Common Pitfalls in Building Spring MVC REST Applications
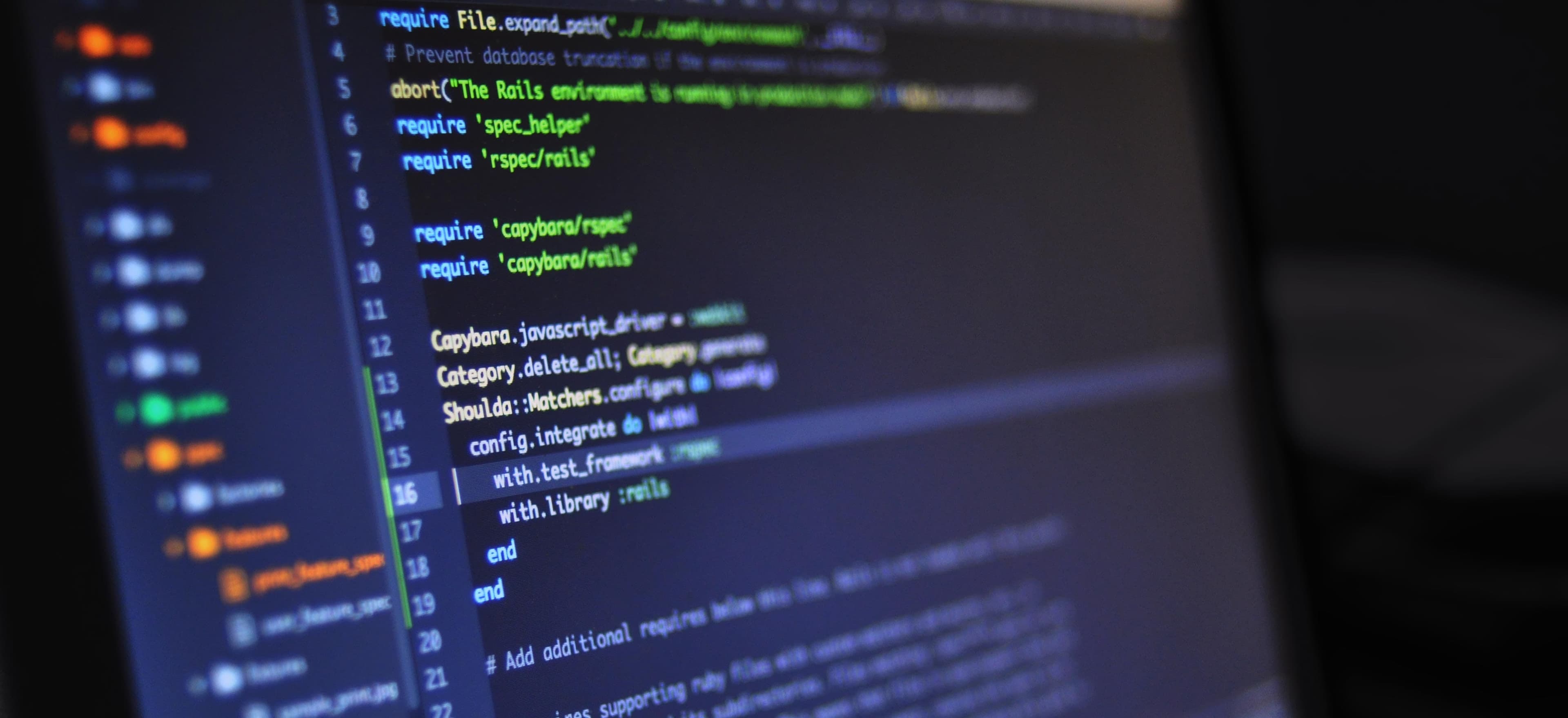
- Published on
Common Pitfalls in Building Spring MVC REST Applications
Building RESTful services with Spring MVC can be an immensely rewarding experience. However, it is not without its challenges. Developers, whether seasoned or novice, often fall prey to common pitfalls that can lead to inefficient, non-scalable, or poorly designed applications. In this blog post, we'll explore these pitfalls and offer solutions to avoid them, ensuring that your Spring MVC REST application is robust, efficient, and easy to maintain.
Understanding REST Architecture
Before diving into the pitfalls, let’s briefly cover the principles of REST (Representational State Transfer). REST is an architectural style characterized by stateless communication and operates over HTTP. The typical operations are:
- GET: Retrieve a resource
- POST: Create a new resource
- PUT: Update an existing resource
- DELETE: Remove a resource
Adhering to these principles is vital in creating a coherent and scalable API.
Common Pitfalls
1. Ignoring Status Codes
HTTP status codes are essential for conveying the outcome of client requests. Many developers forget to use them correctly, leading to confusion.
For instance, returning a 200 OK status for a delete operation can mislead clients into assuming the resource still exists.
Correct Usage
Here’s how you can use status codes effectively:
@DeleteMapping("/users/{id}")
public ResponseEntity<Void> deleteUser(@PathVariable Long id) {
boolean isDeleted = userService.deleteUser(id);
if (isDeleted) {
return ResponseEntity.noContent().build(); // 204 No Content
} else {
return ResponseEntity.notFound().build(); // 404 Not Found
}
}
In this example, a 204 No Content status indicates successful deletion, while 404 Not Found informs the client that the resource to delete was not present.
2. Lack of Input Validation
Failing to validate incoming data can lead to unexpected errors and, in worse cases, security vulnerabilities.
Using Spring's built-in validation features can save you from these headaches.
Implementing Validation
@PostMapping("/users")
public ResponseEntity<User> createUser(@Valid @RequestBody User user) {
User createdUser = userService.createUser(user);
return ResponseEntity.status(HttpStatus.CREATED).body(createdUser);
}
In the above code, the @Valid
annotation checks the user data against defined constraints in the User
class. If the validation fails, Spring automatically returns a 400 Bad Request response.
3. Overcomplicating Endpoints
Creating overly complex or nested endpoints can lead to a confusing API. Avoid deep resource hierarchies and keep your endpoints simple.
Example of Concise Endpoint Design
Instead of a complex URI like /departments/{deptId}/employees/{empId}
, consider simpler endpoints:
/employees
/departments/{deptId}/employees
4. Not Using HATEOAS
HATEOAS (Hypermedia as the Engine of Application State) is a key constraint of REST. Many neglect it, leading to clients that struggle to navigate APIs efficiently.
Implementation Example
Here’s how to implement HATEOAS:
@GetMapping("/users/{id}")
public ResponseEntity<EntityModel<User>> getUserById(@PathVariable Long id) {
User user = userService.findById(id);
EntityModel<User> resource = EntityModel.of(user);
resource.add(linkTo(methodOn(UserController.class).getUserById(id)).withSelfRel());
return ResponseEntity.ok(resource);
}
In this snippet, we return an EntityModel
that contains hyperlinks related to the user. This guides clients in navigating related resources.
5. Poor Error Handling
Failure to handle errors systematically can lead to problematic user experiences. Ideally, your application should return clear error messages along with relevant HTTP status codes.
Custom Error Response Example
You may want to define a consistent error response format:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<ErrorResponse> handleUserNotFoundException(UserNotFoundException ex) {
ErrorResponse error = new ErrorResponse("User not found", ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
}
In doing so, you provide an organized way to manage exceptions and communicate clearly with the client.
6. Not Securing Your API
Securing APIs should not be an afterthought. In the age of increasing cyber threats, implementing security best practices is crucial.
Consider using Spring Security for authentication and authorization.
Basic Security Configuration
A basic configuration might look like this:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
This restricts access to certain endpoints while allowing public resources. Always consider using HTTPS to protect data in transit.
7. Not Utilizing Caching
Caching is vital for performance. Failing to implement caching may lead to performance bottlenecks, especially under high load.
Implementing Caching with Spring
Here's a simple caching example:
@Cacheable("users")
@GetMapping("/users/{id}")
public User getUserById(@PathVariable Long id) {
return userService.findById(id);
}
By annotating the method with @Cacheable
, the result is stored in cache. Repeated calls with the same ID will return the cached result, improving performance.
8. Neglecting API Versioning
APIs evolve over time. Neglecting to implement versioning can lead to breaking changes affecting clients.
Versioning Strategies
You can version your API in several ways, such as through the URL:
@GetMapping("/v1/users/{id}")
public User getUserByIdV1(@PathVariable Long id) {
return userService.findById(id);
}
@GetMapping("/v2/users/{id}")
public User getUserByIdV2(@PathVariable Long id) {
// Updated implementation
}
By managing versions this way, you can ensure backward compatibility for older clients while introducing new functionalities.
Final Thoughts
Developing a Spring MVC REST application comes with its unique set of challenges. By avoiding these common pitfalls, you will enhance your API's usability, maintainability, and performance. Always remember the principles of REST, utilize Spring's powerful features, and most importantly, keep your API user-focused.
For further reading, you may find these resources helpful:
Implement these practices into your development workflow and watch as your applications become more reliable and user-friendly. Happy coding!
Checkout our other articles