Overcoming Common Pitfalls in DJL with Spring Boot
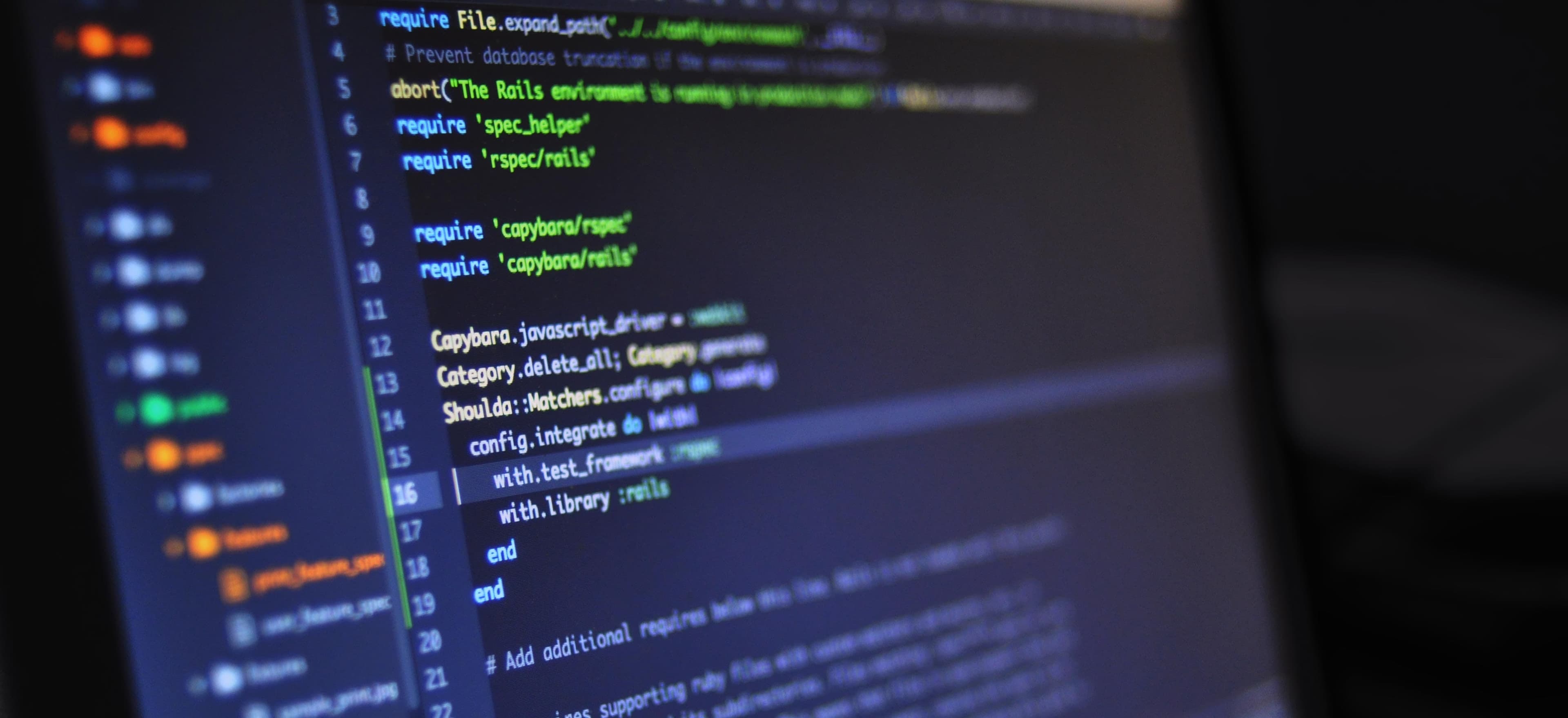
- Published on
Overcoming Common Pitfalls in DJL with Spring Boot
Deep Java Library (DJL) is a powerful framework designed to simplify deep learning in Java. When paired with Spring Boot, it can create robust applications capable of leveraging artificial intelligence. However, as with any technology stack, developers often encounter challenges. In this post, we will explore common pitfalls when using DJL with Spring Boot and suggest effective strategies to overcome them.
Understanding the Landscape of DJL and Spring Boot
Before diving into the common pitfalls, it's important to clarify what DJL and Spring Boot are.
- DJL is an open-source library that supports several deep learning engines, providing a high-level API for building, training, and deploying models in Java applications.
- Spring Boot streamlines the development of Spring-based applications. It is designed to make getting started with Spring easier and faster by eliminating boilerplate code.
By combing DJL with Spring Boot, developers gain the ability to build powerful machine learning applications capable of operating within a microservice architecture. This combination, however, is not without its complications.
Common Pitfalls in DJL with Spring Boot
1. Dependency Management
Pitfall: One of the first hurdles you might face is managing the correct dependencies for DJL along with Spring Boot. Users often overlook version compatibility, leading to runtime errors.
Solution: Make sure you are using compatible versions of DJL, Spring Boot, and their respective dependencies. Here's a sample pom.xml
snippet for Maven users:
<dependency>
<groupId>ai.djl.tensorflow</groupId>
<artifactId>djl-tensorflow-engine</artifactId>
<version>0.14.0</version> <!-- Use the latest available -->
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.0</version> <!-- Use the latest Stable version -->
</dependency>
2. Model Loading and Initialization
Pitfall: Developers often make the mistake of loading models synchronously during application startup. This can significantly delay your application startup time.
Solution: Load models asynchronously or on-demand. Here's a sample code snippet that demonstrates how to load a model asynchronously in a Spring Boot Application:
import ai.djl.MalformedModelException;
import ai.djl.translate.TranslateException;
@Service
public class ModelService {
private Model model;
@PostConstruct
public void init() {
CompletableFuture.runAsync(() -> {
try {
model = Model.newInstance("model_name");
} catch (MalformedModelException e) {
e.printStackTrace();
}
});
}
public String predict(String input) throws TranslateException {
// Ensure the model is loaded before making predictions
if (model == null) {
throw new IllegalStateException("Model not loaded yet!");
}
// Perform prediction logic
}
}
In this example, we initialize the model on a separate thread to minimize startup delays. The @PostConstruct
annotation ensures that the method runs after the service’s construction.
3. Memory Management Issues
Pitfall: Deep learning applications are memory-intensive. Java’s garbage collection (GC) may not handle memory as effectively under heavy loads, leading to OutOfMemoryError
.
Solution: Monitor and fine-tune memory settings. You can also utilize DJL's features to load and unload models as needed. Here’s how to configure JVM options:
java -Xms512m -Xmx2048m -jar your-spring-boot-app.jar
This command sets the initial heap size to 512 MB and the maximum heap size to 2048 MB. Adjust these values according to your application’s needs.
4. Logging and Debugging
Pitfall: Developers often neglect logging, which can make troubleshooting extremely difficult. Without proper logging, pinpointing the source of errors—such as model loading failures or prediction errors—can become a tedious process.
Solution: Implement structured logging in your application. Using libraries like SLF4J makes it easy to log different levels of events. Here’s a simple logging setup:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@RestController
public class PredictionController {
private static final Logger logger = LoggerFactory.getLogger(PredictionController.class);
@GetMapping("/predict")
public ResponseEntity<String> makePrediction(@RequestParam String input) {
logger.info("Received input for prediction: {}", input);
// Prediction logic
String result = predict(input);
logger.info("Prediction result: {}", result);
return ResponseEntity.ok(result);
}
}
In this code, we log events at different stages, allowing developers to trace the flow of requests and identify issues quickly.
5. Resource Cleanup
Pitfall: Not properly managing resources can lead to memory leaks. It's important to release GPU or CPU resources after they're no longer needed.
Solution: Implement resource cleanup logic using the @PreDestroy
annotation to ensure you release resources properly:
@PreDestroy
public void cleanup() {
if (model != null) {
model.close();
}
}
This will safely close the model and free up resources upon application shutdown.
6. Performance Optimization
Pitfall: Developers often overlook performance optimizations, resulting in slow prediction times. Inefficient data preprocessing or model inference can degrade the application’s responsiveness.
Solution: Analyze and optimize your data pipeline. Make use of multithreading for data preparation. Here's a basic idea of how to make predictions concurrently:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
private ExecutorService executorService = Executors.newFixedThreadPool(4);
public CompletableFuture<String> asyncPredict(String input) {
return CompletableFuture.supplyAsync(() -> predict(input), executorService);
}
This approach can significantly enhance performance, especially when dealing with multiple incoming requests.
Lessons Learned
Overcoming the common pitfalls in DJL with Spring Boot requires awareness and proactive strategies. By carefully managing dependencies, optimizing resource usage, ensuring proper initialization, and incorporating effective logging, developers can harness the power of deep learning in Java more efficiently.
For additional context on DJL and Spring Boot, you can check the DJL Documentation and the Spring Boot Documentation.
By adhering to the best practices outlined here, you can navigate through potential hurdles and build scalable and responsive deep learning applications that fully utilize the capabilities of DJL and Spring Boot. Happy coding!
Checkout our other articles