Streamline Your Java 7 File Copying and Moving Techniques
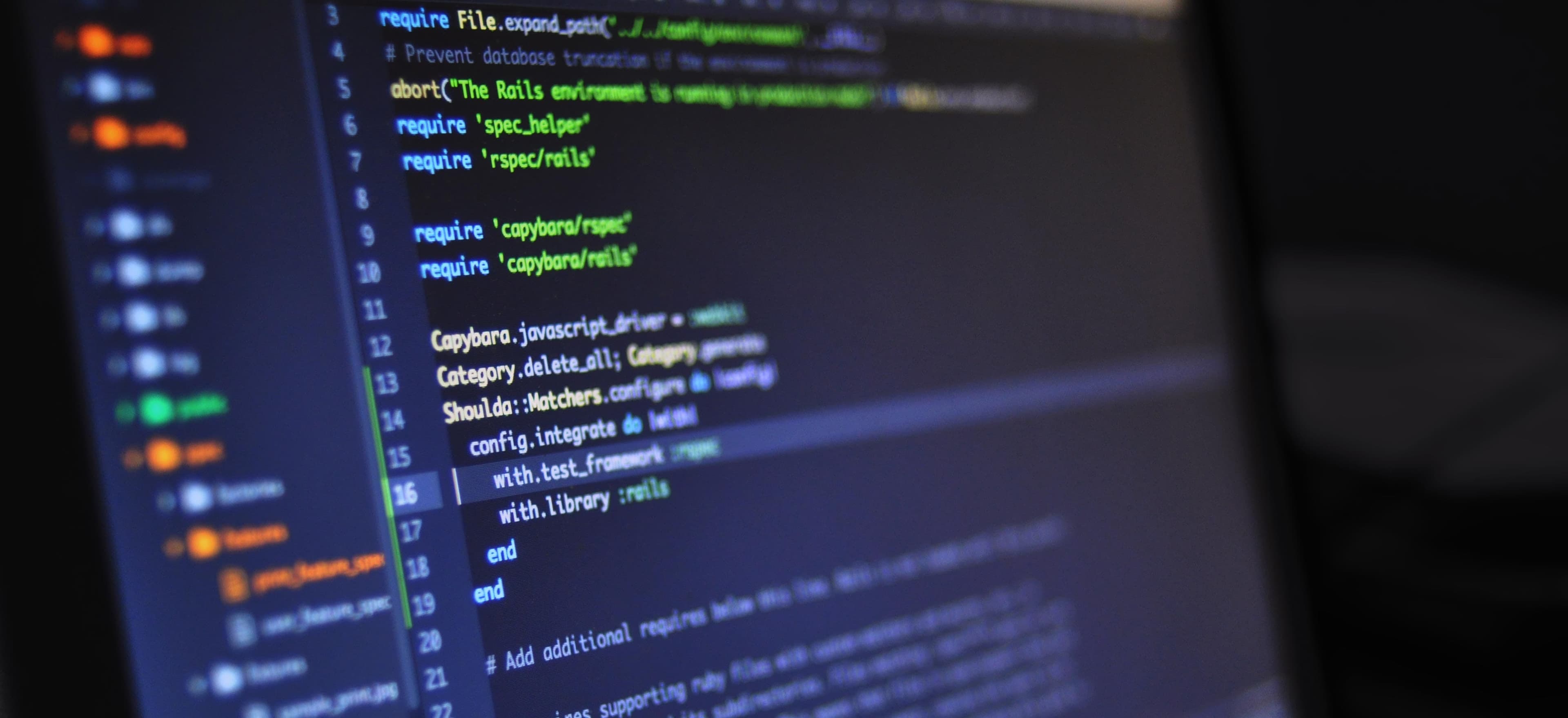
- Published on
Streamline Your Java 7 File Copying and Moving Techniques
Java 7 introduced significant enhancements to file handling with the addition of the NIO.2 (New Input/Output) package. These advancements make it easier and more efficient to copy and move files compared to previous methods. In this blog post, we will explore some of the most efficient ways to copy and move files in Java 7. We will also include code snippets, explanations, and useful tips to streamline your file handling techniques.
Understanding NIO.2
Java 7’s NIO.2 introduced the java.nio.file
package, which provides a unified filesystem API designed for file and directory manipulation. The key components of NIO.2 are:
- Paths: This class represents the path to a file or directory.
- Files: This class offers static methods to operate on files and directories.
- FileSystem: This interface represents the file system and allows for various file operations.
These components make file operations much clearer and manageable compared to the older I/O APIs in Java.
Copying Files in Java 7
To copy files in Java, you will use the Files.copy()
method from the java.nio.file.Files
class. This method allows you to copy files in a simple and effective way. Let’s dive into how to implement it.
Basic File Copy
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.io.IOException;
public class FileCopyExample {
public static void main(String[] args) {
Path source = Paths.get("src/source.txt");
Path destination = Paths.get("dest/destination.txt");
try {
// Copy the source file to the destination with REPLACE_EXISTING option
Files.copy(source, destination, StandardCopyOption.REPLACE_EXISTING);
System.out.println("File copied successfully!");
} catch (IOException e) {
System.err.println("Error occurred while copying the file: " + e.getMessage());
}
}
}
Code Explanation
- Paths: The
Paths.get()
method converts a file path from a string into aPath
object. - Files.copy(): This method copies the file from the source to the destination. The
StandardCopyOption.REPLACE_EXISTING
argument ensures that if the destination file exists, it will be replaced. - Exception Handling: Always encapsulate file operations with try-catch blocks to handle potential
IOExceptions
.
Advanced Copy Strategy
You can also use the Files.copy()
method for copying directories. However, it only works with the files and directory structures of one layer deep. For a deeper copy or to maintain the structure of a directory, you will need to implement a recursive method.
import java.nio.file.*;
import java.io.IOException;
public class DirectoryCopyExample {
public static void main(String[] args) {
Path sourceDir = Paths.get("src");
Path destinationDir = Paths.get("dest");
try {
copyDirectory(sourceDir, destinationDir);
} catch (IOException e) {
System.err.println("Error occurred while copying the directory: " + e.getMessage());
}
}
private static void copyDirectory(Path sourceDir, Path destinationDir) throws IOException {
Files.walk(sourceDir).forEach(sourcePath -> {
Path destPath = destinationDir.resolve(sourceDir.relativize(sourcePath));
try {
Files.copy(sourcePath, destPath, StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
System.err.println("Error copying file: " + e.getMessage());
}
});
}
}
Why Use the Above Code?
- Files.walk(): This method returns a Stream that allows you to recursively visit each file and directory in the source structure.
- Resolving Paths: The use of
resolve()
method helps in creating the correct destination path by maintaining the directory structure.
Moving Files in Java 7
Moving files is as simple as copying them and then deleting the original. However, with Java 7, the Files.move()
method streamlines the process.
Basic File Move
import java.nio.file.*;
public class FileMoveExample {
public static void main(String[] args) {
Path source = Paths.get("src/source.txt");
Path destination = Paths.get("dest/destination.txt");
try {
Files.move(source, destination, StandardCopyOption.REPLACE_EXISTING);
System.out.println("File moved successfully!");
} catch (IOException e) {
System.err.println("Error occurred while moving the file: " + e.getMessage());
}
}
}
Code Explanation
- Files.move(): Just like
Files.copy()
, it moves the source file to the destination path. The optionStandardCopyOption.REPLACE_EXISTING
is again used to handle already existing files.
Moving Directories
Moving directories uses a similar approach to copying them. You can use Files.move()
to transfer an entire directory.
import java.nio.file.*;
public class DirectoryMoveExample {
public static void main(String[] args) {
Path sourceDir = Paths.get("src");
Path destinationDir = Paths.get("dest");
try {
Files.move(sourceDir, destinationDir, StandardCopyOption.REPLACE_EXISTING);
System.out.println("Directory moved successfully!");
} catch (IOException e) {
System.err.println("Error occurred while moving the directory: " + e.getMessage());
}
}
}
Why This Matters?
Moving files and directories avoids extra disk I/O operations, as they change the reference rather than copying the content. Thus, it’s more efficient in both time and space.
To Wrap Things Up
Java 7 has significantly simplified file handling through the introduction of the NIO.2 package. With just a few lines of code, you can easily copy and move files and directories while maintaining best practices with proper error handling.
For more advanced file manipulation scenarios or to understand the comprehensive filesystem operations, consider exploring the official Java documentation.
With these streamlined techniques, ensure that you adopt NIO.2 in your Java applications, improving both performance and code readability. Happy coding!
Checkout our other articles