Mastering Java 8 Streams: Common SQL Parsing Pitfalls
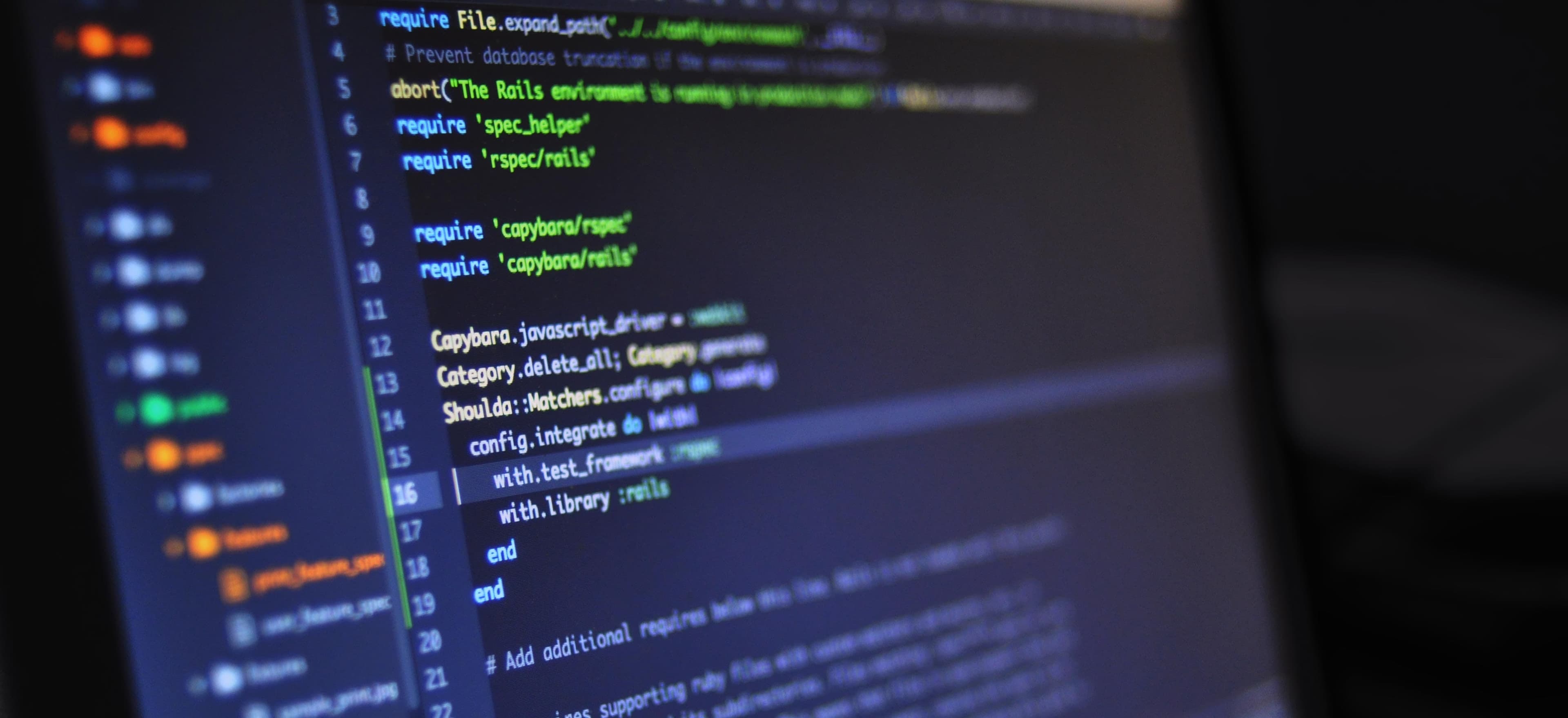
- Published on
Mastering Java 8 Streams: Common SQL Parsing Pitfalls
Java 8 introduced a powerful feature known as Streams, transforming the way we handle data in collections. While the integration of Streams allows for a more functional programming approach, parsing SQL queries with this new tool comes with its own set of pitfalls. In this blog post, we’ll delve into the intricacies of using Java 8 Streams in SQL parsing, highlighting key pitfalls and best practices along the way.
Understanding the Basics of Java 8 Streams
Before we dive into SQL parsing, let’s revise the concepts of Java 8 Streams. A Stream is a sequence of elements supporting sequential and parallel aggregate operations. Here’s a quick example demonstrating how to use Streams in Java to process a list of integers:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using Streams to filter even numbers and square them
List<Integer> squaredEvens = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.toList();
System.out.println(squaredEvens); // Output: [4, 16]
}
}
Explanation
- Creating a Stream: You start with a collection (like a list of integers) and call
stream()
to create a Stream. - Filtering Elements: The
.filter()
method allows you to specify a predicate that determines which elements to keep. - Transforming Elements: With
.map()
, you can transform the filtered elements. In this case, we squared the even numbers. - Collecting Results: Finally, we used
.toList()
to gather the results back into a List.
Common SQL Parsing Pitfalls with Java 8 Streams
While using Streams simplifies various operations, parsing SQL queries presents unique challenges. Below, we highlight some common pitfalls associated with this task.
1. Overcomplicating Query Parsing
One common mistake is adopting a complex approach when the SQL queries could be parsed more straightforwardly. Let's assume you have a SQL string and want to extract the values from the SELECT
statement:
public static void parseSelectQuery(String query) {
String[] parts = query.split(" ");
List<String> selectedColumns = Arrays.stream(parts)
.filter(part -> part.equalsIgnoreCase("SELECT") || part.equals(","))
.collect(Collectors.toList());
System.out.println(selectedColumns);
}
Why This is a Pitfall
While the above method provides a way of filtering parts of the SQL query, it's overly complicated. This can lead to maintainability issues, especially when handling more complex queries. Instead, consider using regular expressions for better readability and performance:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public static void parseSelectQuery(String query) {
String regex = "SELECT\\s+(.*)\\s+FROM";
Pattern pattern = Pattern.compile(regex, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(query);
if (matcher.find()) {
String columns = matcher.group(1);
List<String> selectedColumns = Arrays.asList(columns.split(",\\s*"));
System.out.println(selectedColumns);
}
}
2. Not Handling Different SQL Dialects
Another major pitfall lies in ignoring the fact that SQL can vary dramatically between databases. A one-size-fits-all parsing solution can introduce errors.
The Importance of Tailored Solutions
If you're parsing SQL for multiple databases (like MySQL, PostgreSQL, or Oracle), consider providing different parsing strategies based on the detected dialect. You might use tagged interfaces to define how different SQL types should be parsed.
3. Ignoring Exception Handling
Java Streams encourage a neat and functional style, but this can sometimes lead to neglecting proper exception handling. Consider parsing an SQL query that might fail:
import java.util.Optional;
public static List<String> safeParseQuery(String query) {
return Optional.of(query)
.filter(q -> q.startsWith("SELECT"))
.map(q -> Arrays.asList(q.split(" ")))
.orElse(Collections.emptyList());
}
Why Exception Handling is Key
Using Optional
protects against null values but doesn't replace robust error handling. To enhance this, always include exception logging or handling where necessary to avoid hidden bugs.
4. Performance Concerns with Large Queries
When working with massive SQL queries, it’s crucial to be aware of performance issues. Streams can create overhead if not used efficiently.
public static void parseAndOptimizeQuery(String query) {
// This can be slow for large queries if not handled properly
List<String> keywords = Arrays.asList("SELECT", "FROM", "WHERE", "JOIN");
Arrays.stream(query.split(" "))
.filter(keywords::contains)
.distinct()
.forEach(System.out::println);
}
How to Optimize
Instead of filtering through the entire query at once, consider breaking it down into components before processing. This might include:
- Tokenization: Break the query into manageable tokens based on SQL syntax.
- Caching: If certain queries are repeatedly parsed, consider caching parsed results.
5. Neglecting SQL Injection
A significant concern when parsing SQL queries with Java Streams is the security of your application. SQL injection attacks can be catastrophic. Here's a demonstration of a naive parsing approach that might expose your application to such vulnerabilities:
public void executeQuery(String userInput) {
String sql = "SELECT * FROM users WHERE username = '" + userInput + "'";
// Execute SQL without any input validation
}
Best Practices for Security
To mitigate SQL injection risks, always use Prepared Statements:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public void secureExecuteQuery(String username) {
String sql = "SELECT * FROM users WHERE username = ?";
try (Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement(sql)) {
stmt.setString(1, username);
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
// process results
}
} catch (SQLException e) {
e.printStackTrace();
}
}
Closing Remarks: Best Practices in SQL Parsing with Java Streams
- Keep It Simple: Utilize regular expressions for straightforward SQL parsing tasks instead of complex stream operations.
- Consider SQL Dialects: When parsing SQL, ensure your solution accommodates different database dialects.
- Handle Exceptions: Implement robust error handling and logging within your parsing methods.
- Optimize Performance: Be aware of performance implications when working with large SQL queries; prefer pre-processing solutions.
- Prioritize Security: Always sanitize user input and use prepared statements to mitigate risks associated with SQL injections.
In mastering Java 8 Streams for SQL parsing, keeping these pitfalls in check will lead to more maintainable, efficient, and secure applications. Happy coding!
For further reading, explore the Oracle Java Documentation for a deeper understanding of Streams or SQL Injection Defense techniques to enhance security in your applications.
Checkout our other articles