Troubleshooting Disabled Android Broadcast Receivers
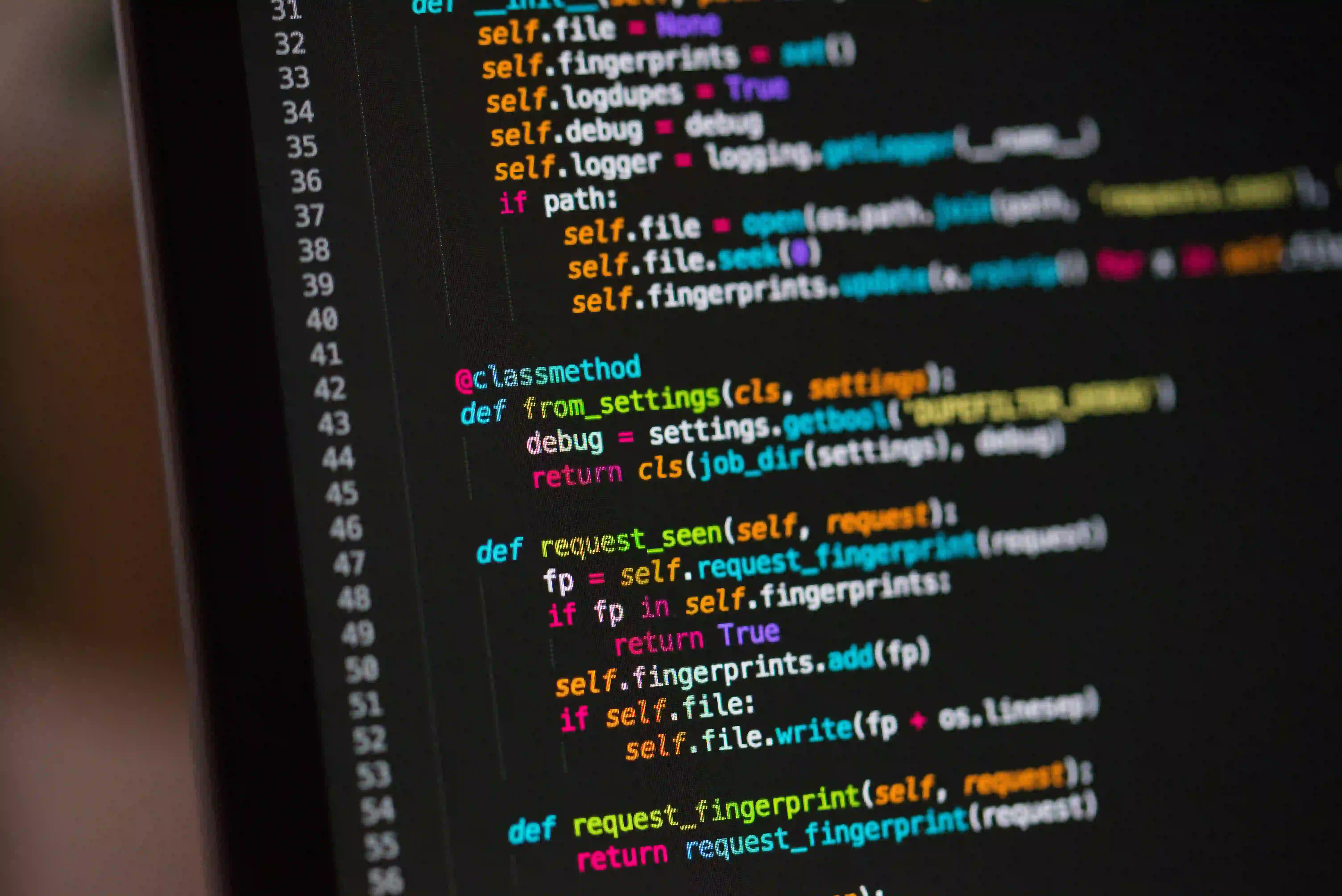
Troubleshooting Disabled Android Broadcast Receivers
Broadcast Receivers in Android play a crucial role in allowing your app to respond to system-wide broadcast announcements. For example, they can respond to events such as incoming calls, SMS messages, or even connectivity changes. However, there can be occasions when these receivers might become disabled, either due to user actions, system configurations, or application code. In this blog post, we will discuss how to effectively troubleshoot disabled Android Broadcast Receivers and ensure your application functions as intended.
What is a Broadcast Receiver?
Before diving into troubleshooting, let's briefly understand what a Broadcast Receiver is. In Android, a Broadcast Receiver is a component that listens for and responds to broadcast messages from other applications or from the Android system itself. This is particularly useful for responding to events and notifications.
A Simple Implementation Example
Here is a simple example of a Broadcast Receiver:
public class MyBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
// Handle the received broadcast message here
Log.d("MyBroadcastReceiver", "Broadcast received!");
}
}
In this basic example, when a broadcast is received, it logs a message to logcat. But if this receiver is disabled, this code will not trigger as expected.
Why Would a Broadcast Receiver Be Disabled?
There are several reasons why a Broadcast Receiver may become disabled:
- Manifest Declaration Issues: The receiver may not be correctly declared in your app’s manifest file.
- User Intervention: Users can disable receivers if they have access to settings that permit them to manage app behaviors.
- Programmatic Disabling: Your application code might intentionally disable the receiver.
- Battery Optimization: Android might restrict certain broadcasts due to battery-saving measures.
Understanding these reasons can help us troubleshoot effectively.
Troubleshooting Steps
Step 1: Check Manifest Declaration
Firstly, ensure that your Broadcast Receiver is declared in the AndroidManifest.xml file. This declaration is crucial for your Broadcast Receiver to operate effectively.
<receiver android:name=".MyBroadcastReceiver">
<intent-filter>
<action android:name="com.example.MY_ACTION" />
</intent-filter>
</receiver>
Make sure that:
- The
android:name
attribute accurately reflects the location of your class. - The
intent-filter
correctly specifies the action you want to listen for.
Step 2: Monitor User Settings
Check if the user has disabled the receiver via application settings. In Android, users can manage app permissions and settings. Users might disable background data or notifications leading to the Broadcast Receiver not firing. A user may find these settings under:
Settings > Apps > Your App > Notifications
Make sure to highlight to users why they need to enable the receiver for necessary functionalities.
Step 3: Verify Code Logic
Sometimes, your application logic might control whether a receiver is enabled or disabled. Here’s an example of how you might programmatically enable or disable a Broadcast Receiver:
if (isConditionMet) {
context.registerReceiver(myBroadcastReceiver, new IntentFilter("com.example.MY_ACTION"));
} else {
context.unregisterReceiver(myBroadcastReceiver);
}
In this code snippet, the receiver is only registered if a certain condition is met. If the condition is not met, the receiver will be unregistered. Ensure that this condition is correctly checked to prevent unintended disabling.
Step 4: Review Battery Optimization Settings
Modern Android versions put a significant emphasis on battery life. Sometimes apps are restricted from running in the background, negatively impacting Broadcast Receivers. You can check the battery optimization settings programmatically or guide your users to adjust these settings:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
Intent intent = new Intent();
String packageName = context.getPackageName();
PowerManager pm = (PowerManager) context.getSystemService(Context.POWER_SERVICE);
if (!pm.isIgnoringBatteryOptimizations(packageName)) {
// Logic to prompt user to change settings
}
}
The above code checks if battery optimization is ignored for your application. If not, you can guide users on how to disable it, which may help enable Broadcast Receivers again.
Testing and Debugging Your Broadcast Receiver
Use Logging
Always use Log
statements within your Broadcast Receiver to confirm whether the reception of the broadcast is taking place. If the logger never appears, it could indicate a disabled receiver.
Use Debugger
Using Android Studio's debugging tools allows you to set breakpoints and verify that your receiver is correctly registered and receiving broadcasts.
Simulate Broadcast
You can also simulate the broadcast to check if your receiver is functioning. Use the following code to send a broadcast for testing:
Intent intent = new Intent("com.example.MY_ACTION");
context.sendBroadcast(intent);
Monitor logcat to see if your receiver responds as expected.
Closing the Chapter
Understanding and troubleshooting disabled Broadcast Receivers in Android is essential for building responsive applications. Regularly verify the declaration in your manifest, check user settings, ensure sound application logic, and keep battery optimization in mind.
For more in-depth insights, consider exploring the Android Developers Guide on Broadcast Receivers for comprehensive documentation and best practices.
With these strategies and considerations in mind, you can confidently troubleshoot and enable Broadcast Receivers for optimal app functionality. Happy coding!