Common Pitfalls When Using Spring Data MongoDB
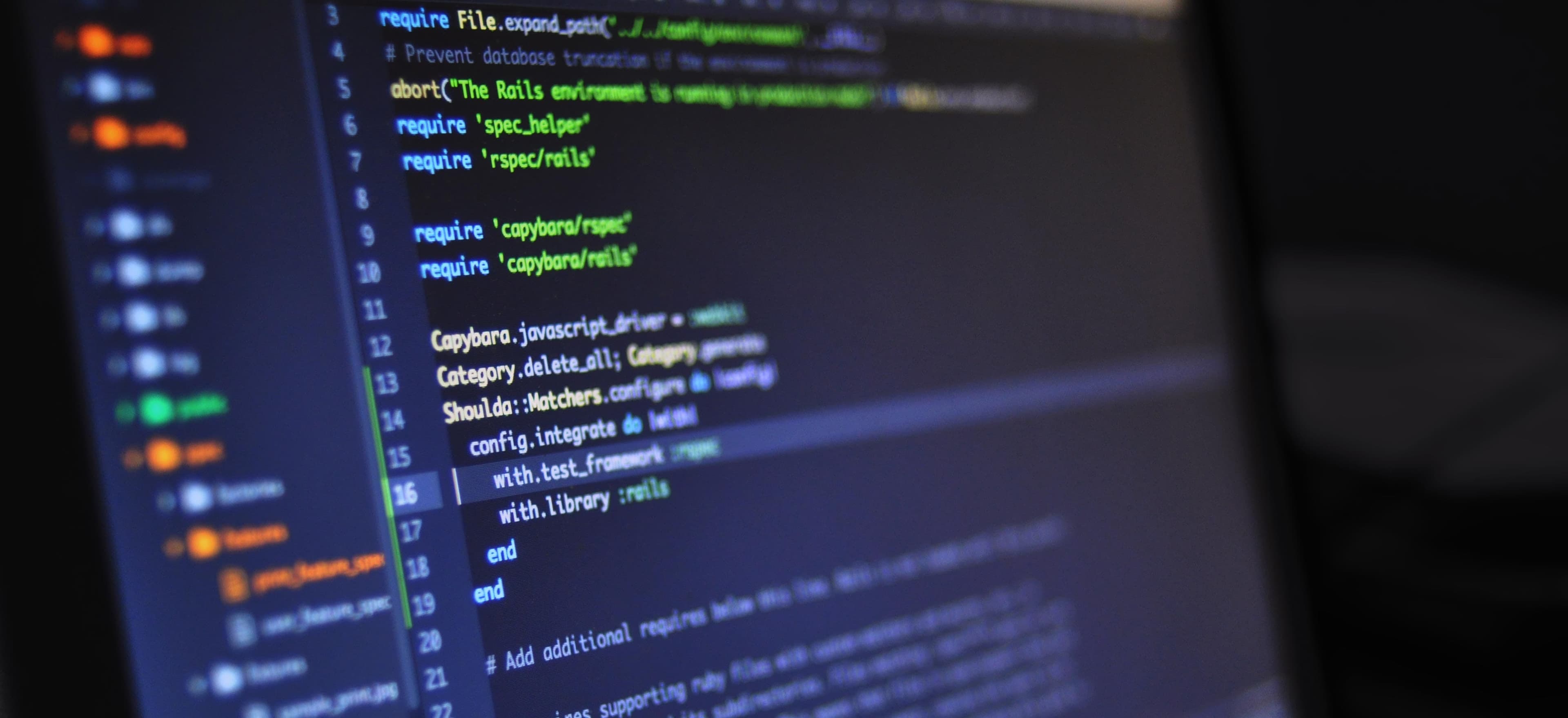
- Published on
Common Pitfalls When Using Spring Data MongoDB
When developing applications in Java, particularly with Spring, MongoDB is frequently chosen as the database solution. It provides a lot of flexibility and is designed to scale. However, like any technology, using Spring Data MongoDB isn’t without its challenges. In this post, we will explore some common pitfalls developers face when working with Spring Data MongoDB and how to avoid them.
Table of Contents
- Understanding Spring Data MongoDB
- Pitfall 1: Ignoring MongoDB's Document Structure
- Pitfall 2: Overusing the Repository Pattern
- Pitfall 3: Neglecting Error Handling
- Pitfall 4: Improper Indexing
- Pitfall 5: Failing to Optimize Queries
- Pitfall 6: Not Utilizing Projections
- Conclusion
Understanding Spring Data MongoDB
Spring Data MongoDB is part of the larger Spring Data project, which aims to provide a uniform approach to data access across various data stores. It simplifies the application development process and allows for leveraging the powerful query capabilities of MongoDB. By mapping Java objects to MongoDB documents, developers can focus on the application's business logic rather than database interactions.
Pitfall 1: Ignoring MongoDB's Document Structure
MongoDB is a NoSQL database that stores data in a flexible, JSON-like format called BSON. One common mistake developers make is neglecting the importance of document structure.
Consider the following Java class:
@Document(collection = "users")
public class User {
@Id
private String id;
private String name;
private List<String> hobbies;
}
Why This Matters:
A poorly thought-out structure could lead to inefficient queries and an inability to leverage MongoDB's strengths. For example, if you frequently need to perform queries based on hobbies, it might be beneficial to store hobbies as sub-documents to allow for easier retrieval.
Ensure your document structure aligns with how your application retrieves and manipulates data. Consider normalization vs. denormalization based on your access patterns.
Pitfall 2: Overusing the Repository Pattern
Spring Data provides the repository pattern to enable CRUD operations with minimal boilerplate code. However, there’s a tendency to overuse this pattern, leading to bloated repositories that become difficult to manage.
Example of a bloated repository:
@Repository
public interface UserRepository extends MongoRepository<User, String> {
List<User> findByName(String name);
List<User> findByHobbies(String hobby);
Optional<User> findById(String id);
// ... many more methods
}
Why This Matters:
Having too many methods in one repository can hinder maintainability. It can be beneficial to create specialized repositories depending on the complexity of business logic.
For complex queries, consider separating them into a service layer or utilizing custom queries with @Query
.
Pitfall 3: Neglecting Error Handling
In the pursuit of a smooth user experience, developers often overlook error handling when working with MongoDB. This can lead to unexpected application crashes and unhandled exceptions.
Example of error handling:
public User findUserById(String id) {
return userRepository.findById(id).orElseThrow(() ->
new UserNotFoundException("User not found with ID: " + id));
}
Why This Matters:
Proper error handling is essential in a production environment. Not only does it improve user experience, but it also aids in diagnosing issues when they arise.
Utilizing custom exceptions can provide better context for errors related to database operations.
Pitfall 4: Improper Indexing
MongoDB can efficiently handle large data sets, but without proper indexing, performance can degrade significantly. Common mistakes include not indexing frequently queried fields.
Basic indexing example:
@Document(collection = "users")
public class User {
@Id
private String id;
@Indexed
private String email;
}
Why This Matters:
Indexing helps speed up search queries and improves performance. Analyze your query patterns and create indexes accordingly. Use tools like db.collection.getIndexes()
in your MongoDB shell to audit existing indexes.
For more on indexing strategies, visit MongoDB Indexing Documentation.
Pitfall 5: Failing to Optimize Queries
Another common pitfall is to assume that all queries will perform well by default. MongoDB can handle various queries, but poorly structured queries can lead to performance issues.
Example of an unoptimized query:
List<User> users = userRepository.findAll();
List<User> filteredUsers = users.stream()
.filter(user -> user.getHobbies().contains("coding"))
.collect(Collectors.toList());
Why This Matters:
In this scenario, all users are fetched into memory before filtering. This can lead to inefficient use of memory and slow performance for large datasets. Instead, leverage MongoDB's querying capabilities to filter data at the database level.
Optimized query example:
List<User> users = userRepository.findByHobbies("coding");
Pitfall 6: Not Utilizing Projections
When working with large documents, it's important to retrieve only the data you need. Developers often forget to use projections, retrieving full documents even when only a subset of fields is necessary.
Example of a projection:
public interface UserSummary {
String getName();
List<String> getHobbies();
}
In your repository, you can create a method like this:
List<UserSummary> findUserSummariesByHobbies(String hobby);
Why This Matters:
By using projections, you can reduce the amount of data transferred and improve performance. This also minimizes the load on your applications and databases.
For further reading on projections, check out the Spring Data MongoDB Projections Documentation.
Final Considerations
Spring Data MongoDB is a powerful tool for developing applications, but it’s crucial to be aware of some common pitfalls that can hinder your project's success. From understanding proper document structure to ensuring error handling and optimizing queries, each aspect plays a pivotal role in effective MongoDB usage.
By being mindful of these pitfalls and utilizing best practices, you can fully leverage the capabilities of Spring Data MongoDB to create efficient, maintainable applications. Ensure you continuously learn and iterate on your database strategies as your application grows.
For more on best practices with Spring Data and MongoDB, you may find the following resources useful:
By avoiding these common pitfalls, you’ll not only save time but also enhance the overall performance and reliability of your applications. Happy coding!