainObject>>() {});
iterator = items.iterator();
}
return iterator.hasNext() ? iterator.next() : null;
}
}
Why XmlItemReader?
Using XmlMapper
from the Jackson XML library allows you to map XML content directly to Java objects. This reduces the complexity of data handling and eliminates the need for manual parsing.
3. Item Processor
The next step is to convert the YourDomainObject
into JSON and prepare it for storage in MongoDB.
import org.springframework.batch.item.ItemProcessor;
public class XmlToJsonProcessor implements ItemProcessor<YourDomainObject, YourDomainObject> {
@Override
public YourDomainObject process(YourDomainObject item) throws Exception {
// Perform any necessary transformations on the item
// This method can also be used to validate or filter items
return item; // In this example, we're returning the same object
}
}
Why XmlToJsonProcessor?
The ItemProcessor
allows for any transformations before the data is written to MongoDB. You can enrich or modify the data as needed.
4. Item Writer
Finally, implement the ItemWriter
that will save the processed data into MongoDB.
import org.springframework.batch.item.ItemWriter;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class MongoItemWriter implements ItemWriter<YourDomainObject> {
private final MongoTemplate mongoTemplate;
public MongoItemWriter(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
@Override
public void write(List<? extends YourDomainObject> items) throws Exception {
for (YourDomainObject item : items) {
mongoTemplate.save(item); // Saves each item to the MongoDB collection
}
}
}
Why MongoItemWriter?
The ItemWriter
is responsible for persisting the processed data. The simplicity of using MongoTemplate
showcases the power of Spring Data’s integration with MongoDB.
Running the Batch Job
You can run the job using the command line or by adding a command-line runner to your Spring Boot application:
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class JobRunner implements CommandLineRunner {
private final JobLauncher jobLauncher;
private final Job xmlToJsonJob;
public JobRunner(JobLauncher jobLauncher, Job xmlToJsonJob) {
this.jobLauncher = jobLauncher;
this.xmlToJsonJob = xmlToJsonJob;
}
@Override
public void run(String... args) throws Exception {
JobParameters params = new JobParametersBuilder()
.addLong("time", System.currentTimeMillis())
.toJobParameters();
jobLauncher.run(xmlToJsonJob, params);
}
}
Why JobRunner?
The CommandLineRunner
ensures the job is initiated upon application startup. It adds flexibility, allowing you to execute the batch job whenever required.
The Last Word
By following these steps, we have simplified the process of converting XML data to JSON format using Spring Batch while storing the output in MongoDB. This approach not only highlights the capabilities of Spring Batch and MongoDB but also provides a robust framework for handling large datasets effectively.
With Spring Batch, you can easily configure and extend your job according to your specific requirements, and with the help of MongoDB, you gain the ability to handle data in the JSON format.
For more in-depth information on Spring Batch, check the official documentation. Additionally, for comprehensive MongoDB integration with Spring, refer to Spring Data MongoDB.
Next Steps
Consider enhancing this basic setup by adding features like error handling, job scheduling with Spring Scheduler, or even integrating Spring Cloud Data Flow for orchestration. With Spring Batch's powerful capabilities, the possibilities are limitless. Happy coding!