Why Black-Box Testing Can Fail in Spring Boot Microservices
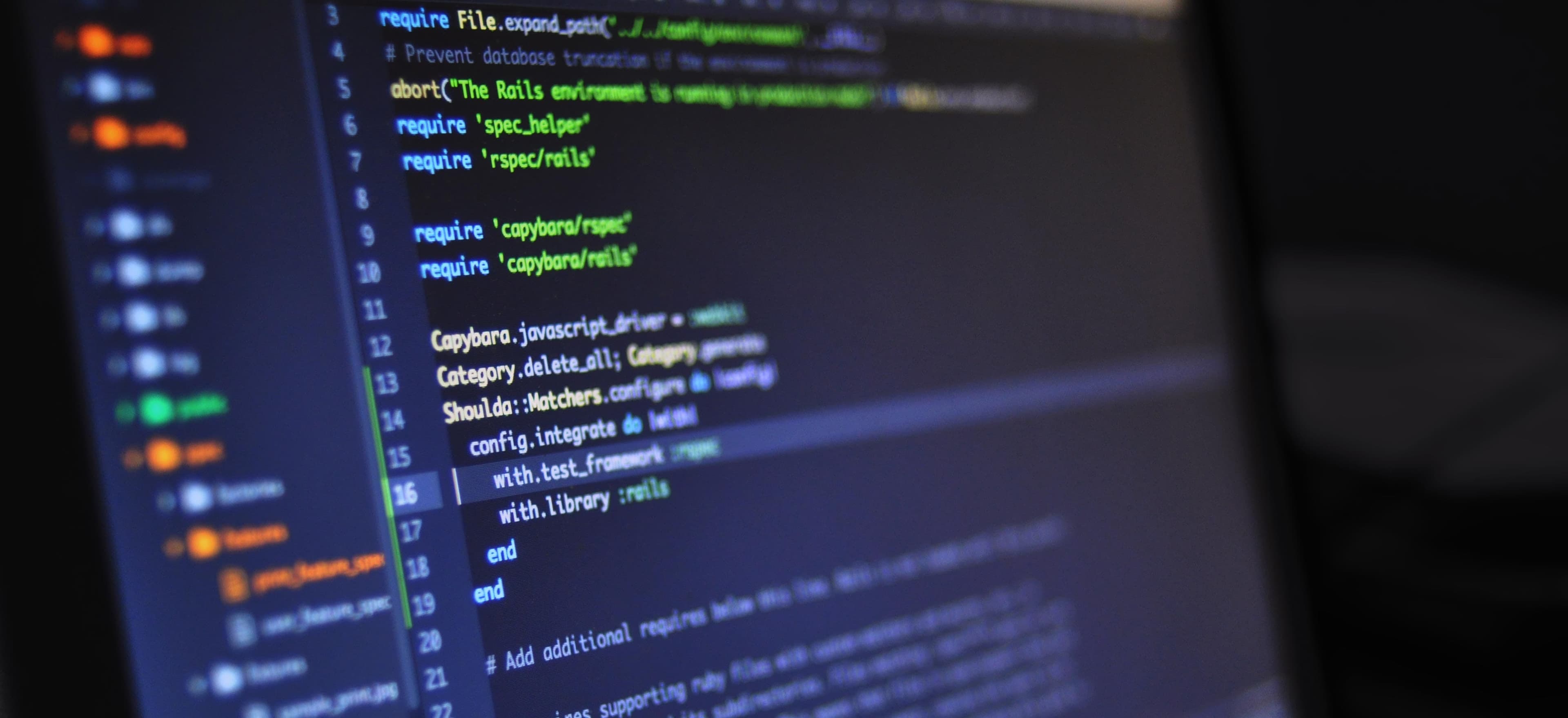
- Published on
Why Black-Box Testing Can Fail in Spring Boot Microservices
In the rapidly evolving world of software development, microservices architecture has become increasingly popular due to its scalability, flexibility, and resilience. Spring Boot has emerged as one of the go-to frameworks for building these microservices. However, as the complexities of these systems increase, ensuring quality through thorough testing becomes critical.
One common testing approach is black-box testing, which focuses on the outputs generated by a system in response to specific inputs. While effective for many applications, black-box testing has its limitations, particularly in the context of Spring Boot microservices. In this blog, we'll explore the reasons why black-box testing can fail in this environment, and we will also look at the best practices for ensuring effective testing in microservices.
Understanding Black-Box Testing
Definition and Approach
Black-box testing treats the system as a "black box" — testers are only concerned with what the application does, not how it does it. This means the internal workings of the application are hidden from the tester. The primary focus is on functional requirements implemented in the system, and it often involves test cases that validate input-output relations.
Key Characteristics of Black-Box Testing
- User-Centric: Focuses on user interactions instead of internal processes.
- Functional Testing: Primarily identifies whether the system meets its functional specifications.
- Independent Testing: Can be performed by individuals who are not involved in the code development.
The Challenges of Black-Box Testing in Spring Boot Microservices
While black-box testing has its strengths, it can fail to effectively assess Spring Boot microservices due to several factors:
1. Complex Interactions Between Services
Microservices are designed to be independent units that communicate with each other. When one service is dependent on another, black-box tests may not cover the complete end-to-end functionality. Here's an illustration:
Suppose we have a User Service
that communicates with an Order Service
to confirm a user's order. Testing the User Service
in isolation without proper context of the Order Service
will fail to validate that the entire transaction flow is functioning correctly.
2. Lack of Knowledge on Internal States
Knowing the internal state of the application can be crucial in debugging issues. Black-box testing does not provide insights into how internal data structures and states change through the life cycle of the application.
For example, a microservice that keeps a cache of users might display accurate user data in responses. However, if the cache is not updated due to a backend issue, it could lead to incorrect responses that aren’t caught in black-box tests.
3. Limited Error Condition Testing
Black-box tests often focus on "happy path" scenarios, which means they might neglect edge cases and error handling conditions. In microservices, a failure in one microservice can have cascading effects across others, making it crucial to evaluate how services handle exceptional situations.
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public User getUser(@PathVariable String id) {
return userService.findById(id)
.orElseThrow(() -> new UserNotFoundException("User not found"));
}
}
In the code above, we are throwing an exception if the user is not found. Black-box tests that only focus on valid user retrieval will miss this critical error condition.
4. Asynchronous Communication
Many microservices communicate asynchronously via message brokers. Black-box testing may not account for message delays, lost messages, or the order of message processing, which are vital for maintaining the integrity of the application.
5. Non-Functional Requirements and Performance
Black-box testing often overlooks non-functional aspects such as performance, scalability, and reliability. Microservices must not only function correctly but also meet performance goals and handle load effectively. Failing to test these parameters can result in a failed deployment in a production environment.
Best Practices for Testing Spring Boot Microservices
To overcome the challenges of black-box testing, developers should consider the following best practices:
1. Adopt a Comprehensive Testing Strategy
Utilize a mix of testing methodologies such as unit testing, integration testing, and end-to-end testing alongside black-box testing. Tools like JUnit and Mockito can be used for unit tests to isolate components:
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTest {
@MockBean
private UserRepository userRepository;
@Autowired
private UserService userService;
@Test
public void testFindUserById() {
User user = new User("1", "John Doe");
when(userRepository.findById("1")).thenReturn(Optional.of(user));
User result = userService.findById("1").orElse(null);
assertThat(result.getName()).isEqualTo("John Doe");
}
}
This code demonstrates the importance of testing individual components for specific functionality.
2. Service Contracts and API Documentation
Implement service contracts using tools like Swagger or Spring REST Docs. Document APIs clearly so that both testers and developers understand how services are expected to interact.
3. Focus on Integration Tests
Integration testing is essential to ensure that various services work well together. Use tools like Spring Test and Postman to create integration tests that validate interactions between services.
4. Simulate Failures
Employ chaos engineering principles to test how your microservices react to failures. This can be done with tools like Chaos Monkey.
5. Load and Performance Testing
Leverage testing tools like JMeter or Gatling to conduct load and performance tests to validate the non-functional requirements of your microservices.
Closing the Chapter
Black-box testing has its merits, but relying solely on this approach in a microservices architecture can lead to shortcomings, as we've explored. By understanding the complexities of microservices and adopting a more comprehensive testing strategy, you can significantly improve the reliability, performance, and robustness of your Spring Boot applications.
Getting testing right is not just about finding defects; it's about ensuring that your microservices can perform seamlessly in the production environment. So diversify your testing strategies and ensure thorough validation to deliver quality applications.
For more information on testing strategies suitable for microservices, consider reading about Microservices Testing Strategies.
By implementing these practices, your Spring Boot microservices can better withstand the unpredictability and challenges of real-world usage, leading to happier users and fewer headaches down the line.
Checkout our other articles