Constructor vs. Method Injection: Choosing the Right Approach
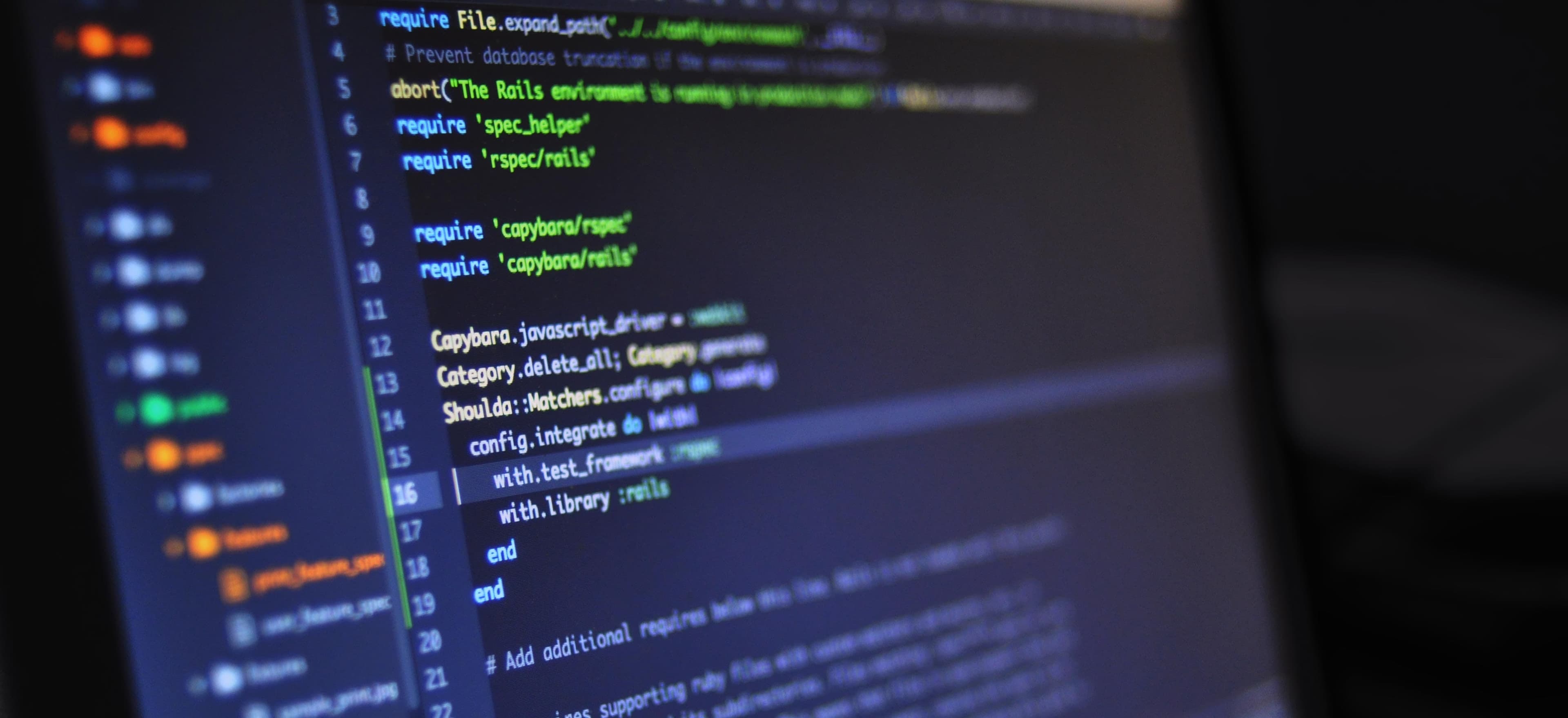
- Published on
Constructor vs. Method Injection: Choosing the Right Approach
In the world of software development, especially within the realm of Java, dependency injection is a vital concept. This design pattern helps create more modular, maintainable, and testable code by managing the dependencies of class instances. When implementing dependency injection, two primary approaches often arise: constructor injection and method injection. Understanding the difference between these two techniques is crucial to making informed architectural decisions. This blog post will explore both strategies, their advantages, and provide code snippets to illustrate their implementation.
What is Dependency Injection?
Dependency injection is a design pattern used in programming to achieve Inversion of Control (IoC) between classes and their dependencies. By using dependency injection, the initialization of a class is separated from its use, making the code less tightly coupled and easier to manage.
The Essence of Constructor Injection
Constructor injection is the process of providing dependencies to a class via its constructor. This approach requires that a class's dependencies be explicitly defined within the constructor parameters.
Example of Constructor Injection
Consider the following example of a simple Java service class that depends on a repository:
public class UserService {
private final UserRepository userRepository;
// Constructor Injection
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void registerUser(User user) {
userRepository.save(user);
}
}
Commentary on Constructor Injection
In this snippet, the UserService
class cannot function without a UserRepository
instance. By enforcing dependency injection through the constructor, we ensure that the class is always in a valid state upon instantiation. This approach has several benefits:
- Immutability: The
userRepository
field is marked final, ensuring that it cannot be changed after the instance is created. This promotes better design and helps avoid unintended side effects. - Required Dependencies: Any instance of
UserService
must provide aUserRepository
, making dependencies explicit and clear. - Testability: Constructor injection makes unit testing easier. We can easily pass a mock instance of
UserRepository
intoUserService
during tests.
For more in-depth insights on dependency injection, you can refer to this article on Dependency Injection in Java.
Understanding Method Injection
Method injection, also known as setter injection, involves providing dependencies through setter methods after a class has been instantiated. This approach allows for more flexibility in configuring dependencies.
Example of Method Injection
Here's a version of the previous service using method injection instead:
public class UserService {
private UserRepository userRepository;
// Setter Injection
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void registerUser(User user) {
if (userRepository == null) {
throw new IllegalStateException("UserRepository is not set.");
}
userRepository.save(user);
}
}
Commentary on Method Injection
In the method injection example, we see how dependencies can be set after instance creation. Here are the key points:
- Flexibility: This approach allows for more flexibility, especially in scenarios where dependencies may change frequently or need to be configured after instantiation.
- Optional Dependencies: Method injection supports optional dependencies. In cases where a dependency is not required, setter methods provide a clean way to configure or ignore it.
- Potential for Null State: The downside here is that it’s possible to have an instance of
UserService
without aUserRepository
, which can lead to runtime exceptions if overlooked.
Comparing Constructor Injection and Method Injection
| Aspect | Constructor Injection | Method Injection | |---------------------------|------------------------------------------|-------------------------------------| | Immutability | Strong, parameters must be supplied | Weak; can change after instantiation| | Dependency Requirement | All dependencies are required | Optional dependencies are possible | | Readability | Clear dependencies visible at a glance | Dependencies may not be obvious | | Testing | Easier to write unit tests | Requires additional checks | | Initialization State | Always initialized | May have default or null state |
When to Use Constructor Injection
Constructor injection is usually preferred when:
- The dependencies are mandatory for the class's functionality.
- You want to maintain immutability and control over your class state.
- You aim for cleaner, more testable code.
When to Use Method Injection
Method injection may be beneficial when:
- You require optional dependencies that may not always be necessary.
- The configuration of your class changes dynamically during the application lifecycle.
- You want to set up complex objects in a step-by-step manner.
Best Practices for Dependency Injection
-
Prefer Constructor Injection for Required Dependencies: Always opt for constructor injection when a class cannot function correctly without a particular dependency.
-
Limit Setter Injection Use: Restrict the use of method injection for optional dependencies to avoid creating instances that may be in an invalid state.
-
Decouple Configuration from Business Logic: Consider using a Dependency Injection Framework such as Spring to manage object creation and dependencies automatically, which can streamline the management of your application's components.
-
Document Dependencies: Always ensure that dependencies are well-documented, whether through code comments or external documentation, to assist in future development and maintenance.
A Final Look
Both constructor and method injection have their places in Java development. By understanding their strengths and weaknesses, you can choose the best method for your specific context. Remember to emphasize clear, testable, and maintainable code as your primary goals.
Whether you prefer the rigidity of constructor injection or the flexibility of method injection, the key takeaway is to use these patterns mindfully to ensure that your codebase remains easily navigable and resilient to change.
Make your decisions based on the specific needs of your application and adhere to good software engineering principles. Happy coding!