Common Pitfalls in Java EE 6 Testing You Should Avoid
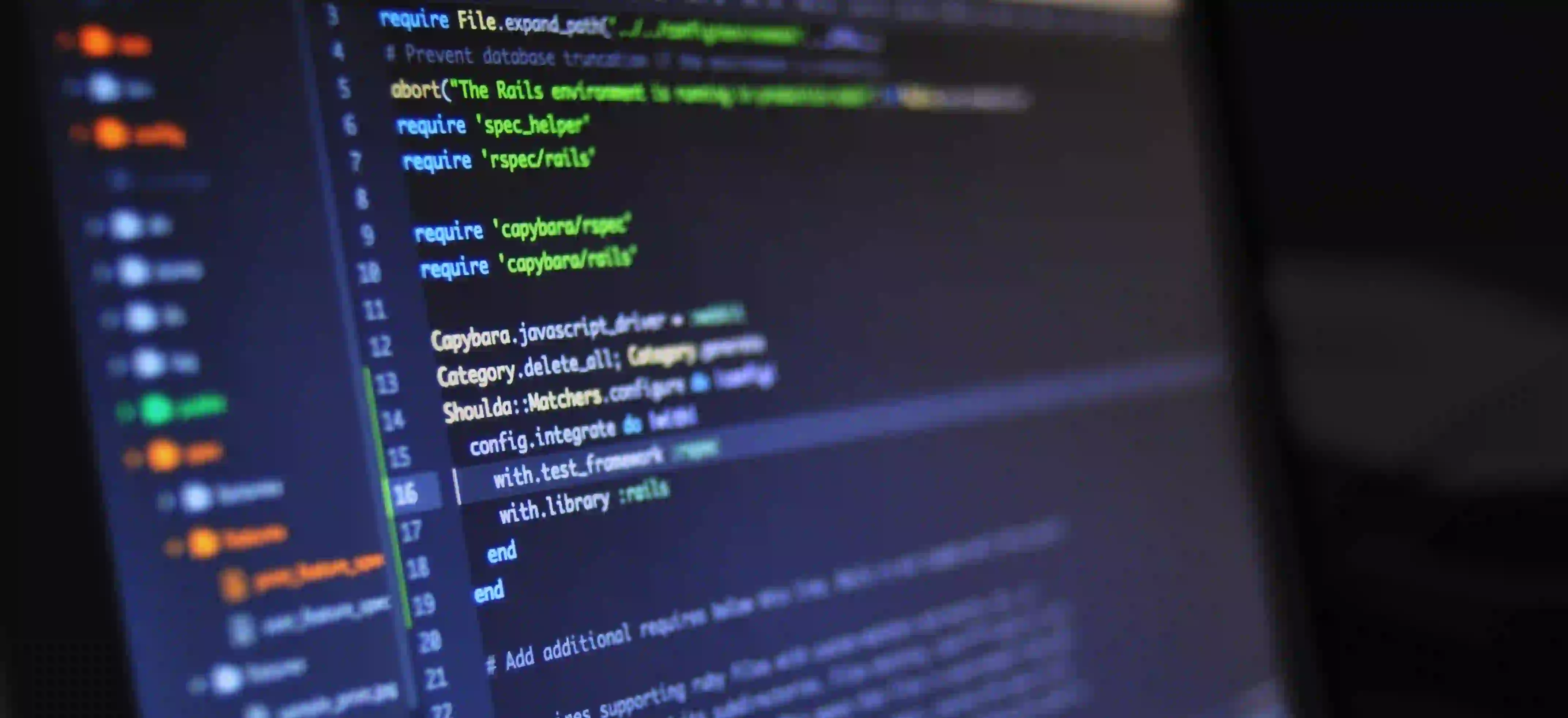
Common Pitfalls in Java EE 6 Testing You Should Avoid
Java Enterprise Edition (Java EE) 6 offers a powerful platform for developing robust, scalable applications. However, with great power comes great responsibility, especially when it comes to testing those applications. Effective testing ensures your application runs smoothly across different environments and handles edge cases gracefully. In this post, we'll explore the common pitfalls in Java EE 6 testing and provide insights on how to avoid them.
Understanding Java EE 6 Testing
Testing in Java EE 6 is unique due to its multi-tier architecture. It involves multiple components such as Servlets, EJBs (Enterprise JavaBeans), and JSF (JavaServer Faces). Each layer has its distinctive features, requiring specialized testing methods.
Testing Types
- Unit Testing: Tests individual components in isolation.
- Integration Testing: Tests the interaction between multiple components.
- Functional Testing: Validates the application against business requirements.
Common Pitfalls in Java EE 6 Testing
1. Ignoring the Importance of Mocking
Pitfall: Many developers tend to avoid mocking dependencies, leading to tests that don't accurately check component interaction.
Solution: Use mocking frameworks like Mockito or EasyMock to simulate components where direct interaction isn’t practical. Mocking allows you to isolate the unit of work and ensures that you are testing its behavior without interference from external factors.
import static org.mockito.Mockito.*;
public class UserServiceTest {
private UserService userService;
private UserRepository userRepository;
@Before
public void setUp() {
userRepository = mock(UserRepository.class);
userService = new UserService(userRepository);
}
@Test
public void testUserCreation() {
User newUser = new User("John Doe");
when(userRepository.save(any(User.class))).thenReturn(newUser);
User createdUser = userService.createUser(newUser);
assertEquals("John Doe", createdUser.getName());
verify(userRepository).save(newUser);
}
}
Commentary: Here, we mock the UserRepository
, allowing us to focus purely on the logic within UserService
. By doing so, the test remains isolated and does not depend on the underlying database or repository implementation.
2. Overlooking Dependency Injection Testing
Pitfall: Not testing classes in the context of a dependency injection container can lead to incomplete tests of your application’s behavior.
Solution: Utilize a test suite designed to support dependency injection, like Spring's testing context or Arquillian for Java EE. These frameworks help manage lifecycle and configuration complexities.
@RunWith(Arquillian.class)
public class SomeServiceTest {
@Inject
private SomeService someService;
@Test
public void testBusinessLogic() {
// test someService logic here
}
@Deployment
public static Archive<?> createDeployment() {
return ShrinkWrap.create(WebArchive.class)
.addClass(SomeService.class)
.addPackage("com.example"); // Add necessary packages
}
}
Commentary: In this scenario, Arquillian manages the deployment of the application and injects the SomeService
instance. This approach provides a more realistic environment for your tests by maintaining the application context and dependencies.
3. Not Utilizing Persistence Contexts Properly
Pitfall: Failing to manage persistence contexts can lead to data inconsistency and unexpected exceptions.
Solution: Always include @Transactional
annotations in testing scenarios that involve database interactions. This ensures transactions are handled correctly, maintaining data integrity.
@Transactional
public void testDatabaseInteraction() {
User user = new User("Jane Doe");
userRepository.save(user);
// assert database state here
}
Commentary: Using @Transactional
annotation handles the transaction lifecycle, rolling it back after the test, thereby preventing side effects on the actual database.
4. Ignoring Asynchronous Operations
Pitfall: If your application utilizes asynchronous operations (via EJB timers or messages), your tests might not accurately reflect the application behavior.
Solution: Use synchronization mechanisms like countdown latches to wait for async tasks to complete within your tests.
@Test
public void testAsynchronousProcess() throws InterruptedException {
CountDownLatch latch = new CountDownLatch(1);
someAsyncService.processAsync(latch);
latch.await(); // Wait for async process to finish
// assert based on expected outcomes from async processing
}
Commentary: The countdown latch accommodates the asynchronous nature of the processAsync
method, ensuring that assertions happen only after the async operation completes.
5. Lack of Integration Tests
Pitfall: Relying heavily on unit tests while neglecting integration tests can hide serious issues related to component interactions.
Solution: Make integration tests an integral part of your testing strategy by deploying your components together and ensuring they interact as expected.
@RunWith(Arquillian.class)
public class IntegrationTest {
@Inject
private CombinedService combinedService;
@Test
public void testCompleteFlow() {
// Trigger full flow that interacts with multiple components
combinedService.triggerFullProcess();
// assert state after full flow
}
}
Commentary: This integration test checks the entire flow of your application, validating that all components work together harmoniously.
6. Forgetting About Performance Testing
Pitfall: Performance is crucial, but developers often overlook it during the testing phase, leading to bottlenecks in production.
Solution: Incorporate performance testing tools like JMeter or Gatling alongside your regular test suite.
# Sample JMeter command to run a test
jmeter -n -t your_test_file.jmx -l results.jtl
Commentary: By executing JMeter tests (or using other performance testing tools), we can simulate multiple users handling various scenarios to understand how the application behaves under load.
7. Not Automating Your Tests
Pitfall: Manual testing is time-consuming and error-prone.
Solution: Set up Continuous Integration (CI) pipelines using tools like Jenkins or GitHub Actions to automatically run your tests every time changes are made.
# Example GitHub Actions YAML for Java EE testing
name: Java CI
on:
push:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Setup JDK
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Run Tests
run: mvn test
Commentary: Automating the testing process not only saves time but also ensures that every code change is subjected to testing rigorously before merging, thus catching issues early.
My Closing Thoughts on the Matter
In summary, Java EE 6 testing presents unique challenges, but by actively avoiding these common pitfalls, you can ensure a robust and reliable application. Remember to mock dependencies, utilize proper testing frameworks, manage persistence contexts, appropriately handle async processes, conduct integration tests, include performance testing, and automate your processes.
By systematically addressing these areas, you improve not only your testing practice but also the overall quality of your applications. Happy coding and testing!
For further reading, check out Java EE 6 - A Tutorial and explore Java EE testing frameworks like Arquillian for effective integration testing.
This blog post is designed to enhance your understanding of common pitfalls in Java EE 6 testing while providing strategies to address them effectively. Engaging with these concepts will empower you to build high-quality enterprise applications with greater confidence.