Troubleshooting Custom Login Failures in Spring MVC
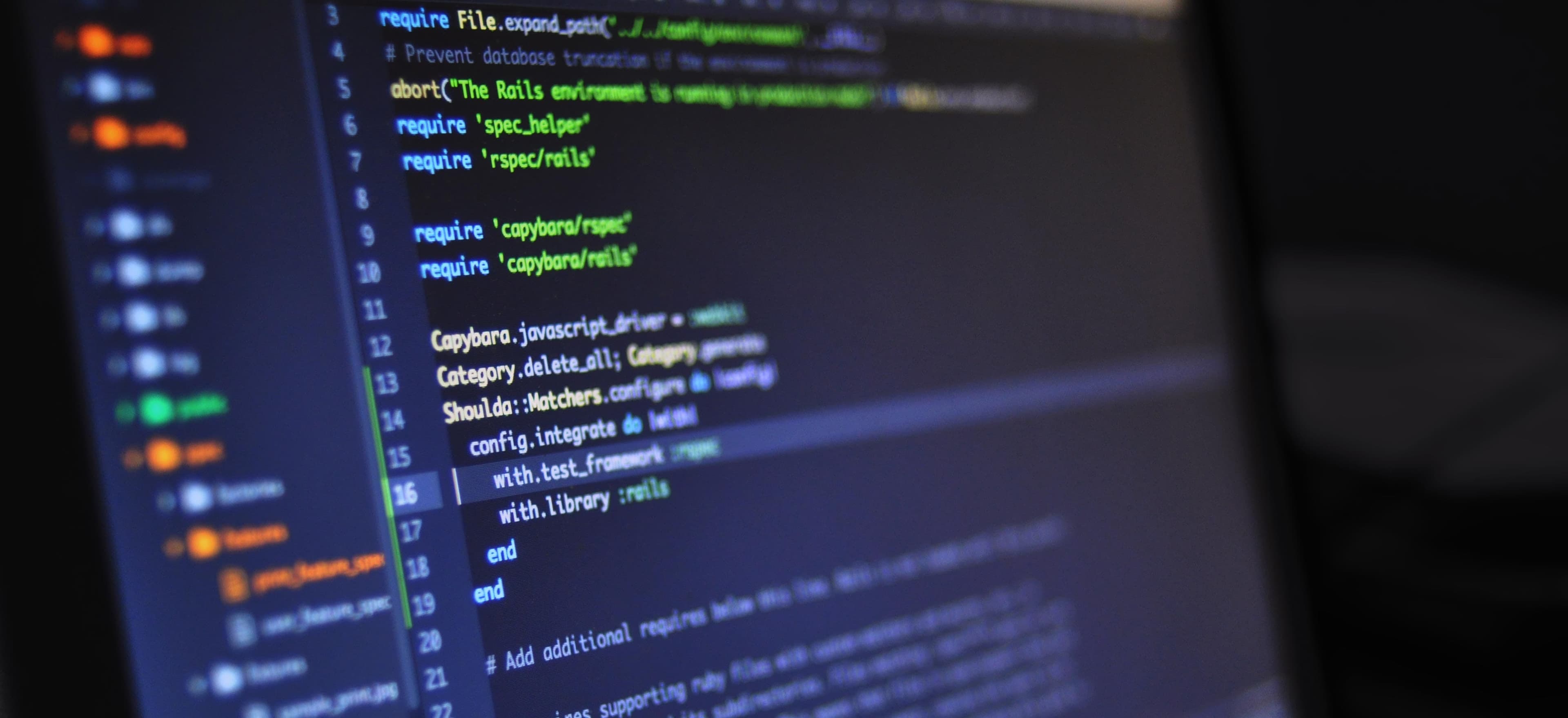
- Published on
Troubleshooting Custom Login Failures in Spring MVC
In today’s digital landscape, user authentication is an essential part of web application development. When working with Spring MVC, implementing a custom login mechanism can provide a smoother user experience. However, it’s not without its challenges. In this post, we will dive into the common issues developers face when building a custom login system in Spring MVC, along with effective troubleshooting strategies.
Table of Contents
- Understanding Spring Security Fundamentals
- Common Login Failures
- Detailed Troubleshooting Techniques
- Sample Code and Explanation
- Best Practices for Authentication
- Conclusion
Understanding Spring Security Fundamentals
Before we begin troubleshooting, it is crucial to have a foundational understanding of Spring Security. Spring Security provides comprehensive security services for Java EE-based enterprise software applications. It offers authentication and access control schemes for applications based on the Spring framework.
At its core, Spring Security allows you to specify how a user is authenticated and authorized, protecting your application’s API or web interface from unauthorized access.
For detailed documentation, refer to the Spring Security Reference.
Common Login Failures
When developing custom login functionality in Spring MVC, developers frequently encounter a few common issues:
-
Invalid Credentials: The most common issue arises when users enter incorrect credentials, leading to failed login attempts.
-
Incorrect URL Mapping: A misconfigured URL mapping may result in a login page that does not respond properly to user input.
-
Session Management Issues: Issues related to user sessions can prevent successful logins.
-
Null Pointer Exceptions: Many developers encounter unexpected null pointer exceptions when accessing user details.
-
Lack of Error Messaging: Users may be unaware of the reasons for failed login attempts, leading to frustration.
Detailed Troubleshooting Techniques
To resolve these common login failures, we can apply a few troubleshooting techniques.
1. Validate the User Credentials
Start by checking the user credentials stored in your database. This includes ensuring that the username and password hash match those provided during login.
2. Review URL Mappings
Ensure that your URL mappings are properly configured in your WebSecurityConfigurerAdapter
. Verify that the login page’s URL is accessible and set:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login", "/error").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.failureUrl("/login?error=true")
.permitAll();
}
}
3. Implement Custom Authentication Provider
You can create a custom authentication provider that handles user details and errors more efficiently. Here’s a simple implementation:
@Service
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Autowired
private UserDetailsService userDetailsService;
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = (String) authentication.getCredentials();
UserDetails user = userDetailsService.loadUserByUsername(username);
if (user == null || !user.getPassword().equals(password)) {
throw new BadCredentialsException("The provided credentials are invalid.");
}
return new UsernamePasswordAuthenticationToken(user, password, user.getAuthorities());
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
In this code snippet, we check the validity of a user’s credentials and provide a relevant error message if they are incorrect.
4. Enhance Error Handling
Implement better error handling on your login page to communicate issues more clearly. Consider adding a model
attribute to show error messages:
@GetMapping("/login")
public String login(Model model,
@RequestParam(value = "error", required = false) String error) {
if (error != null) {
model.addAttribute("errorMessage", "Invalid username or password.");
}
return "login";
}
This way, users can immediately see what went wrong.
5. Utilize Debugging Tools
Utilize logging frameworks like SLF4J or Log4J to log events during the authentication process. This can greatly aid in tracking down what’s going wrong.
private static final Logger logger = LoggerFactory.getLogger(CustomAuthenticationProvider.class);
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
// Log when a user tries to authenticate
logger.info("Authentication attempt for user: {}", authentication.getName());
// Original authentication logic...
}
Sample Code and Explanation
Let’s encapsulate our learning into a simple, effective setup for a custom login form in Spring MVC. Below is a complete setup illustrating user login with appropriate error messages:
Login Controller
@Controller
public class LoginController {
@GetMapping("/login")
public String login(Model model, @RequestParam(value = "error", required = false) String error) {
if (error != null) {
model.addAttribute("errorMessage", "Invalid username or password.");
}
return "login"; // Points to login.html
}
}
Login Form (login.html)
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form th:action="@{/login}" method="post">
<div>
<label for="username">Username</label>
<input type="text" id="username" name="username" required>
</div>
<div>
<label for="password">Password</label>
<input type="password" id="password" name="password" required>
</div>
<div>
<button type="submit">Login</button>
</div>
<div th:if="${errorMessage}" th:text="${errorMessage}" style="color:red;"></div>
</form>
</body>
</html>
This setup demonstrates how we can provide effective feedback to users through a login form.
Best Practices for Authentication
To ensure that your custom login functionality is robust, you should consider the following best practices:
-
Use Password Hashing: Never store passwords in plain text. Use libraries like BCrypt for password hashing.
-
Implement Throttle Mechanisms: To combat brute-force attacks, implement account lockout after several failed attempts.
-
Utilize HTTPS: Always use HTTPS to protect user credentials during transmission.
-
Regularly Update Dependencies: Regularly check for and apply updates to Spring and its components to protect against vulnerabilities.
-
Version Control: Maintain your code in repositories like Git for easier rollbacks and tracking changes.
The Last Word
Building a custom login mechanism in Spring MVC can be a rewarding challenge, but it is also one fraught with potential pitfalls. Following systematic troubleshooting methods, along with detailed and organized code practices, can significantly enhance your application's stability and user experience.
By understanding the underlying principles of Spring Security and employing the tools and techniques outlined above, you can create a resilient authentication process that keeps your users secure and informed.
For a deeper dive into Spring Security, check out the Spring Security Guides. Happy coding!
By employing the strategies discussed in this post, you should be better equipped to handle custom login failures in Spring MVC while maintaining clarity and professionalism in your approach.
Checkout our other articles