Overcoming Unit Testing Challenges in Java and JavaScript
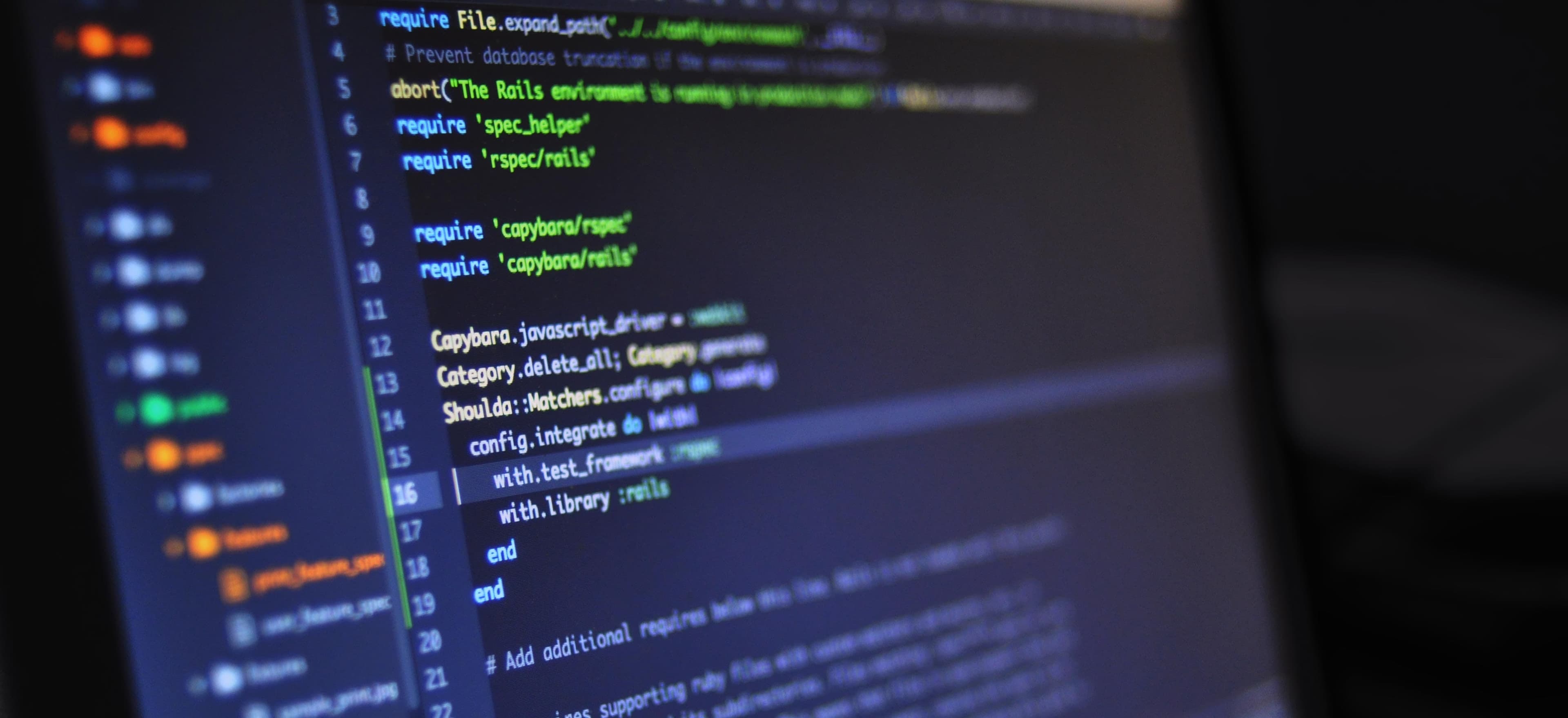
- Published on
Overcoming Unit Testing Challenges in Java and JavaScript
Unit testing is essential for ensuring the quality and reliability of software. It allows developers to test individual parts of the codebase, thus identifying bugs early in the development cycle. However, both Java and JavaScript come with their own unique challenges when it comes to unit testing. In this blog post, we will discuss these challenges and provide effective strategies to overcome them.
Understanding Unit Testing
Unit testing refers to the process of testing individual units or components of software to verify that they work as intended. In both Java and JavaScript, this involves writing tests that check the behavior of functions and methods.
Why Is Unit Testing Important?
- Bug Detection: Unit tests help detect bugs early in the development process, reducing the cost of fixing them later.
- Documentation: They serve as a form of documentation, explaining how a piece of code is supposed to behave.
- Refactoring Assurance: Unit tests give developers the confidence to refactor code, knowing that they have tests that will catch any regressions.
Common Unit Testing Challenges
1. Complex Dependencies
Both Java and JavaScript often have components that depend on other parts of the system or external services. This can make unit testing difficult because it becomes challenging to isolate the unit being tested.
Java Example: Using Mocks
In Java, you can use libraries like Mockito to create mock objects that replace dependencies during testing. Here’s a simple example:
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockRepository = mock(UserRepository.class);
UserService userService = new UserService(mockRepository);
User user = new User(1, "James");
when(mockRepository.findById(1)).thenReturn(user);
User result = userService.getUser(1);
assertEquals("James", result.getName());
}
}
Why This Works: Here, we are mocking the UserRepository
, allowing us to focus solely on testing the UserService
without worrying about the database.
2. Lack of Clear API Contracts
In JavaScript, especially in dynamically typed languages, the API contracts may not be well defined. This can lead to unforeseen issues during testing.
JavaScript Example: JSDoc for Documentation
Using JSDoc can aid in maintaining clear API contracts:
/**
* Adds two numbers.
* @param {number} a - The first number.
* @param {number} b - The second number.
* @return {number} The sum of the two numbers.
*/
function add(a, b) {
return a + b;
}
Why This Matters: By documenting the function with JSDoc, you create a clear contract that indicates what input types are expected and what the output will be. This helps in writing accurate unit tests.
3. Global State and Side Effects
Both Java and JavaScript can suffer from issues related to global state or side effects, which can make unit tests flaky.
Java Example: Avoiding Shared State
A common strategy is to avoid shared state in tests. Instead, use local variables. Here’s a before-and-after example:
public class Calculator {
private int lastResult;
public int add(int a, int b) {
lastResult = a + b;
return lastResult;
}
}
// Bad Practice:
@Test
public void testAdd() {
Calculator calculator1 = new Calculator();
Calculator calculator2 = new Calculator();
assertEquals(3, calculator1.add(1, 2));
assertEquals(5, calculator2.add(2, 3)); // This can affect test outcomes
}
Why Avoid Shared State?: Each test should be able to run in isolation to produce consistent results, regardless of the order in which they are executed.
JavaScript Example: Using Cleanups
In JavaScript, consider using libraries such as Jest that support setup and teardown:
let testUser;
beforeEach(() => {
testUser = createUser("Test Name");
});
afterEach(() => {
testUser = null; // Clean up
});
test("test user creation", () => {
expect(testUser.name).toBe("Test Name");
});
Clean State: By using beforeEach
and afterEach
, we ensure that each test starts with a clean slate.
4. Insufficient Tooling
The landscape of testing tools can be overwhelming. Choosing the right framework is crucial for effective unit testing.
- Java: JUnit and Mockito are the go-to libraries.
- JavaScript: Jest and Mocha have gained significant popularity.
Try to integrate Continuous Integration (CI) tools to automate your testing process as part of the development cycle. This can drastically improve the effectiveness of your unit testing effort.
5. User Input Testing
Testing user input is another significant challenge. You need to simulate how user interaction affects your application.
Java Example: Testing with Spring MVC
In a Spring MVC application, you can simulate user input:
@Test
public void testUserInput() throws Exception {
mockMvc.perform(post("/users")
.param("name", "John"))
.andExpect(status().isOk());
}
Why Simulate User Input?: By leveraging mockMvc, you can perform end-to-end tests that simulate user actions without needing a GUI.
JavaScript Example: Simulating Events
In JavaScript, libraries like Testing Library help simulate user input:
import { render, fireEvent } from '@testing-library/react';
import MyComponent from './MyComponent';
test('handles user input', () => {
const { getByLabelText } = render(<MyComponent />);
const input = getByLabelText(/name/i);
fireEvent.change(input, { target: { value: 'Alice' } });
expect(input.value).toBe('Alice');
});
User Interaction Simulation: Simulating user input helps in understanding how your application behaves under actual user conditions.
Best Practices for Effective Unit Testing
-
Write Testable Code: Design your code with testing in mind. Follow principles like SOLID to create components that are easy to test.
-
Use Descriptive Test Cases: Name your test cases clearly. They should express the purpose and behavior of the code being tested.
-
Keep Tests Isolated: Each test should run independently of others to avoid interdependence.
-
Refactor Regularly: Just as you refactor your production code, refactor your tests to keep them clean and efficient.
-
Leverage Test Coverage Tools: Tools like Jacoco for Java and Istanbul for JavaScript provide valuable insights into untested parts of your code.
In Conclusion, Here is What Matters
Unit testing in Java and JavaScript can present challenges like complex dependencies, unclear API contracts, and global state issues. However, by utilizing the right techniques and best practices, such as mocking dependencies, simulating user input, and maintaining clean test environments, you can effectively overcome these challenges.
Whether you're building large-scale enterprise applications in Java or developing interactive web applications in JavaScript, investing time and resources in unit testing will pay dividends in code quality and maintainability.
For more detailed insights into unit testing practices, you may want to check the JUnit 5 User Guide and Jest Documentation.
By adhering to these principles, you can ensure your applications are not only functional but also robust and reliable. Happy coding!