Mastering Couchbase: Common SQL Query Pitfalls
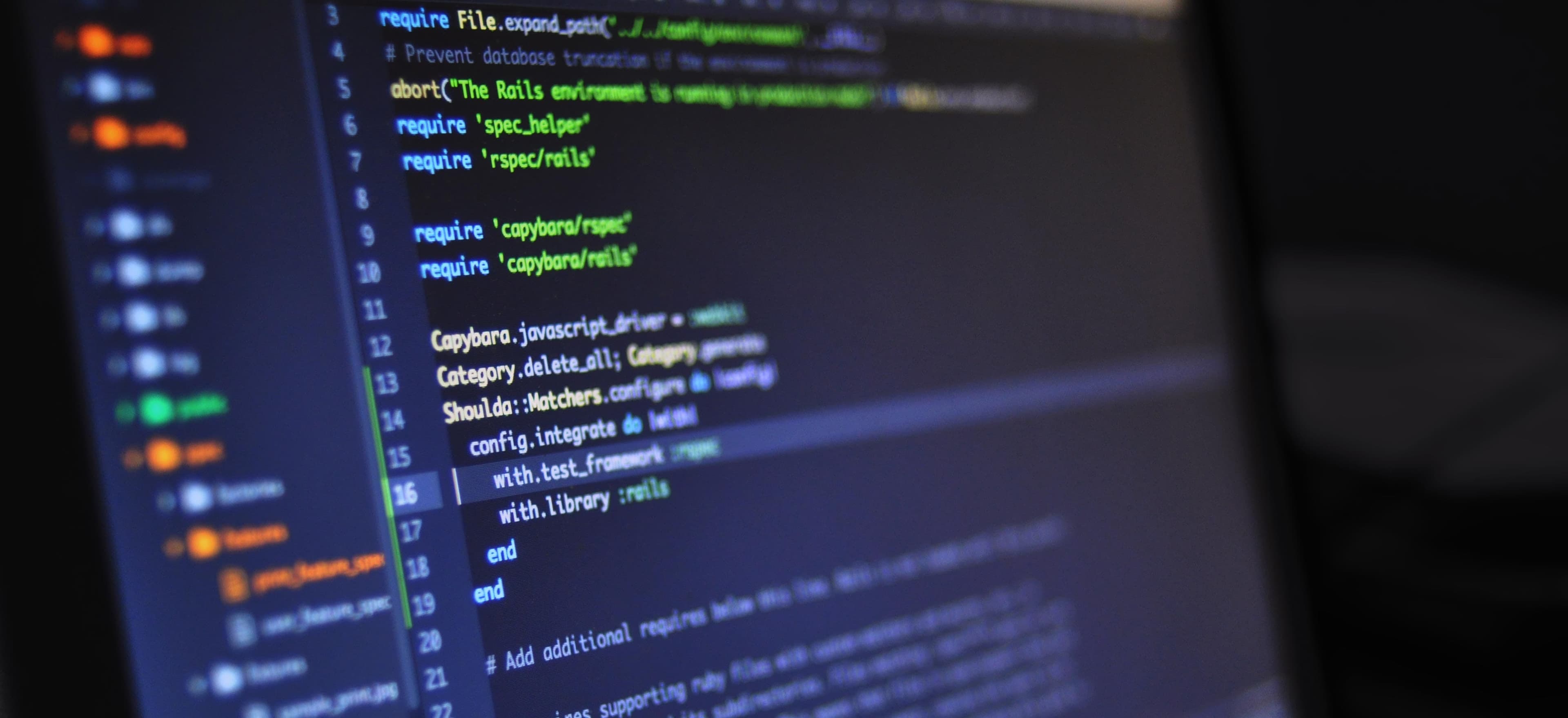
- Published on
Mastering Couchbase: Common SQL Query Pitfalls
Couchbase has gained prominence as a NoSQL database that combines the scalability of NoSQL with the expressive power of SQL. However, as developers venture into using N1QL (Couchbase’s SQL-like query language), they can run into common pitfalls that can hinder performance and lead to unexpected results. This blog post will explore some common SQL query pitfalls when using Couchbase, offering solutions, best practices, and code snippets with commentary on the rationale behind each practice.
Understanding N1QL
N1QL (pronounced "nickel") empowers developers to execute SQL-like queries on JSON documents. If you are familiar with SQL, you will find N1QL syntax quite intuitive, but the underlying structure of NoSQL databases is fundamentally different from relational databases. Thus, some standard SQL practices may not apply directly.
1. Ignoring Indexing
Pitfall: One of the most common mistakes developers make is neglecting to create indexes. A lack of indexes can lead to increased query execution time and resource consumption.
Solution: Always create an appropriate index for your queries.
Example: Consider a simple query to fetch users based on the email
field.
CREATE INDEX idx_email ON `bucket-name`(email);
Creating the index ensures that the query planner can optimize your query effectively. The index significantly reduces the time it takes to fetch results by pruning the documents it needs to examine.
2. Failing to Use Covering Indexes
Pitfall: A query that relies only on the primary index to answer questions can lead to full scans of the data.
Solution: Use covering indexes to include all the necessary fields in your index.
Example:
CREATE INDEX idx_covering ON `bucket-name`(email) INCLUDE (name, age);
By including additional fields in your index, Couchbase can return results directly from the index without accessing the documents. This can tremendously boost your query performance, especially with large datasets.
3. Using SELECT * Ineffectively
Pitfall: Using SELECT *
retrieves all fields and can lead to excessive data transfer and poor performance.
Solution: Only select the fields you need.
Example:
SELECT email, name FROM `bucket-name` WHERE age > 18;
This method reduces the amount of data sent across the network and speed up your queries by avoiding unnecessary data retrieval.
4. Unoptimized JOIN Operations
Pitfall: While Couchbase allows JOIN operations, performing them indiscriminately can lead to performance issues.
Solution: Always assess whether you can redesign your data model to minimize or eliminate the need for JOINs.
Example:
SELECT a.email, b.order_id
FROM `users` AS a
JOIN `orders` AS b ON a.user_id = b.user_id
WHERE a.active = TRUE;
If possible, denormalize your data. For instance, store order details directly in the user document if they are used together frequently.
5. Misunderstanding NULL Values
Pitfall: In SQL, NULL values can complicate queries, leading to unintended results.
Solution: Be explicit when checking for NULL values.
Example:
SELECT email FROM `bucket-name` WHERE last_login IS NULL;
By explicitly checking for NULL using IS NULL
, you avoid potential pitfalls associated with other logical conditions.
6. Inefficient Query Patterns
Pitfall: Writing queries that don't use the appropriate pattern can affect the system's performance.
Solution: Optimize your query patterns based on the nature of your data access.
Example: When querying for ranges, use a proper indexing strategy.
SELECT * FROM `bucket-name` WHERE age BETWEEN 18 AND 30;
Instead of a full table scan, ensure there's an index on the age
field to speed up retrieval.
7. Overlooking Query Options
Pitfall: Not utilizing the OPTIONS clause can lead to missed optimizations.
Solution: Use query options to control aspects of the query execution.
Example:
SELECT email FROM `bucket-name` USE INDEX (idx_email) WHERE age > 18;
In this case, you can tell the query to use a specific index, which may improve performance depending on your data distribution.
8. Querying Large Datasets Without Pagination
Pitfall: Retrieving large datasets all at once can cause memory issues and slow response times.
Solution: Implement pagination.
Example:
SELECT email FROM `bucket-name` LIMIT 10 OFFSET 0;
Using pagination helps manage memory usage while providing a manageable amount of data to clients.
9. Not Analyzing Query Performance
Pitfall: Failing to analyze performance can lead to persistent inefficiencies.
Solution: Utilize the Couchbase query analyzer.
Example:
EXPLAIN SELECT email FROM `bucket-name` WHERE age > 18;
Using EXPLAIN
allows you to see how Couchbase executes your queries. Understanding this can help you refine your indexes and queries.
10. Neglecting To Utilize FTS Capabilities
Pitfall: Assuming that N1QL alone suffices for full-text searches may limit your application.
Solution: Leverage Couchbase's Full Text Search (FTS) capabilities.
Example:
{
"indexType": "fulltext-index",
"name": "fts-index",
"sourceType": "gsi",
"sourceName": "bucket-name",
"index": {
"type": "fulltext",
"analyzers": ["standard"]
}
}
FTS indexes are specialized for text-search capabilities. They enable more complex search functionality that N1QL alone may not allow.
Bringing It All Together
Navigating the transition from relational databases to NoSQL and specifically Couchbase can be fraught with challenges. By avoiding these common SQL query pitfalls, you can ensure that your queries are optimized for performance and reliability. Remember, performance tuning is not a one-time action but an ongoing part of your development process. Regularly assess your database queries, leverage Couchbase's powerful indexing capabilities, and always keep learning about N1QL.
For further reading, check out the Couchbase N1QL documentation to deepen your understanding. Happy querying!
Checkout our other articles