Mastering Custom Crash Reports in Crashlytics for Android
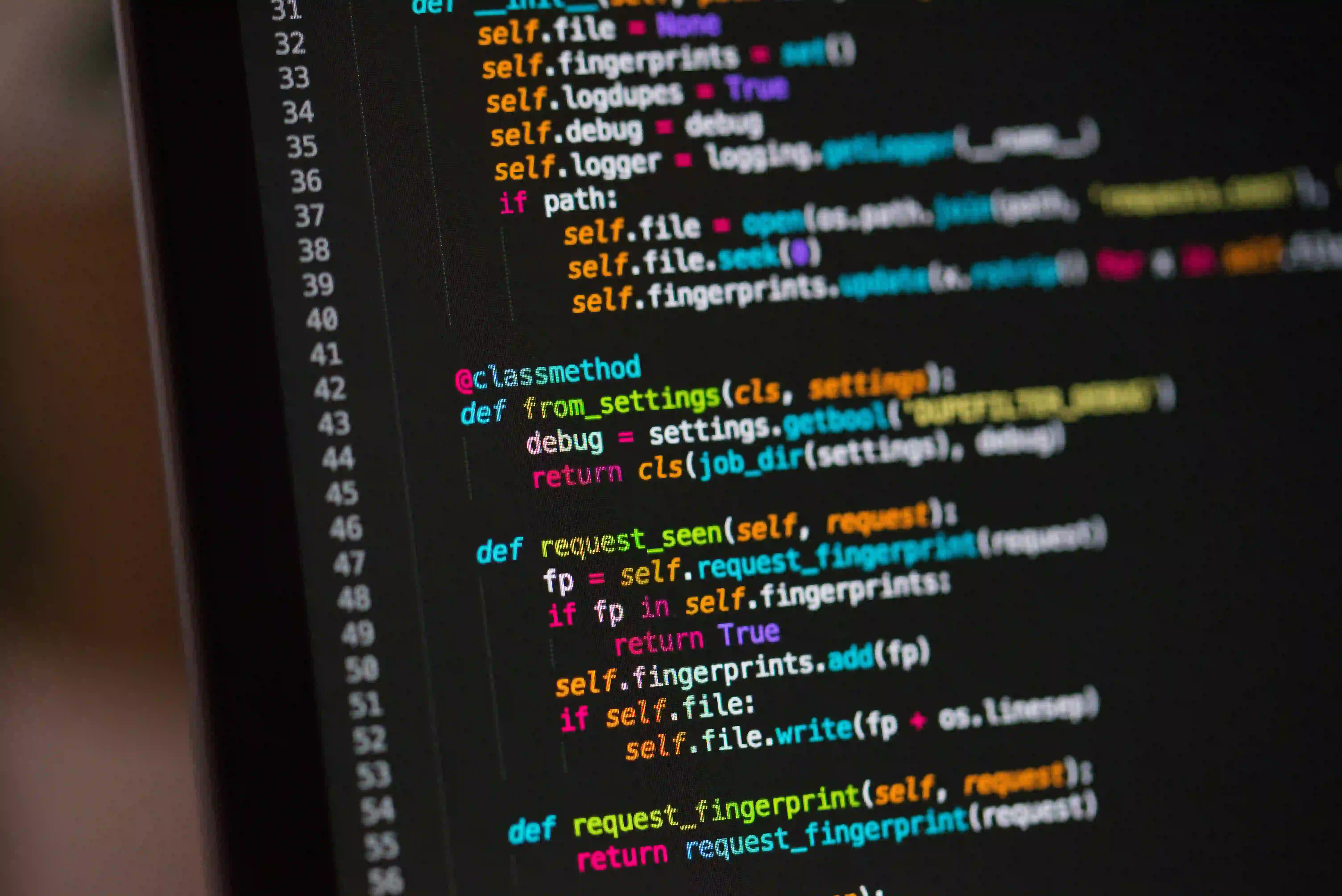
Mastering Custom Crash Reports in Crashlytics for Android
In the fast-evolving world of mobile development, ensuring a seamless user experience is paramount. This is where error reporting tools like Firebase Crashlytics come into play. Not only does it help you track crashes, but it also provides insights into the circumstances surrounding them. This blog post guides you through the essentials of implementing custom crash reports in Crashlytics for your Android applications.
Why Use Crashlytics?
Crashlytics is a powerful, real-time crash reporting tool from Firebase that helps developers diagnose issues within their apps. Here are a few compelling reasons to employ Crashlytics:
-
Real-time reporting: You receive immediate notifications about crashes, allowing for a quick response.
-
Detailed crash data: Crashlytics provides stack traces, logs, and device state details, enabling developers to pinpoint the source of the issue.
-
User engagement insights: Understanding how many users are affected by a crash helps prioritize fixes.
For a deeper dive into Firebase Crashlytics, check out the official Firebase Crashlytics documentation.
Getting Started
To integrate Crashlytics into your Android project, follow these steps:
-
Add dependencies: Include the Crashlytics SDK in your
build.gradle
file.📄snippet.txt// In your app/build.gradle dependencies { implementation 'com.google.firebase:firebase-crashlytics:latest_version' }
-
Initialize Crashlytics: In your
Application
class, initialize Crashlytics.☕snippet.javapublic class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); FirebaseApp.initializeApp(this); } }
-
Enable Crashlytics: Make sure you enable Crashlytics in your Firebase console.
Implementing Custom Crash Reports
Custom crash reports are essential for providing context to crashes, allowing developers to understand not only what went wrong but also the user’s journey leading to the issue. Here’s how to implement them.
Example 1: Logging User Identifiable Information
Suppose you want to log specific user data when a crash occurs. You can set user identifiers and custom keys. Storing relevant user data helps you understand who is affected by a crash.
public void logUserInformation(String userId) {
Crashlytics.getInstance().setUserId(userId);
}
Why This Matters: By setting the user ID, you can track user-specific crashes, thus allowing for targeted troubleshooting based on user behavior.
Example 2: Logging Custom Keys
You can enhance your crash reports by adding custom keys, which allows you to capture specific contextual information about your app's state when a crash occurs.
Here’s an example:
public void logCustomData(String key, String value) {
Crashlytics.getInstance().setString(key, value);
}
Implementation Example:
logCustomData("last_screen_viewed", "HomeFragment");
Why This Matters: In the event of a crash, you can quickly ascertain which screen the user was on before the crash, making it easier to reproduce and investigate the bug.
Example 3: Capturing Non-fatal Exceptions
Not all issues lead to a crash. Sometimes, you need to log non-fatal exceptions. This is particularly useful for catching errors that don't crash the app but may affect user experience.
public void logNonFatalError(Exception e) {
Crashlytics.getInstance().recordException(e);
}
You could add this in a catch block:
try {
// Code that may throw an exception
} catch (Exception e) {
logNonFatalError(e);
}
Why This Matters: Capturing non-fatal exceptions allows you to detect issues early on and address them before they become significant problems.
Example 4: Custom Logging
Sometimes, it’s useful to log arbitrary debug information. Crashlytics allows you to log messages that can be related to the state of your application before a crash.
public void logDebugMessage(String message) {
Crashlytics.getInstance().log(message);
}
Integration Example:
logDebugMessage("User clicked on submit button in the login form.");
Why This Matters: Debug messages help provide context on what the user was doing at the time of a crash, assisting in reproducing and diagnosing the issue.
Testing Crash Reporting
Once you have implemented custom crash reporting, it’s essential to test that it works as expected. Crashlytics provides a simple way to test crashes:
public void triggerCrash() {
throw new RuntimeException("Test Crash"); // Force a crash for testing
}
Simply call the triggerCrash()
method in your app at a suitable point for testing purposes. Ensure that you do this in a dev environment, as it will crash the app.
Monitoring Crash Reports
After setting up Crashlytics and logging custom events, you'll want to monitor the reports:
- Navigate to your Firebase Console.
- Select your project.
- Go to the Crashlytics section to see the crash reports, including the key data you logged.
Lessons Learned
Effectively utilizing Crashlytics can dramatically improve the reliability of your Android applications. Custom crash reports provide essential context for understanding and fixing issues. By logging user information, custom keys, non-fatal exceptions, and debug messages, you empower your development team to make data-driven decisions.
Feel free to explore more about Crashlytics by visiting the Firebase Crashlytics documentation or dive into the Firebase blog for more insights and best practices.
Finally, continuous monitoring and logging in development will help ensure that users receive the best possible experience from your application. Happy coding!